Few very useful PHP functions, part 2
Another straight to the point solution
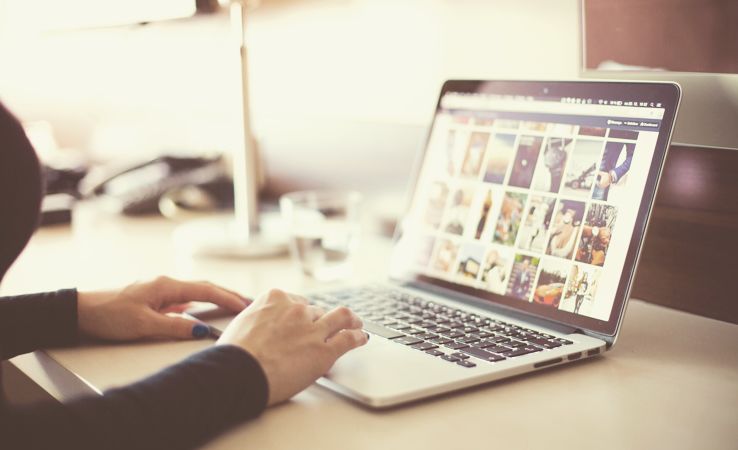
I think you might find them useful too. If you do- please leave a comment, thanks!
Unique file name
This function returns numerical name for a file with given extension in a given directory that does not exist. It can be used as a name for temporary files, like uploads and such. If you call it like uniqFile('upload', '.jpg') it will return something like '1.jpg' or '15523.jpg'.
function uniqFile($dir, $ext)
{
if (substr($dir, -1, 1) != '/')
{
$dir .= '/';
}
for ($i=1; $i<999999; $i++)
{
if (!is_file($dir . $i . $ext))
{
return $i . $ext;
}
}
return false;
}
Human size
This functions returns human-readable size of the file. It's only parameter is size in bytes. humanSize(1030) will return '1 kB'.
function humanSize($size)
{
if ($size < 1024) return sprintf('%d B', $size);
if ($size < 1048576) return sprintf('%.2f kB', $size/1024);
if ($size < 1073741824) return sprintf('%.2f MB', $size/1048576);
if ($size < 1099511627776) return sprintf('%.2f GB', $size/1073741824);
}
To Title
Converts letter case of a given string- every word's first letter becomes uppercase.
function toTitle($title)
{
return ucwords(strtolower($title));
}
Hex to String, String to Hex
These functions convert given hex string into binary string.
function hex2str($s)
{
$s = str_replace(' ', '', $s);
$len = strlen($s);
$out = '';
for ($i=0; $i<$len; $i+=2)
{
$out .= chr(hexdec(substr($s, $i, 2)));
}
return $out;
}
function str2hex($s)
{
$len = strlen($s);
$out = '';
for ($i=0; $i<$len; $i++)
{
$out .= dechex(ord($s[$i])) . ' ';
}
return $out;
}
Comments