(Almost) All PHP functions with simple explanations in one place
Another straight to the point solution
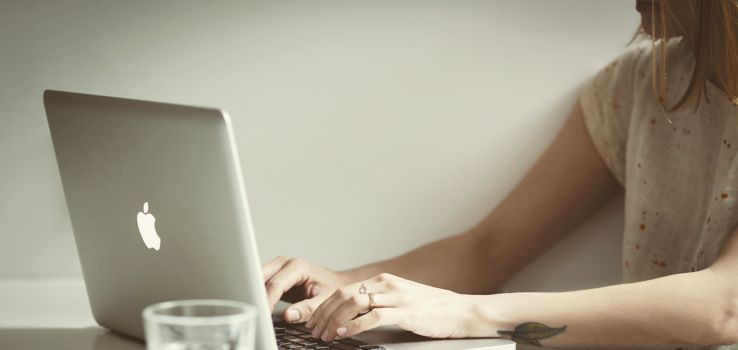
AMQP
AMQPConnection::__construct - Create an instance of AMQPConnection
AMQPConnection::isConnected - Determine if the AMQPConnection object is still connected to the broker.
AMQPExchange::bind - The bind purpose
AMQPExchange::__construct - Create an instance of AMQPExchange
AMQPExchange::declare - Declare a new exchange on the broker.
AMQPExchange::delete - Delete the exchange from the broker.
AMQPExchange::publish - Publish a message to an exchange.
AMQPQueue::ack - Acknowledge the receipt of a message
AMQPQueue::bind - Bind the given queue to a routing key on an exchange.
AMQPQueue::cancel - Cancel a queue binding.
AMQPQueue::__construct - Create an instance of an AMQPQueue object.
AMQPQueue::consume - The consume purpose
AMQPQueue::declare - Declare a new queue
AMQPQueue::delete - Delete a queue and its contents.
AMQPQueue::get - Retrieve the next message from the queue.
AMQPQueue::purge - Purge the contents of a queue
AMQPQueue::unbind - Unbind the queue from a routing key.
Apache
apache_child_terminate - Terminate apache process after this request
apache_get_modules - Get a list of loaded Apache modules
apache_get_version - Fetch Apache version
apache_getenv - Get an Apache subprocess_env variable
apache_lookup_uri - Perform a partial request for the specified URI and return all info about it
apache_note - Get and set apache request notes
apache_request_headers - Fetch all HTTP request headers
apache_reset_timeout - Reset the Apache write timer
apache_response_headers - Fetch all HTTP response headers
apache_setenv - Set an Apache subprocess_env variable
getallheaders - Fetch all HTTP request headers
virtual - Perform an Apache sub-request
Alternative PHP Cache
apc_add - Cache a variable in the data store
apc_bin_dump - Get a binary dump of the given files and user variables
apc_bin_dumpfile - Output a binary dump of cached files and user variables to a file
apc_bin_load - Load a binary dump into the APC file/user cache
apc_bin_loadfile - Load a binary dump from a file into the APC file/user cache
apc_cache_info - Retrieves cached information from APC's data store
apc_clear_cache - Clears the APC cache
apc_compile_file - Stores a file in the bytecode cache, bypassing all filters.
apc_dec - Decrease a stored number
apc_define_constants - Defines a set of constants for retrieval and mass-definition
apc_delete_file - Deletes files from the opcode cache
apc_delete - Removes a stored variable from the cache
apc_exists - Checks if APC key exists
apc_fetch - Fetch a stored variable from the cache
apc_inc - Increase a stored number
apc_load_constants - Loads a set of constants from the cache
apc_sma_info - Retrieves APC's Shared Memory Allocation information
apc_store - Cache a variable in the data store
APCIterator::__construct - Constructs an APCIterator iterator object
APCIterator::current - Get current item
APCIterator::getTotalCount - Get total count
APCIterator::getTotalHits - Get total cache hits
APCIterator::getTotalSize - Get total cache size
APCIterator::key - Get iterator key
APCIterator::next - Move pointer to next item
APCIterator::rewind - Rewinds iterator
APCIterator::valid - Checks if current position is valid
Advanced PHP debugger
apd_breakpoint - Stops the interpreter and waits on a CR from the socket
apd_callstack - Returns the current call stack as an array
apd_clunk - Throw a warning and a callstack
apd_continue - Restarts the interpreter
apd_croak - Throw an error, a callstack and then exit
apd_dump_function_table - Outputs the current function table
apd_dump_persistent_resources - Return all persistent resources as an array
apd_dump_regular_resources - Return all current regular resources as an array
apd_echo - Echo to the debugging socket
apd_get_active_symbols - Get an array of the current variables names in the local scope
apd_set_pprof_trace - Starts the session debugging
apd_set_session_trace_socket - Starts the remote session debugging
apd_set_session_trace - Starts the session debugging
apd_set_session - Changes or sets the current debugging level
override_function - Overrides built-in functions
rename_function - Renames orig_name to new_name in the global function table
Arrays
array_change_key_case - Changes all keys in an array
array_chunk - Split an array into chunks
array_combine - Creates an array by using one array for keys and another for its values
array_count_values - Counts all the values of an array
array_diff_assoc - Computes the difference of arrays with additional index check
array_diff_key - Computes the difference of arrays using keys for comparison
array_diff_uassoc - Computes the difference of arrays with additional index check which is performed by a user supplied callback function
array_diff_ukey - Computes the difference of arrays using a callback function on the keys for comparison
array_diff - Computes the difference of arrays
array_fill_keys - Fill an array with values, specifying keys
array_fill - Fill an array with values
array_filter - Filters elements of an array using a callback function
array_flip - Exchanges all keys with their associated values in an array
array_intersect_assoc - Computes the intersection of arrays with additional index check
array_intersect_key - Computes the intersection of arrays using keys for comparison
array_intersect_uassoc - Computes the intersection of arrays with additional index check, compares indexes by a callback function
array_intersect_ukey - Computes the intersection of arrays using a callback function on the keys for comparison
array_intersect - Computes the intersection of arrays
array_key_exists - Checks if the given key or index exists in the array
array_keys - Return all the keys or a subset of the keys of an array
array_map - Applies the callback to the elements of the given arrays
array_merge_recursive - Merge two or more arrays recursively
array_merge - Merge one or more arrays
array_multisort - Sort multiple or multi-dimensional arrays
array_pad - Pad array to the specified length with a value
array_pop - Pop the element off the end of array
array_product - Calculate the product of values in an array
array_push - Push one or more elements onto the end of array
array_rand - Pick one or more random entries out of an array
array_reduce - Iteratively reduce the array to a single value using a callback function
array_replace_recursive - Replaces elements from passed arrays into the first array recursively
array_replace - Replaces elements from passed arrays into the first array
array_reverse - Return an array with elements in reverse order
array_search - Searches the array for a given value and returns the corresponding key if successful
array_shift - Shift an element off the beginning of array
array_slice - Extract a slice of the array
array_splice - Remove a portion of the array and replace it with something else
array_sum - Calculate the sum of values in an array
array_udiff_assoc - Computes the difference of arrays with additional index check, compares data by a callback function
array_udiff_uassoc - Computes the difference of arrays with additional index check, compares data and indexes by a callback function
array_udiff - Computes the difference of arrays by using a callback function for data comparison
array_uintersect_assoc - Computes the intersection of arrays with additional index check, compares data by a callback function
array_uintersect_uassoc - Computes the intersection of arrays with additional index check, compares data and indexes by a callback functions
array_uintersect - Computes the intersection of arrays, compares data by a callback function
array_unique - Removes duplicate values from an array
array_unshift - Prepend one or more elements to the beginning of an array
array_values - Return all the values of an array
array_walk_recursive - Apply a user function recursively to every member of an array
array_walk - Apply a user function to every member of an array
array - Create an array
arsort - Sort an array in reverse order and maintain index association
asort - Sort an array and maintain index association
compact - Create array containing variables and their values
count - Count all elements in an array, or properties in an object
current - Return the current element in an array
each - Return the current key and value pair from an array and advance the array cursor
end - Set the internal pointer of an array to its last element
extract - Import variables into the current symbol table from an array
in_array - Checks if a value exists in an array
key - Fetch a key from an array
krsort - Sort an array by key in reverse order
ksort - Sort an array by key
list - Assign variables as if they were an array
natcasesort - Sort an array using a case insensitive "natural order" algorithm
natsort - Sort an array using a "natural order" algorithm
next - Advance the internal array pointer of an array
pos - Alias of current
prev - Rewind the internal array pointer
range - Create an array containing a range of elements
reset - Set the internal pointer of an array to its first element
rsort - Sort an array in reverse order
shuffle - Shuffle an array
sizeof - Alias of count
sort - Sort an array
uasort - Sort an array with a user-defined comparison function and maintain index association
uksort - Sort an array by keys using a user-defined comparison function
usort - Sort an array by values using a user-defined comparison function
Bulletin Board Code
bbcode_add_element - Adds a bbcode element
bbcode_add_smiley - Adds a smiley to the parser
bbcode_create - Create a BBCode Resource
bbcode_destroy - Close BBCode_container resource
bbcode_parse - Parse a string following a given rule set
bbcode_set_arg_parser - Attach another parser in order to use another rule set for argument parsing
bbcode_set_flags - Set or alter parser options
BCMath Arbitrary Precision Mathematics
bcadd - Add two arbitrary precision numbers
bccomp - Compare two arbitrary precision numbers
bcdiv - Divide two arbitrary precision numbers
bcmod - Get modulus of an arbitrary precision number
bcmul - Multiply two arbitrary precision number
bcpow - Raise an arbitrary precision number to another
bcpowmod - Raise an arbitrary precision number to another, reduced by a specified modulus
bcscale - Set default scale parameter for all bc math functions
bcsqrt - Get the square root of an arbitrary precision number
bcsub - Subtract one arbitrary precision number from another
PHP bytecode Compiler
bcompiler_load_exe - Reads and creates classes from a bcompiler exe file
bcompiler_load - Reads and creates classes from a bz compressed file
bcompiler_parse_class - Reads the bytecodes of a class and calls back to a user function
bcompiler_read - Reads and creates classes from a filehandle
bcompiler_write_class - Writes an defined class as bytecodes
bcompiler_write_constant - Writes a defined constant as bytecodes
bcompiler_write_exe_footer - Writes the start pos, and sig to the end of a exe type file
bcompiler_write_file - Writes a php source file as bytecodes
bcompiler_write_footer - Writes the single character \x00 to indicate End of compiled data
bcompiler_write_function - Writes an defined function as bytecodes
bcompiler_write_functions_from_file - Writes all functions defined in a file as bytecodes
bcompiler_write_header - Writes the bcompiler header
bcompiler_write_included_filename - Writes an included file as bytecodes
Bzip2
bzclose - Close a bzip2 file
bzcompress - Compress a string into bzip2 encoded data
bzdecompress - Decompresses bzip2 encoded data
bzerrno - Returns a bzip2 error number
bzerror - Returns the bzip2 error number and error string in an array
bzerrstr - Returns a bzip2 error string
bzflush - Force a write of all buffered data
bzopen - Opens a bzip2 compressed file
bzread - Binary safe bzip2 file read
bzwrite - Binary safe bzip2 file write
Cairo
cairo_create - Returns a new CairoContext object on the requested surface.
cairo_font_face_get_type - Description
cairo_font_face_status - Description
cairo_font_options_create - Description
cairo_font_options_equal - Description
cairo_font_options_get_antialias - Description
cairo_font_options_get_hint_metrics - Description
cairo_font_options_get_hint_style - Description
cairo_font_options_get_subpixel_order - Description
cairo_font_options_hash - Description
cairo_font_options_merge - Description
cairo_font_options_set_antialias - Description
cairo_font_options_set_hint_metrics - Description
cairo_font_options_set_hint_style - Description
cairo_font_options_set_subpixel_order - Description
cairo_font_options_status - Description
cairo_format_stride_for_width - Description
cairo_image_surface_create_for_data - Description
cairo_image_surface_create_from_png - Description
cairo_image_surface_create - Description
cairo_image_surface_get_data - Description
cairo_image_surface_get_format - Description
cairo_image_surface_get_height - Description
cairo_image_surface_get_stride - Description
cairo_image_surface_get_width - Description
cairo_matrix_create_scale - Alias of CairoMatrix::initScale
cairo_matrix_create_translate - Alias of CairoMatrix::initTranslate
cairo_matrix_invert - Description
cairo_matrix_multiply - Description
cairo_matrix_rotate - Description
cairo_matrix_transform_distance - Description
cairo_matrix_transform_point - Description
cairo_matrix_translate - Description
cairo_pattern_add_color_stop_rgb - Description
cairo_pattern_add_color_stop_rgba - Description
cairo_pattern_create_for_surface - Description
cairo_pattern_create_linear - Description
cairo_pattern_create_radial - Description
cairo_pattern_create_rgb - Description
cairo_pattern_create_rgba - Description
cairo_pattern_get_color_stop_count - Description
cairo_pattern_get_color_stop_rgba - Description
cairo_pattern_get_extend - Description
cairo_pattern_get_filter - Description
cairo_pattern_get_linear_points - Description
cairo_pattern_get_matrix - Description
cairo_pattern_get_radial_circles - Description
cairo_pattern_get_rgba - Description
cairo_pattern_get_surface - Description
cairo_pattern_get_type - Description
cairo_pattern_set_extend - Description
cairo_pattern_set_filter - Description
cairo_pattern_set_matrix - Description
cairo_pattern_status - Description
cairo_pdf_surface_create - Description
cairo_pdf_surface_set_size - Description
cairo_ps_get_levels - Description
cairo_ps_level_to_string - Description
cairo_ps_surface_create - Description
cairo_ps_surface_dsc_begin_page_setup - Description
cairo_ps_surface_dsc_begin_setup - Description
cairo_ps_surface_dsc_comment - Description
cairo_ps_surface_get_eps - Description
cairo_ps_surface_restrict_to_level - Description
cairo_ps_surface_set_eps - Description
cairo_ps_surface_set_size - Description
cairo_scaled_font_create - Description
cairo_scaled_font_extents - Description
cairo_scaled_font_get_ctm - Description
cairo_scaled_font_get_font_face - Description
cairo_scaled_font_get_font_matrix - Description
cairo_scaled_font_get_font_options - Description
cairo_scaled_font_get_scale_matrix - Description
cairo_scaled_font_get_type - Description
cairo_scaled_font_glyph_extents - Description
cairo_scaled_font_status - Description
cairo_scaled_font_text_extents - Description
cairo_surface_copy_page - Description
cairo_surface_create_similar - Description
cairo_surface_finish - Description
cairo_surface_flush - Description
cairo_surface_get_content - Description
cairo_surface_get_device_offset - Description
cairo_surface_get_font_options - Description
cairo_surface_get_type - Description
cairo_surface_mark_dirty_rectangle - Description
cairo_surface_mark_dirty - Description
cairo_surface_set_device_offset - Description
cairo_surface_set_fallback_resolution - Description
cairo_surface_show_page - Description
cairo_surface_status - Description
cairo_surface_write_to_png - Description
cairo_svg_surface_create - Description
cairo_svg_surface_restrict_to_version - Description
cairo_svg_version_to_string - Description
Cairo::availableFonts - Retrieves the availables font types
Cairo::availableSurfaces - Retrieves all available surfaces
Cairo::statusToString - Retrieves the current status as string
Cairo::version - Retrives cairo's library version
Cairo::versionString - Retrieves cairo version as string
CairoContext::appendPath - Appends a path to current path
CairoContext::arc - Adds a circular arc
CairoContext::arcNegative - Adds a negative arc
CairoContext::clip - Establishes a new clip region
CairoContext::clipExtents - Computes the area inside the current clip
CairoContext::clipPreserve - Establishes a new clip region from the current clip
CairoContext::clipRectangleList - Retrieves the current clip as a list of rectangles
CairoContext::closePath - Closes the current path
CairoContext::__construct - Creates a new CairoContext
CairoContext::copyPage - Emits the current page
CairoContext::copyPath - Creates a copy of the current path
CairoContext::copyPathFlat - Gets a flattened copy of the current path
CairoContext::curveTo - Adds a curve
CairoContext::deviceToUser - Transform a coordinate
CairoContext::deviceToUserDistance - Transform a distance
CairoContext::fill - Fills the current path
CairoContext::fillExtents - Computes the filled area
CairoContext::fillPreserve - Fills and preserve the current path
CairoContext::fontExtents - Get the font extents
CairoContext::getAntialias - Retrives the current antialias mode
CairoContext::getCurrentPoint - The getCurrentPoint purpose
CairoContext::getDash - The getDash purpose
CairoContext::getDashCount - The getDashCount purpose
CairoContext::getFillRule - The getFillRule purpose
CairoContext::getFontFace - The getFontFace purpose
CairoContext::getFontMatrix - The getFontMatrix purpose
CairoContext::getFontOptions - The getFontOptions purpose
CairoContext::getGroupTarget - The getGroupTarget purpose
CairoContext::getLineCap - The getLineCap purpose
CairoContext::getLineJoin - The getLineJoin purpose
CairoContext::getLineWidth - The getLineWidth purpose
CairoContext::getMatrix - The getMatrix purpose
CairoContext::getMiterLimit - The getMiterLimit purpose
CairoContext::getOperator - The getOperator purpose
CairoContext::getScaledFont - The getScaledFont purpose
CairoContext::getSource - The getSource purpose
CairoContext::getTarget - The getTarget purpose
CairoContext::getTolerance - The getTolerance purpose
CairoContext::glyphPath - The glyphPath purpose
CairoContext::hasCurrentPoint - The hasCurrentPoint purpose
CairoContext::identityMatrix - The identityMatrix purpose
CairoContext::inFill - The inFill purpose
CairoContext::inStroke - The inStroke purpose
CairoContext::lineTo - The lineTo purpose
CairoContext::mask - The mask purpose
CairoContext::maskSurface - The maskSurface purpose
CairoContext::moveTo - The moveTo purpose
CairoContext::newPath - The newPath purpose
CairoContext::newSubPath - The newSubPath purpose
CairoContext::paint - The paint purpose
CairoContext::paintWithAlpha - The paintWithAlpha purpose
CairoContext::pathExtents - The pathExtents purpose
CairoContext::popGroup - The popGroup purpose
CairoContext::popGroupToSource - The popGroupToSource purpose
CairoContext::pushGroup - The pushGroup purpose
CairoContext::pushGroupWithContent - The pushGroupWithContent purpose
CairoContext::rectangle - The rectangle purpose
CairoContext::relCurveTo - The relCurveTo purpose
CairoContext::relLineTo - The relLineTo purpose
CairoContext::relMoveTo - The relMoveTo purpose
CairoContext::resetClip - The resetClip purpose
CairoContext::restore - The restore purpose
CairoContext::rotate - The rotate purpose
CairoContext::save - The save purpose
CairoContext::scale - The scale purpose
CairoContext::selectFontFace - The selectFontFace purpose
CairoContext::setAntialias - The setAntialias purpose
CairoContext::setDash - The setDash purpose
CairoContext::setFillRule - The setFillRule purpose
CairoContext::setFontFace - The setFontFace purpose
CairoContext::setFontMatrix - The setFontMatrix purpose
CairoContext::setFontOptions - The setFontOptions purpose
CairoContext::setFontSize - The setFontSize purpose
CairoContext::setLineCap - The setLineCap purpose
CairoContext::setLineJoin - The setLineJoin purpose
CairoContext::setLineWidth - The setLineWidth purpose
CairoContext::setMatrix - The setMatrix purpose
CairoContext::setMiterLimit - The setMiterLimit purpose
CairoContext::setOperator - The setOperator purpose
CairoContext::setScaledFont - The setScaledFont purpose
CairoContext::setSource - The setSource purpose
CairoContext::setSourceRGB - The setSourceRGB purpose
CairoContext::setSourceRGBA - The setSourceRGBA purpose
CairoContext::setSourceSurface - The setSourceSurface purpose
CairoContext::setTolerance - The setTolerance purpose
CairoContext::showPage - The showPage purpose
CairoContext::showText - The showText purpose
CairoContext::status - The status purpose
CairoContext::stroke - The stroke purpose
CairoContext::strokeExtents - The strokeExtents purpose
CairoContext::strokePreserve - The strokePreserve purpose
CairoContext::textExtents - The textExtents purpose
CairoContext::textPath - The textPath purpose
CairoContext::transform - The transform purpose
CairoContext::translate - The translate purpose
CairoContext::userToDevice - The userToDevice purpose
CairoContext::userToDeviceDistance - The userToDeviceDistance purpose
CairoSurface::__construct - The __construct purpose
CairoSurface::copyPage - The copyPage purpose
CairoSurface::createSimilar - The createSimilar purpose
CairoSurface::finish - The finish purpose
CairoSurface::flush - The flush purpose
CairoSurface::getContent - The getContent purpose
CairoSurface::getDeviceOffset - The getDeviceOffset purpose
CairoSurface::getFontOptions - The getFontOptions purpose
CairoSurface::getType - The getType purpose
CairoSurface::markDirty - The markDirty purpose
CairoSurface::markDirtyRectangle - The markDirtyRectangle purpose
CairoSurface::setDeviceOffset - The setDeviceOffset purpose
CairoSurface::setFallbackResolution - The setFallbackResolution purpose
CairoSurface::showPage - The showPage purpose
CairoSurface::status - The status purpose
CairoSurface::writeToPng - The writeToPng purpose
CairoSvgSurface::__construct - The __construct purpose
CairoSvgSurface::getVersions - Used to retrieve a list of supported SVG versions
CairoSvgSurface::restrictToVersion - The restrictToVersion purpose
CairoSvgSurface::versionToString - The versionToString purpose
CairoImageSurface::__construct - Creates a new CairoImageSurface
CairoImageSurface::createForData - The createForData purpose
CairoImageSurface::createFromPng - Creates a new CairoImageSurface form a png image file
CairoImageSurface::getData - Gets the image data as string
CairoImageSurface::getFormat - Get the image format
CairoImageSurface::getHeight - Retrieves the height of the CairoImageSurface
CairoImageSurface::getStride - The getStride purpose
CairoImageSurface::getWidth - Retrieves the width of the CairoImageSurface
CairoPdfSurface::__construct - The __construct purpose
CairoPdfSurface::setSize - The setSize purpose
CairoPsSurface::__construct - The __construct purpose
CairoPsSurface::dscBeginPageSetup - The dscBeginPageSetup purpose
CairoPsSurface::dscBeginSetup - The dscBeginSetup purpose
CairoPsSurface::dscComment - The dscComment purpose
CairoPsSurface::getEps - The getEps purpose
CairoPsSurface::getLevels - The getLevels purpose
CairoPsSurface::levelToString - The levelToString purpose
CairoPsSurface::restrictToLevel - The restrictToLevel purpose
CairoPsSurface::setEps - The setEps purpose
CairoPsSurface::setSize - The setSize purpose
CairoFontFace::__construct - Creates a new CairoFontFace object
CairoFontFace::getType - Retrieves the font face type
CairoFontFace::status - Check for CairoFontFace errors
CairoFontOptions::__construct - The __construct purpose
CairoFontOptions::equal - The equal purpose
CairoFontOptions::getAntialias - The getAntialias purpose
CairoFontOptions::getHintMetrics - The getHintMetrics purpose
CairoFontOptions::getHintStyle - The getHintStyle purpose
CairoFontOptions::getSubpixelOrder - The getSubpixelOrder purpose
CairoFontOptions::hash - The hash purpose
CairoFontOptions::merge - The merge purpose
CairoFontOptions::setAntialias - The setAntialias purpose
CairoFontOptions::setHintMetrics - The setHintMetrics purpose
CairoFontOptions::setHintStyle - The setHintStyle purpose
CairoFontOptions::setSubpixelOrder - The setSubpixelOrder purpose
CairoFontOptions::status - The status purpose
CairoScaledFont::__construct - The __construct purpose
CairoScaledFont::extents - The extents purpose
CairoScaledFont::getCtm - The getCtm purpose
CairoScaledFont::getFontFace - The getFontFace purpose
CairoScaledFont::getFontMatrix - The getFontMatrix purpose
CairoScaledFont::getFontOptions - The getFontOptions purpose
CairoScaledFont::getScaleMatrix - The getScaleMatrix purpose
CairoScaledFont::getType - The getType purpose
CairoScaledFont::glyphExtents - The glyphExtents purpose
CairoScaledFont::status - The status purpose
CairoScaledFont::textExtents - The textExtents purpose
CairoPattern::__construct - The __construct purpose
CairoPattern::getMatrix - The getMatrix purpose
CairoPattern::getType - The getType purpose
CairoPattern::setMatrix - The setMatrix purpose
CairoPattern::status - The status purpose
CairoGradientPattern::addColorStopRgb - The addColorStopRgb purpose
CairoGradientPattern::addColorStopRgba - The addColorStopRgba purpose
CairoGradientPattern::getColorStopCount - The getColorStopCount purpose
CairoGradientPattern::getColorStopRgba - The getColorStopRgba purpose
CairoGradientPattern::getExtend - The getExtend purpose
CairoGradientPattern::setExtend - The setExtend purpose
CairoSolidPattern::__construct - The __construct purpose
CairoSolidPattern::getRgba - The getRgba purpose
CairoSurfacePattern::__construct - The __construct purpose
CairoSurfacePattern::getExtend - The getExtend purpose
CairoSurfacePattern::getFilter - The getFilter purpose
CairoSurfacePattern::getSurface - The getSurface purpose
CairoSurfacePattern::setExtend - The setExtend purpose
CairoSurfacePattern::setFilter - The setFilter purpose
CairoLinearGradient::__construct - The __construct purpose
CairoLinearGradient::getPoints - The getPoints purpose
CairoRadialGradient::__construct - The __construct purpose
CairoRadialGradient::getCircles - The getCircles purpose
CairoFormat::strideForWidth - Provides an appropiate stride to use
CairoMatrix::__construct - Creates a new CairoMatrix object
CairoMatrix::initIdentity - Creates a new identity matrix
CairoMatrix::initRotate - Creates a new rotated matrix
CairoMatrix::initScale - Creates a new scaling matrix
CairoMatrix::initTranslate - Creates a new translation matrix
CairoMatrix::invert - The invert purpose
CairoMatrix::multiply - The multiply purpose
CairoMatrix::rotate - The rotate purpose
CairoMatrix::scale - Applies scaling to a matrix
CairoMatrix::transformDistance - The transformDistance purpose
CairoMatrix::transformPoint - The transformPoint purpose
CairoMatrix::translate - The translate purpose
Calendar
cal_days_in_month - Return the number of days in a month for a given year and calendar
cal_from_jd - Converts from Julian Day Count to a supported calendar
cal_info - Returns information about a particular calendar
cal_to_jd - Converts from a supported calendar to Julian Day Count
easter_date - Get Unix timestamp for midnight on Easter of a given year
easter_days - Get number of days after March 21 on which Easter falls for a given year
FrenchToJD - Converts a date from the French Republican Calendar to a Julian Day Count
GregorianToJD - Converts a Gregorian date to Julian Day Count
JDDayOfWeek - Returns the day of the week
JDMonthName - Returns a month name
JDToFrench - Converts a Julian Day Count to the French Republican Calendar
JDToGregorian - Converts Julian Day Count to Gregorian date
jdtojewish - Converts a Julian day count to a Jewish calendar date
JDToJulian - Converts a Julian Day Count to a Julian Calendar Date
jdtounix - Convert Julian Day to Unix timestamp
JewishToJD - Converts a date in the Jewish Calendar to Julian Day Count
JulianToJD - Converts a Julian Calendar date to Julian Day Count
unixtojd - Convert Unix timestamp to Julian Day
Constant hash database
chdb::__construct - Creates a chdb instance
chdb::get - Gets the value associated with a key
chdb_create - Creates a chdb file
Classkit
classkit_import - Import new class method definitions from a file
classkit_method_add - Dynamically adds a new method to a given class
classkit_method_copy - Copies a method from class to another
classkit_method_redefine - Dynamically changes the code of the given method
classkit_method_remove - Dynamically removes the given method
classkit_method_rename - Dynamically changes the name of the given method
Class/Object Information
call_user_method_array - Call a user method given with an array of parameters [deprecated]
call_user_method - Call a user method on an specific object [deprecated]
class_alias - Creates an alias for a class
class_exists - Checks if the class has been defined
get_called_class - the "Late Static Binding" class name
get_class_methods - Gets the class methods' names
get_class_vars - Get the default properties of the class
get_class - Returns the name of the class of an object
get_declared_classes - Returns an array with the name of the defined classes
get_declared_interfaces - Returns an array of all declared interfaces
get_object_vars - Gets the properties of the given object
get_parent_class - Retrieves the parent class name for object or class
interface_exists - Checks if the interface has been defined
is_a - Checks if the object is of this class or has this class as one of its parents
is_subclass_of - Checks if the object has this class as one of its parents
method_exists - Checks if the class method exists
property_exists - Checks if the object or class has a property
COM and .Net (Windows)
COM - COM class
DOTNET - DOTNET class
VARIANT - VARIANT class
com_addref - Increases the components reference counter [deprecated]
com_create_guid - Generate a globally unique identifier (GUID)
com_event_sink - Connect events from a COM object to a PHP object
com_get_active_object - Returns a handle to an already running instance of a COM object
com_get - Gets the value of a COM Component's property [deprecated]
com_invoke - Calls a COM component's method [deprecated]
com_isenum - Indicates if a COM object has an IEnumVariant interface for iteration [deprecated]
com_load_typelib - Loads a Typelib
com_load - Creates a new reference to a COM component [deprecated]
com_message_pump - Process COM messages, sleeping for up to timeoutms milliseconds
com_print_typeinfo - Print out a PHP class definition for a dispatchable interface
com_propget - Alias of com_get
com_propput - Alias of com_set
com_propset - Alias of com_set
com_release - Decreases the components reference counter [deprecated]
com_set - Assigns a value to a COM component's property
variant_abs - Returns the absolute value of a variant
variant_add - "Adds" two variant values together and returns the result
variant_and - Performs a bitwise AND operation between two variants
variant_cast - Convert a variant into a new variant object of another type
variant_cat - concatenates two variant values together and returns the result
variant_cmp - Compares two variants
variant_date_from_timestamp - Returns a variant date representation of a Unix timestamp
variant_date_to_timestamp - Converts a variant date/time value to Unix timestamp
variant_div - Returns the result from dividing two variants
variant_eqv - Performs a bitwise equivalence on two variants
variant_fix - Returns the integer portion of a variant
variant_get_type - Returns the type of a variant object
variant_idiv - Converts variants to integers and then returns the result from dividing them
variant_imp - Performs a bitwise implication on two variants
variant_int - Returns the integer portion of a variant
variant_mod - Divides two variants and returns only the remainder
variant_mul - Multiplies the values of the two variants
variant_neg - Performs logical negation on a variant
variant_not - Performs bitwise not negation on a variant
variant_or - Performs a logical disjunction on two variants
variant_pow - Returns the result of performing the power function with two variants
variant_round - Rounds a variant to the specified number of decimal places
variant_set_type - Convert a variant into another type "in-place"
variant_set - Assigns a new value for a variant object
variant_sub - Subtracts the value of the right variant from the left variant value
variant_xor - Performs a logical exclusion on two variants
Cracklib
crack_check - Performs an obscure check with the given password
crack_closedict - Closes an open CrackLib dictionary
crack_getlastmessage - Returns the message from the last obscure check
crack_opendict - Opens a new CrackLib dictionary
Character type checking
ctype_alnum - Check for alphanumeric character(s)
ctype_alpha - Check for alphabetic character(s)
ctype_cntrl - Check for control character(s)
ctype_digit - Check for numeric character(s)
ctype_graph - Check for any printable character(s) except space
ctype_lower - Check for lowercase character(s)
ctype_print - Check for printable character(s)
ctype_punct - Check for any printable character which is not whitespace or an
alphanumeric character
ctype_space - Check for whitespace character(s)
ctype_upper - Check for uppercase character(s)
ctype_xdigit - Check for character(s) representing a hexadecimal digit
Cubrid
cubrid_affected_rows - Get number of affected rows in previous Cubrid operation
cubrid_bind - Is used to bind values
cubrid_close_prepare - Closes the request handle
cubrid_close_request - Closes the request handle
cubrid_col_get - Is used to get contents of the elements
cubrid_col_size - Is used to get the number of elements
cubrid_column_names - Is used to get the column names
cubrid_column_types - Gets column types
cubrid_commit - Is used to execute commit on the transaction
cubrid_connect - Is used to establish the environment for connecting to your server
cubrid_current_oid - Is used to get the oid of the current cursor location
cubrid_disconnect - Ends the transaction currently on process
cubrid_drop - Is used to delete an instance
cubrid_error_code_facility - Is used to get the facility code
cubrid_error_code - Is used to get the error code
cubrid_error_msg - Is used to get the error message
cubrid_execute - Is used to execute the given SQL sentence.
cubrid_fetch - Is used to get a single row
cubrid_get_class_name - Is used to get the class name
cubrid_get - Is used to get the attribute
cubrid_is_instance - Is used to check whether the instance
cubrid_load_from_glo - Is used to read a data
cubrid_lock_read - Is used to put read lock
cubrid_lock_write - Is used to put write lock
cubrid_move_cursor - Is used to move the current cursor location
cubrid_new_glo - Is used to create a glo instance
cubrid_num_cols - Is used to get the number of columns
cubrid_num_rows - Is used to get the number of rows
cubrid_prepare - Is a sort of API which represents SQL statements
cubrid_put - Is used to update an attribute
cubrid_rollback - Executes rollback on the transaction
cubrid_save_to_glo - Is used to save requested file
cubrid_schema - Is used to get the requested schema information
cubrid_send_glo - Is used to read data from glo
cubrid_seq_drop - Is used to delete an element
cubrid_seq_insert - Is used to insert an element to a sequence
cubrid_seq_put - Is used to update the content
cubrid_set_add - Is used to insert a single element
cubrid_set_drop - Is used to delete an element
cubrid_version - Is used to get the CUBRID PHP module�s version
Client URL Library
curl_close - Close a cURL session
curl_copy_handle - Copy a cURL handle along with all of its preferences
curl_errno - Return the last error number
curl_error - Return a string containing the last error for the current session
curl_exec - Perform a cURL session
curl_getinfo - Get information regarding a specific transfer
curl_init - Initialize a cURL session
curl_multi_add_handle - Add a normal cURL handle to a cURL multi handle
curl_multi_close - Close a set of cURL handles
curl_multi_exec - Run the sub-connections of the current cURL handle
curl_multi_getcontent - Return the content of a cURL handle if CURLOPT_RETURNTRANSFER is set
curl_multi_info_read - Get information about the current transfers
curl_multi_init - Returns a new cURL multi handle
curl_multi_remove_handle - Remove a multi handle from a set of cURL handles
curl_multi_select - Wait for activity on any curl_multi connection
curl_setopt_array - Set multiple options for a cURL transfer
curl_setopt - Set an option for a cURL transfer
curl_version - Gets cURL version information
Cyrus IMAP administration
cyrus_authenticate - Authenticate against a Cyrus IMAP server
cyrus_bind - Bind callbacks to a Cyrus IMAP connection
cyrus_close - Close connection to a Cyrus IMAP server
cyrus_connect - Connect to a Cyrus IMAP server
cyrus_query - Send a query to a Cyrus IMAP server
cyrus_unbind - Unbind ...
