Currency converter (eg. USD to EUR)
A simple step-by-step guide which will take less than 5 minutes
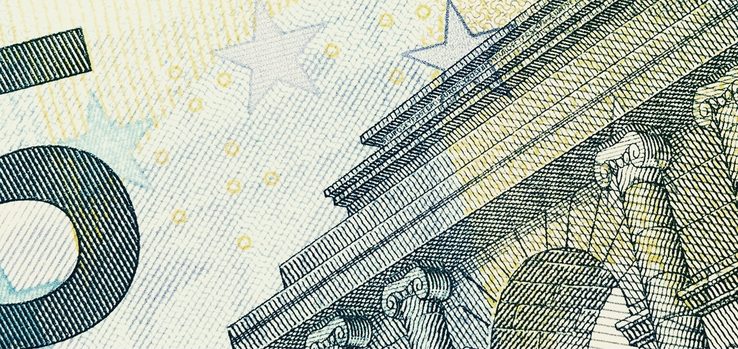
<?php
$amount = '123.45';
$fromCurrency = 'EUR';
$toCurrency = 'USD';
$data = json_decode(file_get_contents('http://api.rest7.com/v1/currency_convert.php?amount=' . $amount . '¤cy_in=' . $fromCurrency . '¤cy_out=' . $toCurrency));
if (@$data->success !== 1)
{
die('Failed');
}
echo $data->amount . ' ' . $data->currency_out;
The code above works just fine but every time we want to convert between currencies we need to use external REST API. We can do this a lot faster if we create conversion tables.Let's say we just want to convert from EUR to USD, USD to EUR and USD to GBP:
<?php
$amount = '1000';
$tables = array();
$tables['EUR']['USD'] = 0;
$tables['USD']['EUR'] = 0;
$tables['USD']['GBP'] = 0;
foreach ($tables AS $fromCurrency => &$toCurrencies)
foreach ($toCurrencies AS $toCurrency => &$val)
{
$data = json_decode(file_get_contents('http://api.rest7.com/v1/currency_convert.php?amount=1000' .
'¤cy_in=' . $fromCurrency .
'¤cy_out=' . $toCurrency));
if (@$data->success !== 1) continue;
$val = $data->amount/1000;
}
file_put_contents('cache.json', json_encode($tables));
We can add this script to cron and let it run once a day. It will save conversion tables into "cache.json". So how to use this cache?
$table = json_decode(file_get_contents('cache.json'));
//converting 123.45 USD to EUR:
echo 123.45 * $table->USD->EUR . ' EUR';
Comments