How to make website load really fast?
Lots of tips and tools for website optimizations
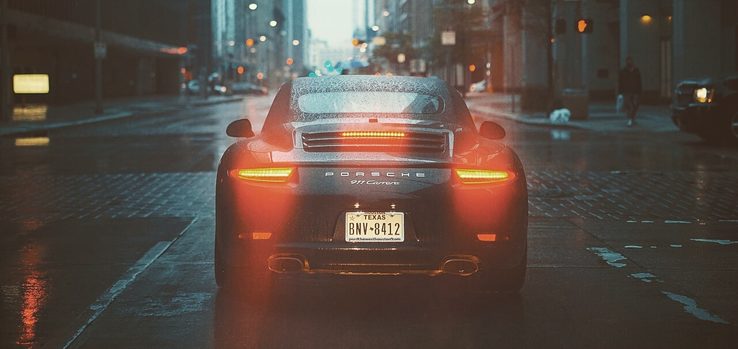
Minimize number of HTTP requests
HTTP requests are expensive. Especially for mobile users who have high pings. How can your website make less requests? Try to concatenate your files. Here's a few things you can do.
Concatenate multiple CSS files into 1 file
You can do this manually or you can create a simple PHP to do so. For example- keep all your CSS files inside "css" folder and create script css.php like this:<?php
$all = '';
foreach (glob('css/*.css') AS $css)
{
$all .= file_get_contents($css);
}
file_put_contents('styles.css', $all);
This simple script grabs all CSS files from "css" folder and saves as "styles.css".Merge multiple Javascript files into a single file
You can do this using similar approach to do one above. Put all your .js files inside "js" directory and create "js.php" file with this content:<?php
$all = '';
foreach (glob('js/*.js') AS $js)
{
$all .= file_get_contents($js);
}
file_put_contents('scripts.js', $all);
Put Javascripts and fonts on your server
Serving Javascripts and fonts from popular CDNs is tempting. But it requires your browser to resolve more DNS names. Having them on your server not only speeds-up loading but also you can try to optimize the files. For example you can minimize JavaScript files if they are not minimized, or you can convert fonts to another format. For example WOFF not only has wider support than TTF/OTF but also is just smaller. You can convert your TTF and OTF fonts to WOFF with FontSquirrel.Consider different JavaScript libraries
For example jQuery is a great library, but it's over 90 kB (minimized) and takes some serious time to parse. There are lightweight alternatives. Although they don't offer full compatibility they are over 10 times smaller and often works faster. You can read more about alternatives to jQuery here.Optimize fonts
If your website is in English then you don't need other alphabets in your fonts. You can strip unnecessary symbols from your fonts with FontSquirrel.Minimize CSS and JavaScript files
Minification is basically stripping all the whitespaces and comments which are not needed for your code to work. You can get free JS & CSS minificatators here.Inline fonts
You can put your whole fonts inside your CSS files. Instead of linking to font files, like that:@font-face
{
font-family: 'MyFont';
src: url(MyFont.woff) format('woff'),
}
You can base64 encode your file:@font-face
{
font-family: 'MyFont';
src: url(data:application/x-font-woff;charset=utf-8;base64,d09GRgABAAAAAHwwABMAAA...) format('woff'),
}
How to do that in PHP? @font-face
{
font-family: 'MyFont';
src: url(data:application/x-font-woff;charset=utf-8;base64,<?php echo base64_encode(file_get_contents('MyFont.woff')) ?>) format('woff'),
}
Inline CSS and JavaScripts
That's a simple thing. Change your scripts from:<link rel="stylesheet" type="text/css" href="styles.css">
<script type="text/javascript" src="functions.js"></script>
to:<style type="text/css">
//place contents of file styles.css in here
</style>
<script type="text/javascript">
//place contents of file functions.js in here
</script>
If you use PHP on your server you can achive it this way:
<style type="text/css">
<?php include 'styles.css' ?>
</style>
<script type="text/javascript">
<?php include 'functions.css' ?>
</script>
or that way:
<style type="text/css">
<?php echo file_get_contents('styles.css') ?>
</style>
<script type="text/javascript">
<?php echo file_get_contents('functions.css') ?>
</script>
Join multiple icons into one file
Let's say you have 2 icons in PNG format, each one is 40x40 pixels. You can create 1 PNG image of size 40x80 or 80x40 and put your both icons in it, one next to another. In order to display them properly on your page you can use background-position, eg:<div class="icon icon1"></div>
<div class="icon icon2"></div>