Date and Time
DateTime::add - Adds an amount of days, months, years, hours, minutes and seconds to a
DateTime object
DateTime::__construct - Returns new DateTime object
DateTime::createFromFormat - Returns new DateTime object formatted according to the specified format
DateTime::diff - Returns the difference between two DateTime objects
DateTime::format - Returns date formatted according to given format
DateTime::getLastErrors - Returns the warnings and errors
DateTime::getOffset - Returns the timezone offset
DateTime::getTimestamp - Gets the Unix timestamp
DateTime::getTimezone - Return time zone relative to given DateTime
DateTime::modify - Alters the timestamp
DateTime::__set_state - The __set_state handler
DateTime::setDate - Sets the date
DateTime::setISODate - Sets the ISO date
DateTime::setTime - Sets the time
DateTime::setTimestamp - Sets the date and time based on an Unix timestamp
DateTime::setTimezone - Sets the time zone for the DateTime object
DateTime::sub - Subtracts an amount of days, months, years, hours, minutes and seconds from
a DateTime object
DateTime::__wakeup - The __wakeup handler
DateTimeZone::__construct - Creates new DateTimeZone object
DateTimeZone::getLocation - Returns location information for a timezone
DateTimeZone::getName - Returns the name of the timezone
DateTimeZone::getOffset - Returns the timezone offset from GMT
DateTimeZone::getTransitions - Returns all transitions for the timezone
DateTimeZone::listAbbreviations - Returns associative array containing dst, offset and the timezone name
DateTimeZone::listIdentifiers - Returns numerically index array with all timezone identifiers
DateInterval::__construct - Creates new DateInterval object
DateInterval::createFromDateString - Sets up a DateInterval from the relative parts of the string
DateInterval::format - Formats the interval
DatePeriod::__construct - Creates new DatePeriod object
checkdate - Validate a Gregorian date
date_add - Alias of DateTime::add
date_create_from_format - Alias of DateTime::createFromFormat
date_create - Alias of DateTime::__construct
date_date_set - Alias of DateTime::setDate
date_default_timezone_get - Gets the default timezone used by all date/time functions in a script
date_default_timezone_set - Sets the default timezone used by all date/time functions in a script
date_diff - Alias of DateTime::diff
date_format - Alias of DateTime::format
date_get_last_errors - Alias of DateTime::getLastErrors
date_interval_create_from_date_string - Alias of DateInterval::createFromDateString
date_interval_format - Alias of DateInterval::format
date_isodate_set - Alias of DateTime::setISODate
date_modify - Alias of DateTime::modify
date_offset_get - Alias of DateTime::getOffset
date_parse_from_format - Get info about given date
date_parse - Returns associative array with detailed info about given date
date_sub - Alias of DateTime::sub
date_sun_info - Returns an array with information about sunset/sunrise and twilight begin/end
date_sunrise - Returns time of sunrise for a given day and location
date_sunset - Returns time of sunset for a given day and location
date_time_set - Alias of DateTime::setTime
date_timestamp_get - Alias of DateTime::getTimestamp
date_timestamp_set - Alias of DateTime::setTimestamp
date_timezone_get - Alias of DateTime::getTimezone
date_timezone_set - Alias of DateTime::setTimezone
date - Format a local time/date
getdate - Get date/time information
gettimeofday - Get current time
gmdate - Format a GMT/UTC date/time
gmmktime - Get Unix timestamp for a GMT date
gmstrftime - Format a GMT/UTC time/date according to locale settings
idate - Format a local time/date as integer
localtime - Get the local time
microtime - Return current Unix timestamp with microseconds
mktime - Get Unix timestamp for a date
strftime - Format a local time/date according to locale settings
strptime - Parse a time/date generated with strftime
strtotime - Parse about any English textual datetime description into a Unix timestamp
time - Return current Unix timestamp
timezone_abbreviations_list - Alias of DateTimeZone::listAbbreviations
timezone_identifiers_list - Alias of DateTimeZone::listIdentifiers
timezone_location_get - Alias of DateTimeZone::getLocation
timezone_name_from_abbr - Returns the timezone name from abbreviation
timezone_name_get - Alias of DateTimeZone::getName
timezone_offset_get - Alias of DateTimeZone::getOffset
timezone_open - Alias of DateTimeZone::__construct
timezone_transitions_get - Alias of DateTimeZone::getTransitions
timezone_version_get - Gets the version of the timezonedb
Database (dbm-style) Abstraction Layer
dba_close - Close a DBA database
dba_delete - Delete DBA entry specified by key
dba_exists - Check whether key exists
dba_fetch - Fetch data specified by key
dba_firstkey - Fetch first key
dba_handlers - List all the handlers available
dba_insert - Insert entry
dba_key_split - Splits a key in string representation into array representation
dba_list - List all open database files
dba_nextkey - Fetch next key
dba_open - Open database
dba_optimize - Optimize database
dba_popen - Open database persistently
dba_replace - Replace or insert entry
dba_sync - Synchronize database
dBase
dbase_add_record - Adds a record to a database
dbase_close - Closes a database
dbase_create - Creates a database
dbase_delete_record - Deletes a record from a database
dbase_get_header_info - Gets the header info of a database
dbase_get_record_with_names - Gets a record from a database as an associative array
dbase_get_record - Gets a record from a database as an indexed array
dbase_numfields - Gets the number of fields of a database
dbase_numrecords - Gets the number of records in a database
dbase_open - Opens a database
dbase_pack - Packs a database
dbase_replace_record - Replaces a record in a database
DB++
dbplus_add - Add a tuple to a relation
dbplus_aql - Perform AQL query
dbplus_chdir - Get/Set database virtual current directory
dbplus_close - Close a relation
dbplus_curr - Get current tuple from relation
dbplus_errcode - Get error string for given errorcode or last error
dbplus_errno - Get error code for last operation
dbplus_find - Set a constraint on a relation
dbplus_first - Get first tuple from relation
dbplus_flush - Flush all changes made on a relation
dbplus_freealllocks - Free all locks held by this client
dbplus_freelock - Release write lock on tuple
dbplus_freerlocks - Free all tuple locks on given relation
dbplus_getlock - Get a write lock on a tuple
dbplus_getunique - Get an id number unique to a relation
dbplus_info - Get information about a relation
dbplus_last - Get last tuple from relation
dbplus_lockrel - Request write lock on relation
dbplus_next - Get next tuple from relation
dbplus_open - Open relation file
dbplus_prev - Get previous tuple from relation
dbplus_rchperm - Change relation permissions
dbplus_rcreate - Creates a new DB++ relation
dbplus_rcrtexact - Creates an exact but empty copy of a relation including indices
dbplus_rcrtlike - Creates an empty copy of a relation with default indices
dbplus_resolve - Resolve host information for relation
dbplus_restorepos - Restore position
dbplus_rkeys - Specify new primary key for a relation
dbplus_ropen - Open relation file local
dbplus_rquery - Perform local (raw) AQL query
dbplus_rrename - Rename a relation
dbplus_rsecindex - Create a new secondary index for a relation
dbplus_runlink - Remove relation from filesystem
dbplus_rzap - Remove all tuples from relation
dbplus_savepos - Save position
dbplus_setindex - Set index
dbplus_setindexbynumber - Set index by number
dbplus_sql - Perform SQL query
dbplus_tcl - Execute TCL code on server side
dbplus_tremove - Remove tuple and return new current tuple
dbplus_undo - Undo
dbplus_undoprepare - Prepare undo
dbplus_unlockrel - Give up write lock on relation
dbplus_unselect - Remove a constraint from relation
dbplus_update - Update specified tuple in relation
dbplus_xlockrel - Request exclusive lock on relation
dbplus_xunlockrel - Free exclusive lock on relation
dbx
dbx_close - Close an open connection/database
dbx_compare - Compare two rows for sorting purposes
dbx_connect - Open a connection/database
dbx_error - Report the error message of the latest function call in the module
dbx_escape_string - Escape a string so it can safely be used in an sql-statement
dbx_fetch_row - Fetches rows from a query-result that had the
DBX_RESULT_UNBUFFERED flag set
dbx_query - Send a query and fetch all results (if any)
dbx_sort - Sort a result from a dbx_query by a custom sort function
Direct IO
dio_close - Closes the file descriptor given by fd
dio_fcntl - Performs a c library fcntl on fd
dio_open - Opens a file (creating it if necessary) at a lower level than the
C library input/ouput stream functions allow.
dio_read - Reads bytes from a file descriptor
dio_seek - Seeks to pos on fd from whence
dio_stat - Gets stat information about the file descriptor fd
dio_tcsetattr - Sets terminal attributes and baud rate for a serial port
dio_truncate - Truncates file descriptor fd to offset bytes
dio_write - Writes data to fd with optional truncation at length
Directories
chdir - Change directory
chroot - Change the root directory
dir - Return an instance of the Directory class
closedir - Close directory handle
getcwd - Gets the current working directory
opendir - Open directory handle
readdir - Read entry from directory handle
rewinddir - Rewind directory handle
scandir - List files and directories inside the specified path
Document Object Model
DOMAttr::__construct - Creates a new DOMAttr object
DOMAttr::isId - Checks if attribute is a defined ID
DOMCharacterData::appendData - Append the string to the end of the character data of the node
DOMCharacterData::deleteData - Remove a range of characters from the node
DOMCharacterData::insertData - Insert a string at the specified 16-bit unit offset
DOMCharacterData::replaceData - Replace a substring within the DOMCharacterData node
DOMCharacterData::substringData - Extracts a range of data from the node
DOMComment::__construct - Creates a new DOMComment object
DOMDocument::__construct - Creates a new DOMDocument object
DOMDocument::createAttribute - Create new attribute
DOMDocument::createAttributeNS - Create new attribute node with an associated namespace
DOMDocument::createCDATASection - Create new cdata node
DOMDocument::createComment - Create new comment node
DOMDocument::createDocumentFragment - Create new document fragment
DOMDocument::createElement - Create new element node
DOMDocument::createElementNS - Create new element node with an associated namespace
DOMDocument::createEntityReference - Create new entity reference node
DOMDocument::createProcessingInstruction - Creates new PI node
DOMDocument::createTextNode - Create new text node
DOMDocument::getElementById - Searches for an element with a certain id
DOMDocument::getElementsByTagName - Searches for all elements with given tag name
DOMDocument::getElementsByTagNameNS - Searches for all elements with given tag name in specified namespace
DOMDocument::importNode - Import node into current document
DOMDocument::load - Load XML from a file
DOMDocument::loadHTML - Load HTML from a string
DOMDocument::loadHTMLFile - Load HTML from a file
DOMDocument::loadXML - Load XML from a string
DOMDocument::normalizeDocument - Normalizes the document
DOMDocument::registerNodeClass - Register extended class used to create base node type
DOMDocument::relaxNGValidate - Performs relaxNG validation on the document
DOMDocument::relaxNGValidateSource - Performs relaxNG validation on the document
DOMDocument::save - Dumps the internal XML tree back into a file
DOMDocument::saveHTML - Dumps the internal document into a string using HTML formatting
DOMDocument::saveHTMLFile - Dumps the internal document into a file using HTML formatting
DOMDocument::saveXML - Dumps the internal XML tree back into a string
DOMDocument::schemaValidate - Validates a document based on a schema
DOMDocument::schemaValidateSource - Validates a document based on a schema
DOMDocument::validate - Validates the document based on its DTD
DOMDocument::xinclude - Substitutes XIncludes in a DOMDocument Object
DOMDocumentFragment::appendXML - Append raw XML data
DOMElement::__construct - Creates a new DOMElement object
DOMElement::getAttribute - Returns value of attribute
DOMElement::getAttributeNode - Returns attribute node
DOMElement::getAttributeNodeNS - Returns attribute node
DOMElement::getAttributeNS - Returns value of attribute
DOMElement::getElementsByTagName - Gets elements by tagname
DOMElement::getElementsByTagNameNS - Get elements by namespaceURI and localName
DOMElement::hasAttribute - Checks to see if attribute exists
DOMElement::hasAttributeNS - Checks to see if attribute exists
DOMElement::removeAttribute - Removes attribute
DOMElement::removeAttributeNode - Removes attribute
DOMElement::removeAttributeNS - Removes attribute
DOMElement::setAttribute - Adds new attribute
DOMElement::setAttributeNode - Adds new attribute node to element
DOMElement::setAttributeNodeNS - Adds new attribute node to element
DOMElement::setAttributeNS - Adds new attribute
DOMElement::setIdAttribute - Declares the attribute specified by name to be of type ID
DOMElement::setIdAttributeNode - Declares the attribute specified by node to be of type ID
DOMElement::setIdAttributeNS - Declares the attribute specified by local name and namespace URI to be of type ID
DOMEntityReference::__construct - Creates a new DOMEntityReference object
DOMImplementation::__construct - Creates a new DOMImplementation object
DOMImplementation::createDocument - Creates a DOMDocument object of the specified type with its document element
DOMImplementation::createDocumentType - Creates an empty DOMDocumentType object
DOMImplementation::hasFeature - Test if the DOM implementation implements a specific feature
DOMNamedNodeMap::getNamedItem - Retrieves a node specified by name
DOMNamedNodeMap::getNamedItemNS - Retrieves a node specified by local name and namespace URI
DOMNamedNodeMap::item - Retrieves a node specified by index
DOMNode::appendChild - Adds new child at the end of the children
DOMNode::cloneNode - Clones a node
DOMNode::getLineNo - Get line number for a node
DOMNode::hasAttributes - Checks if node has attributes
DOMNode::hasChildNodes - Checks if node has children
DOMNode::insertBefore - Adds a new child before a reference node
DOMNode::isDefaultNamespace - Checks if the specified namespaceURI is the default namespace or not
DOMNode::isSameNode - Indicates if two nodes are the same node
DOMNode::isSupported - Checks if feature is supported for specified version
DOMNode::lookupNamespaceURI - Gets the namespace URI of the node based on the prefix
DOMNode::lookupPrefix - Gets the namespace prefix of the node based on the namespace URI
DOMNode::normalize - Normalizes the node
DOMNode::removeChild - Removes child from list of children
DOMNode::replaceChild - Replaces a child
DOMNodelist::item - Retrieves a node specified by index
DOMProcessingInstruction::__construct - Creates a new DOMProcessingInstruction object
DOMText::__construct - Creates a new DOMText object
DOMText::isWhitespaceInElementContent - Indicates whether this text node contains whitespace
DOMText::splitText - Breaks this node into two nodes at the specified offset
DOMXPath::__construct - Creates a new DOMXPath object
DOMXPath::evaluate - Evaluates the given XPath expression and returns a typed result if possible.
DOMXPath::query - Evaluates the given XPath expression
DOMXPath::registerNamespace - Registers the namespace with the DOMXPath object
DOMXPath::registerPhpFunctions - Register PHP functions as XPath functions
dom_import_simplexml - Gets a DOMElement object from a
SimpleXMLElement object
DOM XML (PHP 4)
DomAttribute->name - Returns the name of attribute
DomAttribute->set_value - Sets the value of an attribute
DomAttribute->specified - Checks if attribute is specified
DomAttribute->value - Returns value of attribute
DomDocument->add_root - Adds a root node [deprecated]
DomDocument->create_attribute - Create new attribute
DomDocument->create_cdata_section - Create new cdata node
DomDocument->create_comment - Create new comment node
DomDocument->create_element_ns - Create new element node with an associated namespace
DomDocument->create_element - Create new element node
DomDocument->create_entity_reference - Create an entity reference
DomDocument->create_processing_instruction - Creates new PI node
DomDocument->create_text_node - Create new text node
DomDocument->doctype - Returns the document type
DomDocument->document_element - Returns root element node
DomDocument->dump_file - Dumps the internal XML tree back into a file
DomDocument->dump_mem - Dumps the internal XML tree back into a string
DomDocument->get_element_by_id - Searches for an element with a certain id
DomDocument->get_elements_by_tagname - Returns array with nodes with given tagname in document or empty array, if not found
DomDocument->html_dump_mem - Dumps the internal XML tree back into a string as HTML
DomDocument->xinclude - Substitutes XIncludes in a DomDocument Object
DomDocumentType->entities - Returns list of entities
DomDocumentType->internal_subset - Returns internal subset
DomDocumentType->name - Returns name of document type
DomDocumentType->notations - Returns list of notations
DomDocumentType->public_id - Returns public id of document type
DomDocumentType->system_id - Returns the system id of document type
DomElement->get_attribute_node - Returns the node of the given attribute
DomElement->get_attribute - Returns the value of the given attribute
DomElement->get_elements_by_tagname - Gets elements by tagname
DomElement->has_attribute - Checks to see if an attribute exists in the current node
DomElement->remove_attribute - Removes attribute
DomElement->set_attribute_node - Adds new attribute
DomElement->set_attribute - Sets the value of an attribute
DomElement->tagname - Returns the name of the current element
DomNode->add_namespace - Adds a namespace declaration to a node
DomNode->append_child - Adds a new child at the end of the children
DomNode->append_sibling - Adds new sibling to a node
DomNode->attributes - Returns list of attributes
DomNode->child_nodes - Returns children of node
DomNode->clone_node - Clones a node
DomNode->dump_node - Dumps a single node
DomNode->first_child - Returns first child of node
DomNode->get_content - Gets content of node
DomNode->has_attributes - Checks if node has attributes
DomNode->has_child_nodes - Checks if node has children
DomNode->insert_before - Inserts new node as child
DomNode->is_blank_node - Checks if node is blank
DomNode->last_child - Returns last child of node
DomNode->next_sibling - Returns the next sibling of node
DomNode->node_name - Returns name of node
DomNode->node_type - Returns type of node
DomNode->node_value - Returns value of a node
DomNode->owner_document - Returns the document this node belongs to
DomNode->parent_node - Returns the parent of the node
DomNode->prefix - Returns name space prefix of node
DomNode->previous_sibling - Returns the previous sibling of node
DomNode->remove_child - Removes child from list of children
DomNode->replace_child - Replaces a child
DomNode->replace_node - Replaces node
DomNode->set_content - Sets content of node
DomNode->set_name - Sets name of node
DomNode->set_namespace - Sets namespace of a node
DomNode->unlink_node - Deletes node
DomProcessingInstruction->data - Returns the data of ProcessingInstruction node
DomProcessingInstruction->target - Returns the target of a ProcessingInstruction node
DomXsltStylesheet->process - Applies the XSLT-Transformation on a DomDocument Object
DomXsltStylesheet->result_dump_file - Dumps the result from a XSLT-Transformation into a file
DomXsltStylesheet->result_dump_mem - Dumps the result from a XSLT-Transformation back into a string
domxml_new_doc - Creates new empty XML document
domxml_open_file - Creates a DOM object from an XML file
domxml_open_mem - Creates a DOM object of an XML document
domxml_version - Gets the XML library version
domxml_xmltree - Creates a tree of PHP objects from an XML document
domxml_xslt_stylesheet_doc - Creates a DomXsltStylesheet Object from a DomDocument Object
domxml_xslt_stylesheet_file - Creates a DomXsltStylesheet Object from an XSL document in a file
domxml_xslt_stylesheet - Creates a DomXsltStylesheet object from an XSL document in a string
domxml_xslt_version - Gets the XSLT library version
xpath_eval_expression - Evaluates the XPath Location Path in the given string
xpath_eval - Evaluates the XPath Location Path in the given string
xpath_new_context - Creates new xpath context
xpath_register_ns_auto - Register the given namespace in the passed XPath context
xpath_register_ns - Register the given namespace in the passed XPath context
xptr_eval - Evaluate the XPtr Location Path in the given string
xptr_new_context - Create new XPath Context
.NET
dotnet_load - Loads a DOTNET module
Enchant spelling library
enchant_broker_describe - Enumerates the Enchant providers
enchant_broker_dict_exists - Whether a dictionary exists or not. Using non-empty tag
enchant_broker_free_dict - Free a dictionary resource
enchant_broker_free - Free the broker resource and its dictionnaries
enchant_broker_get_error - Returns the last error of the broker
enchant_broker_init - create a new broker object capable of requesting
enchant_broker_list_dicts - Returns a list of available dictionaries
enchant_broker_request_dict - create a new dictionary using a tag
enchant_broker_request_pwl_dict - creates a dictionary using a PWL file
enchant_broker_set_ordering - Declares a preference of dictionaries to use for the language
enchant_dict_add_to_personal - add a word to personal word list
enchant_dict_add_to_session - add 'word' to this spell-checking session
enchant_dict_check - Check whether a word is correctly spelled or not
enchant_dict_describe - Describes an individual dictionary
enchant_dict_get_error - Returns the last error of the current spelling-session
enchant_dict_is_in_session - whether or not 'word' exists in this spelling-session
enchant_dict_quick_check - Check the word is correctly spelled and provide suggestions
enchant_dict_store_replacement - Add a correction for a word
enchant_dict_suggest - Will return a list of values if any of those pre-conditions are not met
Error Handling and Logging
debug_backtrace - Generates a backtrace
debug_print_backtrace - Prints a backtrace
error_get_last - Get the last occurred error
error_log - Send an error message somewhere
error_reporting - Sets which PHP errors are reported
restore_error_handler - Restores the previous error handler function
restore_exception_handler - Restores the previously defined exception handler function
set_error_handler - Sets a user-defined error handler function
set_exception_handler - Sets a user-defined exception handler function
trigger_error - Generates a user-level error/warning/notice message
user_error - Alias of trigger_error
System program execution
escapeshellarg - Escape a string to be used as a shell argument
escapeshellcmd - Escape shell metacharacters
exec - Execute an external program
passthru - Execute an external program and display raw output
proc_close - Close a process opened by proc_open and return the exit code of that process
proc_get_status - Get information about a process opened by proc_open
proc_nice - Change the priority of the current process
proc_open - Execute a command and open file pointers for input/output
proc_terminate - Kills a process opened by proc_open
shell_exec - Execute command via shell and return the complete output as a string
system - Execute an external program and display the output
Exchangeable image information
exif_imagetype - Determine the type of an image
exif_read_data - Reads the EXIF headers from JPEG or TIFF
exif_tagname - Get the header name for an index
exif_thumbnail - Retrieve the embedded thumbnail of a TIFF or JPEG image
read_exif_data - Alias of exif_read_data
Expect
expect_expectl - Waits until the output from a process matches one
of the patterns, a specified time period has passed, or an EOF is seen
expect_popen - Execute command via Bourne shell, and open the PTY stream to
the process
File Alteration Monitor
fam_cancel_monitor - Terminate monitoring
fam_close - Close FAM connection
fam_monitor_collection - Monitor a collection of files in a directory for changes
fam_monitor_directory - Monitor a directory for changes
fam_monitor_file - Monitor a regular file for changes
fam_next_event - Get next pending FAM event
fam_open - Open connection to FAM daemon
fam_pending - Check for pending FAM events
fam_resume_monitor - Resume suspended monitoring
fam_suspend_monitor - Temporarily suspend monitoring
FrontBase
fbsql_affected_rows - Get number of affected rows in previous FrontBase operation
fbsql_autocommit - Enable or disable autocommit
fbsql_blob_size - Get the size of a BLOB
fbsql_change_user - Change logged in user of the active connection
fbsql_clob_size - Get the size of a CLOB
fbsql_close - Close FrontBase connection
fbsql_commit - Commits a transaction to the database
fbsql_connect - Open a connection to a FrontBase Server
fbsql_create_blob - Create a BLOB
fbsql_create_clob - Create a CLOB
fbsql_create_db - Create a FrontBase database
fbsql_data_seek - Move internal result pointer
fbsql_database_password - Sets or retrieves the password for a FrontBase database
fbsql_database - Get or set the database name used with a connection
fbsql_db_query - Send a FrontBase query
fbsql_db_status - Get the status for a given database
fbsql_drop_db - Drop (delete) a FrontBase database
fbsql_errno - Returns the error number from previous operation
fbsql_error - Returns the error message from previous operation
fbsql_fetch_array - Fetch a result row as an associative array, a numeric array, or both
fbsql_fetch_assoc - Fetch a result row as an associative array
fbsql_fetch_field - Get column information from a result and return as an object
fbsql_fetch_lengths - Get the length of each output in a result
fbsql_fetch_object - Fetch a result row as an object
fbsql_fetch_row - Get a result row as an enumerated array
fbsql_field_flags - Get the flags associated with the specified field in a result
fbsql_field_len - Returns the length of the specified field
fbsql_field_name - Get the name of the specified field in a result
fbsql_field_seek - Set result pointer to a specified field offset
fbsql_field_table - Get name of the table the specified field is in
fbsql_field_type - Get the type of the specified field in a result
fbsql_free_result - Free result memory
fbsql_get_autostart_info - Description
fbsql_hostname - Get or set the host name used with a connection
fbsql_insert_id - Get the id generated from the previous INSERT operation
fbsql_list_dbs - List databases available on a FrontBase server
fbsql_list_fields - List FrontBase result fields
fbsql_list_tables - List tables in a FrontBase database
fbsql_next_result - Move the internal result pointer to the next result
fbsql_num_fields - Get number of fields in result
fbsql_num_rows - Get number of rows in result
fbsql_password - Get or set the user password used with a connection
fbsql_pconnect - Open a persistent connection to a FrontBase Server
fbsql_query - Send a FrontBase query
fbsql_read_blob - Read a BLOB from the database
fbsql_read_clob - Read a CLOB from the database
fbsql_result - Get result data
fbsql_rollback - Rollback a transaction to the database
fbsql_rows_fetched - Get the number of rows affected by the last statement
fbsql_select_db - Select a FrontBase database
fbsql_set_characterset - Change input/output character set
fbsql_set_lob_mode - Set the LOB retrieve mode for a FrontBase result set
fbsql_set_password - Change the password for a given user
fbsql_set_transaction - Set the transaction locking and isolation
fbsql_start_db - Start a database on local or remote server
fbsql_stop_db - Stop a database on local or remote server
fbsql_table_name - Get table name of field
fbsql_tablename - Alias of fbsql_table_name
fbsql_username - Get or set the username for the connection
fbsql_warnings - Enable or disable FrontBase warnings
Forms Data Format
fdf_add_doc_javascript - Adds javascript code to the FDF document
fdf_add_template - Adds a template into the FDF document
fdf_close - Close an FDF document
fdf_create - Create a new FDF document
fdf_enum_values - Call a user defined function for each document value
fdf_errno - Return error code for last fdf operation
fdf_error - Return error description for FDF error code
fdf_get_ap - Get the appearance of a field
fdf_get_attachment - Extracts uploaded file embedded in the FDF
fdf_get_encoding - Get the value of the /Encoding key
fdf_get_file - Get the value of the /F key
fdf_get_flags - Gets the flags of a field
fdf_get_opt - Gets a value from the opt array of a field
fdf_get_status - Get the value of the /STATUS key
fdf_get_value - Get the value of a field
fdf_get_version - Gets version number for FDF API or file
fdf_header - Sets FDF-specific output headers
fdf_next_field_name - Get the next field name
fdf_open_string - Read a FDF document from a string
fdf_open - Open a FDF document
fdf_remove_item - Sets target frame for form
fdf_save_string - Returns the FDF document as a string
fdf_save - Save a FDF document
fdf_set_ap - Set the appearance of a field
fdf_set_encoding - Sets FDF character encoding
fdf_set_file - Set PDF document to display FDF data in
fdf_set_flags - Sets a flag of a field
fdf_set_javascript_action - Sets an javascript action of a field
fdf_set_on_import_javascript - Adds javascript code to be executed when Acrobat opens the FDF
fdf_set_opt - Sets an option of a field
fdf_set_status - Set the value of the /STATUS key
fdf_set_submit_form_action - Sets a submit form action of a field
fdf_set_target_frame - Set target frame for form display
fdf_set_value - Set the value of a field
fdf_set_version - Sets version number for a FDF file
File Information
finfo_buffer - Return information about a string buffer
finfo_close - Close fileinfo resource
finfo_file - Return information about a file
finfo_open - Create a new fileinfo resource
finfo_set_flags - Set libmagic configuration options
mime_content_type - Detect MIME Content-type for a file (deprecated)
filePro
filepro_fieldcount - Find out how many fields are in a filePro database
filepro_fieldname - Gets the name of a field
filepro_fieldtype - Gets the type of a field
filepro_fieldwidth - Gets the width of a field
filepro_retrieve - Retrieves data from a filePro database
filepro_rowcount - Find out how many rows are in a filePro database
filepro - Read and verify the map file
Filesystem
basename - Returns filename component of path
chgrp - Changes file group
chmod - Changes file mode
chown - Changes file owner
clearstatcache - Clears file status cache
copy - Copies file
delete - See unlink or unset
dirname - Returns directory name component of path
disk_free_space - Returns available space on filesystem or disk partition
disk_total_space - Returns the total size of a filesystem or disk partition
diskfreespace - Alias of disk_free_space
fclose - Closes an open file pointer
feof - Tests for end-of-file on a file pointer
fflush - Flushes the output to a file
fgetc - Gets character from file pointer
fgetcsv - Gets line from file pointer and parse for CSV fields
fgets - Gets line from file pointer
fgetss - Gets line from file pointer and strip HTML tags
file_exists - Checks whether a file or directory exists
file_get_contents - Reads entire file into a string
file_put_contents - Write a string to a file
file - Reads entire file into an array
fileatime - Gets last access time of file
filectime - Gets inode change time of file
filegroup - Gets file group
fileinode - Gets file inode
filemtime - Gets file modification time
fileowner - Gets file owner
fileperms - Gets file permissions
filesize - Gets file size
filetype - Gets file type
flock - Portable advisory file locking
fnmatch - Match filename against a pattern
fopen - Opens file or URL
fpassthru - Output all remaining data on a file pointer
fputcsv - Format line as CSV and write to file pointer
fputs - Alias of fwrite
fread - Binary-safe file read
fscanf - Parses input from a file according to a format
fseek - Seeks on a file pointer
fstat - Gets information about a file using an open file pointer
ftell - Returns the current position of the file read/write pointer
ftruncate - Truncates a file to a given length
fwrite - Binary-safe file write
glob - Find pathnames matching a pattern
is_dir - Tells whether the filename is a directory
is_executable - Tells whether the filename is executable
is_file - Tells whether the filename is a regular file
is_link - Tells whether the filename is a symbolic link
is_readable - Tells whether a file exists and is readable
is_uploaded_file - Tells whether the file was uploaded via HTTP POST
is_writable - Tells whether the filename is writable
is_writeable - Alias of is_writable
lchgrp - Changes group ownership of symlink
lchown - Changes user ownership of symlink
link - Create a hard link
linkinfo - Gets information about a link
lstat - Gives information about a file or symbolic link
mkdir - Makes directory
move_uploaded_file - Moves an uploaded file to a new location
parse_ini_file - Parse a configuration file
parse_ini_string - Parse a configuration string
pathinfo - Returns information about a file path
pclose - Closes process file pointer
popen - Opens process file pointer
readfile - Outputs a file
readlink - Returns the target of a symbolic link
realpath_cache_get - Get realpath cache entries
realpath_cache_size - Get realpath cache size
realpath - Returns canonicalized absolute pathname
rename - Renames a file or directory
rewind - Rewind the position of a file pointer
rmdir - Removes directory
set_file_buffer - Alias of stream_set_write_buffer
stat - Gives information about a file
symlink - Creates a symbolic link
tempnam - Create file with unique file name
tmpfile - Creates a temporary file
touch - Sets access and modification time of file
umask - Changes the current umask
unlink - Deletes a file
Data Filtering
filter_has_var - Checks if variable of specified type exists
filter_id - Returns the filter ID belonging to a named filter
filter_input_array - Gets external variables and optionally filters them
filter_input - Gets a specific external variable by name and optionally filters it
filter_list - Returns a list of all supported filters
filter_var_array - Gets multiple variables and optionally filters them
filter_var - Filters a variable with a specified filter
FriBiDi
fribidi_log2vis - Convert a logical string to a visual one
FTP
ftp_alloc - Allocates space for a file to be uploaded
ftp_cdup - Changes to the parent directory
ftp_chdir - Changes the current directory on a FTP server
ftp_chmod - Set permissions on a file via FTP
ftp_close - Closes an FTP connection
ftp_connect - Opens an FTP connection
ftp_delete - Deletes a file on the FTP server
ftp_exec - Requests execution of a command on the FTP server
ftp_fget - Downloads a file from the FTP server and saves to an open file
ftp_fput - Uploads from an open file to the FTP server
ftp_get_option - Retrieves various runtime behaviours of the current FTP stream
ftp_get - Downloads a file from the FTP server
ftp_login - Logs in to an FTP connection
ftp_mdtm - Returns the last modified time of the given file
ftp_mkdir - Creates a directory
ftp_nb_continue - Continues retrieving/sending a file (non-blocking)
ftp_nb_fget - Retrieves a file from the FTP server and writes it to an open file (non-blocking)
ftp_nb_fput - Stores a file from an open file to the FTP server (non-blocking)
ftp_nb_get - Retrieves a file from the FTP server and writes it to a local file (non-blocking)
ftp_nb_put - Stores a file on the FTP server (non-blocking)
ftp_nlist - Returns a list of files in the given directory
ftp_pasv - Turns passive mode on or off
ftp_put - Uploads a file to the FTP server
ftp_pwd - Returns the current directory name
ftp_quit - Alias of ftp_close
ftp_raw - Sends an arbitrary command to an FTP server
ftp_rawlist - Returns a detailed list of files in the given directory
ftp_rename - Renames a file or a directory on the FTP server
ftp_rmdir - Removes a directory
ftp_set_option - Set miscellaneous runtime FTP options
ftp_site - Sends a SITE command to the server
ftp_size - Returns the size of the given file
ftp_ssl_connect - Opens an Secure SSL-FTP connection
ftp_systype - Returns the system type identifier of the remote FTP server
Function Handling
call_user_func_array - Call a user function given with an array of parameters
call_user_func - Call a user function given by the first parameter
create_function - Create an anonymous (lambda-style) function
forward_static_call_array - Call a static method and pass the arguments as array
forward_static_call - Call a static method
func_get_arg - Return an item from the argument list
func_get_args - Returns an array comprising a function's argument list
func_num_args - Returns the number of arguments passed to the function
function_exists - Return TRUE if the given function has been defined
get_defined_functions - Returns an array of all defined functions
register_shutdown_function - Register a function for execution on shutdown
register_tick_function - Register a function for execution on each tick
unregister_tick_function - De-register a function for execution on each tick
Gearman
GearmanClient::addOptions - Add client options
GearmanClient::addServer - Add a job server to the client
GearmanClient::addServers - Add a list of job servers to the client
GearmanClient::addTask - Add a task to be run in parallel
GearmanClient::addTaskBackground - Add a background task to be run in parallel
GearmanClient::addTaskHigh - Add a high priority task to run in parallel
GearmanClient::addTaskHighBackground - Add a high priority background task to be run in parallel
GearmanClient::addTaskLow - Add a low priority task to run in parallel
GearmanClient::addTaskLowBackground - Add a low priority background task to be run in parallel
GearmanClient::addTaskStatus - Add a task to get status
GearmanClient::clearCallbacks - Clear all task callback functions
GearmanClient::clone - Create a copy of a GearmanClient object
GearmanClient::__construct - Create a GearmanClient instance
GearmanClient::context - Get the application context
GearmanClient::data - Get the application data (deprecated)
GearmanClient::do - Run a single task and return a result
GearmanClient::doBackground - Run a task in the background
GearmanClient::doHigh - Run a single high priority task
GearmanClient::doHighBackground - Run a high priority task in the background
GearmanClient::doJobHandle - Get the job handle for the running task
GearmanClient::doLow - Run a single low priority task
GearmanClient::doLowBackground - Run a low priority task in the background
GearmanClient::doStatus - Get the status for the running task
GearmanClient::echo - Send data to all job servers to see if they echo it back
GearmanClient::error - Returns an error string for the last error encountered.
