Import Excel (XLS) document into MySQL database
Another straight to the point solution
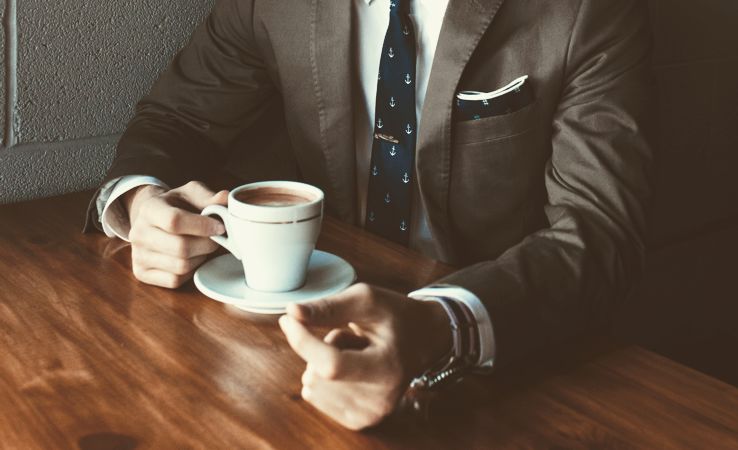
And here's an example how to read an XLS file and create SQL queries for MySQL database:
include 'Excel/reader.php';
$data = new Spreadsheet_Excel_Reader();
$data->setOutputEncoding('CP1251');
$data->read('jxlrwtest.xls');
//columns:
$sql = "INSERT INTO `table` (";
for ($j = 2; $j <= $data->sheets[0]['numCols']; $j++)
{
$sql .= "`" . mysql_escape_string($data->sheets[0]['cells'][1][$j]) . "`,";
}
$sql = substr($sql, 0, -1) . ") VALUES\r\n";
//cells
for ($i = 2; $i <= $data->sheets[0]['numRows']; $i++)
{
$sql .= "(";
for ($j = 2; $j <= $data->sheets[0]['numCols']; $j++)
{
$sql .= "'" . mysql_escape_string($data->sheets[0]['cells'][$i][$j]) . "',";
}
$sql = substr($sql, 0, -1) . "),\r\n";
}
$sql = substr($sql, 0, -3) . ";";
echo '<pre>';
echo $sql;
[code]
It will output something like this:
[code]
INSERT INTO `table` (`First`,`Middle`,`Last`,`Email`) VALUES
('Jane','H','Kowalski','jane@kowalski.com'),
('Michael','J','Canne','michael.canne@yahoo.com');
Comments