<style>
.icon
{
height: 40px;
width: 40px;
background-image: url('icons.png');
}
.icon1
{
background-position: 0 40px;
}
.icon2
{
background-position: 0 0;
}
</style>
Here's my online tool you can try to join your images into one sprite.Inline images: img and background-image
Instead of linking to image files from your HTML:<img src="myimage.png">
you can put entire image in your HTML code:<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhE...">
Again you can make your life easier with a bit of PHP:<img src="data:image/png;base64,<?php echo base64_encode(file_get_contents('myimage.png')) ?>">
Cache your pages to plain HTML
Landing pages of your website are visited more often than others. It can speed your website a lot if you cache them to HTML instead of running through PHP every time. For example:- rename your index.php to index_dynamic.php
- create a cronjob script to call index_dynamic.php, get output HTML code and save as index.html
$htm = file_get_contents('http://your_server.com/index_dynamic.php');
file_put_contents('index.html', $htm);
Cache your ajax calls
Perhaps instead of serving some dynamic content, for example taken from MySQL database, you could save the requests ever hour to .json files? Or even move some of the Ajax actions into pure JavaScript. For example instead of using AJAX to do some calculations with PHP server-side you could do these calculations in JavaScript?Use static gzip files
Sending GZIP compressed JS, CSS and HTML code to your clients can save quite some bandwidth and time. While gzipping on the fly is possible and often used it's not as effective as serving already gzipped files. Serving static gzip files saves a lot of CPU power but also makes it possible to achieve smaller sizes (higher compression). You can cache parts of your website to static JS, CSS and HTML files, then gzip them and send pregzipped files to your visitors. For example- put your css files inside "css" directory and create a ".htaccess" file inside that directory with this content:Header set Expires "Tue, 16 Jun 2030 20:00:00 GMT"
Header set Cache-Control "public"
Header set Content-Type "text/css"
Header set Content-Encoding "gzip"
Header unset Pragma
Header unset ETag
FileETag None
It requires "headers_module" to be installed in Apache.Compress your images further
Websites today are filled with images and images take a lot more space (and bandwidth) than text. Optimizing your images can often make them up to 50% smaller without reducing quality and resolution. Of course if you want to reduce resolution then you can save even more space.
Compress JPEG images
How to shink your JPEGS?- If your JPEG is black&white you can save in grayscale instead of truecolor
- Strip all metadata, including image thumbnail
- Try to recompress with mozjpeg using levels as low as possible. It's a very efficient encoder and can make your files smaller without worsening the quality in a noticeable way
Optimize PNG images
You can make your PNG images smaller if you:- Reduce number of colors
- Compress them using zopfli compression algorithm instead of deflate
- Try multiple filter combinations
- Alter invisible pixels behind alpha channel
Reduce size of GIF images
What you can do with your GIF images? Consider reducing resolution and number of colors. Most static GIF images will be smaller when converted to PNG and they won't loose any quality. Every pixel will stay intact, including transparency. Of course as long as you use the right tool.If your GIF is animated you might reduce its framerate, for example remove every second frame to convert image from 30 fps to 15 fps, or convert it to MP4/WEBM. Videos in MP4 and WEBM formats are widely supported by browsers and they take up a lot less space than GIFs. They don't, unfortunately, support transparency.
To convert gif to mp4 you can use ffmpeg (free, open source tool):
ffmpeg -i input.gif output.mp4
Comments