GearmanClient::getErrno - Get an errno value
GearmanClient::jobStatus - Get the status of a background job
GearmanClient::removeOptions - Remove client options
GearmanClient::returnCode - Get the last Gearman return code
GearmanClient::runTasks - Run a list of tasks in parallel
GearmanClient::setClientCallback - Callback function when there is a data packet for a task (deprecated)
GearmanClient::setCompleteCallback - Set a function to be called on task completion
GearmanClient::setContext - Set application context
GearmanClient::setCreatedCallback - Set a callback for when a task is queued
GearmanClient::setData - Set application data (deprecated)
GearmanClient::setDataCallback - Callback function when there is a data packet for a task
GearmanClient::setExceptionCallback - Set a callback for worker exceptions
GearmanClient::setFailCallback - Set callback for job failure
GearmanClient::setOptions - Set client options
GearmanClient::setStatusCallback - Set a callback for collecting task status
GearmanClient::setTimeout - Set socket I/O activity timeout
GearmanClient::setWarningCallback - Set a callback for worker warnings
GearmanClient::setWorkloadCallback - Set a callback for accepting incremental data updates
GearmanClient::timeout - Get current socket I/O activity timeout value
GearmanJob::complete - Send the result and complete status (deprecated)
GearmanJob::__construct - Create a GearmanJob instance
GearmanJob::data - Send data for a running job (deprecated)
GearmanJob::exception - Send exception for running job (deprecated)
GearmanJob::fail - Send fail status (deprecated)
GearmanJob::functionName - Get function name
GearmanJob::handle - Get the job handle
GearmanJob::returnCode - Get last return code
GearmanJob::sendComplete - Send the result and complete status
GearmanJob::sendData - Send data for a running job
GearmanJob::sendException - Send exception for running job (exception)
GearmanJob::sendFail - Send fail status
GearmanJob::sendStatus - Send status
GearmanJob::sendWarning - Send a warning
GearmanJob::setReturn - Set a return value
GearmanJob::status - Send status (deprecated)
GearmanJob::unique - Get the unique identifier
GearmanJob::warning - Send a warning (deprecated)
GearmanJob::workload - Get workload
GearmanJob::workloadSize - Get size of work load
GearmanTask::__construct - Create a GearmanTask instance
GearmanTask::create - Create a task (deprecated)
GearmanTask::data - Get data returned for a task
GearmanTask::dataSize - Get the size of returned data
GearmanTask::function - Get associated function name (deprecated)
GearmanTask::functionName - Get associated function name
GearmanTask::isKnown - Determine if task is known
GearmanTask::isRunning - Test whether the task is currently running
GearmanTask::jobHandle - Get the job handle
GearmanTask::recvData - Read work or result data into a buffer for a task
GearmanTask::returnCode - Get the last return code
GearmanTask::sendData - Send data for a task (deprecated)
GearmanTask::sendWorkload - Send data for a task
GearmanTask::taskDenominator - Get completion percentage denominator
GearmanTask::taskNumerator - Get completion percentage numerator
GearmanTask::unique - Get the unique identifier for a task
GearmanTask::uuid - Get the unique identifier for a task (deprecated)
GearmanWorker::addFunction - Register and add callback function
GearmanWorker::addOptions - Add worker options
GearmanWorker::addServer - Add a job server
GearmanWorker::addServers - Add job servers
GearmanWorker::clone - Create a copy of the worker
GearmanWorker::__construct - Create a GearmanWorker instance
GearmanWorker::echo - Test job server response
GearmanWorker::error - Get the last error encountered
GearmanWorker::getErrno - Get errno
GearmanWorker::options - Get worker options
GearmanWorker::register - Register a function with the job server
GearmanWorker::removeOptions - Remove worker options
GearmanWorker::returnCode - Get last Gearman return code
GearmanWorker::setOptions - Set worker options
GearmanWorker::setTimeout - Set socket I/O activity timeout
GearmanWorker::timeout - Get socket I/O activity timeout
GearmanWorker::unregister - Unregister a function name with the job servers
GearmanWorker::unregisterAll - Unregister all function names with the job servers
GearmanWorker::wait - Wait for activity from one of the job servers
GearmanWorker::work - Wait for and perform jobs
Geo IP Location
geoip_continent_code_by_name - Get the two letter continent code
geoip_country_code_by_name - Get the two letter country code
geoip_country_code3_by_name - Get the three letter country code
geoip_country_name_by_name - Get the full country name
geoip_database_info - Get GeoIP Database information
geoip_db_avail - Determine if GeoIP Database is available
geoip_db_filename - Returns the filename of the corresponding GeoIP Database
geoip_db_get_all_info - Returns detailed information about all GeoIP database types
geoip_id_by_name - Get the Internet connection type
geoip_isp_by_name - Get the Internet Service Provider (ISP) name
geoip_org_by_name - Get the organization name
geoip_record_by_name - Returns the detailed City information found in the GeoIP Database
geoip_region_by_name - Get the country code and region
geoip_region_name_by_code - Returns the region name for some country and region code combo
geoip_time_zone_by_country_and_region - Returns the time zone for some country and region code combo
Gettext
bind_textdomain_codeset - Specify the character encoding in which the messages from the DOMAIN message catalog will be returned
bindtextdomain - Sets the path for a domain
dcgettext - Overrides the domain for a single lookup
dcngettext - Plural version of dcgettext
dgettext - Override the current domain
dngettext - Plural version of dgettext
gettext - Lookup a message in the current domain
ngettext - Plural version of gettext
textdomain - Sets the default domain
Gmagick
Gmagick::addimage - Adds new image to Gmagick object image list
Gmagick::addnoiseimage - Adds random noise to the image
Gmagick::annotateimage - Annotates an image with text
Gmagick::blurimage - Adds blur filter to image
Gmagick::borderimage - Surrounds the image with a border
Gmagick::charcoalimage - Simulates a charcoal drawing
Gmagick::chopimage - Removes a region of an image and trims
Gmagick::clear - Clears all resources associated to Gmagick object
Gmagick::commentimage - Adds a comment to your image
Gmagick::compositeimage - Composite one image onto another
Gmagick::__construct - The Gmagick constructor
Gmagick::cropimage - Extracts a region of the image
Gmagick::cropthumbnailimage - Creates a crop thumbnail
Gmagick::current - The current purpose
Gmagick::cyclecolormapimage - Displaces an image's colormap
Gmagick::deconstructimages - Returns certain pixel differences between images
Gmagick::despeckleimage - The despeckleimage purpose
Gmagick::destroy - The destroy purpose
Gmagick::drawimage - Renders the GmagickDraw object on the current image
Gmagick::edgeimage - Enhance edges within the image
Gmagick::embossimage - Returns a grayscale image with a three-dimensional effect
Gmagick::enhanceimage - Improves the quality of a noisy image
Gmagick::equalizeimage - Equalizes the image histogram
Gmagick::flipimage - Creates a vertical mirror image
Gmagick::flopimage - The flopimage purpose
Gmagick::frameimage - Adds a simulated three-dimensional border
Gmagick::gammaimage - Gamma-corrects an image
Gmagick::getcopyright - Returns the GraphicsMagick API copyright as a string
Gmagick::getfilename - The filename associated with an image sequence
Gmagick::getimagebackgroundcolor - Returns the image background color
Gmagick::getimageblueprimary - Returns the chromaticy blue primary point
Gmagick::getimagebordercolor - Returns the image border color
Gmagick::getimagechanneldepth - Gets the depth for a particular image channel
Gmagick::getimagecolors - Returns the color of the specified colormap index
Gmagick::getimagecolorspace - Gets the image colorspace
Gmagick::getimagecompose - Returns the composite operator associated with the image
Gmagick::getimagedelay - Gets the image delay
Gmagick::getimagedepth - Gets the depth of the image
Gmagick::getimagedispose - Gets the image disposal method
Gmagick::getimageextrema - Gets the extrema for the image
Gmagick::getimagefilename - Returns the filename of a particular image in a sequence
Gmagick::getimageformat - Returns the format of a particular image in a sequence
Gmagick::getimagegamma - Gets the image gamma
Gmagick::getimagegreenprimary - Returns the chromaticy green primary point
Gmagick::getimageheight - Returns the image height
Gmagick::getimagehistogram - Gets the image histogram
Gmagick::getimageindex - Gets the index of the current active image
Gmagick::getimageinterlacescheme - Gets the image interlace scheme
Gmagick::getimageiterations - Gets the image iterations
Gmagick::getimagematte - Return if the image has a matte channel
Gmagick::getimagemattecolor - Returns the image matte color
Gmagick::getimageprofile - Returns the named image profile.
Gmagick::getimageredprimary - Returns the chromaticity red primary point
Gmagick::getimagerenderingintent - Gets the image rendering intent
Gmagick::getimageresolution - Gets the image X and Y resolution
Gmagick::getimagescene - Gets the image scene
Gmagick::getimagesignature - Generates an SHA-256 message digest
Gmagick::getimagetype - Gets the potential image type.
Gmagick::getimageunits - Gets the image units of resolution
Gmagick::getimagewhitepoint - Returns the chromaticity white point
Gmagick::getimagewidth - Returns the width of the image
Gmagick::getpackagename - Returns the GraphicsMagick package name.
Gmagick::getquantumdepth - Returns the Gmagick quantum depth as a string.
Gmagick::getreleasedate - Returns the GraphicsMagick release date as a string.
Gmagick::getsamplingfactors - Gets the horizontal and vertical sampling factor.
Gmagick::getsize - Returns the size associated with the Gmagick object
Gmagick::getversion - Returns the GraphicsMagick API version
Gmagick::hasnextimage - Checks if the object has more images
Gmagick::haspreviousimage - Checks if the object has a previous image
Gmagick::implodeimage - Creates a new image as a copy
Gmagick::labelimage - Adds a label to an image.
Gmagick::levelimage - Adjusts the levels of an image
Gmagick::magnifyimage - Scales an image proportionally 2x
Gmagick::mapimage - Replaces the colors of an image with the closest color from a reference image.
Gmagick::medianfilterimage - Applies a digital filter
Gmagick::minifyimage - Scales an image proportionally to half its size
Gmagick::modulateimage - Control the brightness, saturation, and hue
Gmagick::motionblurimage - Simulates motion blur
Gmagick::newimage - Creates a new image
Gmagick::nextimage - Moves to the next image
Gmagick::normalizeimage - Enhances the contrast of a color image
Gmagick::oilpaintimage - Simulates an oil painting
Gmagick::previousimage - Move to the previous image in the object
Gmagick::profileimage - Adds or removes a profile from an image
Gmagick::quantizeimage - Analyzes the colors within a reference image
Gmagick::quantizeimages - The quantizeimages purpose
Gmagick::queryfontmetrics - Returns an array representing the font metrics
Gmagick::queryfonts - Returns the configured fonts
Gmagick::queryformats - Returns formats supported by Gmagick.
Gmagick::radialblurimage - Radial blurs an image
Gmagick::raiseimage - Creates a simulated 3d button-like effect
Gmagick::read - Reads image from filename
Gmagick::readimage - Reads image from filename
Gmagick::readimageblob - Reads image from a binary string
Gmagick::readimagefile - The readimagefile purpose
Gmagick::reducenoiseimage - Smooths the contours of an image
Gmagick::removeimage - Removes an image from the image list
Gmagick::removeimageprofile - Removes the named image profile and returns it
Gmagick::resampleimage - Resample image to desired resolution
Gmagick::resizeimage - Scales an image
Gmagick::rollimage - Offsets an image
Gmagick::rotateimage - Rotates an image
Gmagick::scaleimage - Scales the size of an image
Gmagick::separateimagechannel - Separates a channel from the image
Gmagick::setfilename - Sets the filename before you read or write the image
Gmagick::setimagebackgroundcolor - Sets the image background color.
Gmagick::setimageblueprimary - Sets the image chromaticity blue primary point.
Gmagick::setimagebordercolor - Sets the image border color.
Gmagick::setimagechanneldepth - Sets the depth of a particular image channel
Gmagick::setimagecolorspace - Sets the image colorspace
Gmagick::setimagecompose - Sets the image composite operator
Gmagick::setimagedelay - Sets the image delay
Gmagick::setimagedepth - Sets the image depth
Gmagick::setimagedispose - Sets the image disposal method
Gmagick::setimagefilename - Sets the filename of a particular image in a sequence
Gmagick::setimageformat - Sets the format of a particular image
Gmagick::setimagegamma - Sets the image gamma
Gmagick::setimagegreenprimary - TSets the image chromaticity green primary point.
Gmagick::setimageindex - Set the iterator to the position in the image list specified with the index parameter
Gmagick::setimageinterlacescheme - Sets the interlace scheme of the image.
Gmagick::setimageiterations - Sets the image iterations.
Gmagick::setimageprofile - Adds a named profile to the Gmagick object
Gmagick::setimageredprimary - Sets the image chromaticity red primary point.
Gmagick::setimagerenderingintent - Sets the image rendering intent
Gmagick::setimageresolution - Sets the image resolution
Gmagick::setimagescene - Sets the image scene
Gmagick::setimagetype - Sets the image type
Gmagick::setimageunits - Sets the image units of resolution.
Gmagick::setimagewhitepoint - Sets the image chromaticity white point.
Gmagick::setsamplingfactors - Sets the image sampling factors.
Gmagick::setsize - Sets the size of the Gmagick object
Gmagick::shearimage - Creating a parallelogram
Gmagick::solarizeimage - Applies a solarizing effect to the image
Gmagick::spreadimage - Randomly displaces each pixel in a block
Gmagick::stripimage - Strips an image of all profiles and comments
Gmagick::swirlimage - Swirls the pixels about the center of the image
Gmagick::thumbnailimage - Changes the size of an image
Gmagick::trimimage - Remove edges from the image
Gmagick::write - Writes an image to the specified filename
Gmagick::writeimage - Writes an image to the specified filename
GmagickDraw::annotate - Draws text on the image
GmagickDraw::arc - Draws an arc
GmagickDraw::bezier - Draws a bezier curve
GmagickDraw::ellipse - Draws an ellipse on the image
GmagickDraw::getfillcolor - Returns the fill color
GmagickDraw::getfillopacity - Returns the opacity used when drawing
GmagickDraw::getfont - Returns the font
GmagickDraw::getfontsize - Returns the font pointsize
GmagickDraw::getfontstyle - Returns the font style
GmagickDraw::getfontweight - Returns the font weight
GmagickDraw::getstrokecolor - Returns the color used for stroking object outlines
GmagickDraw::getstrokeopacity - Returns the opacity of stroked object outlines
GmagickDraw::getstrokewidth - Returns the width of the stroke used to draw object outlines
GmagickDraw::gettextdecoration - Returns the text decoration
GmagickDraw::gettextencoding - Returns the code set used for text annotations
GmagickDraw::line - The line purpose
GmagickDraw::point - Draws a point
GmagickDraw::polygon - Draws a polygon
GmagickDraw::polyline - Draws a polyline
GmagickDraw::rectangle - Draws a rectangle
GmagickDraw::rotate - Applies the specified rotation to the current coordinate space
GmagickDraw::roundrectangle - Draws a rounded rectangle
GmagickDraw::scale - Adjusts the scaling factor
GmagickDraw::setfillcolor - Sets the fill color to be used for drawing filled objects.
GmagickDraw::setfillopacity - The setfillopacity purpose
GmagickDraw::setfont - Sets the fully-specified font to use when annotating with text.
GmagickDraw::setfontsize - Sets the font pointsize to use when annotating with text.
GmagickDraw::setfontstyle - Sets the font style to use when annotating with text
GmagickDraw::setfontweight - Sets the font weight
GmagickDraw::setstrokecolor - Sets the color used for stroking object outlines.
GmagickDraw::setstrokeopacity - Specifies the opacity of stroked object outlines.
GmagickDraw::setstrokewidth - Sets the width of the stroke used to draw object outlines.
GmagickDraw::settextdecoration - Specifies a decoration
GmagickDraw::settextencoding - Specifies specifies the text code set
GmagickPixel::__construct - The GmagickPixel constructor
GmagickPixel::getcolor - Returns the color
GmagickPixel::getcolorcount - Returns the color count associated with this color
GmagickPixel::getcolorvalue - Gets the normalized value of the provided color channel
GmagickPixel::setcolor - Sets the color
GmagickPixel::setcolorvalue - Sets the normalized value of one of the channels
GNU Multiple Precision
gmp_abs - Absolute value
gmp_add - Add numbers
gmp_and - Bitwise AND
gmp_clrbit - Clear bit
gmp_cmp - Compare numbers
gmp_com - Calculates one's complement
gmp_div_q - Divide numbers
gmp_div_qr - Divide numbers and get quotient and remainder
gmp_div_r - Remainder of the division of numbers
gmp_div - Alias of gmp_div_q
gmp_divexact - Exact division of numbers
gmp_fact - Factorial
gmp_gcd - Calculate GCD
gmp_gcdext - Calculate GCD and multipliers
gmp_hamdist - Hamming distance
gmp_init - Create GMP number
gmp_intval - Convert GMP number to integer
gmp_invert - Inverse by modulo
gmp_jacobi - Jacobi symbol
gmp_legendre - Legendre symbol
gmp_mod - Modulo operation
gmp_mul - Multiply numbers
gmp_neg - Negate number
gmp_nextprime - Find next prime number
gmp_or - Bitwise OR
gmp_perfect_square - Perfect square check
gmp_popcount - Population count
gmp_pow - Raise number into power
gmp_powm - Raise number into power with modulo
gmp_prob_prime - Check if number is "probably prime"
gmp_random - Random number
gmp_scan0 - Scan for 0
gmp_scan1 - Scan for 1
gmp_setbit - Set bit
gmp_sign - Sign of number
gmp_sqrt - Calculate square root
gmp_sqrtrem - Square root with remainder
gmp_strval - Convert GMP number to string
gmp_sub - Subtract numbers
gmp_testbit - Tests if a bit is set
gmp_xor - Bitwise XOR
GNU Privacy Guard
gnupg_adddecryptkey - Add a key for decryption
gnupg_addencryptkey - Add a key for encryption
gnupg_addsignkey - Add a key for signing
gnupg_cleardecryptkeys - Removes all keys which were set for decryption before
gnupg_clearencryptkeys - Removes all keys which were set for encryption before
gnupg_clearsignkeys - Removes all keys which were set for signing before
gnupg_decrypt - Decrypts a given text
gnupg_decryptverify - Decrypts and verifies a given text
gnupg_encrypt - Encrypts a given text
gnupg_encryptsign - Encrypts and signs a given text
gnupg_export - Exports a key
gnupg_geterror - Returns the errortext, if a function fails
gnupg_getprotocol - Returns the currently active protocol for all operations
gnupg_import - Imports a key
gnupg_init - Initialize a connection
gnupg_keyinfo - Returns an array with information about all keys that matches the given pattern
gnupg_setarmor - Toggle armored output
gnupg_seterrormode - Sets the mode for error_reporting
gnupg_setsignmode - Sets the mode for signing
gnupg_sign - Signs a given text
gnupg_verify - Verifies a signed text
Gupnp
gupnp_context_get_host_ip - Get the IP address
gupnp_context_get_port - Get the port
gupnp_context_get_subscription_timeout - Get the event subscription timeout
gupnp_context_host_path - Start hosting
gupnp_context_new - Create a new context
gupnp_context_set_subscription_timeout - Sets the event subscription timeout
gupnp_context_timeout_add - Sets a function to be called at regular intervals
gupnp_context_unhost_path - Stop hosting
gupnp_control_point_browse_start - Start browsing
gupnp_control_point_browse_stop - Stop browsing
gupnp_control_point_callback_set - Set control point callback
gupnp_control_point_new - Create a new control point
gupnp_device_action_callback_set - Set device callback function
gupnp_device_info_get_service - Get the service with type
gupnp_device_info_get - Get info of root device
gupnp_root_device_get_available - Check whether root device is available
gupnp_root_device_get_relative_location - Get the relative location of root device.
gupnp_root_device_new - Create a new root device
gupnp_root_device_set_available - Set whether or not root_device is available
gupnp_root_device_start - Start main loop
gupnp_root_device_stop - Stop main loop
gupnp_service_action_get - Retrieves the specified action arguments
gupnp_service_action_return_error - Return error code
gupnp_service_action_return - Return succesfully
gupnp_service_action_set - Sets the specified action return values
gupnp_service_freeze_notify - Freeze new notifications
gupnp_service_info_get_introspection - Get resource introspection of service
gupnp_service_info_get - Get full info of service
gupnp_service_introspection_get_state_variable - Returns the state variable data
gupnp_service_notify - Notifies listening clients
gupnp_service_proxy_action_get - Send action to the service and get value
gupnp_service_proxy_action_set - Send action to the service and set value
gupnp_service_proxy_add_notify - Sets up callback for variable change notification
gupnp_service_proxy_callback_set - Set service proxy callback for signal
gupnp_service_proxy_get_subscribed - Check whether subscription is valid to the service
gupnp_service_proxy_remove_notify - Cancels the variable change notification
gupnp_service_proxy_send_action - Send action with multiple parameters synchronously
gupnp_service_proxy_set_subscribed - (Un)subscribes to the service.
gupnp_service_thaw_notify - Sends out any pending notifications and stops queuing of new ones.
Haru PDF
HaruDoc::addPage - Add new page to the document
HaruDoc::addPageLabel - Set the numbering style for the specified range of pages
HaruDoc::__construct - Construct new HaruDoc instance
HaruDoc::createOutline - Create a HaruOutline instance
HaruDoc::getCurrentEncoder - Get HaruEncoder currently used in the document
HaruDoc::getCurrentPage - Return current page of the document
HaruDoc::getEncoder - Get HaruEncoder instance for the specified encoding
HaruDoc::getFont - Get HaruFont instance
HaruDoc::getInfoAttr - Get current value of the specified document attribute
HaruDoc::getPageLayout - Get current page layout
HaruDoc::getPageMode - Get current page mode
HaruDoc::getStreamSize - Get the size of the temporary stream
HaruDoc::insertPage - Insert new page just before the specified page
HaruDoc::loadJPEG - Load a JPEG image
HaruDoc::loadPNG - Load PNG image and return HaruImage instance
HaruDoc::loadRaw - Load a RAW image
HaruDoc::loadTTC - Load the font with the specified index from TTC file
HaruDoc::loadTTF - Load TTF font file
HaruDoc::loadType1 - Load Type1 font
HaruDoc::output - Write the document data to the output buffer
HaruDoc::readFromStream - Read data from the temporary stream
HaruDoc::resetError - Reset error state of the document handle
HaruDoc::resetStream - Rewind the temporary stream
HaruDoc::save - Save the document into the specified file
HaruDoc::saveToStream - Save the document into a temporary stream
HaruDoc::setCompressionMode - Set compression mode for the document
HaruDoc::setCurrentEncoder - Set the current encoder for the document
HaruDoc::setEncryptionMode - Set encryption mode for the document
HaruDoc::setInfoAttr - Set the info attribute of the document
HaruDoc::setInfoDateAttr - Set the datetime info attributes of the document
HaruDoc::setOpenAction - Define which page is shown when the document is opened
HaruDoc::setPageLayout - Set how pages should be displayed
HaruDoc::setPageMode - Set how the document should be displayed
HaruDoc::setPagesConfiguration - Set the number of pages per set of pages
HaruDoc::setPassword - Set owner and user passwords for the document
HaruDoc::setPermission - Set permissions for the document
HaruDoc::useCNSEncodings - Enable Chinese simplified encodings
HaruDoc::useCNSFonts - Enable builtin Chinese simplified fonts
HaruDoc::useCNTEncodings - Enable Chinese traditional encodings
HaruDoc::useCNTFonts - Enable builtin Chinese traditional fonts
HaruDoc::useJPEncodings - Enable Japanese encodings
HaruDoc::useJPFonts - Enable builtin Japanese fonts
HaruDoc::useKREncodings - Enable Korean encodings
HaruDoc::useKRFonts - Enable builtin Korean fonts
HaruPage::arc - Append an arc to the current path
HaruPage::beginText - Begin a text object and set the current text position to (0,0)
HaruPage::circle - Append a circle to the current path
HaruPage::closePath - Append a straight line from the current point to the start point of the path
HaruPage::concat - Concatenate current transformation matrix of the page and the specified matrix
HaruPage::createDestination - Create new HaruDestination instance
HaruPage::createLinkAnnotation - Create new HaruAnnotation instance
HaruPage::createTextAnnotation - Create new HaruAnnotation instance
HaruPage::createURLAnnotation - Create and return new HaruAnnotation instance
HaruPage::curveTo2 - Append a Bezier curve to the current path
HaruPage::curveTo3 - Append a Bezier curve to the current path
HaruPage::curveTo - Append a Bezier curve to the current path
HaruPage::drawImage - Show image at the page
HaruPage::ellipse - Append an ellipse to the current path
HaruPage::endPath - End current path object without filling and painting operations
HaruPage::endText - End current text object
HaruPage::eofill - Fill current path using even-odd rule
HaruPage::eoFillStroke - Fill current path using even-odd rule, then paint the path
HaruPage::fill - Fill current path using nonzero winding number rule
HaruPage::fillStroke - Fill current path using nonzero winding number rule, then paint the path
HaruPage::getCharSpace - Get the current value of character spacing
HaruPage::getCMYKFill - Get the current filling color
HaruPage::getCMYKStroke - Get the current stroking color
HaruPage::getCurrentFont - Get the currently used font
HaruPage::getCurrentFontSize - Get the current font size
HaruPage::getCurrentPos - Get the current position for path painting
HaruPage::getCurrentTextPos - Get the current position for text printing
HaruPage::getDash - Get the current dash pattern
HaruPage::getFillingColorSpace - Get the current filling color space
HaruPage::getFlatness - Get the flatness of the page
HaruPage::getGMode - Get the current graphics mode
HaruPage::getGrayFill - Get the current filling color
HaruPage::getGrayStroke - Get the current stroking color
HaruPage::getHeight - Get the height of the page
HaruPage::getHorizontalScaling - Get the current value of horizontal scaling
HaruPage::getLineCap - Get the current line cap style
HaruPage::getLineJoin - Get the current line join style
HaruPage::getLineWidth - Get the current line width
HaruPage::getMiterLimit - Get the value of miter limit
HaruPage::getRGBFill - Get the current filling color
HaruPage::getRGBStroke - Get the current stroking color
HaruPage::getStrokingColorSpace - Get the current stroking color space
HaruPage::getTextLeading - Get the current value of line spacing
HaruPage::getTextMatrix - Get the current text transformation matrix of the page
HaruPage::getTextRenderingMode - Get the current text rendering mode
HaruPage::getTextRise - Get the current value of text rising
HaruPage::getTextWidth - Get the width of the text using current fontsize, character spacing and word spacing
HaruPage::getTransMatrix - Get the current transformation matrix of the page
HaruPage::getWidth - Get the width of the page
HaruPage::getWordSpace - Get the current value of word spacing
HaruPage::lineTo - Draw a line from the current point to the specified point
HaruPage::measureText - Calculate the number of characters which can be included within the specified width
HaruPage::moveTextPos - Move text position to the specified offset
HaruPage::moveTo - Set starting point for new drawing path
HaruPage::moveToNextLine - Move text position to the start of the next line
HaruPage::rectangle - Append a rectangle to the current path
HaruPage::setCharSpace - Set character spacing for the page
HaruPage::setCMYKFill - Set filling color for the page
HaruPage::setCMYKStroke - Set stroking color for the page
HaruPage::setDash - Set the dash pattern for the page
HaruPage::setFlatness - Set flatness for the page
HaruPage::setFontAndSize - Set font and fontsize for the page
HaruPage::setGrayFill - Set filling color for the page
HaruPage::setGrayStroke - Sets stroking color for the page
HaruPage::setHeight - Set height of the page
HaruPage::setHorizontalScaling - Set horizontal scaling for the page
HaruPage::setLineCap - Set the shape to be used at the ends of lines
HaruPage::setLineJoin - Set line join style for the page
HaruPage::setLineWidth - Set line width for the page
HaruPage::setMiterLimit - Set the current value of the miter limit of the page
HaruPage::setRGBFill - Set filling color for the page
HaruPage::setRGBStroke - Set stroking color for the page
HaruPage::setRotate - Set rotation angle of the page
HaruPage::setSize - Set size and direction of the page
HaruPage::setSlideShow - Set transition style for the page
HaruPage::setTextLeading - Set text leading (line spacing) for the page
HaruPage::setTextMatrix - Set the current text transformation matrix of the page
HaruPage::setTextRenderingMode - Set text rendering mode for the page
HaruPage::setTextRise - Set the current value of text rising
HaruPage::setWidth - Set width of the page
HaruPage::setWordSpace - Set word spacing for the page
HaruPage::showText - Print text at the current position of the page
HaruPage::showTextNextLine - Move the current position to the start of the next line and print the text
HaruPage::stroke - Paint current path
HaruPage::textOut - Print the text on the specified position
HaruPage::textRect - Print the text inside the specified region
HaruFont::getAscent - Get the vertical ascent of the font
HaruFont::getCapHeight - Get the distance from the baseline of uppercase letters
HaruFont::getDescent - Get the vertical descent of the font
HaruFont::getEncodingName - Get the name of the encoding
HaruFont::getFontName - Get the name of the font
HaruFont::getTextWidth - Get the total width of the text, number of characters, number of words and number of spaces
HaruFont::getUnicodeWidth - Get the width of the character in the font
HaruFont::getXHeight - Get the distance from the baseline of lowercase letters
HaruFont::measureText - Calculate the number of characters which can be included within the specified width
HaruImage::getBitsPerComponent - Get the number of bits used to describe each color component of the image
HaruImage::getColorSpace - Get the name of the color space
HaruImage::getHeight - Get the height of the image
HaruImage::getSize - Get size of the image
HaruImage::getWidth - Get the width of the image
HaruImage::setColorMask - Set the color mask of the image
HaruImage::setMaskImage - Set the image mask
HaruEncoder::getByteType - Get the type of the byte in the text
HaruEncoder::getType - Get the type of the encoder
HaruEncoder::getUnicode - Convert the specified character to unicode
HaruEncoder::getWritingMode - Get the writing mode of the encoder
HaruOutline::setDestination - Set the destination for the outline
HaruOutline::setOpened - Set the initial state of the outline
HaruAnnotation::setBorderStyle - Set the border style of the annotation
HaruAnnotation::setHighlightMode - Set the highlighting mode of the annotation
HaruAnnotation::setIcon - Set the icon style of the annotation
HaruAnnotation::setOpened - Set the initial state of the annotation
HaruDestination::setFit - Set the appearance of the page to fit the window
HaruDestination::setFitB - Set the appearance of the page to fit the bounding box of the page within the window
HaruDestination::setFitBH - Set the appearance of the page to fit the width of the bounding box
HaruDestination::setFitBV - Set the appearance of the page to fit the height of the boudning box
HaruDestination::setFitH - Set the appearance of the page to fit the window width
HaruDestination::setFitR - Set the appearance of the page to fit the specified rectangle
HaruDestination::setFitV - Set the appearance of the page to fit the window height
HaruDestination::setXYZ - Set the appearance of the page
HASH Message Digest Framework
hash_algos - Return a list of registered hashing algorithms
hash_copy - Copy hashing context
hash_file - Generate a hash value using the contents of a given file
hash_final - Finalize an incremental hash and return resulting digest
hash_hmac_file - Generate a keyed hash value using the HMAC method and the contents of a given file
hash_hmac - Generate a keyed hash value using the HMAC method
hash_init - Initialize an incremental hashing context
hash_update_file - Pump data into an active hashing context from a file
hash_update_stream - Pump data into an active hashing context from an open stream
hash_update - Pump data into an active hashing context
hash - Generate a hash value (message digest)
htaccess-like support for all SAPIs
HTTP
Installing the HTTP extension - Installation/Configuration
HttpDeflateStream::__construct - HttpDeflateStream class constructor
HttpDeflateStream::factory - HttpDeflateStream class factory
HttpDeflateStream::finish - Finalize deflate stream
HttpDeflateStream::flush - Flush deflate stream
HttpDeflateStream::update - Update deflate stream
HttpInflateStream::__construct - HttpInflateStream class constructor
HttpInflateStream::factory - HttpInflateStream class factory
HttpInflateStream::finish - Finalize inflate stream
HttpInflateStream::flush - Flush inflate stream
HttpInflateStream::update - Update inflate stream
HttpMessage::addHeaders - Add headers
HttpMessage::__construct - HttpMessage constructor
HttpMessage::detach - Detach HttpMessage
HttpMessage::factory - Create HttpMessage from string
HttpMessage::fromEnv - Create HttpMessage from environment
HttpMessage::fromString - Create HttpMessage from string
HttpMessage::getBody - Get message body
HttpMessage::getHeader - Get header
HttpMessage::getHeaders - Get message headers
HttpMessage::getHttpVersion - Get HTTP version
HttpMessage::getParentMessage - Get parent message
HttpMessage::getRequestMethod - Get request method
HttpMessage::getRequestUrl - Get request URL
HttpMessage::getResponseCode - Get response code
HttpMessage::getResponseStatus - Get response status
HttpMessage::getType - Get message type
HttpMessage::guessContentType - Guess content type
HttpMessage::prepend - Prepend message(s)
HttpMessage::reverse - Reverse message chain
HttpMessage::send - Send message
HttpMessage::setBody - Set message body
HttpMessage::setHeaders - Set headers
HttpMessage::setHttpVersion - Set HTTP version
HttpMessage::setRequestMethod - Set request method
HttpMessage::setRequestUrl - Set request URL
HttpMessage::setResponseCode - Set response code
HttpMessage::setResponseStatus - Set response status
HttpMessage::setType - Set message type
HttpMessage::toMessageTypeObject - Create HTTP object regarding message type
HttpMessage::toString - Get string representation
HttpQueryString::__construct - HttpQueryString constructor
HttpQueryString::get - Get (part of) query string
HttpQueryString::mod - Modifiy query string copy
HttpQueryString::set - Set query string params
HttpQueryString::singleton - HttpQueryString singleton
HttpQueryString::toArray - Get query string as array
HttpQueryString::toString - Get query string
HttpQueryString::xlate - Change query strings charset
HttpRequest::addCookies - Add cookies
HttpRequest::addHeaders - Add headers
HttpRequest::addPostFields - Add post fields
HttpRequest::addPostFile - Add post file
HttpRequest::addPutData - Add put data
HttpRequest::addQueryData - Add query data
HttpRequest::addRawPostData - Add raw post data
HttpRequest::addSslOptions - Add ssl options
HttpRequest::clearHistory - Clear history
HttpRequest::__construct - HttpRequest constructor
HttpRequest::enableCookies - Enable cookies
HttpRequest::getContentType - Get content type
HttpRequest::getCookies - Get cookies
HttpRequest::getHeaders - Get headers
HttpRequest::getHistory - Get history
HttpRequest::getMethod - Get method
HttpRequest::getOptions - Get options
HttpRequest::getPostFields - Get post fields
HttpRequest::getPostFiles - Get post files
HttpRequest::getPutData - Get put data
HttpRequest::getPutFile - Get put file
HttpRequest::getQueryData - Get query data
HttpRequest::getRawPostData - Get raw post data
HttpRequest::getRawRequestMessage - Get raw request message
HttpRequest::getRawResponseMessage - Get raw response message
HttpRequest::getRequestMessage - Get request message
HttpRequest::getResponseBody - Get response body
HttpRequest::getResponseCode - Get response code
HttpRequest::getResponseCookies - Get response cookie(s)
HttpRequest::getResponseData - Get response data
HttpRequest::getResponseHeader - Get response header(s)
HttpRequest::getResponseInfo - Get response info
HttpRequest::getResponseMessage - Get response message
HttpRequest::getResponseStatus - Get response status
HttpRequest::getSslOptions - Get ssl options
HttpRequest::getUrl - Get url
HttpRequest::resetCookies - Reset cookies
HttpRequest::send - Send request
HttpRequest::setContentType - Set content type
HttpRequest::setCookies - Set cookies
HttpRequest::setHeaders - Set headers
HttpRequest::setMethod - Set method
HttpRequest::setOptions - Set options
