PHP Class: fast find text (string) in files (recursively)
Scanning your server for specifies phrases
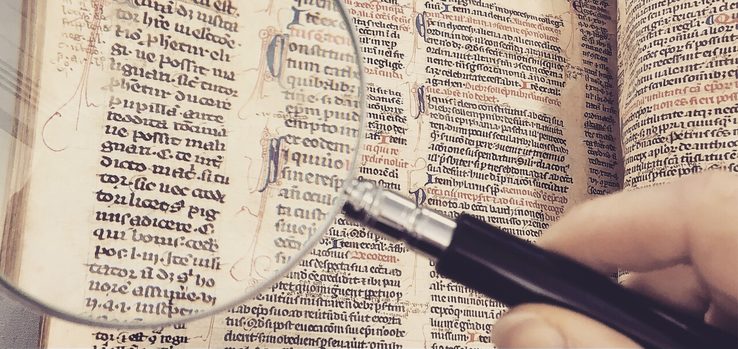
Use case 1: find all files with text "hello", but not "Hello"
include 'FindTxt.php';
$f = new FindTxt;
$f->caseSensitive = true; //find "hello", but not "Hello" (case sensitive search)
$f->formats = array();
$res = $f->find('directory', 'hello');
print_r($res);
Use case 2: find all files with text "hello" but also "Hello"
include 'FindTxt.php';
$f = new FindTxt;
$f->caseSensitive = false; //find "hello" and "Hello" (case insensitive search)
$f->formats = array();
$res = $f->find('directory', 'hello');
print_r($res);
Use case 3: find all files with text "hello" but also "Hello"; ignore GIF, JPEG and PNG files
include 'FindTxt.php';
$f = new FindTxt;
$f->caseSensitive = false; //find "hello" and "Hello" (case insensitive search)
$f->excludeMode = true;
$f->formats = array('.gif', '.jpg', '.jpeg', '.jpe', '.png'); //exclude these files from search
$res = $f->find('directory', 'hello');
print_r($res);
Use case 4: find all .TXT and .XML files with text "hello" but also "Hello"
include 'FindTxt.php';
$f = new FindTxt;
$f->caseSensitive = false; //find "hello" and "Hello" (case insensitive search)
$f->excludeMode = false;
$f->formats = array('.txt', '.xml'); //include only these files in search
$res = $f->find('directory', 'hello');
print_r($res);
Licensed under MIT. Click below to download and please leave a comment!
Comments