How to convert mp3 to mp4 or flac?
Two simple ways to convert audio files under PHP
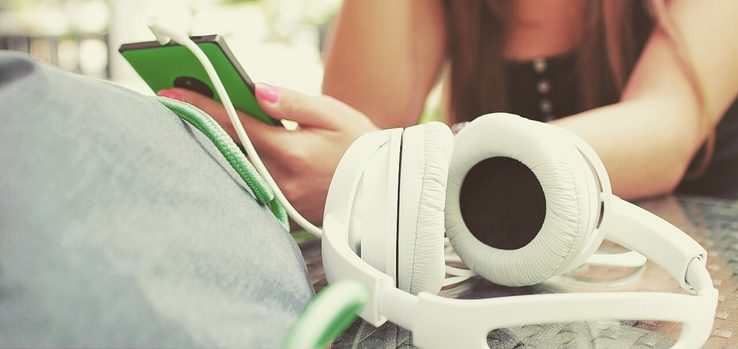
Installing ffmpeg
You can install it under Debian 9 with:
apt-get install ffmpeg
For Debian 8 it's a bit more complicated.
First add add the following line to the bottom of /etc/apt/sources.list
deb http://ftp.uk.debian.org/debian jessie-backports main
Then update apt and install ffmpeg:apt-get update
apt-get install ffmpeg
Under Windows just visit:
https://ffmpeg.zeranoe.com/builds/
and download a binary.If you have OSX and Homebrew type:
brew install ffmpeg
Converting with ffmpeg
Usage is very simple:
ffmpeg -i input.flac -ar 44100 -ab 128k output.mp3
-i input.flac is your input file
-ab 128k is the bitrate
-ar 44100 is the sample rate
You can always just let ffmpeg use default values:
ffmpeg -i input.flac output.wav
Using REST API for conversion
If you can't install ffmpeg or don't want to use it then there is an API:
http://api.rest7.com/v1/sound_convert.php?format=mp3&url=http://yourserver.com/yourfile.wav
Instead of using a URL to your input file you can also send it via HTTP POST. Sample PHP code:
$post = array(
'file' => new CurlFile('input.flac'),
'format' => 'mp3', //specify output format, eg. mp3, wav, flac
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'http://api.rest7.com/v1/sound_convert.php');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post);
$data = curl_exec($ch);
curl_close($ch);
$json = @json_decode($data);
if ($json->success)
{
$res = file_get_contents($json->file);
file_put_contents('output.mp3', $res);
}
else
{
echo 'An error occured';
}
Comments