HttpRequest::setPostFields - Set post fields
HttpRequest::setPostFiles - Set post files
HttpRequest::setPutData - Set put data
HttpRequest::setPutFile - Set put file
HttpRequest::setQueryData - Set query data
HttpRequest::setRawPostData - Set raw post data
HttpRequest::setSslOptions - Set ssl options
HttpRequest::setUrl - Set URL
HttpRequestPool::attach - Attach HttpRequest
HttpRequestPool::__construct - HttpRequestPool constructor
HttpRequestPool::__destruct - HttpRequestPool destructor
HttpRequestPool::detach - Detach HttpRequest
HttpRequestPool::getAttachedRequests - Get attached requests
HttpRequestPool::getFinishedRequests - Get finished requests
HttpRequestPool::reset - Reset request pool
HttpRequestPool::send - Send all requests
HttpRequestPool::socketPerform - Perform socket actions
HttpRequestPool::socketSelect - Perform socket select
HttpResponse::capture - Capture script output
HttpResponse::getBufferSize - Get buffer size
HttpResponse::getCache - Get cache
HttpResponse::getCacheControl - Get cache control
HttpResponse::getContentDisposition - Get content disposition
HttpResponse::getContentType - Get content type
HttpResponse::getData - Get data
HttpResponse::getETag - Get ETag
HttpResponse::getFile - Get file
HttpResponse::getGzip - Get gzip
HttpResponse::getHeader - Get header
HttpResponse::getLastModified - Get last modified
HttpResponse::getRequestBody - Get request body
HttpResponse::getRequestBodyStream - Get request body stream
HttpResponse::getRequestHeaders - Get request headers
HttpResponse::getStream - Get Stream
HttpResponse::getThrottleDelay - Get throttle delay
HttpResponse::guessContentType - Guess content type
HttpResponse::redirect - Redirect
HttpResponse::send - Send response
HttpResponse::setBufferSize - Set buffer size
HttpResponse::setCache - Set cache
HttpResponse::setCacheControl - Set cache control
HttpResponse::setContentDisposition - Set content disposition
HttpResponse::setContentType - Set content type
HttpResponse::setData - Set data
HttpResponse::setETag - Set ETag
HttpResponse::setFile - Set file
HttpResponse::setGzip - Set gzip
HttpResponse::setHeader - Set header
HttpResponse::setLastModified - Set last modified
HttpResponse::setStream - Set stream
HttpResponse::setThrottleDelay - Set throttle delay
HttpResponse::status - Send HTTP response status
http_cache_etag - Caching by ETag
http_cache_last_modified - Caching by last modification
http_chunked_decode - Decode chunked-encoded data
http_deflate - Deflate data
http_inflate - Inflate data
http_build_cookie - Build cookie string
http_date - Compose HTTP RFC compliant date
http_get_request_body_stream - Get request body as stream
http_get_request_body - Get request body as string
http_get_request_headers - Get request headers as array
http_match_etag - Match ETag
http_match_modified - Match last modification
http_match_request_header - Match any header
http_support - Check built-in HTTP support
http_negotiate_charset - Negotiate clients preferred character set
http_negotiate_content_type - Negotiate clients preferred content type
http_negotiate_language - Negotiate clients preferred language
ob_deflatehandler - Deflate output handler
ob_etaghandler - ETag output handler
ob_inflatehandler - Inflate output handler
http_parse_cookie - Parse HTTP cookie
http_parse_headers - Parse HTTP headers
http_parse_message - Parse HTTP messages
http_parse_params - Parse parameter list
http_persistent_handles_clean - Clean up persistent handles
http_persistent_handles_count - Stat persistent handles
http_persistent_handles_ident - Get/set ident of persistent handles
http_get - Perform GET request
http_head - Perform HEAD request
http_post_data - Perform POST request with pre-encoded data
http_post_fields - Perform POST request with data to be encoded
http_put_data - Perform PUT request with data
http_put_file - Perform PUT request with file
http_put_stream - Perform PUT request with stream
http_request_body_encode - Encode request body
http_request_method_exists - Check whether request method exists
http_request_method_name - Get request method name
http_request_method_register - Register request method
http_request_method_unregister - Unregister request method
http_request - Perform custom request
http_redirect - Issue HTTP redirect
http_send_content_disposition - Send Content-Disposition
http_send_content_type - Send Content-Type
http_send_data - Send arbitrary data
http_send_file - Send file
http_send_last_modified - Send Last-Modified
http_send_status - Send HTTP response status
http_send_stream - Send stream
http_throttle - HTTP throttling
http_build_str - Build query string
http_build_url - Build a URL
Hyperwave
hw_Array2Objrec - Convert attributes from object array to object record
hw_changeobject - Changes attributes of an object (obsolete)
hw_Children - Object ids of children
hw_ChildrenObj - Object records of children
hw_Close - Closes the Hyperwave connection
hw_Connect - Opens a connection
hw_connection_info - Prints information about the connection to Hyperwave server
hw_cp - Copies objects
hw_Deleteobject - Deletes object
hw_DocByAnchor - Object id object belonging to anchor
hw_DocByAnchorObj - Object record object belonging to anchor
hw_Document_Attributes - Object record of hw_document
hw_Document_BodyTag - Body tag of hw_document
hw_Document_Content - Returns content of hw_document
hw_Document_SetContent - Sets/replaces content of hw_document
hw_Document_Size - Size of hw_document
hw_dummy - Hyperwave dummy function
hw_EditText - Retrieve text document
hw_Error - Error number
hw_ErrorMsg - Returns error message
hw_Free_Document - Frees hw_document
hw_GetAnchors - Object ids of anchors of document
hw_GetAnchorsObj - Object records of anchors of document
hw_GetAndLock - Return object record and lock object
hw_GetChildColl - Object ids of child collections
hw_GetChildCollObj - Object records of child collections
hw_GetChildDocColl - Object ids of child documents of collection
hw_GetChildDocCollObj - Object records of child documents of collection
hw_GetObject - Object record
hw_GetObjectByQuery - Search object
hw_GetObjectByQueryColl - Search object in collection
hw_GetObjectByQueryCollObj - Search object in collection
hw_GetObjectByQueryObj - Search object
hw_GetParents - Object ids of parents
hw_GetParentsObj - Object records of parents
hw_getrellink - Get link from source to dest relative to rootid
hw_GetRemote - Gets a remote document
hw_getremotechildren - Gets children of remote document
hw_GetSrcByDestObj - Returns anchors pointing at object
hw_GetText - Retrieve text document
hw_getusername - Name of currently logged in user
hw_Identify - Identifies as user
hw_InCollections - Check if object ids in collections
hw_Info - Info about connection
hw_InsColl - Insert collection
hw_InsDoc - Insert document
hw_insertanchors - Inserts only anchors into text
hw_InsertDocument - Upload any document
hw_InsertObject - Inserts an object record
hw_mapid - Maps global id on virtual local id
hw_Modifyobject - Modifies object record
hw_mv - Moves objects
hw_New_Document - Create new document
hw_objrec2array - Convert attributes from object record to object array
hw_Output_Document - Prints hw_document
hw_pConnect - Make a persistent database connection
hw_PipeDocument - Retrieve any document
hw_Root - Root object id
hw_setlinkroot - Set the id to which links are calculated
hw_stat - Returns status string
hw_Unlock - Unlock object
hw_Who - List of currently logged in users
Hyperwave API
hw_api_attribute->key - Returns key of the attribute
hw_api_attribute->langdepvalue - Returns value for a given language
hw_api_attribute->value - Returns value of the attribute
hw_api_attribute->values - Returns all values of the attribute
hw_api_attribute - Creates instance of class hw_api_attribute
hw_api->checkin - Checks in an object
hw_api->checkout - Checks out an object
hw_api->children - Returns children of an object
hw_api_content->mimetype - Returns mimetype
hw_api_content->read - Read content
hw_api->content - Returns content of an object
hw_api->copy - Copies physically
hw_api->dbstat - Returns statistics about database server
hw_api->dcstat - Returns statistics about document cache server
hw_api->dstanchors - Returns a list of all destination anchors
hw_api->dstofsrcanchor - Returns destination of a source anchor
hw_api_error->count - Returns number of reasons
hw_api_error->reason - Returns reason of error
hw_api->find - Search for objects
hw_api->ftstat - Returns statistics about fulltext server
hwapi_hgcsp - Returns object of class hw_api
hw_api->hwstat - Returns statistics about Hyperwave server
hw_api->identify - Log into Hyperwave Server
hw_api->info - Returns information about server configuration
hw_api->insert - Inserts a new object
hw_api->insertanchor - Inserts a new object of type anchor
hw_api->insertcollection - Inserts a new object of type collection
hw_api->insertdocument - Inserts a new object of type document
hw_api->link - Creates a link to an object
hw_api->lock - Locks an object
hw_api->move - Moves object between collections
hw_api_content - Create new instance of class hw_api_content
hw_api_object->assign - Clones object
hw_api_object->attreditable - Checks whether an attribute is editable
hw_api_object->count - Returns number of attributes
hw_api_object->insert - Inserts new attribute
hw_api_object - Creates a new instance of class hw_api_object
hw_api_object->remove - Removes attribute
hw_api_object->title - Returns the title attribute
hw_api_object->value - Returns value of attribute
hw_api->object - Retrieve attribute information
hw_api->objectbyanchor - Returns the object an anchor belongs to
hw_api->parents - Returns parents of an object
hw_api_reason->description - Returns description of reason
hw_api_reason->type - Returns type of reason
hw_api->remove - Delete an object
hw_api->replace - Replaces an object
hw_api->setcommittedversion - Commits version other than last version
hw_api->srcanchors - Returns a list of all source anchors
hw_api->srcsofdst - Returns source of a destination object
hw_api->unlock - Unlocks a locked object
hw_api->user - Returns the own user object
hw_api->userlist - Returns a list of all logged in users
Firebird/InterBase
ibase_add_user - Add a user to a security database (only for IB6 or later)
ibase_affected_rows - Return the number of rows that were affected by the previous query
ibase_backup - Initiates a backup task in the service manager and returns immediately
ibase_blob_add - Add data into a newly created blob
ibase_blob_cancel - Cancel creating blob
ibase_blob_close - Close blob
ibase_blob_create - Create a new blob for adding data
ibase_blob_echo - Output blob contents to browser
ibase_blob_get - Get len bytes data from open blob
ibase_blob_import - Create blob, copy file in it, and close it
ibase_blob_info - Return blob length and other useful info
ibase_blob_open - Open blob for retrieving data parts
ibase_close - Close a connection to an InterBase database
ibase_commit_ret - Commit a transaction without closing it
ibase_commit - Commit a transaction
ibase_connect - Open a connection to an InterBase database
ibase_db_info - Request statistics about a database
ibase_delete_user - Delete a user from a security database (only for IB6 or later)
ibase_drop_db - Drops a database
ibase_errcode - Return an error code
ibase_errmsg - Return error messages
ibase_execute - Execute a previously prepared query
ibase_fetch_assoc - Fetch a result row from a query as an associative array
ibase_fetch_object - Get an object from a InterBase database
ibase_fetch_row - Fetch a row from an InterBase database
ibase_field_info - Get information about a field
ibase_free_event_handler - Cancels a registered event handler
ibase_free_query - Free memory allocated by a prepared query
ibase_free_result - Free a result set
ibase_gen_id - Increments the named generator and returns its new value
ibase_maintain_db - Execute a maintenance command on the database server
ibase_modify_user - Modify a user to a security database (only for IB6 or later)
ibase_name_result - Assigns a name to a result set
ibase_num_fields - Get the number of fields in a result set
ibase_num_params - Return the number of parameters in a prepared query
ibase_param_info - Return information about a parameter in a prepared query
ibase_pconnect - Open a persistent connection to an InterBase database
ibase_prepare - Prepare a query for later binding of parameter placeholders and execution
ibase_query - Execute a query on an InterBase database
ibase_restore - Initiates a restore task in the service manager and returns immediately
ibase_rollback_ret - Roll back a transaction without closing it
ibase_rollback - Roll back a transaction
ibase_server_info - Request information about a database server
ibase_service_attach - Connect to the service manager
ibase_service_detach - Disconnect from the service manager
ibase_set_event_handler - Register a callback function to be called when events are posted
ibase_timefmt - Sets the format of timestamp, date and time type columns returned from queries
ibase_trans - Begin a transaction
ibase_wait_event - Wait for an event to be posted by the database
IBM DB2, Cloudscape and Apache Derby
db2_autocommit - Returns or sets the AUTOCOMMIT state for a database connection
db2_bind_param - Binds a PHP variable to an SQL statement parameter
db2_client_info - Returns an object with properties that describe the DB2 database client
db2_close - Closes a database connection
db2_column_privileges - Returns a result set listing the columns and associated privileges for a table
db2_columns - Returns a result set listing the columns and associated metadata for a table
db2_commit - Commits a transaction
db2_conn_error - Returns a string containing the SQLSTATE returned by the last connection attempt
db2_conn_errormsg - Returns the last connection error message and SQLCODE value
db2_connect - Returns a connection to a database
db2_cursor_type - Returns the cursor type used by a statement resource
db2_escape_string - Used to escape certain characters
db2_exec - Executes an SQL statement directly
db2_execute - Executes a prepared SQL statement
db2_fetch_array - Returns an array, indexed by column position, representing a row in a result set
db2_fetch_assoc - Returns an array, indexed by column name, representing a row in a result set
db2_fetch_both - Returns an array, indexed by both column name and position, representing a row in a result set
db2_fetch_object - Returns an object with properties representing columns in the fetched row
db2_fetch_row - Sets the result set pointer to the next row or requested row
db2_field_display_size - Returns the maximum number of bytes required to display a column
db2_field_name - Returns the name of the column in the result set
db2_field_num - Returns the position of the named column in a result set
db2_field_precision - Returns the precision of the indicated column in a result set
db2_field_scale - Returns the scale of the indicated column in a result set
db2_field_type - Returns the data type of the indicated column in a result set
db2_field_width - Returns the width of the current value of the indicated column in a result set
db2_foreign_keys - Returns a result set listing the foreign keys for a table
db2_free_result - Frees resources associated with a result set
db2_free_stmt - Frees resources associated with the indicated statement resource
db2_get_option - Retrieves an option value for a statement resource or a connection resource
db2_last_insert_id - Returns the auto generated ID of the last insert query that successfully
executed on this connection
db2_lob_read - Gets a user defined size of LOB files with each invocation
db2_next_result - Requests the next result set from a stored procedure
db2_num_fields - Returns the number of fields contained in a result set
db2_num_rows - Returns the number of rows affected by an SQL statement
db2_pclose - Closes a persistent database connection
db2_pconnect - Returns a persistent connection to a database
db2_prepare - Prepares an SQL statement to be executed
db2_primary_keys - Returns a result set listing primary keys for a table
db2_procedure_columns - Returns a result set listing stored procedure parameters
db2_procedures - Returns a result set listing the stored procedures registered in a database
db2_result - Returns a single column from a row in the result set
db2_rollback - Rolls back a transaction
db2_server_info - Returns an object with properties that describe the DB2 database server
db2_set_option - Set options for connection or statement resources
db2_special_columns - Returns a result set listing the unique row identifier columns for a table
db2_statistics - Returns a result set listing the index and statistics for a table
db2_stmt_error - Returns a string containing the SQLSTATE returned by an SQL statement
db2_stmt_errormsg - Returns a string containing the last SQL statement error message
db2_table_privileges - Returns a result set listing the tables and associated privileges in a database
db2_tables - Returns a result set listing the tables and associated metadata in a database
iconv
iconv_get_encoding - Retrieve internal configuration variables of iconv extension
iconv_mime_decode_headers - Decodes multiple MIME header fields at once
iconv_mime_decode - Decodes a MIME header field
iconv_mime_encode - Composes a MIME header field
iconv_set_encoding - Set current setting for character encoding conversion
iconv_strlen - Returns the character count of string
iconv_strpos - Finds position of first occurrence of a needle within a haystack
iconv_strrpos - Finds the last occurrence of a needle within a haystack
iconv_substr - Cut out part of a string
iconv - Convert string to requested character encoding
ob_iconv_handler - Convert character encoding as output buffer handler
ID3 Tags
id3_get_frame_long_name - Get the long name of an ID3v2 frame
id3_get_frame_short_name - Get the short name of an ID3v2 frame
id3_get_genre_id - Get the id for a genre
id3_get_genre_list - Get all possible genre values
id3_get_genre_name - Get the name for a genre id
id3_get_tag - Get all information stored in an ID3 tag
id3_get_version - Get version of an ID3 tag
id3_remove_tag - Remove an existing ID3 tag
id3_set_tag - Update information stored in an ID3 tag
Informix
ifx_affected_rows - Get number of rows affected by a query
ifx_blobinfile_mode - Set the default blob mode for all select queries
ifx_byteasvarchar - Set the default byte mode
ifx_close - Close Informix connection
ifx_connect - Open Informix server connection
ifx_copy_blob - Duplicates the given blob object
ifx_create_blob - Creates an blob object
ifx_create_char - Creates an char object
ifx_do - Execute a previously prepared SQL-statement
ifx_error - Returns error code of last Informix call
ifx_errormsg - Returns error message of last Informix call
ifx_fetch_row - Get row as an associative array
ifx_fieldproperties - List of SQL fieldproperties
ifx_fieldtypes - List of Informix SQL fields
ifx_free_blob - Deletes the blob object
ifx_free_char - Deletes the char object
ifx_free_result - Releases resources for the query
ifx_get_blob - Return the content of a blob object
ifx_get_char - Return the content of the char object
ifx_getsqlca - Get the contents of sqlca.sqlerrd[0..5] after a query
ifx_htmltbl_result - Formats all rows of a query into a HTML table
ifx_nullformat - Sets the default return value on a fetch row
ifx_num_fields - Returns the number of columns in the query
ifx_num_rows - Count the rows already fetched from a query
ifx_pconnect - Open persistent Informix connection
ifx_prepare - Prepare an SQL-statement for execution
ifx_query - Send Informix query
ifx_textasvarchar - Set the default text mode
ifx_update_blob - Updates the content of the blob object
ifx_update_char - Updates the content of the char object
ifxus_close_slob - Deletes the slob object
ifxus_create_slob - Creates an slob object and opens it
ifxus_free_slob - Deletes the slob object
ifxus_open_slob - Opens an slob object
ifxus_read_slob - Reads nbytes of the slob object
ifxus_seek_slob - Sets the current file or seek position
ifxus_tell_slob - Returns the current file or seek position
ifxus_write_slob - Writes a string into the slob object
IIS Administration
iis_add_server - Creates a new virtual web server
iis_get_dir_security - Gets Directory Security
iis_get_script_map - Gets script mapping on a virtual directory for a specific extension
iis_get_server_by_comment - Return the instance number associated with the Comment
iis_get_server_by_path - Return the instance number associated with the Path
iis_get_server_rights - Gets server rights
iis_get_service_state - Returns the state for the service defined by ServiceId
iis_remove_server - Removes the virtual web server indicated by ServerInstance
iis_set_app_settings - Creates application scope for a virtual directory
iis_set_dir_security - Sets Directory Security
iis_set_script_map - Sets script mapping on a virtual directory
iis_set_server_rights - Sets server rights
iis_start_server - Starts the virtual web server
iis_start_service - Starts the service defined by ServiceId
iis_stop_server - Stops the virtual web server
iis_stop_service - Stops the service defined by ServiceId
Image Processing and GD
gd_info - Retrieve information about the currently installed GD library
getimagesize - Get the size of an image
image_type_to_extension - Get file extension for image type
image_type_to_mime_type - Get Mime-Type for image-type returned by getimagesize,
exif_read_data, exif_thumbnail, exif_imagetype
image2wbmp - Output image to browser or file
imagealphablending - Set the blending mode for an image
imageantialias - Should antialias functions be used or not
imagearc - Draws an arc
imagechar - Draw a character horizontally
imagecharup - Draw a character vertically
imagecolorallocate - Allocate a color for an image
imagecolorallocatealpha - Allocate a color for an image
imagecolorat - Get the index of the color of a pixel
imagecolorclosest - Get the index of the closest color to the specified color
imagecolorclosestalpha - Get the index of the closest color to the specified color + alpha
imagecolorclosesthwb - Get the index of the color which has the hue, white and blackness
imagecolordeallocate - De-allocate a color for an image
imagecolorexact - Get the index of the specified color
imagecolorexactalpha - Get the index of the specified color + alpha
imagecolormatch - Makes the colors of the palette version of an image more closely match the true color version
imagecolorresolve - Get the index of the specified color or its closest possible alternative
imagecolorresolvealpha - Get the index of the specified color + alpha or its closest possible alternative
imagecolorset - Set the color for the specified palette index
imagecolorsforindex - Get the colors for an index
imagecolorstotal - Find out the number of colors in an image's palette
imagecolortransparent - Define a color as transparent
imageconvolution - Apply a 3x3 convolution matrix, using coefficient and offset
imagecopy - Copy part of an image
imagecopymerge - Copy and merge part of an image
imagecopymergegray - Copy and merge part of an image with gray scale
imagecopyresampled - Copy and resize part of an image with resampling
imagecopyresized - Copy and resize part of an image
imagecreate - Create a new palette based image
imagecreatefromgd2 - Create a new image from GD2 file or URL
imagecreatefromgd2part - Create a new image from a given part of GD2 file or URL
imagecreatefromgd - Create a new image from GD file or URL
imagecreatefromgif - Create a new image from file or URL
imagecreatefromjpeg - Create a new image from file or URL
imagecreatefrompng - Create a new image from file or URL
imagecreatefromstring - Create a new image from the image stream in the string
imagecreatefromwbmp - Create a new image from file or URL
imagecreatefromxbm - Create a new image from file or URL
imagecreatefromxpm - Create a new image from file or URL
imagecreatetruecolor - Create a new true color image
imagedashedline - Draw a dashed line
imagedestroy - Destroy an image
imageellipse - Draw an ellipse
imagefill - Flood fill
imagefilledarc - Draw a partial arc and fill it
imagefilledellipse - Draw a filled ellipse
imagefilledpolygon - Draw a filled polygon
imagefilledrectangle - Draw a filled rectangle
imagefilltoborder - Flood fill to specific color
imagefilter - Applies a filter to an image
imagefontheight - Get font height
imagefontwidth - Get font width
imageftbbox - Give the bounding box of a text using fonts via freetype2
imagefttext - Write text to the image using fonts using FreeType 2
imagegammacorrect - Apply a gamma correction to a GD image
imagegd2 - Output GD2 image to browser or file
imagegd - Output GD image to browser or file
imagegif - Output image to browser or file
imagegrabscreen - Captures the whole screen
imagegrabwindow - Captures a window
imageinterlace - Enable or disable interlace
imageistruecolor - Finds whether an image is a truecolor image
imagejpeg - Output image to browser or file
imagelayereffect - Set the alpha blending flag to use the bundled libgd layering effects
imageline - Draw a line
imageloadfont - Load a new font
imagepalettecopy - Copy the palette from one image to another
imagepng - Output a PNG image to either the browser or a file
imagepolygon - Draws a polygon
imagepsbbox - Give the bounding box of a text rectangle using PostScript Type1 fonts
imagepsencodefont - Change the character encoding vector of a font
imagepsextendfont - Extend or condense a font
imagepsfreefont - Free memory used by a PostScript Type 1 font
imagepsloadfont - Load a PostScript Type 1 font from file
imagepsslantfont - Slant a font
imagepstext - Draws a text over an image using PostScript Type1 fonts
imagerectangle - Draw a rectangle
imagerotate - Rotate an image with a given angle
imagesavealpha - Set the flag to save full alpha channel information (as opposed to single-color transparency) when saving PNG images
imagesetbrush - Set the brush image for line drawing
imagesetpixel - Set a single pixel
imagesetstyle - Set the style for line drawing
imagesetthickness - Set the thickness for line drawing
imagesettile - Set the tile image for filling
imagestring - Draw a string horizontally
imagestringup - Draw a string vertically
imagesx - Get image width
imagesy - Get image height
imagetruecolortopalette - Convert a true color image to a palette image
imagettfbbox - Give the bounding box of a text using TrueType fonts
imagettftext - Write text to the image using TrueType fonts
imagetypes - Return the image types supported by this PHP build
imagewbmp - Output image to browser or file
imagexbm - Output XBM image to browser or file
iptcembed - Embeds binary IPTC data into a JPEG image
iptcparse - Parse a binary IPTC block into single tags.
jpeg2wbmp - Convert JPEG image file to WBMP image file
png2wbmp - Convert PNG image file to WBMP image file
Image Processing (ImageMagick)
Imagick::adaptiveBlurImage - Adds adaptive blur filter to image
Imagick::adaptiveResizeImage - Adaptively resize image with data dependent triangulation
Imagick::adaptiveSharpenImage - Adaptively sharpen the image
Imagick::adaptiveThresholdImage - Selects a threshold for each pixel based on a range of intensity
Imagick::addImage - Adds new image to Imagick object image list
Imagick::addNoiseImage - Adds random noise to the image
Imagick::affineTransformImage - Transforms an image
Imagick::animateImages - Animates an image or images
Imagick::annotateImage - Annotates an image with text
Imagick::appendImages - Append a set of images
Imagick::averageImages - Average a set of images
Imagick::blackThresholdImage - Forces all pixels below the threshold into black
Imagick::blurImage - Adds blur filter to image
Imagick::borderImage - Surrounds the image with a border
Imagick::charcoalImage - Simulates a charcoal drawing
Imagick::chopImage - Removes a region of an image and trims
Imagick::clear - Clears all resources associated to Imagick object
Imagick::clipImage - Clips along the first path from the 8BIM profile
Imagick::clipPathImage - Clips along the named paths from the 8BIM profile
Imagick::clone - Makes an exact copy of the Imagick object
Imagick::clutImage - Replaces colors in the image
Imagick::coalesceImages - Composites a set of images
Imagick::colorFloodfillImage - Changes the color value of any pixel that matches target
Imagick::colorizeImage - Blends the fill color with the image
Imagick::combineImages - Combines one or more images into a single image
Imagick::commentImage - Adds a comment to your image
Imagick::compareImageChannels - Returns the difference in one or more images
Imagick::compareImageLayers - Returns the maximum bounding region between images
Imagick::compareImages - Compares an image to a reconstructed image
Imagick::compositeImage - Composite one image onto another
Imagick::__construct - The Imagick constructor
Imagick::contrastImage - Change the contrast of the image
Imagick::contrastStretchImage - Enhances the contrast of a color image
Imagick::convolveImage - Applies a custom convolution kernel to the image
Imagick::cropImage - Extracts a region of the image
Imagick::cropThumbnailImage - Creates a crop thumbnail
Imagick::current - Returns a reference to the current Imagick object
Imagick::cycleColormapImage - Displaces an image's colormap
Imagick::decipherImage - Deciphers an image
Imagick::deconstructImages - Returns certain pixel differences between images
Imagick::deleteImageArtifact - Delete image artifact
Imagick::deskewImage - Removes skew from the image
Imagick::despeckleImage - Reduces the speckle noise in an image
Imagick::destroy - Destroys the Imagick object
Imagick::displayImage - Displays an image
Imagick::displayImages - Displays an image or image sequence
Imagick::distortImage - Distorts an image using various distortion methods
Imagick::drawImage - Renders the ImagickDraw object on the current image
Imagick::edgeImage - Enhance edges within the image
Imagick::embossImage - Returns a grayscale image with a three-dimensional effect
Imagick::encipherImage - Enciphers an image
Imagick::enhanceImage - Improves the quality of a noisy image
Imagick::equalizeImage - Equalizes the image histogram
Imagick::evaluateImage - Applies an expression to an image
Imagick::exportImagePixels - Exports raw image pixels
Imagick::extentImage - Set image size
Imagick::flattenImages - Merges a sequence of images
Imagick::flipImage - Creates a vertical mirror image
Imagick::floodFillPaintImage - Changes the color value of any pixel that matches target
Imagick::flopImage - Creates a horizontal mirror image
Imagick::frameImage - Adds a simulated three-dimensional border
Imagick::functionImage - Applies a function on the image
Imagick::fxImage - Evaluate expression for each pixel in the image
Imagick::gammaImage - Gamma-corrects an image
Imagick::gaussianBlurImage - Blurs an image
Imagick::getColorspace - Gets the colorspace
Imagick::getCompression - Gets the object compression type
Imagick::getCompressionQuality - Gets the object compression quality
Imagick::getCopyright - Returns the ImageMagick API copyright as a string
Imagick::getFilename - The filename associated with an image sequence
Imagick::getFont - Gets font
Imagick::getFormat - Returns the format of the Imagick object
Imagick::getGravity - Gets the gravity
Imagick::getHomeURL - Returns the ImageMagick home URL
Imagick::getImage - Returns a new Imagick object
Imagick::getImageAlphaChannel - Gets the image alpha channel
Imagick::getImageArtifact - Get image artifact
Imagick::getImageBackgroundColor - Returns the image background color
Imagick::getImageBlob - Returns the image sequence as a blob
Imagick::getImageBluePrimary - Returns the chromaticy blue primary point
Imagick::getImageBorderColor - Returns the image border color
Imagick::getImageChannelDepth - Gets the depth for a particular image channel
Imagick::getImageChannelDistortion - Compares image channels of an image to a reconstructed image
Imagick::getImageChannelDistortions - Gets channel distortions
Imagick::getImageChannelExtrema - Gets the extrema for one or more image channels
Imagick::getImageChannelKurtosis - The getImageChannelKurtosis purpose
Imagick::getImageChannelMean - Gets the mean and standard deviation
Imagick::getImageChannelRange - Gets channel range
Imagick::getImageChannelStatistics - Returns statistics for each channel in the image
Imagick::getImageClipMask - Gets image clip mask
Imagick::getImageColormapColor - Returns the color of the specified colormap index
Imagick::getImageColors - Gets the number of unique colors in the image
Imagick::getImageColorspace - Gets the image colorspace
Imagick::getImageCompose - Returns the composite operator associated with the image
Imagick::getImageCompression - Gets the current image's compression type
Imagick::getImageCompressionQuality - Gets the current image's compression quality
Imagick::getImageDelay - Gets the image delay
Imagick::getImageDepth - Gets the image depth
Imagick::getImageDispose - Gets the image disposal method
Imagick::getImageDistortion - Compares an image to a reconstructed image
Imagick::getImageExtrema - Gets the extrema for the image
Imagick::getImageFilename - Returns the filename of a particular image in a sequence
Imagick::getImageFormat - Returns the format of a particular image in a sequence
Imagick::getImageGamma - Gets the image gamma
Imagick::getImageGeometry - Gets the width and height as an associative array
Imagick::getImageGravity - Gets the image gravity
Imagick::getImageGreenPrimary - Returns the chromaticy green primary point
Imagick::getImageHeight - Returns the image height
Imagick::getImageHistogram - Gets the image histogram
Imagick::getImageIndex - Gets the index of the current active image
Imagick::getImageInterlaceScheme - Gets the image interlace scheme
Imagick::getImageInterpolateMethod - Returns the interpolation method
Imagick::getImageIterations - Gets the image iterations
Imagick::getImageLength - Returns the image length in bytes
Imagick::getImageMagickLicense - Returns a string containing the ImageMagick license
Imagick::getImageMatte - Return if the image has a matte channel
Imagick::getImageMatteColor - Returns the image matte color
Imagick::getImageOrientation - Gets the image orientation
Imagick::getImagePage - Returns the page geometry
Imagick::getImagePixelColor - Returns the color of the specified pixel
Imagick::getImageProfile - Returns the named image profile
Imagick::getImageProfiles - Returns the image profiles
Imagick::getImageProperties - Returns the image properties
Imagick::getImageProperty - Returns the named image property
Imagick::getImageRedPrimary - Returns the chromaticity red primary point
Imagick::getImageRegion - Extracts a region of the image
Imagick::getImageRenderingIntent - Gets the image rendering intent
Imagick::getImageResolution - Gets the image X and Y resolution
Imagick::getImagesBlob - Returns all image sequences as a blob
Imagick::getImageScene - Gets the image scene
Imagick::getImageSignature - Generates an SHA-256 message digest
Imagick::getImageSize - Returns the image length in bytes
Imagick::getImageTicksPerSecond - Gets the image ticks-per-second
Imagick::getImageTotalInkDensity - Gets the image total ink density
Imagick::getImageType - Gets the potential image type
Imagick::getImageUnits - Gets the image units of resolution
Imagick::getImageVirtualPixelMethod - Returns the virtual pixel method
Imagick::getImageWhitePoint - Returns the chromaticity white point
Imagick::getImageWidth - Returns the image width
Imagick::getInterlaceScheme - Gets the object interlace scheme
Imagick::getIteratorIndex - Gets the index of the current active image
Imagick::getNumberImages - Returns the number of images in the object
Imagick::getOption - Returns a value associated with the specified key
Imagick::getPackageName - Returns the ImageMagick package name
Imagick::getPage - Returns the page geometry
Imagick::getPixelIterator - Returns a MagickPixelIterator
Imagick::getPixelRegionIterator - Get an ImagickPixelIterator for an image section
Imagick::getPointSize - Gets point size
Imagick::getQuantumDepth - Gets the quantum depth
Imagick::getQuantumRange - Returns the Imagick quantum range
Imagick::getReleaseDate - Returns the ImageMagick release date
Imagick::getResource - Returns the specified resource's memory usage
Imagick::getResourceLimit - Returns the specified resource limit
Imagick::getSamplingFactors - Gets the horizontal and vertical sampling factor
Imagick::getSize - Returns the size associated with the Imagick object
Imagick::getSizeOffset - Returns the size offset
Imagick::getVersion - Returns the ImageMagick API version
Imagick::haldClutImage - Replaces colors in the image
Imagick::hasNextImage - Checks if the object has more images
Imagick::hasPreviousImage - Checks if the object has a previous image
Imagick::identifyImage - Identifies an image and fetches attributes
Imagick::implodeImage - Creates a new image as a copy
Imagick::importImagePixels - Imports image pixels
Imagick::labelImage - Adds a label to an image
Imagick::levelImage - Adjusts the levels of an image
Imagick::linearStretchImage - Stretches with saturation the image intensity
Imagick::liquidRescaleImage - Animates an image or images
Imagick::magnifyImage - Scales an image proportionally 2x
Imagick::mapImage - Replaces the colors of an image with the closest color from a reference image.
