Few very useful PHP functions
Open source functions to make your life easier
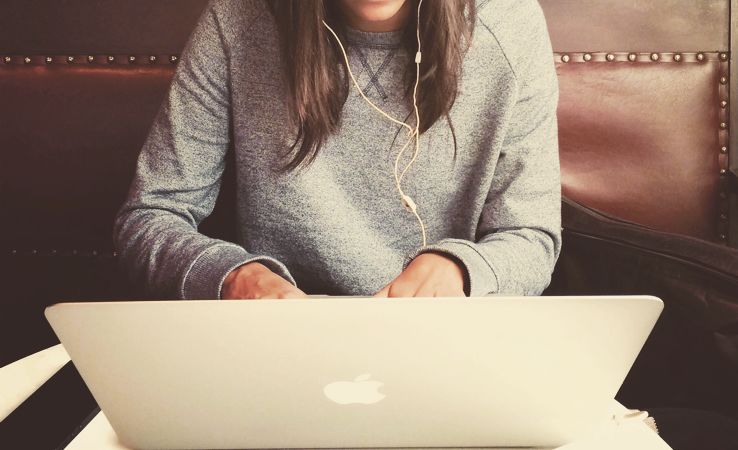
The functions are not super-advanced, but really handy. You can use them freely as they all are licensed under MIT.
I think there is a big chance you will find them useful too. If you do- please leave a comment, thanks!
Nice date
This function shows date formatted as "Y-m-d" for dates older than today or "today H:i" for today's dates.
function niceDate($date)
{
if (substr($date, 0, 10) == date('Y-m-d'))
{
return 'today, ' . substr($date, 11, 5);
}
return substr($date, 0, 10);
}
File icon
Here's a function which returns image icon for a set of predefined file types (based on extension).
function makeIcon($file)
{
$ico = substr($file, strrpos($file, '.'));
switch ($ico)
{
case 'pdf':
case 'doc':
case 'rtf': $icon = 'document.png'; break;
case 'zip':
case 'rar':
case '7z' :
case 'tar':
case 'gz' : $icon = 'archive.png'; break;
default : $icon = 'default.png'; break;
}
return $icon;
}
Shorten text
Make text shorten, but don't cut words!
function shorten($str, $len = 50)
{
$cut = "\x1\x2\x3";
$str = strip_tags($str);
list($str) = explode($cut, wordwrap($str, $len, $cut));
return $str;
}
List files in a directory
This function lists files in a directory. It ignores subdirectories.
function scandir2($dir)
{
$out = array();
if (is_dir($dir))
{
if ($dh = opendir($dir))
{
while (($file = readdir($dh)) !== false)
{
if ($file == '.' or $file == '..') continue;
if (!is_dir($dir . '/'. $file))
{
$out[] = $dir . '/' . $file;
}
}
closedir($dh);
}
}
sort($out);
return $out;
}
Return next value from a set of values
Given a set of values it returns next value each time it is called. Example:
cycle('one','two'); cycle('one','two'); cycle('one','two');
will print 'one', then 'two', then 'one'.
Can be used in HTML templates:
<?php for ($i=0; $i<5; $i++) { ?>
<div style="color: <?php cycle('red','white','blue') ?>">Text here</div>
<?php } ?>
function cycle()
{
global $cycleCounter;
$cycleCounter = isset($cycleCounter) ? $cycleCounter+1 : 0;
$cycleCounter = $cycleCounter % func_num_args();
echo func_get_arg($cycleCounter);
}
Comments