How to get HTTP headers of any remote file?
Another straight to the point solution
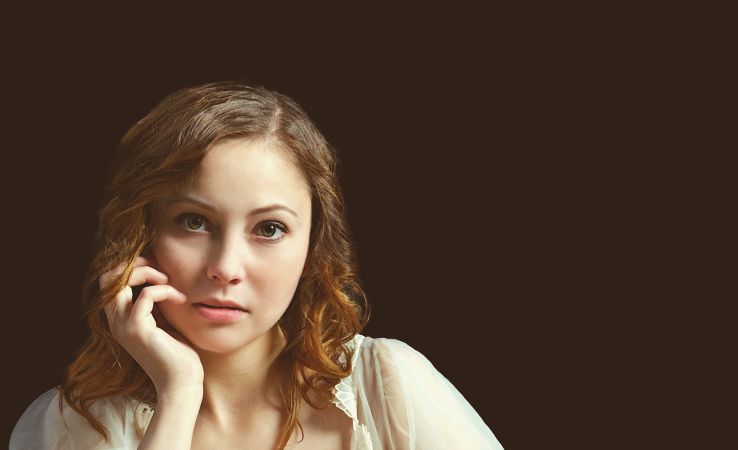
Then you should just request headers and not the whole file from a remote server using the HTTP protocol.
Here's how to do this using fsockopen:
function getHeaders($url)
{
$path = parse_url($url);
if (isset($path['query']))
{
$path['path'] .= '?' . $path['query'];
}
if (!isset($path['port']))
{
$path['port'] = 80;
}
$request = "HEAD " . $path['path']. " HTTP/1.1\r\n";
$request .= "Host: " . $path['host'] . "\r\n";
$request .= "Content-type: application/x-www-form-urlencoded\r\n";
$request .= "Cache-Control: no-cache\r\n";
$request .= "User-Agent: MSIE\r\n";
$request .= "Connection: close\r\n";
$request .= "\r\n";
$f = fsockopen($path['host'], $path['port'], $errno, $errstr);
if ($f)
{
fputs($f, $request);
while (!feof($f))
{
$headers[] = fgets($f);
}
fclose($f);
}
return $headers;
}
You might also consider using cURL for this job:
function getHeadersCURL($url)
{
$user_agent = 'MSIE';
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HEADER, 1);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'HEAD');
curl_setopt($ch, CURLOPT_USERAGENT, $user_agent);
curl_setopt($ch, CURLOPT_TIMEOUT, 3);
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 3);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1);
$result = curl_exec($ch);
curl_close($ch);
$headers = array();
$result = explode("\r", $result);
if (count($result)>0)
foreach ($result AS $line)
{
$headers[] = $line;
}
return $headers;
}
How to use it?
$headers = getHeaders('http://google.com');
print_r($headers);
Comments