Imagick::matteFloodfillImage - Changes the transparency value of a color
Imagick::medianFilterImage - Applies a digital filter
Imagick::mergeImageLayers - Merges image layers
Imagick::minifyImage - Scales an image proportionally to half its size
Imagick::modulateImage - Control the brightness, saturation, and hue
Imagick::montageImage - Creates a composite image
Imagick::morphImages - Method morphs a set of images
Imagick::mosaicImages - Forms a mosaic from images
Imagick::motionBlurImage - Simulates motion blur
Imagick::negateImage - Negates the colors in the reference image
Imagick::newImage - Creates a new image
Imagick::newPseudoImage - Creates a new image
Imagick::nextImage - Moves to the next image
Imagick::normalizeImage - Enhances the contrast of a color image
Imagick::oilPaintImage - Simulates an oil painting
Imagick::opaquePaintImage - Changes the color value of any pixel that matches target
Imagick::optimizeImageLayers - Removes repeated portions of images to optimize
Imagick::orderedPosterizeImage - Performs an ordered dither
Imagick::paintFloodfillImage - Changes the color value of any pixel that matches target
Imagick::paintOpaqueImage - Change any pixel that matches color
Imagick::paintTransparentImage - Changes any pixel that matches color with the color defined by fill
Imagick::pingImage - Fetch basic attributes about the image
Imagick::pingImageBlob - Quickly fetch attributes
Imagick::pingImageFile - Get basic image attributes in a lightweight manner
Imagick::polaroidImage - Simulates a Polaroid picture
Imagick::posterizeImage - Reduces the image to a limited number of color level
Imagick::previewImages - Quickly pin-point appropriate parameters for image processing
Imagick::previousImage - Move to the previous image in the object
Imagick::profileImage - Adds or removes a profile from an image
Imagick::quantizeImage - Analyzes the colors within a reference image
Imagick::quantizeImages - Analyzes the colors within a sequence of images
Imagick::queryFontMetrics - Returns an array representing the font metrics
Imagick::queryFonts - Returns the configured fonts
Imagick::queryFormats - Returns formats supported by Imagick
Imagick::radialBlurImage - Radial blurs an image
Imagick::raiseImage - Creates a simulated 3d button-like effect
Imagick::randomThresholdImage - Creates a high-contrast, two-color image
Imagick::readImage - Reads image from filename
Imagick::readImageBlob - Reads image from a binary string
Imagick::readImageFile - Reads image from open filehandle
Imagick::recolorImage - Recolors image
Imagick::reduceNoiseImage - Smooths the contours of an image
Imagick::remapImage - Remaps image colors
Imagick::removeImage - Removes an image from the image list
Imagick::removeImageProfile - Removes the named image profile and returns it
Imagick::render - Renders all preceding drawing commands
Imagick::resampleImage - Resample image to desired resolution
Imagick::resetImagePage - Reset image page
Imagick::resizeImage - Scales an image
Imagick::rollImage - Offsets an image
Imagick::rotateImage - Rotates an image
Imagick::roundCorners - Rounds image corners
Imagick::sampleImage - Scales an image with pixel sampling
Imagick::scaleImage - Scales the size of an image
Imagick::segmentImage - Segments an image
Imagick::separateImageChannel - Separates a channel from the image
Imagick::sepiaToneImage - Sepia tones an image
Imagick::setBackgroundColor - Sets the object's default background color
Imagick::setColorspace - Set colorspace
Imagick::setCompression - Sets the object's default compression type
Imagick::setCompressionQuality - Sets the object's default compression quality
Imagick::setFilename - Sets the filename before you read or write the image
Imagick::setFirstIterator - Sets the Imagick iterator to the first image
Imagick::setFont - Sets font
Imagick::setFormat - Sets the format of the Imagick object
Imagick::setGravity - Sets the gravity
Imagick::setImage - Replaces image in the object
Imagick::setImageAlphaChannel - Sets image alpha channel
Imagick::setImageArtifact - Set image artifact
Imagick::setImageBackgroundColor - Sets the image background color
Imagick::setImageBias - Sets the image bias for any method that convolves an image
Imagick::setImageBluePrimary - Sets the image chromaticity blue primary point
Imagick::setImageBorderColor - Sets the image border color
Imagick::setImageChannelDepth - Sets the depth of a particular image channel
Imagick::setImageClipMask - Sets image clip mask
Imagick::setImageColormapColor - Sets the color of the specified colormap index
Imagick::setImageColorspace - Sets the image colorspace
Imagick::setImageCompose - Sets the image composite operator
Imagick::setImageCompression - Sets the image compression
Imagick::setImageCompressionQuality - Sets the image compression quality
Imagick::setImageDelay - Sets the image delay
Imagick::setImageDepth - Sets the image depth
Imagick::setImageDispose - Sets the image disposal method
Imagick::setImageExtent - Sets the image size
Imagick::setImageFilename - Sets the filename of a particular image
Imagick::setImageFormat - Sets the format of a particular image
Imagick::setImageGamma - Sets the image gamma
Imagick::setImageGravity - Sets the image gravity
Imagick::setImageGreenPrimary - Sets the image chromaticity green primary point
Imagick::setImageIndex - Set the iterator position
Imagick::setImageInterlaceScheme - Sets the image compression
Imagick::setImageInterpolateMethod - Sets the image interpolate pixel method
Imagick::setImageIterations - Sets the image iterations
Imagick::setImageMatte - Sets the image matte channel
Imagick::setImageMatteColor - Sets the image matte color
Imagick::setImageOpacity - Sets the image opacity level
Imagick::setImageOrientation - Sets the image orientation
Imagick::setImagePage - Sets the page geometry of the image
Imagick::setImageProfile - Adds a named profile to the Imagick object
Imagick::setImageProperty - Sets an image property
Imagick::setImageRedPrimary - Sets the image chromaticity red primary point
Imagick::setImageRenderingIntent - Sets the image rendering intent
Imagick::setImageResolution - Sets the image resolution
Imagick::setImageScene - Sets the image scene
Imagick::setImageTicksPerSecond - Sets the image ticks-per-second
Imagick::setImageType - Sets the image type
Imagick::setImageUnits - Sets the image units of resolution
Imagick::setImageVirtualPixelMethod - Sets the image virtual pixel method
Imagick::setImageWhitePoint - Sets the image chromaticity white point
Imagick::setInterlaceScheme - Sets the image compression
Imagick::setIteratorIndex - Set the iterator position
Imagick::setLastIterator - Sets the Imagick iterator to the last image
Imagick::setOption - Set an option
Imagick::setPage - Sets the page geometry of the Imagick object
Imagick::setPointSize - Sets point size
Imagick::setResolution - Sets the image resolution
Imagick::setResourceLimit - Sets the limit for a particular resource in megabytes
Imagick::setSamplingFactors - Sets the image sampling factors
Imagick::setSize - Sets the size of the Imagick object
Imagick::setSizeOffset - Sets the size and offset of the Imagick object
Imagick::setType - Sets the image type attribute
Imagick::shadeImage - Creates a 3D effect
Imagick::shadowImage - Simulates an image shadow
Imagick::sharpenImage - Sharpens an image
Imagick::shaveImage - Shaves pixels from the image edges
Imagick::shearImage - Creating a parallelogram
Imagick::sigmoidalContrastImage - Adjusts the contrast of an image
Imagick::sketchImage - Simulates a pencil sketch
Imagick::solarizeImage - Applies a solarizing effect to the image
Imagick::sparseColorImage - Interpolates colors
Imagick::spliceImage - Splices a solid color into the image
Imagick::spreadImage - Randomly displaces each pixel in a block
Imagick::steganoImage - Hides a digital watermark within the image
Imagick::stereoImage - Composites two images
Imagick::stripImage - Strips an image of all profiles and comments
Imagick::swirlImage - Swirls the pixels about the center of the image
Imagick::textureImage - Repeatedly tiles the texture image
Imagick::thresholdImage - Changes the value of individual pixels based on a threshold
Imagick::thumbnailImage - Changes the size of an image
Imagick::tintImage - Applies a color vector to each pixel in the image
Imagick::transformImage - Convenience method for setting crop size and the image geometry
Imagick::transparentPaintImage - Paints pixels transparent
Imagick::transposeImage - Creates a vertical mirror image
Imagick::transverseImage - Creates a horizontal mirror image
Imagick::trimImage - Remove edges from the image
Imagick::uniqueImageColors - Discards all but one of any pixel color
Imagick::unsharpMaskImage - Sharpens an image
Imagick::valid - Checks if the current item is valid
Imagick::vignetteImage - Adds vignette filter to the image
Imagick::waveImage - Applies wave filter to the image
Imagick::whiteThresholdImage - Force all pixels above the threshold into white
Imagick::writeImage - Writes an image to the specified filename
Imagick::writeImageFile - Writes an image to a filehandle
Imagick::writeImages - Writes an image or image sequence
Imagick::writeImagesFile - Writes frames to a filehandle
ImagickDraw::affine - Adjusts the current affine transformation matrix
ImagickDraw::annotation - Draws text on the image
ImagickDraw::arc - Draws an arc
ImagickDraw::bezier - Draws a bezier curve
ImagickDraw::circle - Draws a circle
ImagickDraw::clear - Clears the ImagickDraw
ImagickDraw::clone - Makes an exact copy of the specified ImagickDraw object
ImagickDraw::color - Draws color on image
ImagickDraw::comment - Adds a comment
ImagickDraw::composite - Composites an image onto the current image
ImagickDraw::__construct - The ImagickDraw constructor
ImagickDraw::destroy - Frees all associated resources
ImagickDraw::ellipse - Draws an ellipse on the image
ImagickDraw::getClipPath - Obtains the current clipping path ID
ImagickDraw::getClipRule - Returns the current polygon fill rule
ImagickDraw::getClipUnits - Returns the interpretation of clip path units
ImagickDraw::getFillColor - Returns the fill color
ImagickDraw::getFillOpacity - Returns the opacity used when drawing
ImagickDraw::getFillRule - Returns the fill rule
ImagickDraw::getFont - Returns the font
ImagickDraw::getFontFamily - Returns the font family
ImagickDraw::getFontSize - Returns the font pointsize
ImagickDraw::getFontStyle - Returns the font style
ImagickDraw::getFontWeight - Returns the font weight
ImagickDraw::getGravity - Returns the text placement gravity
ImagickDraw::getStrokeAntialias - Returns the current stroke antialias setting
ImagickDraw::getStrokeColor - Returns the color used for stroking object outlines
ImagickDraw::getStrokeDashArray - Returns an array representing the pattern of dashes and gaps used to stroke paths
ImagickDraw::getStrokeDashOffset - Returns the offset into the dash pattern to start the dash
ImagickDraw::getStrokeLineCap - Returns the shape to be used at the end of open subpaths when they are stroked
ImagickDraw::getStrokeLineJoin - Returns the shape to be used at the corners of paths when they are stroked
ImagickDraw::getStrokeMiterLimit - Returns the stroke miter limit
ImagickDraw::getStrokeOpacity - Returns the opacity of stroked object outlines
ImagickDraw::getStrokeWidth - Returns the width of the stroke used to draw object outlines
ImagickDraw::getTextAlignment - Returns the text alignment
ImagickDraw::getTextAntialias - Returns the current text antialias setting
ImagickDraw::getTextDecoration - Returns the text decoration
ImagickDraw::getTextEncoding - Returns the code set used for text annotations
ImagickDraw::getTextUnderColor - Returns the text under color
ImagickDraw::getVectorGraphics - Returns a string containing vector graphics
ImagickDraw::line - Draws a line
ImagickDraw::matte - Paints on the image's opacity channel
ImagickDraw::pathClose - Adds a path element to the current path
ImagickDraw::pathCurveToAbsolute - Draws a cubic Bezier curve
ImagickDraw::pathCurveToQuadraticBezierAbsolute - Draws a quadratic Bezier curve
ImagickDraw::pathCurveToQuadraticBezierRelative - Draws a quadratic Bezier curve
ImagickDraw::pathCurveToQuadraticBezierSmoothAbsolute - Draws a quadratic Bezier curve
ImagickDraw::pathCurveToQuadraticBezierSmoothRelative - Draws a quadratic Bezier curve
ImagickDraw::pathCurveToRelative - Draws a cubic Bezier curve
ImagickDraw::pathCurveToSmoothAbsolute - Draws a cubic Bezier curve
ImagickDraw::pathCurveToSmoothRelative - Draws a cubic Bezier curve
ImagickDraw::pathEllipticArcAbsolute - Draws an elliptical arc
ImagickDraw::pathEllipticArcRelative - Draws an elliptical arc
ImagickDraw::pathFinish - Terminates the current path
ImagickDraw::pathLineToAbsolute - Draws a line path
ImagickDraw::pathLineToHorizontalAbsolute - Draws a horizontal line path
ImagickDraw::pathLineToHorizontalRelative - Draws a horizontal line
ImagickDraw::pathLineToRelative - Draws a line path
ImagickDraw::pathLineToVerticalAbsolute - Draws a vertical line
ImagickDraw::pathLineToVerticalRelative - Draws a vertical line path
ImagickDraw::pathMoveToAbsolute - Starts a new sub-path
ImagickDraw::pathMoveToRelative - Starts a new sub-path
ImagickDraw::pathStart - Declares the start of a path drawing list
ImagickDraw::point - Draws a point
ImagickDraw::polygon - Draws a polygon
ImagickDraw::polyline - Draws a polyline
ImagickDraw::pop - Destroys the current ImagickDraw in the stack, and returns to the previously pushed ImagickDraw
ImagickDraw::popClipPath - Terminates a clip path definition
ImagickDraw::popDefs - Terminates a definition list
ImagickDraw::popPattern - Terminates a pattern definition
ImagickDraw::push - Clones the current ImagickDraw and pushes it to the stack
ImagickDraw::pushClipPath - Starts a clip path definition
ImagickDraw::pushDefs - Indicates that following commands create named elements for early processing
ImagickDraw::pushPattern - Indicates that subsequent commands up to a ImagickDraw::opPattern() command comprise the definition of a named pattern
ImagickDraw::rectangle - Draws a rectangle
ImagickDraw::render - Renders all preceding drawing commands onto the image
ImagickDraw::rotate - Applies the specified rotation to the current coordinate space
ImagickDraw::roundRectangle - Draws a rounded rectangle
ImagickDraw::scale - Adjusts the scaling factor
ImagickDraw::setClipPath - Associates a named clipping path with the image
ImagickDraw::setClipRule - Set the polygon fill rule to be used by the clipping path
ImagickDraw::setClipUnits - Sets the interpretation of clip path units
ImagickDraw::setFillAlpha - Sets the opacity to use when drawing using the fill color or fill texture
ImagickDraw::setFillColor - Sets the fill color to be used for drawing filled objects
ImagickDraw::setFillOpacity - Sets the opacity to use when drawing using the fill color or fill texture
ImagickDraw::setFillPatternURL - Sets the URL to use as a fill pattern for filling objects
ImagickDraw::setFillRule - Sets the fill rule to use while drawing polygons
ImagickDraw::setFont - Sets the fully-specified font to use when annotating with text
ImagickDraw::setFontFamily - Sets the font family to use when annotating with text
ImagickDraw::setFontSize - Sets the font pointsize to use when annotating with text
ImagickDraw::setFontStretch - Sets the font stretch to use when annotating with text
ImagickDraw::setFontStyle - Sets the font style to use when annotating with text
ImagickDraw::setFontWeight - Sets the font weight
ImagickDraw::setGravity - Sets the text placement gravity
ImagickDraw::setStrokeAlpha - Specifies the opacity of stroked object outlines
ImagickDraw::setStrokeAntialias - Controls whether stroked outlines are antialiased
ImagickDraw::setStrokeColor - Sets the color used for stroking object outlines
ImagickDraw::setStrokeDashArray - Specifies the pattern of dashes and gaps used to stroke paths
ImagickDraw::setStrokeDashOffset - Specifies the offset into the dash pattern to start the dash
ImagickDraw::setStrokeLineCap - Specifies the shape to be used at the end of open subpaths when they are stroked
ImagickDraw::setStrokeLineJoin - Specifies the shape to be used at the corners of paths when they are stroked
ImagickDraw::setStrokeMiterLimit - Specifies the miter limit
ImagickDraw::setStrokeOpacity - Specifies the opacity of stroked object outlines
ImagickDraw::setStrokePatternURL - Sets the pattern used for stroking object outlines
ImagickDraw::setStrokeWidth - Sets the width of the stroke used to draw object outlines
ImagickDraw::setTextAlignment - Specifies a text alignment
ImagickDraw::setTextAntialias - Controls whether text is antialiased
ImagickDraw::setTextDecoration - Specifies a decoration
ImagickDraw::setTextEncoding - Specifies specifies the text code set
ImagickDraw::setTextUnderColor - Specifies the color of a background rectangle
ImagickDraw::setVectorGraphics - Sets the vector graphics
ImagickDraw::setViewbox - Sets the overall canvas size
ImagickDraw::skewX - Skews the current coordinate system in the horizontal direction
ImagickDraw::skewY - Skews the current coordinate system in the vertical direction
ImagickDraw::translate - Applies a translation to the current coordinate system
ImagickPixel::clear - Clears resources associated with this object
ImagickPixel::__construct - The ImagickPixel constructor
ImagickPixel::destroy - Deallocates resources associated with this object
ImagickPixel::getColor - Returns the color
ImagickPixel::getColorAsString - Returns the color as a string
ImagickPixel::getColorCount - Returns the color count associated with this color
ImagickPixel::getColorValue - Gets the normalized value of the provided color channel
ImagickPixel::getHSL - Returns the normalized HSL color of the ImagickPixel object
ImagickPixel::isSimilar - Check the distance between this color and another
ImagickPixel::setColor - Sets the color
ImagickPixel::setColorValue - Sets the normalized value of one of the channels
ImagickPixel::setHSL - Sets the normalized HSL color
ImagickPixelIterator::clear - Clear resources associated with a PixelIterator
ImagickPixelIterator::__construct - The ImagickPixelIterator constructor
ImagickPixelIterator::destroy - Deallocates resources associated with a PixelIterator
ImagickPixelIterator::getCurrentIteratorRow - Returns the current row of ImagickPixel objects
ImagickPixelIterator::getIteratorRow - Returns the current pixel iterator row
ImagickPixelIterator::getNextIteratorRow - Returns the next row of the pixel iterator
ImagickPixelIterator::getPreviousIteratorRow - Returns the previous row
ImagickPixelIterator::newPixelIterator - Returns a new pixel iterator
ImagickPixelIterator::newPixelRegionIterator - Returns a new pixel iterator
ImagickPixelIterator::resetIterator - Resets the pixel iterator
ImagickPixelIterator::setIteratorFirstRow - Sets the pixel iterator to the first pixel row
ImagickPixelIterator::setIteratorLastRow - Sets the pixel iterator to the last pixel row
ImagickPixelIterator::setIteratorRow - Set the pixel iterator row
ImagickPixelIterator::syncIterator - Syncs the pixel iterator
IMAP, POP3 and NNTP
imap_8bit - Convert an 8bit string to a quoted-printable string
imap_alerts - Returns all IMAP alert messages that have occurred
imap_append - Append a string message to a specified mailbox
imap_base64 - Decode BASE64 encoded text
imap_binary - Convert an 8bit string to a base64 string
imap_body - Read the message body
imap_bodystruct - Read the structure of a specified body section of a specific message
imap_check - Check current mailbox
imap_clearflag_full - Clears flags on messages
imap_close - Close an IMAP stream
imap_createmailbox - Create a new mailbox
imap_delete - Mark a message for deletion from current mailbox
imap_deletemailbox - Delete a mailbox
imap_errors - Returns all of the IMAP errors that have occured
imap_expunge - Delete all messages marked for deletion
imap_fetch_overview - Read an overview of the information in the headers of the given message
imap_fetchbody - Fetch a particular section of the body of the message
imap_fetchheader - Returns header for a message
imap_fetchstructure - Read the structure of a particular message
imap_gc - Clears IMAP cache
imap_get_quota - Retrieve the quota level settings, and usage statics per mailbox
imap_get_quotaroot - Retrieve the quota settings per user
imap_getacl - Gets the ACL for a given mailbox
imap_getmailboxes - Read the list of mailboxes, returning detailed information on each one
imap_getsubscribed - List all the subscribed mailboxes
imap_header - Alias of imap_headerinfo
imap_headerinfo - Read the header of the message
imap_headers - Returns headers for all messages in a mailbox
imap_last_error - Gets the last IMAP error that occurred during this page request
imap_list - Read the list of mailboxes
imap_listmailbox - Alias of imap_list
imap_listscan - Returns the list of mailboxes that matches the given text
imap_listsubscribed - Alias of imap_lsub
imap_lsub - List all the subscribed mailboxes
imap_mail_compose - Create a MIME message based on given envelope and body sections
imap_mail_copy - Copy specified messages to a mailbox
imap_mail_move - Move specified messages to a mailbox
imap_mail - Send an email message
imap_mailboxmsginfo - Get information about the current mailbox
imap_mime_header_decode - Decode MIME header elements
imap_msgno - Gets the message sequence number for the given UID
imap_num_msg - Gets the number of messages in the current mailbox
imap_num_recent - Gets the number of recent messages in current mailbox
imap_open - Open an IMAP stream to a mailbox
imap_ping - Check if the IMAP stream is still active
imap_qprint - Convert a quoted-printable string to an 8 bit string
imap_renamemailbox - Rename an old mailbox to new mailbox
imap_reopen - Reopen IMAP stream to new mailbox
imap_rfc822_parse_adrlist - Parses an address string
imap_rfc822_parse_headers - Parse mail headers from a string
imap_rfc822_write_address - Returns a properly formatted email address given the mailbox, host, and personal info
imap_savebody - Save a specific body section to a file
imap_scanmailbox - Alias of imap_listscan
imap_search - This function returns an array of messages matching the given search criteria
imap_set_quota - Sets a quota for a given mailbox
imap_setacl - Sets the ACL for a giving mailbox
imap_setflag_full - Sets flags on messages
imap_sort - Gets and sort messages
imap_status - Returns status information on a mailbox
imap_subscribe - Subscribe to a mailbox
imap_thread - Returns a tree of threaded message
imap_timeout - Set or fetch imap timeout
imap_uid - This function returns the UID for the given message sequence number
imap_undelete - Unmark the message which is marked deleted
imap_unsubscribe - Unsubscribe from a mailbox
imap_utf7_decode - Decodes a modified UTF-7 encoded string
imap_utf7_encode - Converts ISO-8859-1 string to modified UTF-7 text
imap_utf8 - Converts MIME-encoded text to UTF-8
Inclusion hierarchy viewer
inclued_get_data - Get the inclued data
PHP Options and Information
assert_options - Set/get the various assert flags
assert - Checks if assertion is FALSE
dl - Loads a PHP extension at runtime
extension_loaded - Find out whether an extension is loaded
gc_collect_cycles - Forces collection of any existing garbage cycles
gc_disable - Deactivates the circular reference collector
gc_enable - Activates the circular reference collector
gc_enabled - Returns status of the circular reference collector
get_cfg_var - Gets the value of a PHP configuration option
get_current_user - Gets the name of the owner of the current PHP script
get_defined_constants - Returns an associative array with the names of all the constants and their values
get_extension_funcs - Returns an array with the names of the functions of a module
get_include_path - Gets the current include_path configuration option
get_included_files - Returns an array with the names of included or required files
get_loaded_extensions - Returns an array with the names of all modules compiled and loaded
get_magic_quotes_gpc - Gets the current configuration setting of magic_quotes_gpc
get_magic_quotes_runtime - Gets the current active configuration setting of magic_quotes_runtime
get_required_files - Alias of get_included_files
getenv - Gets the value of an environment variable
getlastmod - Gets time of last page modification
getmygid - Get PHP script owner's GID
getmyinode - Gets the inode of the current script
getmypid - Gets PHP's process ID
getmyuid - Gets PHP script owner's UID
getopt - Gets options from the command line argument list
getrusage - Gets the current resource usages
ini_alter - Alias of ini_set
ini_get_all - Gets all configuration options
ini_get - Gets the value of a configuration option
ini_restore - Restores the value of a configuration option
ini_set - Sets the value of a configuration option
magic_quotes_runtime - Alias of set_magic_quotes_runtime
main - Dummy for main
memory_get_peak_usage - Returns the peak of memory allocated by PHP
memory_get_usage - Returns the amount of memory allocated to PHP
php_ini_loaded_file - Retrieve a path to the loaded php.ini file
php_ini_scanned_files - Return a list of .ini files parsed from the additional ini dir
php_logo_guid - Gets the logo guid
php_sapi_name - Returns the type of interface between web server and PHP
php_uname - Returns information about the operating system PHP is running on
phpcredits - Prints out the credits for PHP
phpinfo - Outputs information about PHP's configuration
phpversion - Gets the current PHP version
putenv - Sets the value of an environment variable
restore_include_path - Restores the value of the include_path configuration option
set_include_path - Sets the include_path configuration option
set_magic_quotes_runtime - Sets the current active configuration setting of magic_quotes_runtime
set_time_limit - Limits the maximum execution time
sys_get_temp_dir - Returns directory path used for temporary files
version_compare - Compares two "PHP-standardized" version number strings
zend_logo_guid - Gets the Zend guid
zend_thread_id - Returns a unique identifier for the current thread
zend_version - Gets the version of the current Zend engine
Ingres DBMS, EDBC, and Enterprise Access Gateways
ingres_autocommit_state - Test if the connection is using autocommit
ingres_autocommit - Switch autocommit on or off
ingres_charset - Returns the installation character set
ingres_close - Close an Ingres database connection
ingres_commit - Commit a transaction
ingres_connect - Open a connection to an Ingres database
ingres_cursor - Get a cursor name for a given result resource
ingres_errno - Get the last Ingres error number generated
ingres_error - Get a meaningful error message for the last error generated
ingres_errsqlstate - Get the last SQLSTATE error code generated
ingres_escape_string - Escape special characters for use in a query
ingres_execute - Execute a prepared query
ingres_fetch_array - Fetch a row of result into an array
ingres_fetch_assoc - Fetch a row of result into an associative array
ingres_fetch_object - Fetch a row of result into an object
ingres_fetch_proc_return - Get the return value from a procedure call
ingres_fetch_row - Fetch a row of result into an enumerated array
ingres_field_length - Get the length of a field
ingres_field_name - Get the name of a field in a query result
ingres_field_nullable - Test if a field is nullable
ingres_field_precision - Get the precision of a field
ingres_field_scale - Get the scale of a field
ingres_field_type - Get the type of a field in a query result
ingres_free_result - Free the resources associated with a result identifier
ingres_next_error - Get the next Ingres error
ingres_num_fields - Get the number of fields returned by the last query
ingres_num_rows - Get the number of rows affected or returned by a query
ingres_pconnect - Open a persistent connection to an Ingres database
ingres_prepare - Prepare a query for later execution
ingres_query - Send an SQL query to Ingres
ingres_result_seek - Set the row position before fetching data
ingres_rollback - Roll back a transaction
ingres_set_environment - Set environment features controlling output options
ingres_unbuffered_query - Send an unbuffered SQL query to Ingres
Inotify
inotify_add_watch - Add a watch to an initialized inotify instance
inotify_init - Initialize an inotify instance
inotify_queue_len - Return a number upper than zero if there are pending events
inotify_read - Read events from an inotify instance
inotify_rm_watch - Remove an existing watch from an inotify instance
Internationalization Functions
Collator::asort - Sort array maintaining index association
Collator::compare - Compare two Unicode strings
Collator::__construct - Create a collator
Collator::create - Create a collator
Collator::getAttribute - Get collation attribute value
Collator::getErrorCode - Get collator's last error code
Collator::getErrorMessage - Get text for collator's last error code
Collator::getLocale - Get the locale name of the collator
Collator::getSortKey - Get sorting key for a string
Collator::getStrength - Get current collation strength
Collator::setAttribute - Set collation attribute
Collator::setStrength - Set collation strength
Collator::sortWithSortKeys - Sort array using specified collator and sort keys
Collator::sort - Sort array using specified collator
NumberFormatter::create - Create a number formatter
NumberFormatter::formatCurrency - Format a currency value
NumberFormatter::format - Format a number
NumberFormatter::getAttribute - Get an attribute
NumberFormatter::getErrorCode - Get formatter's last error code.
NumberFormatter::getErrorMessage - Get formatter's last error message.
NumberFormatter::getLocale - Get formatter locale
NumberFormatter::getPattern - Get formatter pattern
NumberFormatter::getSymbol - Get a symbol value
NumberFormatter::getTextAttribute - Get a text attribute
NumberFormatter::parseCurrency - Parse a currency number
NumberFormatter::parse - Parse a number
NumberFormatter::setAttribute - Set an attribute
NumberFormatter::setPattern - Set formatter pattern
NumberFormatter::setSymbol - Set a symbol value
NumberFormatter::setTextAttribute - Set a text attribute
Locale::acceptFromHttp - Tries to find out best available locale based on HTTP "Accept-Language" header
Locale::composeLocale - Returns a correctly ordered and delimited locale ID
Locale::filterMatches - Checks if a language tag filter matches with locale
Locale::getAllVariants - Gets the variants for the input locale
Locale::getDefault - Gets the default locale value from the INTL global 'default_locale'
Locale::getDisplayLanguage - Returns an appropriately localized display name for language of the inputlocale
Locale::getDisplayName - Returns an appropriately localized display name for the input locale
Locale::getDisplayRegion - Returns an appropriately localized display name for region of the input locale
Locale::getDisplayScript - Returns an appropriately localized display name for script of the input locale
Locale::getDisplayVariant - Returns an appropriately localized display name for variants of the input locale
Locale::getKeywords - Gets the keywords for the input locale
Locale::getPrimaryLanguage - Gets the primary language for the input locale
Locale::getRegion - Gets the region for the input locale
Locale::getScript - Gets the script for the input locale
Locale::lookup - Searches the language tag list for the best match to the language
Locale::parseLocale - Returns a key-value array of locale ID subtag elements.
Locale::setDefault - sets the default runtime locale
Normalizer::isNormalized - Checks if the provided string is already in the specified normalization
form.
Normalizer::normalize - Normalizes the input provided and returns the normalized string
MessageFormatter::create - Constructs a new Message Formatter
MessageFormatter::formatMessage - Quick format message
MessageFormatter::format - Format the message
MessageFormatter::getErrorCode - Get the error code from last operation
MessageFormatter::getErrorMessage - Get the error text from the last operation
MessageFormatter::getLocale - Get the locale for which the formatter was created.
MessageFormatter::getPattern - Get the pattern used by the formatter
MessageFormatter::parseMessage - Quick parse input string
MessageFormatter::parse - Parse input string according to pattern
MessageFormatter::setPattern - Set the pattern used by the formatter
IntlDateFormatter::create - Create a date formatter
IntlDateFormatter::format - Format the date/time value as a string
IntlDateFormatter::getCalendar - Get the calendar used for the IntlDateFormatter
IntlDateFormatter::getDateType - Get the datetype used for the IntlDateFormatter
IntlDateFormatter::getErrorCode - Get the error code from last operation
IntlDateFormatter::getErrorMessage - Get the error text from the last operation.
IntlDateFormatter::getLocale - Get the locale used by formatter
IntlDateFormatter::getPattern - Get the pattern used for the IntlDateFormatter
IntlDateFormatter::getTimeType - Get the timetype used for the IntlDateFormatter
IntlDateFormatter::getTimeZoneId - Get the timezone-id used for the IntlDateFormatter
IntlDateFormatter::isLenient - Get the lenient used for the IntlDateFormatter
IntlDateFormatter::localtime - Parse string to a field-based time value
IntlDateFormatter::parse - Parse string to a timestamp value
IntlDateFormatter::setCalendar - sets the calendar used to the appropriate calendar, which must be
IntlDateFormatter::setLenient - Set the leniency of the parser
IntlDateFormatter::setPattern - Set the pattern used for the IntlDateFormatter
IntlDateFormatter::setTimeZoneId - Sets the time zone to use
ResourceBundle::count - Get number of elements in the bundle
ResourceBundle::create - Create a resource bundle
ResourceBundle::getErrorCode - Get bundle's last error code.
ResourceBundle::getErrorMessage - Get bundle's last error message.
ResourceBundle::get - Get data from the bundle
ResourceBundle::getLocales - Get supported locales
grapheme_extract - Function to extract a sequence of default grapheme clusters from a text buffer, which must be encoded in UTF-8.
grapheme_stripos - Find position (in grapheme units) of first occurrence of a case-insensitive string
grapheme_stristr - Returns part of haystack string from the first occurrence of case-insensitive needle to the end of haystack.
grapheme_strlen - Get string length in grapheme units
grapheme_strpos - Find position (in grapheme units) of first occurrence of a string
grapheme_strripos - Find position (in grapheme units) of last occurrence of a case-insensitive string
grapheme_strrpos - Find position (in grapheme units) of last occurrence of a string
grapheme_strstr - Returns part of haystack string from the first occurrence of needle to the end of haystack.
grapheme_substr - Return part of a string
idn_to_ascii - Convert domain name to IDNA ASCII form.
idn_to_unicode - Alias of idn_to_utf8
idn_to_utf8 - Convert domain name from IDNA ASCII to Unicode.
intl_error_name - Get symbolic name for a given error code
intl_get_error_code - Get the last error code
intl_get_error_message - Get description of the last error
intl_is_failure - Check whether the given error code indicates failure
PHP / Java Integration
java_last_exception_clear - Clear last Java exception
java_last_exception_get - Get last Java exception
JavaScript Object Notation
json_decode - Decodes a JSON string
json_encode - Returns the JSON representation of a value
json_last_error - Returns the last error occurred
Kerberos V
kadm5_chpass_principal - Changes the principal's password
kadm5_create_principal - Creates a kerberos principal with the given parameters
kadm5_delete_principal - Deletes a kerberos principal
kadm5_destroy - Closes the connection to the admin server and releases all related resources
kadm5_flush - Flush all changes to the Kerberos database
kadm5_get_policies - Gets all policies from the Kerberos database
kadm5_get_principal - Gets the principal's entries from the Kerberos database
kadm5_get_principals - Gets all principals from the Kerberos database
kadm5_init_with_password - Opens a connection to the KADM5 library
kadm5_modify_principal - Modifies a kerberos principal with the given parameters
KTaglib
KTaglib_MPEG_File::__construct - Opens a new file
KTaglib_MPEG_File::getAudioProperties - Returns an object that provides access to the audio properties
KTaglib_MPEG_File::getID3v1Tag - Returns an object representing an ID3v1 tag
KTaglib_MPEG_File::getID3v2Tag - Returns a ID3v2 object
KTaglib_MPEG_AudioProperties::getBitrate - Returns the bitrate of the MPEG file
KTaglib_MPEG_AudioProperties::getChannels - Returns the amount of channels of a MPEG file
KTaglib_MPEG_AudioProperties::getLayer - Returns the layer of a MPEG file
KTaglib_MPEG_AudioProperties::getLength - Returns the length of a MPEG file
KTaglib_MPEG_AudioProperties::getSampleBitrate - Returns the sample bitrate of a MPEG file
KTaglib_MPEG_AudioProperties::getVersion - Returns the version of a MPEG file
KTaglib_MPEG_AudioProperties::isCopyrighted - Returns the length of a MPEG file
KTaglib_MPEG_AudioProperties::isOriginal - Returns the length of a MPEG file
KTaglib_MPEG_AudioProperties::isProtectionEnabled - Returns the length of a MPEG file
KTaglib_Tag::getAlbum - Returns the title string from a ID3 tag
KTaglib_Tag::getArtist - Returns the artist string from a ID3 tag
KTaglib_Tag::getComment - Returns the comment from a ID3 tag
KTaglib_Tag::getGenre - Returns the genre from a ID3 tag
KTaglib_Tag::getTitle - Returns the title string from a ID3 tag
KTaglib_Tag::getTrack - Returns the track number from a ID3 tag
KTaglib_Tag::getYear - Returns the year from a ID3 tag
KTaglib_Tag::isEmpty - Returns true if the tag is empty
KTaglib_ID3v2_Tag::addFrame - Add a frame to the ID3v2 tag
KTaglib_ID3v2_Tag::getFrameList - Returns an array of ID3v2 frames, associated with the ID3v2 tag
KTaglib_ID3v2_Frame::getSize - Returns the size of the frame in bytes
KTaglib_ID3v2_Frame::__toString - Returns a string representation of the frame
KTaglib_ID3v2_AttachedPictureFrame::getDescription - Returns a description for the picture in a picture frame
KTaglib_ID3v2_AttachedPictureFrame::getMimeType - Returns the mime type of the picture
KTaglib_ID3v2_AttachedPictureFrame::getType - Returns the type of the image
KTaglib_ID3v2_AttachedPictureFrame::savePicture - Saves the picture to a file
KTaglib_ID3v2_AttachedPictureFrame::setMimeType - Set's the mime type of the picture
KTaglib_ID3v2_AttachedPictureFrame::setPicture - Sets the frame picture to the given image
KTaglib_ID3v2_AttachedPictureFrame::setType - Set the type of the image
Lightweight Directory Access Protocol
ldap_8859_to_t61 - Translate 8859 characters to t61 characters
ldap_add - Add entries to LDAP directory
ldap_bind - Bind to LDAP directory
ldap_close - Alias of ldap_unbind
ldap_compare - Compare value of attribute found in entry specified with DN
ldap_connect - Connect to an LDAP server
ldap_count_entries - Count the number of entries in a search
ldap_delete - Delete an entry from a directory
ldap_dn2ufn - Convert DN to User Friendly Naming format
ldap_err2str - Convert LDAP error number into string error message
ldap_errno - Return the LDAP error number of the last LDAP command
ldap_error - Return the LDAP error message of the last LDAP command
ldap_explode_dn - Splits DN into its component parts
ldap_first_attribute - Return first attribute
ldap_first_entry - Return first result id
ldap_first_reference - Return first reference
ldap_free_result - Free result memory
ldap_get_attributes - Get attributes from a search result entry
ldap_get_dn - Get the DN of a result entry
ldap_get_entries - Get all result entries
ldap_get_option - Get the current value for given option
ldap_get_values_len - Get all binary values from a result entry
ldap_get_values - Get all values from a result entry
ldap_list - Single-level search
ldap_mod_add - Add attribute values to current attributes
ldap_mod_del - Delete attribute values from current attributes
ldap_mod_replace - Replace attribute values with new ones
ldap_modify - Modify an LDAP entry
ldap_next_attribute - Get the next attribute in result
ldap_next_entry - Get next result entry
ldap_next_reference - Get next reference
ldap_parse_reference - Extract information from reference entry
ldap_parse_result - Extract information from result
ldap_read - Read an entry
ldap_rename - Modify the name of an entry
ldap_sasl_bind - Bind to LDAP directory using SASL
ldap_search - Search LDAP tree
ldap_set_option - Set the value of the given option
ldap_set_rebind_proc - Set a callback function to do re-binds on referral chasing
ldap_sort - Sort LDAP result entries
ldap_start_tls - Start TLS
ldap_t61_to_8859 - Translate t61 characters to 8859 characters
ldap_unbind - Unbind from LDAP directory
Libevent
event_add - Add an event to the set of monitored events
event_base_free - Destroy event base
event_base_loop - Handle events
event_base_loopbreak - Abort event loop
event_base_loopexit - Exit loop after a time
event_base_new - Create and initialize new event base
event_base_priority_init - Set the number of event priority levels
event_base_set - Associate event base with an event
event_buffer_base_set - Associate buffered event with an event base
event_buffer_disable - Disable a buffered event
event_buffer_enable - Enable a buffered event
event_buffer_fd_set - Change a buffered event file descriptor
event_buffer_free - Destroy buffered event
event_buffer_new - Create new buffered event
event_buffer_priority_set - Assign a priority to a buffered event
event_buffer_read - Read data from a buffered event
event_buffer_set_callback - Set or reset callbacks for a buffered event
event_buffer_timeout_set - Set read and write timeouts for a buffered event
event_buffer_watermark_set - Set the watermarks for read and write events
event_buffer_write - Write data to a buffered event
event_del - Remove an event from the set of monitored events
event_free - Free event resource
event_new - Create new event
event_set - Prepare an event
libxml
libxml_clear_errors - Clear libxml error buffer
libxml_disable_entity_loader - Disable the ability to load external entities
libxml_get_errors - Retrieve array of errors
libxml_get_last_error - Retrieve last error from libxml
libxml_set_streams_context - Set the streams context for the next libxml document load or write
libxml_use_internal_errors - Disable libxml errors and allow user to fetch error information as needed
LZF
lzf_compress - LZF compression
lzf_decompress - LZF decompression
lzf_optimized_for - Determines what LZF extension was optimized for
ezmlm_hash - Calculate the hash value needed by EZMLM
mail - Send mail
Mailparse
mailparse_determine_best_xfer_encoding - Gets the best way of encoding
mailparse_msg_create - Create a mime mail resource
mailparse_msg_extract_part_file - Extracts/decodes a message section
mailparse_msg_extract_part - Extracts/decodes a message section
mailparse_msg_extract_whole_part_file - Extracts a message section including headers without decoding the transfer encoding
mailparse_msg_free - Frees a MIME resource
mailparse_msg_get_part_data - Returns an associative array of info about the message
mailparse_msg_get_part - Returns a handle on a given section in a mimemessage
mailparse_msg_get_structure - Returns an array of mime section names in the supplied message
mailparse_msg_parse_file - Parses a file
mailparse_msg_parse - Incrementally parse data into buffer
mailparse_rfc822_parse_addresses - Parse RFC 822 compliant addresses
mailparse_stream_encode - Streams data from source file pointer, apply encoding and write to destfp
mailparse_uudecode_all - Scans the data from fp and extract each embedded uuencoded file
Mathematical Functions
abs - Absolute value
acos - Arc cosine
acosh - Inverse hyperbolic cosine
asin - Arc sine
asinh - Inverse hyperbolic sine
atan2 - Arc tangent of two variables
atan - Arc tangent
atanh - Inverse hyperbolic tangent
base_convert - Convert a number between arbitrary bases
bindec - Binary to decimal
ceil - Round fractions up
cos - Cosine
cosh - Hyperbolic cosine
decbin - Decimal to binary
dechex - Decimal to hexadecimal
decoct - Decimal to octal
deg2rad - Converts the number in degrees to the radian equivalent
exp - Calculates the exponent of e
expm1 - Returns exp(number) - 1, computed in a way that is accurate even
when the value of number is close to zero
floor - Round fractions down
fmod - Returns the floating point remainder (modulo) of the division
of the arguments
getrandmax - Show largest possible random value
hexdec - Hexadecimal to decimal
hypot - Calculate the length of the hypotenuse of a right-angle triangle
is_finite - Finds whether a value is a legal finite number
is_infinite - Finds whether a value is infinite
is_nan - Finds whether a value is not a number
lcg_value - Combined linear congruential generator
log10 - Base-10 logarithm
log1p - Returns log(1 + number), computed in a way that is accurate even when
the value of number is close to zero
log - Natural logarithm
max - Find highest value
min - Find lowest value
mt_getrandmax - Show largest possible random value
mt_rand - Generate a better random value
mt_srand - Seed the better random number generator
octdec - Octal to decimal
pi - Get value of pi
pow - Exponential expression
rad2deg - Converts the radian number to the equivalent number in degrees
rand - Generate a random integer
round - Rounds a float
sin - Sine
sinh - Hyperbolic sine
sqrt - Square root
srand - Seed the random number generator
tan - Tangent
tanh - Hyperbolic tangent
MaxDB
maxdb_affected_rows - Gets the number of affected rows in a previous MaxDB operation
maxdb_autocommit - Turns on or off auto-commiting database modifications
maxdb_bind_param - Alias of maxdb_stmt_bind_param
maxdb_bind_result - Alias of maxdb_stmt_bind_result
maxdb_change_user - Changes the user of the specified database connection
maxdb_character_set_name - Returns the default character set for the database connection
maxdb_client_encoding - Alias of maxdb_character_set_name
maxdb_close_long_data - Alias of maxdb_stmt_close_long_data
maxdb_close - Closes a previously opened database connection
maxdb_commit - Commits the current transaction
maxdb_connect_errno - Returns the error code from last connect call
maxdb_connect_error - Returns a string description of the last connect error
maxdb_connect - Open a new connection to the MaxDB server
maxdb_data_seek - Adjusts the result pointer to an arbitary row in the result
maxdb_debug - Performs debugging operations
maxdb_disable_reads_from_master - Disable reads from master
maxdb_disable_rpl_parse - Disable RPL parse
maxdb_dump_debug_info - Dump debugging information into the log
maxdb_embedded_connect - Open a connection to an embedded MaxDB server
maxdb_enable_reads_from_master - Enable reads from master
maxdb_enable_rpl_parse - Enable RPL parse
maxdb_errno - Returns the error code for the most recent function call
maxdb_error - Returns a string description of the last error
maxdb_escape_string - Alias of maxdb_real_escape_string
maxdb_execute - Alias of maxdb_stmt_execute
maxdb_fetch_array - Fetch a result row as an associative, a numeric array, or both
maxdb_fetch_assoc - Fetch a result row as an associative array
maxdb_fetch_field_direct - Fetch meta-data for a single field
maxdb_fetch_field - Returns the next field in the result set
maxdb_fetch_fields - Returns an array of resources representing the fields in a result set
maxdb_fetch_lengths - Returns the lengths of the columns of the current row in the result set
maxdb_fetch_object - Returns the current row of a result set as an object
maxdb_fetch_row - Get a result row as an enumerated array
maxdb_fetch - Alias of maxdb_stmt_fetch
maxdb_field_count - Returns the number of columns for the most recent query
maxdb_field_seek - Set result pointer to a specified field offset
maxdb_field_tell - Get current field offset of a result pointer
maxdb_free_result - Frees the memory associated with a result
maxdb_get_client_info - Returns the MaxDB client version as a string
maxdb_get_client_version - Get MaxDB client info
maxdb_get_host_info - Returns a string representing the type of connection used
maxdb_get_metadata - Alias of maxdb_stmt_result_metadata
maxdb_get_proto_info - Returns the version of the MaxDB protocol used
maxdb_get_server_info - Returns the version of the MaxDB server
maxdb_get_server_version - Returns the version of the MaxDB server as an integer
maxdb_info - Retrieves information about the most recently executed query
maxdb_init - Initializes MaxDB and returns an resource for use with maxdb_real_connect
maxdb_insert_id - Returns the auto generated id used in the last query
maxdb_kill - Disconnects from a MaxDB server
maxdb_master_query - Enforce execution of a query on the master in a master/slave setup
maxdb_more_results - Check if there any more query results from a multi query
maxdb_multi_query - Performs a query on the database
maxdb_next_result - Prepare next result from multi_query
maxdb_num_fields - Get the number of fields in a result
maxdb_num_rows - Gets the number of rows in a result
maxdb_options - Set options
maxdb_param_count - Alias of maxdb_stmt_param_count
maxdb_ping - Pings a server connection, or tries to reconnect if the connection has gone down
maxdb_prepare - Prepare an SQL statement for execution
maxdb_query - Performs a query on the database
maxdb_real_connect - Opens a connection to a MaxDB server
maxdb_real_escape_string - Escapes special characters in a string for use in an SQL statement, taking into account the current charset of the connection
maxdb_real_query - Execute an SQL query
maxdb_report - Enables or disables internal report functions
maxdb_rollback - Rolls back current transaction
maxdb_rpl_parse_enabled - Check if RPL parse is enabled
maxdb_rpl_probe - RPL probe
maxdb_rpl_query_type - Returns RPL query type
maxdb_select_db - Selects the default database for database queries
maxdb_send_long_data - Alias of maxdb_stmt_send_long_data
maxdb_send_query - Send the query and return
maxdb_server_end - Shut down the embedded server
maxdb_server_init - Initialize embedded server
maxdb_set_opt - Alias of maxdb_options
maxdb_sqlstate - Returns the SQLSTATE error from previous MaxDB operation
maxdb_ssl_set - Used for establishing secure connections using SSL
maxdb_stat - Gets the current system status
maxdb_stmt_affected_rows - Returns the total number of rows changed, deleted, or
inserted by the last executed statement
maxdb_stmt_bind_param - Binds variables to a prepared statement as parameters
maxdb_stmt_bind_result - Binds variables to a prepared statement for result storage
maxdb_stmt_close_long_data - Ends a sequence of maxdb_stmt_send_long_data
maxdb_stmt_close - Closes a prepared statement
maxdb_stmt_data_seek - Seeks to an arbitray row in statement result set
maxdb_stmt_errno - Returns the error code for the most recent statement call
maxdb_stmt_error - Returns a string description for last statement error
maxdb_stmt_execute - Executes a prepared Query
maxdb_stmt_fetch - Fetch results from a prepared statement into the bound variables
maxdb_stmt_free_result - Frees stored result memory for the given statement handle
maxdb_stmt_init - Initializes a statement and returns an resource for use with maxdb_stmt_prepare
maxdb_stmt_num_rows - Return the number of rows in statements result set
maxdb_stmt_param_count - Returns the number of parameter for the given statement
maxdb_stmt_prepare - Prepare an SQL statement for execution
maxdb_stmt_reset - Resets a prepared statement
maxdb_stmt_result_metadata - Returns result set metadata from a prepared statement
maxdb_stmt_send_long_data - Send data in blocks
maxdb_stmt_sqlstate - Returns SQLSTATE error from previous statement operation
maxdb_stmt_store_result - Transfers a result set from a prepared statement
maxdb_store_result - Transfers a result set from the last query
maxdb_thread_id - Returns the thread ID for the current connection
maxdb_thread_safe - Returns whether thread safety is given or not
maxdb_use_result - Initiate a result set retrieval
maxdb_warning_count - Returns the number of warnings from the last query for the given link
Multibyte String
mb_check_encoding - Check if the string is valid for the specified encoding
mb_convert_case - Perform case folding on a string
mb_convert_encoding - Convert character encoding
mb_convert_kana - Convert "kana" one from another ("zen-kaku", "han-kaku" and more)
mb_convert_variables - Convert character code in variable(s)
mb_decode_mimeheader - Decode string in MIME header field
mb_decode_numericentity - Decode HTML numeric string reference to character
mb_detect_encoding - Detect character encoding
mb_detect_order - Set/Get character encoding detection order
mb_encode_mimeheader - Encode string for MIME header
mb_encode_numericentity - Encode character to HTML numeric string reference
mb_encoding_aliases - Get aliases of a known encoding type
mb_ereg_match - Regular expression match for multibyte string
mb_ereg_replace - Replace regular expression with multibyte support
mb_ereg_search_getpos - Returns start point for next regular expression match
mb_ereg_search_getregs - Retrieve the result from the last multibyte regular expression match
mb_ereg_search_init - Setup string and regular expression for a multibyte regular expression match
mb_ereg_search_pos - Returns position and length of a matched part of the multibyte regular expression for a predefined multibyte string
mb_ereg_search_regs - Returns the matched part of a multibyte regular expression
mb_ereg_search_setpos - Set start point of next regular expression match
mb_ereg_search - Multibyte regular expression match for predefined multibyte string
mb_ereg - Regular expression match with multibyte support
mb_eregi_replace - Replace regular expression with multibyte support ignoring case
mb_eregi - Regular expression match ignoring case with multibyte support
mb_get_info - Get internal settings of mbstring
mb_http_input - Detect HTTP input character encoding
mb_http_output - Set/Get HTTP output character encoding
mb_internal_encoding - Set/Get internal character encoding
mb_language - Set/Get current language
mb_list_encodings - Returns an array of all supported encodings
mb_output_handler - Callback function converts character encoding in output buffer
mb_parse_str - Parse GET/POST/COOKIE data and set global variable
mb_preferred_mime_name - Get MIME charset string
mb_regex_encoding - Returns current encoding for multibyte regex as string
mb_regex_set_options - Set/Get the default options for mbregex functions
mb_send_mail - Send encoded mail
mb_split - Split multibyte string using regular expression
mb_strcut - Get part of string
mb_strimwidth - Get truncated string with specified width
mb_stripos - Finds position of first occurrence of a string within another, case insensitive
mb_stristr - Finds first occurrence of a string within another, case insensitive
mb_strlen - Get string length
mb_strpos - Find position of first occurrence of string in a string
mb_strrchr - Finds the last occurrence of a character in a string within another
mb_strrichr - Finds the last occurrence of a character in a string within another, case insensitive
mb_strripos - Finds position of last occurrence of a string within another, case insensitive
mb_strrpos - Find position of last occurrence of a string in a string
mb_strstr - Finds first occurrence of a string within another
mb_strtolower - Make a string lowercase
mb_strtoupper - Make a string uppercase
mb_strwidth - Return width of string
mb_substitute_character - Set/Get substitution character
mb_substr_count - Count the number of substring occurrences
mb_substr - Get part of string
Mcrypt
mcrypt_cbc - Encrypt/decrypt data in CBC mode
mcrypt_cfb - Encrypt/decrypt data in CFB mode
mcrypt_create_iv - Create an initialization vector (IV) from a random source
mcrypt_decrypt - Decrypts crypttext with given parameters
mcrypt_ecb - Deprecated: Encrypt/decrypt data in ECB mode
mcrypt_enc_get_algorithms_name - Returns the name of the opened algorithm
mcrypt_enc_get_block_size - Returns the blocksize of the opened algorithm
mcrypt_enc_get_iv_size - Returns the size of the IV of the opened algorithm
mcrypt_enc_get_key_size - Returns the maximum supported keysize of the opened mode
mcrypt_enc_get_modes_name - Returns the name of the opened mode
mcrypt_enc_get_supported_key_sizes - Returns an array with the supported keysizes of the opened algorithm
mcrypt_enc_is_block_algorithm_mode - Checks whether the encryption of the opened mode works on blocks
mcrypt_enc_is_block_algorithm - Checks whether the algorithm of the opened mode is a block algorithm
mcrypt_enc_is_block_mode - Checks whether the opened mode outputs blocks
mcrypt_enc_self_test - Runs a self test on the opened module
mcrypt_encrypt - Encrypts plaintext with given parameters
mcrypt_generic_deinit - This function deinitializes an encryption module
mcrypt_generic_end - This function terminates encryption
mcrypt_generic_init - This function initializes all buffers needed for encryption
mcrypt_generic - This function encrypts data
mcrypt_get_block_size - Get the block size of the specified cipher
mcrypt_get_cipher_name - Get the name of the specified cipher
mcrypt_get_iv_size - Returns the size of the IV belonging to a specific cipher/mode combination
mcrypt_get_key_size - Get the key size of the specified cipher
mcrypt_list_algorithms - Get an array of all supported ciphers
mcrypt_list_modes - Get an array of all supported modes
mcrypt_module_close - Close the mcrypt module
mcrypt_module_get_algo_block_size - Returns the blocksize of the specified algorithm
mcrypt_module_get_algo_key_size - Returns the maximum supported keysize of the opened mode
mcrypt_module_get_supported_key_sizes - Returns an array with the supported keysizes of the opened algorithm
mcrypt_module_is_block_algorithm_mode - Returns if the specified module is a block algorithm or not
mcrypt_module_is_block_algorithm - This function checks whether the specified algorithm is a block algorithm
mcrypt_module_is_block_mode - Returns if the specified mode outputs blocks or not
mcrypt_module_open - Opens the module of the algorithm and the mode to be used
mcrypt_module_self_test - This function runs a self test on the specified module
mcrypt_ofb - Encrypt/decrypt data in OFB mode
mdecrypt_generic - Decrypt data
MCVE (Monetra) Payment
m_checkstatus - Check to see if a transaction has completed
m_completeauthorizations - Number of complete authorizations in queue, returning an array of their identifiers
m_connect - Establish the connection to MCVE
m_connectionerror - Get a textual representation of why a connection failed
m_deletetrans - Delete specified transaction from MCVE_CONN structure
m_destroyconn - Destroy the connection and MCVE_CONN structure
m_destroyengine - Free memory associated with IP/SSL connectivity
m_getcell - Get a specific cell from a comma delimited response by column name
m_getcellbynum - Get a specific cell from a comma delimited response by column number
m_getcommadelimited - Get the RAW comma delimited data returned from MCVE
m_getheader - Get the name of the column in a comma-delimited response
m_initconn - Create and initialize an MCVE_CONN structure
m_initengine - Ready the client for IP/SSL Communication
m_iscommadelimited - Checks to see if response is comma delimited
m_maxconntimeout - The maximum amount of time the API will attempt a connection to MCVE
m_monitor - Perform communication with MCVE (send/receive data) Non-blocking
m_numcolumns - Number of columns returned in a comma delimited response
m_numrows - Number of rows returned in a comma delimited response
m_parsecommadelimited - Parse the comma delimited response so m_getcell, etc will work
m_responsekeys - Returns array of strings which represents the keys that can be used
for response parameters on this transaction
m_responseparam - Get a custom response parameter
m_returnstatus - Check to see if the transaction was successful
m_setblocking - Set blocking/non-blocking mode for connection
m_setdropfile - Set the connection method to Drop-File
m_setip - Set the connection method to IP
m_setssl_cafile - Set SSL CA (Certificate Authority) file for verification of server
certificate
m_setssl_files - Set certificate key files and certificates if server requires client certificate
verification
m_setssl - Set the connection method to SSL
m_settimeout - Set maximum transaction time (per trans)
m_sslcert_gen_hash - Generate hash for SSL client certificate verification
m_transactionssent - Check to see if outgoing buffer is clear
m_transinqueue - Number of transactions in client-queue
m_transkeyval - Add key/value pair to a transaction. Replaces deprecated transparam()
m_transnew - Start a new transaction
m_transsend - Finalize and send the transaction
m_uwait - Wait x microsecs
m_validateidentifier - Whether or not to validate the passed identifier on any transaction it is passed to
m_verifyconnection - Set whether or not to PING upon connect to verify connection
m_verifysslcert - Set whether or not to verify the server ssl certificate
Memcache
Memcache::add - Add an item to the server
Memcache::addServer - Add a memcached server to connection pool
Memcache::close - Close memcached server connection
Memcache::connect - Open memcached server connection
Memcache::decrement - Decrement item's value
Memcache::delete - Delete item from the server
Memcache::flush - Flush all existing items at the server
Memcache::get - Retrieve item from the server
Memcache::getExtendedStats - Get statistics from all servers in pool
Memcache::getServerStatus - Returns server status
Memcache::getStats - Get statistics of the server
Memcache::getVersion - Return version of the server
Memcache::increment - Increment item's value
Memcache::pconnect - Open memcached server persistent connection
Memcache::replace - Replace value of the existing item
Memcache::set - Store data at the server
Memcache::setCompressThreshold - Enable automatic compression of large values
Memcache::setServerParams - Changes server parameters and status at runtime
memcache_debug - Turn debug output on/off
Memcached
Memcached::add - Add an item under a new key
Memcached::addByKey - Add an item under a new key on a specific server
Memcached::addServer - Add a server to the server pool
Memcached::addServers - Add multiple servers to the server pool
Memcached::append - Append data to an existing item
Memcached::appendByKey - Append data to an existing item on a specific server
Memcached::cas - Compare and swap an item
Memcached::casByKey - Compare and swap an item on a specific server
Memcached::__construct - Create a Memcached instance
Memcached::decrement - Decrement numeric item's value
Memcached::delete - Delete an item
Memcached::deleteByKey - Delete an item from a specific server
Memcached::fetch - Fetch the next result
Memcached::fetchAll - Fetch all the remaining results
Memcached::flush - Invalidate all items in the cache
Memcached::get - Retrieve an item
Memcached::getByKey - Retrieve an item from a specific server
Memcached::getDelayed - Request multiple items
Memcached::getDelayedByKey - Request multiple items from a specific server
Memcached::getMulti - Retrieve multiple items
Memcached::getMultiByKey - Retrieve multiple items from a specific server
Memcached::getOption - Retrieve a Memcached option value
Memcached::getResultCode - Return the result code of the last operation
Memcached::getResultMessage - Return the message describing the result of the last operation
Memcached::getServerByKey - Map a key to a server
Memcached::getServerList - Get the list of the servers in the pool
Memcached::getStats - Get server pool statistics
Memcached::getVersion - Get server pool version info
Memcached::increment - Increment numeric item's value
Memcached::prepend - Prepend data to an existing item
Memcached::prependByKey - Prepend data to an existing item on a specific server
Memcached::replace - Replace the item under an existing key
Memcached::replaceByKey - Replace the item under an existing key on a specific server
Memcached::set - Store an item
Memcached::setByKey - Store an item on a specific server
Memcached::setMulti - Store multiple items
Memcached::setMultiByKey - Store multiple items on a specific server
Memcached::setOption - Set a Memcached option
Memtrack
Mhash
mhash_count - Get the highest available hash id
mhash_get_block_size - Get the block size of the specified hash
mhash_get_hash_name - Get the name of the specified hash
mhash_keygen_s2k - Generates a key
mhash - Compute hash
Mimetype
Ming (flash)
ming_keypress - Returns the action flag for keyPress(char)
ming_setcubicthreshold - Set cubic threshold
ming_setscale - Set the global scaling factor.
ming_setswfcompression - Sets the SWF output compression
ming_useconstants - Use constant pool
ming_useswfversion - Sets the SWF version
SWFAction->__construct - Creates a new SWFAction
SWFBitmap->__construct - Loads Bitmap object
SWFBitmap->getHeight - Returns the bitmap's height
SWFBitmap->getWidth - Returns the bitmap's width
SWFButton->addAction - Adds an action
SWFButton->addASound - Associates a sound with a button transition
SWFButton->addShape - Adds a shape to a button
SWFButton->__construct - Creates a new Button
SWFButton->setAction - Sets the action
SWFButton->setDown - Alias for addShape(shape, SWFBUTTON_DOWN)
SWFButton->setHit - Alias for addShape(shape, SWFBUTTON_HIT)
SWFButton->setMenu - enable track as menu button behaviour
SWFButton->setOver - Alias for addShape(shape, SWFBUTTON_OVER)
SWFButton->setUp - Alias for addShape(shape, SWFBUTTON_UP)
SWFDisplayItem->addAction - Adds this SWFAction to the given SWFSprite instance
SWFDisplayItem->addColor - Adds the given color to this item's color transform
SWFDisplayItem->endMask - Another way of defining a MASK layer
SWFDisplayItem->getRot - Description
SWFDisplayItem->getX - Description
SWFDisplayItem->getXScale - Description
SWFDisplayItem->getXSkew - Description
SWFDisplayItem->getY - Description
SWFDisplayItem->getYScale - Description
SWFDisplayItem->getYSkew - Description
SWFDisplayItem->move - Moves object in relative coordinates
SWFDisplayItem->moveTo - Moves object in global coordinates
SWFDisplayItem->multColor - Multiplies the item's color transform
SWFDisplayItem->remove - Removes the object from the movie
SWFDisplayItem->rotate - Rotates in relative coordinates
SWFDisplayItem->rotateTo - Rotates the object in global coordinates
SWFDisplayItem->scale - Scales the object in relative coordinates
SWFDisplayItem->scaleTo - Scales the object in global coordinates
SWFDisplayItem->setDepth - Sets z-order
SWFDisplayItem->setMaskLevel - Defines a MASK layer at level
SWFDisplayItem->setMatrix - Sets the item's transform matrix
SWFDisplayItem->setName - Sets the object's name
SWFDisplayItem->setRatio - Sets the object's ratio
SWFDisplayItem->skewX - Sets the X-skew
SWFDisplayItem->skewXTo - Sets the X-skew
SWFDisplayItem->skewY - Sets the Y-skew
SWFDisplayItem->skewYTo - Sets the Y-skew
SWFFill->moveTo - Moves fill origin
SWFFill->rotateTo - Sets fill's rotation
SWFFill->scaleTo - Sets fill's scale
SWFFill->skewXTo - Sets fill x-skew
SWFFill->skewYTo - Sets fill y-skew
SWFFont->__construct - Loads a font definition
SWFFont->getAscent - Returns the ascent of the font, or 0 if not available
SWFFont->getDescent - Returns the descent of the font, or 0 if not available
SWFFont->getLeading - Returns the leading of the font, or 0 if not available
SWFFont->getShape - Returns the glyph shape of a char as a text string
SWFFont->getUTF8Width - Calculates the width of the given string in this font at full height
SWFFont->getWidth - Returns the string's width
SWFFontChar->addChars - Adds characters to a font for exporting font
SWFFontChar->addUTF8Chars - Adds characters to a font for exporting font
SWFGradient->addEntry - Adds an entry to the gradient list
SWFGradient->__construct - Creates a gradient object
SWFMorph->__construct - Creates a new SWFMorph object
SWFMorph->getShape1 - Gets a handle to the starting shape
SWFMorph->getShape2 - Gets a handle to the ending shape
SWFMovie->add - Adds any type of data to a movie
SWFMovie->addExport - Description
SWFMovie->addFont - Description
SWFMovie->__construct - Creates a new movie object, representing an SWF version 4 movie
SWFMovie->importChar - Description
SWFMovie->importFont - Description
SWFMovie->labelFrame - Labels a frame
SWFMovie->nextFrame - Moves to the next frame of the animation
SWFMovie->output - Dumps your lovingly prepared movie out
SWFMovie->remove - Removes the object instance from the display list
SWFMovie->save - Saves the SWF movie in a file
SWFMovie->saveToFile - Description
SWFMovie->setbackground - Sets the background color
SWFMovie->setDimension - Sets the movie's width and height
SWFMovie->setFrames - Sets the total number of frames in the animation
SWFMovie->setRate - Sets the animation's frame rate
SWFMovie->startSound - Description
SWFMovie->stopSound - Description
SWFMovie->streamMP3 - Streams a MP3 file
SWFMovie->writeExports - Description
SWFPrebuiltClip->__construct - Returns a SWFPrebuiltClip object
SWFShape->addFill - Adds a solid fill to the shape
SWFShape->__construct - Creates a new shape object
SWFShape->drawArc - Draws an arc of radius r centered at the current location, from angle startAngle to angle endAngle measured clockwise from 12 o'clock
SWFShape->drawCircle - Draws a circle of radius r centered at the current location, in a counter-clockwise fashion
SWFShape->drawCubic - Draws a cubic bezier curve using the current position and the three given points as control points
SWFShape->drawCubicTo - Draws a cubic bezier curve using the current position and the three given points as control points
SWFShape->drawCurve - Draws a curve (relative)
SWFShape->drawCurveTo - Draws a curve
SWFShape->drawGlyph - Draws the first character in the given string into the shape using the glyph definition from the given font
SWFShape->drawLine - Draws a line (relative)
SWFShape->drawLineTo - Draws a line
SWFShape->movePen - Moves the shape's pen (relative)
SWFShape->movePenTo - Moves the shape's pen
SWFShape->setLeftFill - Sets left rasterizing color
SWFShape->setLine - Sets the shape's line style
SWFShape->setRightFill - Sets right rasterizing color
SWFSound - Returns a new SWFSound object from given file
SWFSoundInstance->loopCount - Description
SWFSoundInstance->loopInPoint - Description
SWFSoundInstance->loopOutPoint - Description
SWFSoundInstance->noMultiple - Description
SWFSprite->add - Adds an object to a sprite
SWFSprite->__construct - Creates a movie clip (a sprite)
SWFSprite->labelFrame - Labels frame
SWFSprite->nextFrame - Moves to the next frame of the animation
SWFSprite->remove - Removes an object to a sprite
SWFSprite->setFrames - Sets the total number of frames in the animation
SWFSprite->startSound - Description
SWFSprite->stopSound - Description
SWFText->addString - Draws a string
SWFText->addUTF8String - Writes the given text into this SWFText object at the current pen position,
using the current font, height, spacing, and color
SWFText->__construct - Creates a new SWFText object
SWFText->getAscent - Returns the ascent of the current font at its current size, or 0 if not available
SWFText->getDescent - Returns the descent of the current font at its current size, or 0 if not available
SWFText->getLeading - Returns the leading of the current font at its current size, or 0 if not available
SWFText->getUTF8Width - calculates the width of the given string in this text objects current font and size
SWFText->getWidth - Computes string's width
SWFText->moveTo - Moves the pen
SWFText->setColor - Sets the current text color
SWFText->setFont - Sets the current font
SWFText->setHeight - Sets the current font height
SWFText->setSpacing - Sets the current font spacing
SWFTextField->addChars - adds characters to a font that will be available within a textfield
SWFTextField->addString - Concatenates the given string to the text field
SWFTextField->align - Sets the text field alignment
SWFTextField->__construct - Creates a text field object
SWFTextField->setBounds - Sets the text field width and height
SWFTextField->setColor - Sets the color of the text field
SWFTextField->setFont - Sets the text field font
SWFTextField->setHeight - Sets the font height of this text field font
SWFTextField->setIndentation - Sets the indentation of the first line
SWFTextField->setLeftMargin - Sets the left margin width of the text field
SWFTextField->setLineSpacing - Sets the line spacing of the text field
SWFTextField->setMargins - Sets the margins width of the text field
SWFTextField->setName - Sets the variable name
SWFTextField->setPadding - Sets the padding of this textfield
SWFTextField->setRightMargin - Sets the right margin width of the text field
SWFVideoStream->__construct - Returns a SWFVideoStream object
SWFVideoStream->getNumFrames - Returns the number of frames in the video
SWFVideoStream->setDimension - Sets video dimension
Miscellaneous Functions
connection_aborted - Check whether client disconnected
connection_status - Returns connection status bitfield
connection_timeout - Check if the script timed out
constant - Returns the value of a constant
define - Defines a named constant
defined - Checks whether a given named constant exists
die - Equivalent to exit
eval - Evaluate a string as PHP code
exit - Output a message and terminate the current script
get_browser - Tells what the user's browser is capable of
__halt_compiler - Halts the compiler execution
highlight_file - Syntax highlighting of a file
highlight_string - Syntax highlighting of a string
ignore_user_abort - Set whether a client disconnect should abort script execution
pack - Pack data into binary string
php_check_syntax - Check the PHP syntax of (and execute) the specified file
php_strip_whitespace - Return source with stripped comments and whitespace
show_source - Alias of highlight_file
sleep - Delay execution
sys_getloadavg - Gets system load average
time_nanosleep - Delay for a number of seconds and nanoseconds
time_sleep_until - Make the script sleep until the specified time
uniqid - Generate a unique ID
unpack - Unpack data from binary string
usleep - Delay execution in microseconds
mnoGoSearch
udm_add_search_limit - Add various search limits
udm_alloc_agent_array - Allocate mnoGoSearch session
udm_alloc_agent - Allocate mnoGoSearch session
udm_api_version - Get mnoGoSearch API version
udm_cat_list - Get all the categories on the same level with the current one
udm_cat_path - Get the path to the current category
udm_check_charset - Check if the given charset is known to mnogosearch
udm_check_stored - Check connection to stored
udm_clear_search_limits - Clear all mnoGoSearch search restrictions
udm_close_stored - Close connection to stored
udm_crc32 - Return CRC32 checksum of given string
udm_errno - Get mnoGoSearch error number
udm_error - Get mnoGoSearch error message
udm_find - Perform search
udm_free_agent - Free mnoGoSearch session
udm_free_ispell_data - Free memory allocated for ispell data
udm_free_res - Free mnoGoSearch result
udm_get_doc_count - Get total number of documents in database
udm_get_res_field - Fetch a result field
udm_get_res_param - Get mnoGoSearch result parameters
udm_hash32 - Return Hash32 checksum of gived string
udm_load_ispell_data - Load ispell data
udm_open_stored - Open connection to stored
udm_set_agent_param - Set mnoGoSearch agent session parameters
Mongo
Mongo - The Mongo class
MongoDB - The MongoDB class
MongoCollection - The MongoCollection class
MongoCursor - The MongoCursor class
MongoId - The MongoId class
MongoCode - The MongoCode class
MongoDate - The MongoDate class
MongoRegex - The MongoRegex class
MongoBinData - The MongoBinData class
MongoDBRef - The MongoDBRef class
MongoMinKey - The MongoMinKey class
MongoMaxKey - The MongoMaxKey class
MongoTimestamp - The MongoTimestamp class
MongoGridFS - The MongoGridFS class
MongoGridFSFile - The MongoGridFSFile class
MongoGridFSCursor - The MongoGridFSCursor class
MongoException - The MongoException class
MongoCursorException - The MongoCursorException class
MongoCursorTimeoutException - The MongoCursorTimeoutException class
MongoConnectionException - The MongoConnectionException class
MongoGridFSException - The MongoGridFSException class
mqseries
mqseries_back - MQSeries MQBACK
mqseries_begin - MQseries MQBEGIN
mqseries_close - MQSeries MQCLOSE
mqseries_cmit - MQSeries MQCMIT
mqseries_conn - MQSeries MQCONN
mqseries_connx - MQSeries MQCONNX
mqseries_disc - MQSeries MQDISC
mqseries_get - MQSeries MQGET
mqseries_inq - MQSeries MQINQ
mqseries_open - MQSeries MQOPEN
mqseries_put1 - MQSeries MQPUT1
mqseries_put - MQSeries MQPUT
mqseries_set - MQSeries MQSET
mqseries_strerror - Returns the error message corresponding to a result code (MQRC).
Mohawk Software Session Handler Functions
msession_connect - Connect to msession server
msession_count - Get session count
msession_create - Create a session
msession_destroy - Destroy a session
msession_disconnect - Close connection to msession server
msession_find - Find all sessions with name and value
msession_get_array - Get array of msession variables
msession_get_data - Get data session unstructured data
msession_get - Get value from session
msession_inc - Increment value in session
msession_list - List all sessions
msession_listvar - List sessions with variable
msession_lock - Lock a session
msession_plugin - Call an escape function within the msession personality plugin
msession_randstr - Get random string
msession_set_array - Set msession variables from an array
msession_set_data - Set data session unstructured data
msession_set - Set value in session
msession_timeout - Set/get session timeout
msession_uniq - Get unique id
msession_unlock - Unlock a session
mSQL
msql_affected_rows - Returns number of affected rows
msql_close - Close mSQL connection
msql_connect - Open mSQL connection
msql_create_db - Create mSQL database
msql_createdb - Alias of msql_create_db
msql_data_seek - Move internal row pointer
msql_db_query - Send mSQL query
msql_dbname - Alias of msql_result
msql_drop_db - Drop (delete) mSQL database
msql_error - Returns error message of last msql call
msql_fetch_array - Fetch row as array
msql_fetch_field - Get field information
msql_fetch_object - Fetch row as object
msql_fetch_row - Get row as enumerated array
msql_field_flags - Get field flags
msql_field_len - Get field length
msql_field_name - Get the name of the specified field in a result
msql_field_seek - Set field offset
msql_field_table - Get table name for field
msql_field_type - Get field type
msql_fieldflags - Alias of msql_field_flags
msql_fieldlen - Alias of msql_field_len
msql_fieldname - Alias of msql_field_name
msql_fieldtable - Alias of msql_field_table
msql_fieldtype - Alias of msql_field_type
msql_free_result - Free result memory
msql_list_dbs - List mSQL databases on server
msql_list_fields - List result fields
msql_list_tables - List tables in an mSQL database
msql_num_fields - Get number of fields in result
msql_num_rows - Get number of rows in result
msql_numfields - Alias of msql_num_fields
msql_numrows - Alias of msql_num_rows
msql_pconnect - Open persistent mSQL connection
msql_query - Send mSQL query
msql_regcase - Alias of sql_regcase
msql_result - Get result data
msql_select_db - Select mSQL database
msql_tablename - Alias of msql_result
msql - Alias of msql_db_query
Microsoft SQL Server
mssql_bind - Adds a parameter to a stored procedure or a remote stored procedure
mssql_close - Close MS SQL Server connection
mssql_connect - Open MS SQL server connection
mssql_data_seek - Moves internal row pointer
mssql_execute - Executes a stored procedure on a MS SQL server database
mssql_fetch_array - Fetch a result row as an associative array, a numeric array, or both
mssql_fetch_assoc - Returns an associative array of the current row in the result
mssql_fetch_batch - Returns the next batch of records
mssql_fetch_field - Get field information
mssql_fetch_object - Fetch row as object
mssql_fetch_row - Get row as enumerated array
mssql_field_length - Get the length of a field
mssql_field_name - Get the name of a field
mssql_field_seek - Seeks to the specified field offset
mssql_field_type - Gets the type of a field
mssql_free_result - Free result memory
mssql_free_statement - Free statement memory
mssql_get_last_message - Returns the last message from the server
mssql_guid_string - Converts a 16 byte binary GUID to a string
mssql_init - Initializes a stored procedure or a remote stored procedure
mssql_min_error_severity - Sets the minimum error severity
mssql_min_message_severity - Sets the minimum message severity
mssql_next_result - Move the internal result pointer to the next result
mssql_num_fields - Gets the number of fields in result
mssql_num_rows - Gets the number of rows in result
mssql_pconnect - Open persistent MS SQL connection
mssql_query - Send MS SQL query
mssql_result - Get result data
mssql_rows_affected - Returns the number of records affected by the query
mssql_select_db - Select MS SQL database
MySQL
mysql_affected_rows - Get number of affected rows in previous MySQL operation
mysql_client_encoding - Returns the name of the character set
mysql_close - Close MySQL connection
mysql_connect - Open a connection to a MySQL Server
mysql_create_db - Create a MySQL database
mysql_data_seek - Move internal result pointer
mysql_db_name - Get result data
mysql_db_query - Send a MySQL query
mysql_drop_db - Drop (delete) a MySQL database
mysql_errno - Returns the numerical value of the error message from previous MySQL operation
mysql_error - Returns the text of the error message from previous MySQL operation
mysql_escape_string - Escapes a string for use in a mysql_query
mysql_fetch_array - Fetch a result row as an associative array, a numeric array, or both
mysql_fetch_assoc - Fetch a result row as an associative array
mysql_fetch_field - Get column information from a result and return as an object
mysql_fetch_lengths - Get the length of each output in a result
mysql_fetch_object - Fetch a result row as an object
mysql_fetch_row - Get a result row as an enumerated array
mysql_field_flags - Get the flags associated with the specified field in a result
mysql_field_len - Returns the length of the specified field
mysql_field_name - Get the name of the specified field in a result
mysql_field_seek - Set result pointer to a specified field offset
mysql_field_table - Get name of the table the specified field is in
mysql_field_type - Get the type of the specified field in a result
mysql_free_result - Free result memory
mysql_get_client_info - Get MySQL client info
mysql_get_host_info - Get MySQL host info
mysql_get_proto_info - Get MySQL protocol info
mysql_get_server_info - Get MySQL server info
mysql_info - Get information about the most recent query
mysql_insert_id - Get the ID generated in the last query
mysql_list_dbs - List databases available on a MySQL server
mysql_list_fields - List MySQL table fields
mysql_list_processes - List MySQL processes
mysql_list_tables - List tables in a MySQL database
mysql_num_fields - Get number of fields in result
mysql_num_rows - Get number of rows in result
mysql_pconnect - Open a persistent connection to a MySQL server
mysql_ping - Ping a server connection or reconnect if there is no connection
mysql_query - Send a MySQL query
mysql_real_escape_string - Escapes special characters in a string for use in an SQL statement
mysql_result - Get result data
mysql_select_db - Select a MySQL database
mysql_set_charset - Sets the client character set
mysql_stat - Get current system status
mysql_tablename - Get table name of field
mysql_thread_id - Return the current thread ID
mysql_unbuffered_query - Send an SQL query to MySQL without fetching and buffering the result rows.
MySQL Improved Extension
mysqli->affected_rows - Gets the number of affected rows in a previous MySQL operation
mysqli::autocommit - Turns on or off auto-commiting database modifications
mysqli::change_user - Changes the user of the specified database connection
mysqli::character_set_name - Returns the default character set for the database connection
mysqli->client_info - Returns the MySQL client version as a string
mysqli->client_version - Get MySQL client info
mysqli::close - Closes a previously opened database connection
mysqli::commit - Commits the current transaction
mysqli->connect_errno - Returns the error code from last connect call
mysqli->connect_error - Returns a string description of the last connect error
mysqli::__construct - Open a new connection to the MySQL server
mysqli::debug - Performs debugging operations
mysqli::dump_debug_info - Dump debugging information into the log
mysqli->errno - Returns the error code for the most recent function call
mysqli->error - Returns a string description of the last error
mysqli->field_count - Returns the number of columns for the most recent query
mysqli::get_charset - Returns a character set object
mysqli->get_client_info - Returns the MySQL client version as a string
mysqli->client_version - Get MySQL client info
mysqli::get_connection_stats - Returns statistics about the client connection
mysqli->host_info - Returns a string representing the type of connection used
mysqli->protocol_version - Returns the version of the MySQL protocol used
mysqli->server_info - Returns the version of the MySQL server
mysqli->server_version - Returns the version of the MySQL server as an integer
mysqli::get_warnings - Get result of SHOW WARNINGS
mysqli->info - Retrieves information about the most recently executed query
mysqli::init - Initializes MySQLi and returns a resource for use with mysqli_real_connect()
mysqli->insert_id - Returns the auto generated id used in the last query
mysqli::kill - Asks the server to kill a MySQL thread
mysqli::more_results - Check if there are any more query results from a multi query
mysqli::multi_query - Performs a query on the database
mysqli::next_result - Prepare next result from multi_query
mysqli::options - Set options
mysqli::ping - Pings a server connection, or tries to reconnect if the connection has gone down
mysqli::poll - Poll connections
mysqli::prepare - Prepare an SQL statement for execution
mysqli::query - Performs a query on the database
mysqli::real_connect - Opens a connection to a mysql server
mysqli::real_escape_string - Escapes special characters in a string for use in an SQL statement, taking into account the current charset of the connection
mysqli::real_query - Execute an SQL query
mysqli::reap_async_query - Get result from async query
mysqli::rollback - Rolls back current transaction
mysqli::select_db - Selects the default database for database queries
mysqli::set_charset - Sets the default client character set
mysqli::set_local_infile_default - Unsets user defined handler for load local infile command
mysqli::set_local_infile_handler - Set callback function for LOAD DATA LOCAL INFILE command
mysqli->sqlstate - Returns the SQLSTATE error from previous MySQL operation
mysqli::ssl_set - Used for establishing secure connections using SSL
mysqli::stat - Gets the current system status
mysqli::stmt_init - Initializes a statement and returns an object for use with mysqli_stmt_prepare
mysqli::store_result - Transfers a result set from the last query
mysqli->thread_id - Returns the thread ID for the current connection
mysqli::thread_safe - Returns whether thread safety is given or not
mysqli::use_result - Initiate a result set retrieval
mysqli->warning_count - Returns the number of warnings from the last query for the given link
mysqli_stmt->affected_rows - Returns the total number of rows changed, deleted, or
inserted by the last executed statement
mysqli_stmt::attr_get - Used to get the current value of a statement attribute
mysqli_stmt::attr_set - Used to modify the behavior of a prepared statement
mysqli_stmt::bind_param - Binds variables to a prepared statement as parameters
mysqli_stmt::bind_result - Binds variables to a prepared statement for result storage
mysqli_stmt::close - Closes a prepared statement
mysqli_stmt::data_seek - Seeks to an arbitrary row in statement result set
mysqli_stmt->errno - Returns the error code for the most recent statement call
mysqli_stmt->error - Returns a string description for last statement error
mysqli_stmt::execute - Executes a prepared Query
mysqli_stmt::fetch - Fetch results from a prepared statement into the bound variables
mysqli_stmt->field_count - Returns the number of field in the given statement
mysqli_stmt::free_result - Frees stored result memory for the given statement handle
mysqli_stmt::get_warnings - Get result of SHOW WARNINGS
mysqli_stmt->insert_id - Get the ID generated from the previous INSERT operation
mysqli_stmt::num_rows - Return the number of rows in statements result set
mysqli_stmt->param_count - Returns the number of parameter for the given statement
mysqli_stmt::prepare - Prepare an SQL statement for execution
mysqli_stmt::reset - Resets a prepared statement
mysqli_stmt::result_metadata - Returns result set metadata from a prepared statement
mysqli_stmt::send_long_data - Send data in blocks
mysqli_stmt::sqlstate - Returns SQLSTATE error from previous statement operation
mysqli_stmt::store_result - Transfers a result set from a prepared statement
mysqli_result->current_field - Get current field offset of a result pointer
mysqli_result::data_seek - Adjusts the result pointer to an arbitary row in the result
mysqli_result::fetch_all - Fetches all result rows as an associative array, a numeric array, or both
mysqli_result::fetch_array - Fetch a result row as an associative, a numeric array, or both
mysqli_result::fetch_assoc - Fetch a result row as an associative array
mysqli_result::fetch_field_direct - Fetch meta-data for a single field
mysqli_result::fetch_field - Returns the next field in the result set
mysqli_result::fetch_fields - Returns an array of objects representing the fields in a result set
mysqli_result::fetch_object - Returns the current row of a result set as an object
mysqli_result::fetch_row - Get a result row as an enumerated array
mysqli_result->field_count - Get the number of fields in a result
mysqli_result::field_seek - Set result pointer to a specified field offset
mysqli_result::free - Frees the memory associated with a result
mysqli_result->lengths - Returns the lengths of the columns of the current row in the result set
mysqli_result->num_rows - Gets the number of rows in a result
mysqli_driver::embedded_server_end - Stop embedded server
mysqli_driver::embedded_server_start - Initialize and start embedded server
mysqli_warning::__construct - The __construct purpose
mysqli_warning::next - The next purpose
mysqli_bind_param - Alias for mysqli_stmt_bind_param
mysqli_bind_result - Alias for mysqli_stmt_bind_result
mysqli_client_encoding - Alias of mysqli_character_set_name
mysqli_connect - Alias of mysqli::__construct
mysqli_disable_reads_from_master - Disable reads from master
mysqli_disable_rpl_parse - Disable RPL parse
mysqli_enable_reads_from_master - Enable reads from master
mysqli_enable_rpl_parse - Enable RPL parse
mysqli_escape_string - Alias of mysqli_real_escape_string
mysqli_execute - Alias for mysqli_stmt_execute
mysqli_fetch - Alias for mysqli_stmt_fetch
mysqli_get_cache_stats - Returns client Zval cache statistics
mysqli_get_client_stats - Returns client per-process statistics
mysqli_get_metadata - Alias for mysqli_stmt_result_metadata
mysqli_master_query - Enforce execution of a query on the master in a master/slave setup
mysqli_param_count - Alias for mysqli_stmt_param_count
mysqli_report - Enables or disables internal report functions
mysqli_rpl_parse_enabled - Check if RPL parse is enabled
mysqli_rpl_probe - RPL probe
mysqli_rpl_query_type - Returns RPL query type
mysqli_send_long_data - Alias for mysqli_stmt_send_long_data
mysqli_send_query - Send the query and return
mysqli_set_opt - Alias of mysqli_options
mysqli_slave_query - Force execution of a query on a slave in a master/slave setup
Mysqlnd query result cache plugin
mysqlnd_qc_change_handler - Change current storage handler
mysqlnd_qc_clear_cache - Flush all cache contents
mysqlnd_qc_get_cache_info - Returns information on the current handler, the number of cache entries and cache entries, if available
mysqlnd_qc_get_core_stats - Statistics collected by the core of the query cache
mysqlnd_qc_get_handler - Returns a list of available storage handler
mysqlnd_qc_get_query_trace_log - Returns a backtrace for each query inspected by the query cache
mysqlnd_qc_set_user_handlers - Sets the callback functions for a user-defined procedural storage handler
MySQL Native Driver
Ncurses Terminal Screen Control
ncurses_addch - Add character at current position and advance cursor
ncurses_addchnstr - Add attributed string with specified length at current position
ncurses_addchstr - Add attributed string at current position
ncurses_addnstr - Add string with specified length at current position
ncurses_addstr - Output text at current position
ncurses_assume_default_colors - Define default colors for color 0
ncurses_attroff - Turn off the given attributes
ncurses_attron - Turn on the given attributes
ncurses_attrset - Set given attributes
ncurses_baudrate - Returns baudrate of terminal
ncurses_beep - Let the terminal beep
ncurses_bkgd - Set background property for terminal screen
ncurses_bkgdset - Control screen background
ncurses_border - Draw a border around the screen using attributed characters
ncurses_bottom_panel - Moves a visible panel to the bottom of the stack
ncurses_can_change_color - Checks if terminal color definitions can be changed
ncurses_cbreak - Switch of input buffering
ncurses_clear - Clear screen
ncurses_clrtobot - Clear screen from current position to bottom
ncurses_clrtoeol - Clear screen from current position to end of line
ncurses_color_content - Retrieves RGB components of a color
ncurses_color_set - Set active foreground and background colors
ncurses_curs_set - Set cursor state
ncurses_def_prog_mode - Saves terminals (program) mode
ncurses_def_shell_mode - Saves terminals (shell) mode
ncurses_define_key - Define a keycode
ncurses_del_panel - Remove panel from the stack and delete it (but not the associated window)
ncurses_delay_output - Delay output on terminal using padding characters
ncurses_delch - Delete character at current position, move rest of line left
ncurses_deleteln - Delete line at current position, move rest of screen up
ncurses_delwin - Delete a ncurses window
ncurses_doupdate - Write all prepared refreshes to terminal
ncurses_echo - Activate keyboard input echo
ncurses_echochar - Single character output including refresh
ncurses_end - Stop using ncurses, clean up the screen
ncurses_erase - Erase terminal screen
ncurses_erasechar - Returns current erase character
ncurses_filter - Set LINES for iniscr() and newterm() to 1
ncurses_flash - Flash terminal screen (visual bell)
ncurses_flushinp - Flush keyboard input buffer
ncurses_getch - Read a character from keyboard
ncurses_getmaxyx - Returns the size of a window
ncurses_getmouse - Reads mouse event
ncurses_getyx - Returns the current cursor position for a window
ncurses_halfdelay - Put terminal into halfdelay mode
ncurses_has_colors - Checks if terminal has color capabilities
ncurses_has_ic - Check for insert- and delete-capabilities
ncurses_has_il - Check for line insert- and delete-capabilities
ncurses_has_key - Check for presence of a function key on terminal keyboard
ncurses_hide_panel - Remove panel from the stack, making it invisible
ncurses_hline - Draw a horizontal line at current position using an attributed character and max. n characters long
ncurses_inch - Get character and attribute at current position
ncurses_init_color - Define a terminal color
ncurses_init_pair - Define a color pair
ncurses_init - Initialize ncurses
ncurses_insch - Insert character moving rest of line including character at current position
ncurses_insdelln - Insert lines before current line scrolling down (negative numbers delete and scroll up)
ncurses_insertln - Insert a line, move rest of screen down
ncurses_insstr - Insert string at current position, moving rest of line right
ncurses_instr - Reads string from terminal screen
ncurses_isendwin - Ncurses is in endwin mode, normal screen output may be performed
ncurses_keyok - Enable or disable a keycode
ncurses_keypad - Turns keypad on or off
ncurses_killchar - Returns current line kill character
ncurses_longname - Returns terminals description
ncurses_meta - Enables/Disable 8-bit meta key information
ncurses_mouse_trafo - Transforms coordinates
ncurses_mouseinterval - Set timeout for mouse button clicks
ncurses_mousemask - Sets mouse options
ncurses_move_panel - Moves a panel so that its upper-left corner is at [startx, starty]
ncurses_move - Move output position
ncurses_mvaddch - Move current position and add character
ncurses_mvaddchnstr - Move position and add attributed string with specified length
ncurses_mvaddchstr - Move position and add attributed string
ncurses_mvaddnstr - Move position and add string with specified length
ncurses_mvaddstr - Move position and add string
ncurses_mvcur - Move cursor immediately
ncurses_mvdelch - Move position and delete character, shift rest of line left
ncurses_mvgetch - Move position and get character at new position
ncurses_mvhline - Set new position and draw a horizontal line using an attributed character and max. n characters long
ncurses_mvinch - Move position and get attributed character at new position
ncurses_mvvline - Set new position and draw a vertical line using an attributed character and max. n characters long
ncurses_mvwaddstr - Add string at new position in window
ncurses_napms - Sleep
ncurses_new_panel - Create a new panel and associate it with window
ncurses_newpad - Creates a new pad (window)
ncurses_newwin - Create a new window
ncurses_nl - Translate newline and carriage return / line feed
ncurses_nocbreak - Switch terminal to cooked mode
ncurses_noecho - Switch off keyboard input echo
ncurses_nonl - Do not translate newline and carriage return / line feed
ncurses_noqiflush - Do not flush on signal characters
ncurses_noraw - Switch terminal out of raw mode
ncurses_pair_content - Retrieves foreground and background colors of a color pair
ncurses_panel_above - Returns the panel above panel
ncurses_panel_below - Returns the panel below panel
ncurses_panel_window - Returns the window associated with panel
ncurses_pnoutrefresh - Copies a region from a pad into the virtual screen
ncurses_prefresh - Copies a region from a pad into the virtual screen
ncurses_putp - Apply padding information to the string and output it
ncurses_qiflush - Flush on signal characters
ncurses_raw - Switch terminal into raw mode
ncurses_refresh - Refresh screen
ncurses_replace_panel - Replaces the window associated with panel
ncurses_reset_prog_mode - Resets the prog mode saved by def_prog_mode
ncurses_reset_shell_mode - Resets the shell mode saved by def_shell_mode
ncurses_resetty - Restores saved terminal state
ncurses_savetty - Saves terminal state
ncurses_scr_dump - Dump screen content to file
ncurses_scr_init - Initialize screen from file dump
ncurses_scr_restore - Restore screen from file dump
ncurses_scr_set - Inherit screen from file dump
ncurses_scrl - Scroll window content up or down without changing current position
ncurses_show_panel - Places an invisible panel on top of the stack, making it visible
ncurses_slk_attr - Returns current soft label key attribute
ncurses_slk_attroff - Turn off the given attributes for soft function-key labels
ncurses_slk_attron - Turn on the given attributes for soft function-key labels
ncurses_slk_attrset - Set given attributes for soft function-key labels
ncurses_slk_clear - Clears soft labels from screen
ncurses_slk_color - Sets color for soft label keys
ncurses_slk_init - Initializes soft label key functions
ncurses_slk_noutrefresh - Copies soft label keys to virtual screen
ncurses_slk_refresh - Copies soft label keys to screen
ncurses_slk_restore - Restores soft label keys
ncurses_slk_set - Sets function key labels
ncurses_slk_touch - Forces output when ncurses_slk_noutrefresh is performed
ncurses_standend - Stop using 'standout' attribute
ncurses_standout - Start using 'standout' attribute
ncurses_start_color - Initializes color functionality
ncurses_termattrs - Returns a logical OR of all attribute flags supported by terminal
ncurses_termname - Returns terminals (short)-name
ncurses_timeout - Set timeout for special key sequences
ncurses_top_panel - Moves a visible panel to the top of the stack
ncurses_typeahead - Specify different filedescriptor for typeahead checking
ncurses_ungetch - Put a character back into the input stream
ncurses_ungetmouse - Pushes mouse event to queue
ncurses_update_panels - Refreshes the virtual screen to reflect the relations between panels in the stack
ncurses_use_default_colors - Assign terminal default colors to color id -1
ncurses_use_env - Control use of environment information about terminal size
ncurses_use_extended_names - Control use of extended names in terminfo descriptions
ncurses_vidattr - Display the string on the terminal in the video attribute mode
ncurses_vline - Draw a vertical line at current position using an attributed character and max. n characters long
ncurses_waddch - Adds character at current position in a window and advance cursor
ncurses_waddstr - Outputs text at current postion in window
ncurses_wattroff - Turns off attributes for a window
ncurses_wattron - Turns on attributes for a window
ncurses_wattrset - Set the attributes for a window
ncurses_wborder - Draws a border around the window using attributed characters
ncurses_wclear - Clears window
ncurses_wcolor_set - Sets windows color pairings
ncurses_werase - Erase window contents
ncurses_wgetch - Reads a character from keyboard (window)
ncurses_whline - Draws a horizontal line in a window at current position using an attributed character and max. n characters long
ncurses_wmouse_trafo - Transforms window/stdscr coordinates
ncurses_wmove - Moves windows output position
ncurses_wnoutrefresh - Copies window to virtual screen
ncurses_wrefresh - Refresh window on terminal screen
ncurses_wstandend - End standout mode for a window
ncurses_wstandout - Enter standout mode for a window
ncurses_wvline - Draws a vertical line in a window at current position using an attributed character and max. n characters long
Net Gopher
gopher_parsedir - Translate a gopher formatted directory entry into an associative array.
Network
checkdnsrr - Check DNS records corresponding to a given Internet host name or IP address
closelog - Close connection to system logger
define_syslog_variables - Initializes all syslog related variables
dns_check_record - Alias of checkdnsrr
dns_get_mx - Alias of getmxrr
dns_get_record - Fetch DNS Resource Records associated with a hostname
fsockopen - Open Internet or Unix domain socket connection
gethostbyaddr - Get the Internet host name corresponding to a given IP address
gethostbyname - Get the IPv4 address corresponding to a given Internet host name
gethostbynamel - Get a list of IPv4 addresses corresponding to a given Internet host
name
gethostname - Gets the host name
getmxrr - Get MX records corresponding to a given Internet host name
getprotobyname - Get protocol number associated with protocol name
getprotobynumber - Get protocol name associated with protocol number
getservbyname - Get port number associated with an Internet service and protocol
getservbyport - Get Internet service which corresponds to port and protocol
header_remove - Remove previously set headers
header - Send a raw HTTP header
headers_list - Returns a list of response headers sent (or ready to send)
headers_sent - Checks if or where headers have been sent
inet_ntop - Converts a packed internet address to a human readable representation
inet_pton - Converts a human readable IP address to its packed in_addr representation
ip2long - Converts a string containing an (IPv4) Internet Protocol dotted address into a proper address
long2ip - Converts an (IPv4) Internet network address into a string in Internet standard dotted format
openlog - Open connection to system logger
pfsockopen - Open persistent Internet or Unix domain socket connection
setcookie - Send a cookie
setrawcookie - Send a cookie without urlencoding the cookie value
socket_get_status - Alias of stream_get_meta_data
socket_set_blocking - Alias of stream_set_blocking
socket_set_timeout - Alias of stream_set_timeout
syslog - Generate a system log message
Newt
newt_bell - Send a beep to the terminal
newt_button_bar - This function returns a grid containing the buttons created.
newt_button - Create a new button
newt_centered_window - Open a centered window of the specified size
newt_checkbox_get_value - Retreives value of checkox resource
newt_checkbox_set_flags - Configures checkbox resource
newt_checkbox_set_value - Sets the value of the checkbox
newt_checkbox_tree_add_item - Adds new item to the checkbox tree
newt_checkbox_tree_find_item - Finds an item in the checkbox tree
newt_checkbox_tree_get_current - Returns checkbox tree selected item
newt_checkbox_tree_get_entry_value - Description
newt_checkbox_tree_get_multi_selection - Description
newt_checkbox_tree_get_selection - Description
newt_checkbox_tree_multi - Description
newt_checkbox_tree_set_current - Description
newt_checkbox_tree_set_entry_value - Description
newt_checkbox_tree_set_entry - Description
newt_checkbox_tree_set_width - Description
newt_checkbox_tree - Description
newt_checkbox - Description
newt_clear_key_buffer - Discards the contents of the terminal's input buffer without
waiting for additional input
newt_cls - Description
newt_compact_button - Description
newt_component_add_callback - Description
newt_component_takes_focus - Description
newt_create_grid - Description
newt_cursor_off - Description
newt_cursor_on - Description
newt_delay - Description
newt_draw_form - Description
newt_draw_root_text - Displays the string text at the position indicated
newt_entry_get_value - Description
newt_entry_set_filter - Description
newt_entry_set_flags - Description
newt_entry_set - Description
newt_entry - Description
newt_finished - Uninitializes newt interface
newt_form_add_component - Adds a single component to the form
newt_form_add_components - Add several components to the form
newt_form_add_hot_key - Description
newt_form_destroy - Destroys a form
newt_form_get_current - Description
newt_form_run - Runs a form
newt_form_set_background - Description
newt_form_set_height - Description
newt_form_set_size - Description
newt_form_set_timer - Description
newt_form_set_width - Description
newt_form_watch_fd - Description
newt_form - Create a form
newt_get_screen_size - Fills in the passed references with the current size of the
terminal
newt_grid_add_components_to_form - Description
newt_grid_basic_window - Description
newt_grid_free - Description
newt_grid_get_size - Description
newt_grid_h_close_stacked - Description
newt_grid_h_stacked - Description
newt_grid_place - Description
newt_grid_set_field - Description
newt_grid_simple_window - Description
newt_grid_v_close_stacked - Description
newt_grid_v_stacked - Description
newt_grid_wrapped_window_at - Description
newt_grid_wrapped_window - Description
newt_init - Initialize newt
newt_label_set_text - Description
newt_label - Description
newt_listbox_append_entry - Description
newt_listbox_clear_selection - Description
newt_listbox_clear - Description
newt_listbox_delete_entry - Description
newt_listbox_get_current - Description
newt_listbox_get_selection - Description
newt_listbox_insert_entry - Description
newt_listbox_item_count - Description
newt_listbox_select_item - Description
newt_listbox_set_current_by_key - Description
newt_listbox_set_current - Description
newt_listbox_set_data - Description
newt_listbox_set_entry - Description
newt_listbox_set_width - Description
newt_listbox - Description
newt_listitem_get_data - Description
newt_listitem_set - Description
newt_listitem - Description
newt_open_window - Open a window of the specified size and position
newt_pop_help_line - Replaces the current help line with the one from the stack
newt_pop_window - Removes the top window from the display
newt_push_help_line - Saves the current help line on a stack, and displays the new line
newt_radio_get_current - Description
newt_radiobutton - Description
newt_redraw_help_line - Description
newt_reflow_text - Description
newt_refresh - Updates modified portions of the screen
newt_resize_screen - Description
newt_resume - Resume using the newt interface after calling
newt_suspend
newt_run_form - Runs a form
newt_scale_set - Description
newt_scale - Description
newt_scrollbar_set - Description
newt_set_help_callback - Description
newt_set_suspend_callback - Set a callback function which gets invoked when user
presses the suspend key
newt_suspend - Tells newt to return the terminal to its initial state
newt_textbox_get_num_lines - Description
newt_textbox_reflowed - Description
newt_textbox_set_height - Description
newt_textbox_set_text - Description
newt_textbox - Description
newt_vertical_scrollbar - Description
newt_wait_for_key - Doesn't return until a key has been pressed
newt_win_choice - Description
newt_win_entries - Description
newt_win_menu - Description
newt_win_message - Description
newt_win_messagev - Description
newt_win_ternary - Description
YP/NIS
yp_all - Traverse the map and call a function on each entry
yp_cat - Return an array containing the entire map
yp_err_string - Returns the error string associated with the given error code
yp_errno - Returns the error code of the previous operation
yp_first - Returns the first key-value pair from the named map
yp_get_default_domain - Fetches the machine's default NIS domain
yp_master - Returns the machine name of the master NIS server for a map
yp_match - Returns the matched line
yp_next - Returns the next key-value pair in the named map
yp_order - Returns the order number for a map
Lotus Notes
notes_body - Open the message msg_number in the specified mailbox on the specified server (leave serv
notes_copy_db - Copy a Lotus Notes database
notes_create_db - Create a Lotus Notes database
notes_create_note - Create a note using form form_name
notes_drop_db - Drop a Lotus Notes database
notes_find_note - Returns a note id found in database_name
notes_header_info - Open the message msg_number in the specified mailbox on the specified server (leave serv
notes_list_msgs - Returns the notes from a selected database_name
notes_mark_read - Mark a note_id as read for the User user_name
notes_mark_unread - Mark a note_id as unread for the User user_name
notes_nav_create - Create a navigator name, in database_name
notes_search - Find notes that match keywords in database_name
notes_unread - Returns the unread note id's for the current User user_name
notes_version - Get the version Lotus Notes
NSAPI
nsapi_request_headers - Fetch all HTTP request headers
nsapi_response_headers - Fetch all HTTP response headers
nsapi_virtual - Perform an NSAPI sub-request
OAuth
oauth_get_sbs - Generate a Signature Base String
oauth_urlencode - Encode a URI to RFC 3986
OAuth::__construct - Create a new OAuth object
OAuth::__destruct - The destructor
OAuth::disableDebug - Turn off verbose debugging
OAuth::disableRedirects - Turn off redirects
OAuth::disableSSLChecks - Turn off SSL checks
OAuth::enableDebug - Turn on verbose debugging
OAuth::enableRedirects - Turn on redirects
OAuth::enableSSLChecks - Turn on SSL checks
OAuth::fetch - Fetch an OAuth protected resource
OAuth::getAccessToken - Fetch an access token
OAuth::getCAPath - Gets CA information
OAuth::getLastResponse - Get the last response
OAuth::getLastResponseInfo - Get HTTP information about the last response
OAuth::getRequestToken - Fetch a request token
OAuth::setAuthType - Set authorization type
OAuth::setCAPath - Set CA path and info
OAuth::setNonce - Set the nonce for subsequent requests
OAuth::setRequestEngine - The setRequestEngine purpose
OAuth::setRSACertificate - Set the RSA certificate
OAuth::setTimestamp - Set the timestamp
OAuth::setToken - Sets the token and secret
OAuth::setVersion - Set the OAuth version
OAuthProvider::addRequiredParameter - Add required parameters
OAuthProvider::callconsumerHandler - Calls the consumerNonceHandler callback
OAuthProvider::callTimestampNonceHandler - Calls the timestampNonceHandler callback
OAuthProvider::calltokenHandler - Calls the tokenNonceHandler callback
OAuthProvider::checkOAuthRequest - Check an oauth request
OAuthProvider::__construct - Constructs a new OAuthProvider object
OAuthProvider::consumerHandler - Set the consumerHandler handler callback
OAuthProvider::generateToken - Generate a random token
OAuthProvider::is2LeggedEndpoint - is2LeggedEndpoint
OAuthProvider::isRequestTokenEndpoint - Sets isRequestTokenEndpoint
OAuthProvider::removeRequiredParameter - Remove a required parameter
OAuthProvider::reportProblem - Report a problem
OAuthProvider::setParam - Set a parameter
OAuthProvider::setRequestTokenPath - Set request token path
OAuthProvider::timestampNonceHandler - Set the timestampNonceHandler handler callback
OAuthProvider::tokenHandler - Set the tokenHandler handler callback
Object Aggregation/Composition [PHP 4]
aggregate_info - Gets aggregation information for a given object
aggregate_methods_by_list - Selective dynamic class methods aggregation to an object
aggregate_methods_by_regexp - Selective class methods aggregation to an object using a regular
expression
aggregate_methods - Dynamic class and object aggregation of methods
aggregate_properties_by_list - Selective dynamic class properties aggregation to an object
aggregate_properties_by_regexp - Selective class properties aggregation to an object using a regular
expression
aggregate_properties - Dynamic aggregation of class properties to an object
aggregate - Dynamic class and object aggregation of methods and properties
aggregation_info - Alias of aggregate_info
deaggregate - Removes the aggregated methods and properties from an object
Oracle OCI8
oci_bind_array_by_name - Binds PHP array to Oracle PL/SQL array by name
oci_bind_by_name - Binds a PHP variable to an Oracle placeholder
oci_cancel - Cancels reading from cursor
oci_close - Closes an Oracle connection
OCI-Collection->append - Appends element to the collection
OCI-Collection->assign - Assigns a value to the collection from another existing collection
OCI-Collection->assignElem - Assigns a value to the element of the collection
OCI-Collection->free - Frees the resources associated with the collection object
OCI-Collection->getElem - Returns value of the element
OCI-Collection->max - Returns the maximum number of elements in the collection
OCI-Collection->size - Returns size of the collection
OCI-Collection->trim - Trims elements from the end of the collection
oci_commit - Commits the outstanding database transaction
oci_connect - Connect to an Oracle database
oci_define_by_name - Associates a PHP variable with a column for query fetches
oci_error - Returns the last error found
oci_execute - Executes a statement
oci_fetch_all - Fetches multiple rows from a query into a two-dimensional array
oci_fetch_array - Returns the next row from a query as an associative or numeric array
oci_fetch_assoc - Returns the next row from a query as an associative array
oci_fetch_object - Returns the next row from a query as an object
oci_fetch_row - Returns the next row from a query as a numeric array
oci_fetch - Fetches the next row from a query into internal buffers
oci_field_is_null - Checks if the field is NULL
oci_field_name - Returns the name of a field from the statement
oci_field_precision - Tell the precision of a field
oci_field_scale - Tell the scale of the field
oci_field_size - Returns field's size
oci_field_type_raw - Tell the raw Oracle data type of the field
oci_field_type - Returns field's data type
oci_free_statement - Frees all resources associated with statement or cursor
oci_internal_debug - Enables or disables internal debug output
OCI-Lob->append - Appends data from the large object to another large object
OCI-Lob->close - Closes LOB descriptor
oci_lob_copy - Copies large object
OCI-Lob->eof - Tests for end-of-file on a large object's descriptor
OCI-Lob->erase - Erases a specified portion of the internal LOB data
OCI-Lob->export - Exports LOB's contents to a file
OCI-Lob->flush - Flushes/writes buffer of the LOB to the server
OCI-Lob->free - Frees resources associated with the LOB descriptor
OCI-Lob->getBuffering - Returns current state of buffering for the large object
OCI-Lob->import - Imports file data to the LOB
oci_lob_is_equal - Compares two LOB/FILE locators for equality
OCI-Lob->load - Returns large object's contents
OCI-Lob->read - Reads part of the large object
OCI-Lob->rewind - Moves the internal pointer to the beginning of the large object
OCI-Lob->save - Saves data to the large object
OCI-Lob->saveFile - Alias of oci_lob_import
OCI-Lob->seek - Sets the internal pointer of the large object
OCI-Lob->setBuffering - Changes current state of buffering for the large object
OCI-Lob->size - Returns size of large object
OCI-Lob->tell - Returns the current position of internal pointer of large object
OCI-Lob->truncate - Truncates large object
OCI-Lob->write - Writes data to the large object
OCI-Lob->writeTemporary - Writes a temporary large object
OCI-Lob->writeToFile - Alias of oci_lob_export
oci_new_collection - Allocates new collection object
oci_new_connect - Connect to the Oracle server using a unique connection
oci_new_cursor - Allocates and returns a new cursor (statement handle)
oci_new_descriptor - Initializes a new empty LOB or FILE descriptor
oci_num_fields - Returns the number of result columns in a statement
oci_num_rows - Returns number of rows affected during statement execution
oci_parse - Prepares an Oracle statement for execution
oci_password_change - Changes password of Oracle's user
oci_pconnect - Connect to an Oracle database using a persistent connection
oci_result - Returns field's value from the fetched row
oci_rollback - Rolls back the outstanding database transaction
oci_server_version - Returns server version
oci_set_action - Sets the action name
oci_set_client_identifier - Sets the client identifier
oci_set_client_info - Sets the client information
oci_set_edition - Sets the database edition
oci_set_module_name - Sets the module name
oci_set_prefetch - Sets number of rows to be prefetched by queries
oci_statement_type - Returns the type of a statement
ocibindbyname - Alias of oci_bind_by_name
ocicancel - Alias of oci_cancel
ocicloselob - Alias of
ocicollappend - Alias of
ocicollassign - Alias of
ocicollassignelem - Alias of
ocicollgetelem - Alias of
ocicollmax - Alias of
ocicollsize - Alias of
ocicolltrim - Alias of
ocicolumnisnull - Alias of oci_field_is_null
ocicolumnname - Alias of oci_field_name
ocicolumnprecision - Alias of oci_field_precision
ocicolumnscale - Alias of oci_field_scale
ocicolumnsize - Alias of oci_field_size
ocicolumntype - Alias of oci_field_type
ocicolumntyperaw - Alias of oci_field_type_raw
ocicommit - Alias of oci_commit
ocidefinebyname - Alias of oci_define_by_name
ocierror - Alias of oci_error
ociexecute - Alias of oci_execute
ocifetch - Alias of oci_fetch
ocifetchinto - Fetches the next row into an array (deprecated)
ocifetchstatement - Alias of oci_fetch_all
ocifreecollection - Alias of
ocifreecursor - Alias of oci_free_statement
ocifreedesc - Alias of
ocifreestatement - Alias of oci_free_statement
ociinternaldebug - Alias of oci_internal_debug
ociloadlob - Alias of
ocilogoff - Alias of oci_close
ocilogon - Alias of oci_connect
ocinewcollection - Alias of oci_new_collection
ocinewcursor - Alias of oci_new_cursor
ocinewdescriptor - Alias of oci_new_descriptor
ocinlogon - Alias of oci_new_connect
ocinumcols - Alias of oci_num_fields
ociparse - Alias of oci_parse
ociplogon - Alias of oci_pconnect
ociresult - Alias of oci_result
ocirollback - Alias of oci_rollback
ocirowcount - Alias of oci_num_rows
ocisavelob - Alias of
ocisavelobfile - Alias of
ociserverversion - Alias of oci_server_version
ocisetprefetch - Alias of oci_set_prefetch
ocistatementtype - Alias of oci_statement_type
ociwritelobtofile - Alias of
ociwritetemporarylob - Alias of
OGG/Vorbis
OpenAL Audio Bindings
openal_buffer_create - Generate OpenAL buffer
openal_buffer_data - Load a buffer with data
openal_buffer_destroy - Destroys an OpenAL buffer
openal_buffer_get - Retrieve an OpenAL buffer property
openal_buffer_loadwav - Load a .wav file into a buffer
openal_context_create - Create an audio processing context
openal_context_current - Make the specified context current
openal_context_destroy - Destroys a context
openal_context_process - Process the specified context
openal_context_suspend - Suspend the specified context
openal_device_close - Close an OpenAL device
openal_device_open - Initialize the OpenAL audio layer
openal_listener_get - Retrieve a listener property
openal_listener_set - Set a listener property
openal_source_create - Generate a source resource
openal_source_destroy - Destroy a source resource
openal_source_get - Retrieve an OpenAL source property
openal_source_pause - Pause the source
openal_source_play - Start playing the source
openal_source_rewind - Rewind the source
openal_source_set - Set source property
openal_source_stop - Stop playing the source
openal_stream - Begin streaming on a source
OpenSSL
openssl_csr_export_to_file - Exports a CSR to a file
openssl_csr_export - Exports a CSR as a string
openssl_csr_get_public_key - Returns the public key of a CERT
openssl_csr_get_subject - Returns the subject of a CERT
openssl_csr_new - Generates a CSR
openssl_csr_sign - Sign a CSR with another certificate (or itself) and generate a certificate
openssl_decrypt - Decrypts data
openssl_dh_compute_key - Computes shared secret for public value of remote DH key and local DH key
openssl_digest - Computes a digest
openssl_encrypt - Encrypts data
openssl_error_string - Return openSSL error message
openssl_free_key - Free key resource
openssl_get_cipher_methods - Gets available cipher methods
openssl_get_md_methods - Gets available digest methods
openssl_get_privatekey - Alias of openssl_pkey_get_private
openssl_get_publickey - Alias of openssl_pkey_get_public
openssl_open - Open sealed data
openssl_pkcs12_export_to_file - Exports a PKCS#12 Compatible Certificate Store File
openssl_pkcs12_export - Exports a PKCS#12 Compatible Certificate Store File to variable.
openssl_pkcs12_read - Parse a PKCS#12 Certificate Store into an array
openssl_pkcs7_decrypt - Decrypts an S/MIME encrypted message
openssl_pkcs7_encrypt - Encrypt an S/MIME message
openssl_pkcs7_sign - Sign an S/MIME message
openssl_pkcs7_verify - Verifies the signature of an S/MIME signed message
openssl_pkey_export_to_file - Gets an exportable representation of a key into a file
openssl_pkey_export - Gets an exportable representation of a key into a string
openssl_pkey_free - Frees a private key
openssl_pkey_get_details - Returns an array with the key details
openssl_pkey_get_private - Get a private key
openssl_pkey_get_public - Extract public key from certificate and prepare it for use
openssl_pkey_new - Generates a new private key
openssl_private_decrypt - Decrypts data with private key
openssl_private_encrypt - Encrypts data with private key
openssl_public_decrypt - Decrypts data with public key
openssl_public_encrypt - Encrypts data with public key
openssl_random_pseudo_bytes - Generate a pseudo-random string of bytes
openssl_seal - Seal (encrypt) data
openssl_sign - Generate signature
openssl_verify - Verify signature
openssl_x509_check_private_key - Checks if a private key corresponds to a certificate
openssl_x509_checkpurpose - Verifies if a certificate can be used for a particular purpose
openssl_x509_export_to_file - Exports a certificate to file
openssl_x509_export - Exports a certificate as a string
openssl_x509_free - Free certificate resource
openssl_x509_parse - Parse an X509 certificate and return the information as an array
openssl_x509_read - Parse an X.509 certificate and return a resource identifier for
it
Output Buffering Control
flush - Flush the output buffer
ob_clean - Clean (erase) the output buffer
ob_end_clean - Clean (erase) the output buffer and turn off output buffering
ob_end_flush - Flush (send) the output buffer and turn off output buffering
ob_flush - Flush (send) the output buffer
ob_get_clean - Get current buffer contents and delete current output buffer
ob_get_contents - Return the contents of the output buffer
ob_get_flush - Flush the output buffer, return it as a string and turn off output buffering
ob_get_length - Return the length of the output buffer
ob_get_level - Return the nesting level of the output buffering mechanism
ob_get_status - Get status of output buffers
ob_gzhandler - ob_start callback function to gzip output buffer
ob_implicit_flush - Turn implicit flush on/off
ob_list_handlers - List all output handlers in use
ob_start - Turn on output buffering
output_add_rewrite_var - Add URL rewriter values
output_reset_rewrite_vars - Reset URL rewriter values
Object property and method call overloading
overload - Enable property and method call overloading for a class
Ovrimos SQL
ovrimos_close - Closes the connection to ovrimos
ovrimos_commit - Commits the transaction
ovrimos_connect - Connect to the specified database
ovrimos_cursor - Returns the name of the cursor
ovrimos_exec - Executes an SQL statement
ovrimos_execute - Executes a prepared SQL statement
ovrimos_fetch_into - Fetches a row from the result set
ovrimos_fetch_row - Fetches a row from the result set
ovrimos_field_len - Returns the length of the output column
ovrimos_field_name - Returns the output column name
ovrimos_field_num - Returns the (1-based) index of the output column
ovrimos_field_type - Returns the type of the output column
ovrimos_free_result - Frees the specified result_id
ovrimos_longreadlen - Specifies how many bytes are to be retrieved from long datatypes
ovrimos_num_fields - Returns the number of columns
ovrimos_num_rows - Returns the number of rows affected by update operations
ovrimos_prepare - Prepares an SQL statement
ovrimos_result_all - Prints the whole result set as an HTML table
ovrimos_result - Retrieves the output column
ovrimos_rollback - Rolls back the transaction
Paradox File Access
px_close - Closes a paradox database
px_create_fp - Create a new paradox database
px_date2string - Converts a date into a string.
px_delete_record - Deletes record from paradox database
px_delete - Deletes resource of paradox database
px_get_field - Returns the specification of a single field
px_get_info - Return lots of information about a paradox file
px_get_parameter - Gets a parameter
px_get_record - Returns record of paradox database
px_get_schema - Returns the database schema
px_get_value - Gets a value
px_insert_record - Inserts record into paradox database
px_new - Create a new paradox object
px_numfields - Returns number of fields in a database
px_numrecords - Returns number of records in a database
px_open_fp - Open paradox database
px_put_record - Stores record into paradox database
px_retrieve_record - Returns record of paradox database
px_set_blob_file - Sets the file where blobs are read from
px_set_parameter - Sets a parameter
px_set_tablename - Sets the name of a table (deprecated)
px_set_targetencoding - Sets the encoding for character fields (deprecated)
px_set_value - Sets a value
px_timestamp2string - Converts the timestamp into a string.
px_update_record - Updates record in paradox database
Parsekit
parsekit_compile_file - Compile a string of PHP code and return the resulting op array
parsekit_compile_string - Compile a string of PHP code and return the resulting op array
parsekit_func_arginfo - Return information regarding function argument(s)
Process Control
pcntl_alarm - Set an alarm clock for delivery of a signal
pcntl_exec - Executes specified program in current process space
pcntl_fork - Forks the currently running process
pcntl_getpriority - Get the priority of any process
pcntl_setpriority - Change the priority of any process
pcntl_signal_dispatch - Calls signal handlers for pending signals
pcntl_signal - Installs a signal handler
pcntl_sigprocmask - Sets and retrieves blocked signals
pcntl_sigtimedwait - Waits for signals, with a timeout
pcntl_sigwaitinfo - Waits for signals
pcntl_wait - Waits on or returns the status of a forked child
pcntl_waitpid - Waits on or returns the status of a forked child
pcntl_wexitstatus - Returns the return code of a terminated child
pcntl_wifexited - Checks if status code represents a normal exit
pcntl_wifsignaled - Checks whether the status code represents a termination due to a signal
pcntl_wifstopped - Checks whether the child process is currently stopped
pcntl_wstopsig - Returns the signal which caused the child to stop
pcntl_wtermsig - Returns the signal which caused the child to terminate
Regular Expressions (Perl-Compatible)
Possible modifiers in regex patterns - Pattern Modifiers
Differences From Perl - Perl Differences
PCRE regex syntax - Pattern Syntax
preg_filter - Perform a regular expression search and replace
preg_grep - Return array entries that match the pattern
preg_last_error - Returns the error code of the last PCRE regex execution
preg_match_all - Perform a global regular expression match
preg_match - Perform a regular expression match
preg_quote - Quote regular expression characters
preg_replace_callback - Perform a regular expression search and replace using a callback
preg_replace - Perform a regular expression search and replace
preg_split - Split string by a regular expression
PDF_activate_item - Activate structure element or other content item
PDF_add_annotation - Add annotation [deprecated]
PDF_add_bookmark - Add bookmark for current page [deprecated]
PDF_add_launchlink - Add launch annotation for current page [deprecated]
PDF_add_locallink - Add link annotation for current page [deprecated]
PDF_add_nameddest - Create named destination
PDF_add_note - Set annotation for current page [deprecated]
PDF_add_outline - Add bookmark for current page [deprecated]
PDF_add_pdflink - Add file link annotation for current page [deprecated]
PDF_add_table_cell - Add a cell to a new or existing table
PDF_add_textflow - Create Textflow or add text to existing Textflow
PDF_add_thumbnail - Add thumbnail for current page
PDF_add_weblink - Add weblink for current page [deprecated]
PDF_arc - Draw a counterclockwise circular arc segment
PDF_arcn - Draw a clockwise circular arc segment
PDF_attach_file - Add file attachment for current page [deprecated]
PDF_begin_document - Create new PDF file
PDF_begin_font - Start a Type 3 font definition
PDF_begin_glyph - Start glyph definition for Type 3 font
PDF_begin_item - Open structure element or other content item
PDF_begin_layer - Start layer
PDF_begin_page_ext - Start new page
PDF_begin_page - Start new page [deprecated]
PDF_begin_pattern - Start pattern definition
PDF_begin_template_ext - Start template definition
PDF_begin_template - Start template definition [deprecated]
PDF_circle - Draw a circle
PDF_clip - Clip to current path
PDF_close_image - Close image
PDF_close_pdi_page - Close the page handle
PDF_close_pdi - Close the input PDF document [deprecated]
PDF_close - Close pdf resource [deprecated]
PDF_closepath_fill_stroke - Close, fill and stroke current path
PDF_closepath_stroke - Close and stroke path
PDF_closepath - Close current path
PDF_concat - Concatenate a matrix to the CTM
PDF_continue_text - Output text in next line
PDF_create_3dview - Create 3D view
PDF_create_action - Create action for objects or events
PDF_create_annotation - Create rectangular annotation
PDF_create_bookmark - Create bookmark
PDF_create_field - Create form field
PDF_create_fieldgroup - Create form field group
PDF_create_gstate - Create graphics state object
PDF_create_pvf - Create PDFlib virtual file
PDF_create_textflow - Create textflow object
PDF_curveto - Draw Bezier curve
PDF_define_layer - Create layer definition
PDF_delete_pvf - Delete PDFlib virtual file
PDF_delete_table - Delete table object
PDF_delete_textflow - Delete textflow object
PDF_delete - Delete PDFlib object
PDF_encoding_set_char - Add glyph name and/or Unicode value
PDF_end_document - Close PDF file
PDF_end_font - Terminate Type 3 font definition
PDF_end_glyph - Terminate glyph definition for Type 3 font
PDF_end_item - Close structure element or other content item
PDF_end_layer - Deactivate all active layers
PDF_end_page_ext - Finish page
PDF_end_page - Finish page
PDF_end_pattern - Finish pattern
PDF_end_template - Finish template
PDF_endpath - End current path
PDF_fill_imageblock - Fill image block with variable data
PDF_fill_pdfblock - Fill PDF block with variable data
PDF_fill_stroke - Fill and stroke path
PDF_fill_textblock - Fill text block with variable data
PDF_fill - Fill current path
PDF_findfont - Prepare font for later use [deprecated]
PDF_fit_image - Place image or template
PDF_fit_pdi_page - Place imported PDF page
PDF_fit_table - Place table on page
PDF_fit_textflow - Format textflow in rectangular area
PDF_fit_textline - Place single line of text
PDF_get_apiname - Get name of unsuccessfull API function
PDF_get_buffer - Get PDF output buffer
PDF_get_errmsg - Get error text
PDF_get_errnum - Get error number
PDF_get_font - Get font [deprecated]
PDF_get_fontname - Get font name [deprecated]
PDF_get_fontsize - Font handling [deprecated]
PDF_get_image_height - Get image height [deprecated]
PDF_get_image_width - Get image width [deprecated]
PDF_get_majorversion - Get major version number [deprecated]
PDF_get_minorversion - Get minor version number [deprecated]
PDF_get_parameter - Get string parameter
PDF_get_pdi_parameter - Get PDI string parameter [deprecated]
PDF_get_pdi_value - Get PDI numerical parameter [deprecated]
PDF_get_value - Get numerical parameter
PDF_info_font - Query detailed information about a loaded font
PDF_info_matchbox - Query matchbox information
PDF_info_table - Retrieve table information
PDF_info_textflow - Query textflow state
PDF_info_textline - Perform textline formatting and query metrics
PDF_initgraphics - Reset graphic state
PDF_lineto - Draw a line
PDF_load_3ddata - Load 3D model
PDF_load_font - Search and prepare font
PDF_load_iccprofile - Search and prepare ICC profile
PDF_load_image - Open image file
PDF_makespotcolor - Make spot color
PDF_moveto - Set current point
PDF_new - Create PDFlib object
PDF_open_ccitt - Open raw CCITT image [deprecated]
PDF_open_file - Create PDF file [deprecated]
PDF_open_gif - Open GIF image [deprecated]
PDF_open_image_file - Read image from file [deprecated]
PDF_open_image - Use image data [deprecated]
PDF_open_jpeg - Open JPEG image [deprecated]
PDF_open_memory_image - Open image created with PHP's image functions [not supported]
PDF_open_pdi_page - Prepare a page
PDF_open_pdi - Open PDF file [deprecated]
PDF_open_tiff - Open TIFF image [deprecated]
PDF_pcos_get_number - Get value of pCOS path with type number or boolean
PDF_pcos_get_stream - Get contents of pCOS path with type stream, fstream, or string
PDF_pcos_get_string - Get value of pCOS path with type name, string, or boolean
PDF_place_image - Place image on the page [deprecated]
PDF_place_pdi_page - Place PDF page [deprecated]
PDF_process_pdi - Process imported PDF document
PDF_rect - Draw rectangle
PDF_restore - Restore graphics state
PDF_resume_page - Resume page
PDF_rotate - Rotate coordinate system
PDF_save - Save graphics state
PDF_scale - Scale coordinate system
PDF_set_border_color - Set border color of annotations [deprecated]
PDF_set_border_dash - Set border dash style of annotations [deprecated]
PDF_set_border_style - Set border style of annotations [deprecated]
PDF_set_char_spacing - Set character spacing [deprecated]
PDF_set_duration - Set duration between pages [deprecated]
PDF_set_gstate - Activate graphics state object
PDF_set_horiz_scaling - Set horizontal text scaling [deprecated]
PDF_set_info_author - Fill the author document info field [deprecated]
PDF_set_info_creator - Fill the creator document info field [deprecated]
PDF_set_info_keywords - Fill the keywords document info field [deprecated]
PDF_set_info_subject - Fill the subject document info field [deprecated]
PDF_set_info_title - Fill the title document info field [deprecated]
PDF_set_info - Fill document info field
PDF_set_layer_dependency - Define relationships among layers
PDF_set_leading - Set distance between text lines [deprecated]
PDF_set_parameter - Set string parameter
PDF_set_text_matrix - Set text matrix [deprecated]
PDF_set_text_pos - Set text position
PDF_set_text_rendering - Determine text rendering [deprecated]
PDF_set_text_rise - Set text rise [deprecated]
PDF_set_value - Set numerical parameter
PDF_set_word_spacing - Set spacing between words [deprecated]
PDF_setcolor - Set fill and stroke color
PDF_setdash - Set simple dash pattern
PDF_setdashpattern - Set dash pattern
PDF_setflat - Set flatness
PDF_setfont - Set font
PDF_setgray_fill - Set fill color to gray [deprecated]
PDF_setgray_stroke - Set stroke color to gray [deprecated]
PDF_setgray - Set color to gray [deprecated]
PDF_setlinecap - Set linecap parameter
PDF_setlinejoin - Set linejoin parameter
PDF_setlinewidth - Set line width
PDF_setmatrix - Set current transformation matrix
PDF_setmiterlimit - Set miter limit
PDF_setpolydash - Set complicated dash pattern [deprecated]
PDF_setrgbcolor_fill - Set fill rgb color values [deprecated]
PDF_setrgbcolor_stroke - Set stroke rgb color values [deprecated]
PDF_setrgbcolor - Set fill and stroke rgb color values [deprecated]
PDF_shading_pattern - Define shading pattern
PDF_shading - Define blend
PDF_shfill - Fill area with shading
PDF_show_boxed - Output text in a box [deprecated]
PDF_show_xy - Output text at given position
PDF_show - Output text at current position
PDF_skew - Skew the coordinate system
PDF_stringwidth - Return width of text
PDF_stroke - Stroke path
PDF_suspend_page - Suspend page
PDF_translate - Set origin of coordinate system
PDF_utf16_to_utf8 - Convert string from UTF-16 to UTF-8
PDF_utf32_to_utf16 - Convert string from UTF-32 to UTF-16
PDF_utf8_to_utf16 - Convert string from UTF-8 to UTF-16
PHP Data Objects
PDO::beginTransaction - Initiates a transaction
PDO::commit - Commits a transaction
PDO::__construct - Creates a PDO instance representing a connection to a database
PDO::errorCode - Fetch the SQLSTATE associated with the last operation on the database handle
PDO::errorInfo - Fetch extended error information associated with the last operation on the database handle
PDO::exec - Execute an SQL statement and return the number of affected rows
PDO::getAttribute - Retrieve a database connection attribute
PDO::getAvailableDrivers - Return an array of available PDO drivers
PDO::lastInsertId - Returns the ID of the last inserted row or sequence value
PDO::prepare - Prepares a statement for execution and returns a statement object
PDO::query - Executes an SQL statement, returning a result set as a PDOStatement object
PDO::quote - Quotes a string for use in a query.
PDO::rollBack - Rolls back a transaction
PDO::setAttribute - Set an attribute
PDOStatement->bindColumn - Bind a column to a PHP variable
PDOStatement->bindParam - Binds a parameter to the specified variable name
PDOStatement->bindValue - Binds a value to a parameter
PDOStatement->closeCursor - Closes the cursor, enabling the statement to be executed again.
PDOStatement->columnCount - Returns the number of columns in the result set
PDOStatement->debugDumpParams - Dump an SQL prepared command
PDOStatement->errorCode - Fetch the SQLSTATE associated with the last operation on the statement handle
PDOStatement->errorInfo - Fetch extended error information associated with the last operation on the statement handle
PDOStatement->execute - Executes a prepared statement
PDOStatement->fetch - Fetches the next row from a result set
PDOStatement->fetchAll - Returns an array containing all of the result set rows
PDOStatement->fetchColumn - Returns a single column from the next row of a result set
PDOStatement->fetchObject - Fetches the next row and returns it as an object.
PDOStatement->getAttribute - Retrieve a statement attribute
PDOStatement->getColumnMeta - Returns metadata for a column in a result set
PDOStatement->nextRowset - Advances to the next rowset in a multi-rowset statement handle
PDOStatement->rowCount - Returns the number of rows affected by the last SQL statement
PDOStatement->setAttribute - Set a statement attribute
PDOStatement->setFetchMode - Set the default fetch mode for this statement
MS SQL Server (PDO) - Microsoft SQL Server and Sybase Functions (PDO_DBLIB)
Firebird/Interbase (PDO) - Firebird/Interbase Functions (PDO_FIREBIRD)
IBM (PDO) - IBM Functions (PDO_IBM)
Informix (PDO) - Informix Functions (PDO_INFORMIX)
MySQL (PDO) - MySQL Functions (PDO_MYSQL)
Oracle (PDO) - Oracle Functions (PDO_OCI)
ODBC and DB2 (PDO) - ODBC and DB2 Functions (PDO_ODBC)
PostgreSQL (PDO) - PostgreSQL Functions (PDO_PGSQL)
SQLite (PDO) - SQLite Functions (PDO_SQLITE)
4D (PDO) - 4D Functions (PDO_4D)
PostgreSQL
pg_affected_rows - Returns number of affected records (tuples)
pg_cancel_query - Cancel an asynchronous query
pg_client_encoding - Gets the client encoding
pg_close - Closes a PostgreSQL connection
pg_connect - Open a PostgreSQL connection
pg_connection_busy - Get connection is busy or not
pg_connection_reset - Reset connection (reconnect)
pg_connection_status - Get connection status
pg_convert - Convert associative array values into suitable for SQL statement
pg_copy_from - Insert records into a table from an array
pg_copy_to - Copy a table to an array
pg_dbname - Get the database name
pg_delete - Deletes records
pg_end_copy - Sync with PostgreSQL backend
pg_escape_bytea - Escape a string for insertion into a bytea field
pg_escape_string - Escape a string for insertion into a text field
pg_execute - Sends a request to execute a prepared statement with given parameters, and waits for the result.
pg_fetch_all_columns - Fetches all rows in a particular result column as an array
pg_fetch_all - Fetches all rows from a result as an array
pg_fetch_array - Fetch a row as an array
pg_fetch_assoc - Fetch a row as an associative array
pg_fetch_object - Fetch a row as an object
pg_fetch_result - Returns values from a result resource
pg_fetch_row - Get a row as an enumerated array
pg_field_is_null - Test if a field is SQL NULL
pg_field_name - Returns the name of a field
pg_field_num - Returns the field number of the named field
pg_field_prtlen - Returns the printed length
pg_field_size - Returns the internal storage size of the named field
pg_field_table - Returns the name or oid of the tables field
pg_field_type_oid - Returns the type ID (OID) for the corresponding field number
pg_field_type - Returns the type name for the corresponding field number
pg_free_result - Free result memory
pg_get_notify - Gets SQL NOTIFY message
pg_get_pid - Gets the backend's process ID
pg_get_result - Get asynchronous query result
pg_host - Returns the host name associated with the connection
pg_insert - Insert array into table
pg_last_error - Get the last error message string of a connection
pg_last_notice - Returns the last notice message from PostgreSQL server
pg_last_oid - Returns the last row's OID
pg_lo_close - Close a large object
pg_lo_create - Create a large object
pg_lo_export - Export a large object to file
pg_lo_import - Import a large object from file
pg_lo_open - Open a large object
pg_lo_read_all - Reads an entire large object and send straight to browser
pg_lo_read - Read a large object
pg_lo_seek - Seeks position within a large object
pg_lo_tell - Returns current seek position a of large object
pg_lo_unlink - Delete a large object
pg_lo_write - Write to a large object
pg_meta_data - Get meta data for table
pg_num_fields - Returns the number of fields in a result
pg_num_rows - Returns the number of rows in a result
pg_options - Get the options associated with the connection
pg_parameter_status - Looks up a current parameter setting of the server.
pg_pconnect - Open a persistent PostgreSQL connection
pg_ping - Ping database connection
pg_port - Return the port number associated with the connection
pg_prepare - Submits a request to create a prepared statement with the
given parameters, and waits for completion.
pg_put_line - Send a NULL-terminated string to PostgreSQL backend
pg_query_params - Submits a command to the server and waits for the result, with the ability to pass parameters separately from the SQL command text.
pg_query - Execute a query
pg_result_error_field - Returns an individual field of an error report.
pg_result_error - Get error message associated with result
pg_result_seek - Set internal row offset in result resource
pg_result_status - Get status of query result
pg_select - Select records
pg_send_execute - Sends a request to execute a prepared statement with given parameters, without waiting for the result(s).
pg_send_prepare - Sends a request to create a prepared statement with the given parameters, without waiting for completion.
pg_send_query_params - Submits a command and separate parameters to the server without waiting for the result(s).
pg_send_query - Sends asynchronous query
pg_set_client_encoding - Set the client encoding
pg_set_error_verbosity - Determines the verbosity of messages returned by pg_last_error
and pg_result_error.
pg_trace - Enable tracing a PostgreSQL connection
pg_transaction_status - Returns the current in-transaction status of the server.
pg_tty - Return the TTY name associated with the connection
pg_unescape_bytea - Unescape binary for bytea type
pg_untrace - Disable tracing of a PostgreSQL connection
pg_update - Update table
pg_version - Returns an array with client, protocol and server version (when available)
Phar
Phar::addEmptyDir - Add an empty directory to the phar archive
Phar::addFile - Add a file from the filesystem to the phar archive
Phar::addFromString - Add a file from the filesystem to the phar archive
Phar::apiVersion - Returns the api version
Phar::buildFromDirectory - Construct a phar archive from the files within a directory.
Phar::buildFromIterator - Construct a phar archive from an iterator.
Phar::canCompress - Returns whether phar extension supports compression using either zlib or bzip2
Phar::canWrite - Returns whether phar extension supports writing and creating phars
Phar::compress - Compresses the entire Phar archive using Gzip or Bzip2 compression
Phar::compressAllFilesBZIP2 - Compresses all files in the current Phar archive using Bzip2 compression
Phar::compressAllFilesGZ - Compresses all files in the current Phar archive using Gzip compression
Phar::compressFiles - Compresses all files in the current Phar archive
Phar::__construct - Construct a Phar archive object
Phar::convertToData - Convert a phar archive to a non-executable tar or zip file
Phar::convertToExecutable - Convert a phar archive to another executable phar archive file format
Phar::copy - Copy a file internal to the phar archive to another new file within the phar
Phar::count - Returns the number of entries (files) in the Phar archive
Phar::createDefaultStub - Create a phar-file format specific stub
Phar::decompress - Decompresses the entire Phar archive
Phar::decompressFiles - Decompresses all files in the current Phar archive
Phar::delMetadata - Deletes the global metadata of the phar
Phar::delete - Delete a file within a phar archive
Phar::extractTo - Extract the contents of a phar archive to a directory
Phar::getMetaData - Returns phar archive meta-data
Phar::getModified - Return whether phar was modified
Phar::getSignature - Return MD5/SHA1/SHA256/SHA512/OpenSSL signature of a Phar archive
Phar::getStub - Return the PHP loader or bootstrap stub of a Phar archive
Phar::getSupportedCompression - Return array of supported compression algorithms
Phar::getSupportedSignatures - Return array of supported signature types
Phar::getVersion - Return version info of Phar archive
Phar::hasMetaData - Returns whether phar has global meta-data
Phar::interceptFileFuncs - instructs phar to intercept fopen, file_get_contents, opendir, and all of the stat-related functions
Phar::isBuffering - Used to determine whether Phar write operations are being buffered, or are flushing directly to disk
Phar::isCompressed - Returns Phar::GZ or PHAR::BZ2 if the entire phar archive is compressed (.tar.gz/tar.bz and so on)
Phar::isFileFormat - Returns true if the phar archive is based on the tar/phar/zip file format depending on the parameter
Phar::isValidPharFilename - Returns whether the given filename is a valid phar filename
Phar::isWritable - Returns true if the phar archive can be modified
Phar::loadPhar - Loads any phar archive with an alias
Phar::mapPhar - Reads the currently executed file (a phar) and registers its manifest
Phar::mount - Mount an external path or file to a virtual location within the phar archive
Phar::mungServer - Defines a list of up to 4 $_SERVER variables that should be modified for execution
Phar::offsetExists - determines whether a file exists in the phar
Phar::offsetGet - Gets a PharFileInfo object for a specific file
Phar::offsetSet - set the contents of an internal file to those of an external file
Phar::offsetUnset - remove a file from a phar
Phar::running - Returns the full path on disk or full phar URL to the currently executing Phar archive
Phar::setAlias - Set the alias for the Phar archive
Phar::setDefaultStub - Used to set the PHP loader or bootstrap stub of a Phar archive to the default loader
Phar::setMetadata - Sets phar archive meta-data
Phar::setSignatureAlgorithm - set the signature algorithm for a phar and apply it.
Phar::setStub - Used to set the PHP loader or bootstrap stub of a Phar archive
Phar::startBuffering - Start buffering Phar write operations, do not modify the Phar object on disk
Phar::stopBuffering - Stop buffering write requests to the Phar archive, and save changes to disk
Phar::uncompressAllFiles - Uncompresses all files in the current Phar archive
Phar::unlinkArchive - Completely remove a phar archive from disk and from memory
Phar::webPhar - mapPhar for web-based phars. front controller for web applications
PharData::addEmptyDir - Add an empty directory to the tar/zip archive
PharData::addFile - Add a file from the filesystem to the tar/zip archive
PharData::addFromString - Add a file from the filesystem to the tar/zip archive
PharData::buildFromDirectory - Construct a tar/zip archive from the files within a directory.
PharData::buildFromIterator - Construct a tar or zip archive from an iterator.
PharData::compress - Compresses the entire tar/zip archive using Gzip or Bzip2 compression
PharData::compressFiles - Compresses all files in the current tar/zip archive
PharData::__construct - Construct a non-executable tar or zip archive object
PharData::convertToData - Convert a phar archive to a non-executable tar or zip file
PharData::convertToExecutable - Convert a non-executable tar/zip archive to an executable phar archive
PharData::copy - Copy a file internal to the phar archive to another new file within the phar
PharData::decompress - Decompresses the entire Phar archive
PharData::decompressFiles - Decompresses all files in the current zip archive
PharData::delMetadata - Deletes the global metadata of a zip archive
PharData::delete - Delete a file within a tar/zip archive
PharData::extractTo - Extract the contents of a tar/zip archive to a directory
PharData::isWritable - Returns true if the tar/zip archive can be modified
PharData::offsetSet - set the contents of a file within the tar/zip to those of an external file or string
PharData::offsetUnset - remove a file from a tar/zip archive
PharData::setAlias - dummy function (Phar::setAlias is not valid for PharData)
PharData::setDefaultStub - dummy function (Phar::setDefaultStub is not valid for PharData)
Phar::setMetadata - Sets phar archive meta-data
Phar::setSignatureAlgorithm - set the signature algorithm for a phar and apply it. The
PharData::setStub - dummy function (Phar::setStub is not valid for PharData)
PharFileInfo::chmod - Sets file-specific permission bits
PharFileInfo::compress - Compresses the current Phar entry with either zlib or bzip2 compression
PharFileInfo::__construct - Construct a Phar entry object
PharFileInfo::decompress - Decompresses the current Phar entry within the phar
PharFileInfo::delMetadata - Deletes the metadata of the entry
PharFileInfo::getCRC32 - Returns CRC32 code or throws an exception if CRC has not been verified
PharFileInfo::getCompressedSize - Returns the actual size of the file (with compression) inside the Phar archive
PharFileInfo::getMetaData - Returns file-specific meta-data saved with a file
PharFileInfo::getPharFlags - Returns the Phar file entry flags
PharFileInfo::hasMetadata - Returns the metadata of the entry
PharFileInfo::isCRCChecked - Returns whether file entry has had its CRC verified
PharFileInfo::isCompressed - Returns whether the entry is compressed
PharFileInfo::isCompressedBZIP2 - Returns whether the entry is compressed using bzip2
PharFileInfo::isCompressedGZ - Returns whether the entry is compressed using gz
PharFileInfo::setCompressedBZIP2 - Compresses the current Phar entry within the phar using Bzip2 compression
PharFileInfo::setCompressedGZ - Compresses the current Phar entry within the phar using gz compression
PharFileInfo::setMetaData - Sets file-specific meta-data saved with a file
PharFileInfo::setUncompressed - Uncompresses the current Phar entry within the phar, if it is compressed
PharException - The PharException class provides a phar-specific exception class
for try/catch blocks.
POSIX
posix_access - Determine accessibility of a file
posix_ctermid - Get path name of controlling terminal
posix_errno - Alias of posix_get_last_error
posix_get_last_error - Retrieve the error number set by the last posix function that failed
posix_getcwd - Pathname of current directory
posix_getegid - Return the effective group ID of the current process
posix_geteuid - Return the effective user ID of the current process
posix_getgid - Return the real group ID of the current process
posix_getgrgid - Return info about a group by group id
posix_getgrnam - Return info about a group by name
posix_getgroups - Return the group set of the current process
posix_getlogin - Return login name
posix_getpgid - Get process group id for job control
posix_getpgrp - Return the current process group identifier
posix_getpid - Return the current process identifier
posix_getppid - Return the parent process identifier
posix_getpwnam - Return info about a user by username
posix_getpwuid - Return info about a user by user id
posix_getrlimit - Return info about system resource limits
posix_getsid - Get the current sid of the process
posix_getuid - Return the real user ID of the current process
posix_initgroups - Calculate the group access list
posix_isatty - Determine if a file descriptor is an interactive terminal
posix_kill - Send a signal to a process
posix_mkfifo - Create a fifo special file (a named pipe)
posix_mknod - Create a special or ordinary file (POSIX.1)
posix_setegid - Set the effective GID of the current process
posix_seteuid - Set the effective UID of the current process
posix_setgid - Set the GID of the current process
posix_setpgid - Set process group id for job control
posix_setsid - Make the current process a session leader
posix_setuid - Set the UID of the current process
posix_strerror - Retrieve the system error message associated with the given errno
posix_times - Get process times
posix_ttyname - Determine terminal device name
posix_uname - Get system name
Printer
printer_abort - Deletes the printer's spool file
printer_close - Close an open printer connection
printer_create_brush - Create a new brush
printer_create_dc - Create a new device context
printer_create_font - Create a new font
printer_create_pen - Create a new pen
printer_delete_brush - Delete a brush
printer_delete_dc - Delete a device context
printer_delete_font - Delete a font
printer_delete_pen - Delete a pen
printer_draw_bmp - Draw a bmp
printer_draw_chord - Draw a chord
printer_draw_elipse - Draw an ellipse
printer_draw_line - Draw a line
printer_draw_pie - Draw a pie
printer_draw_rectangle - Draw a rectangle
printer_draw_roundrect - Draw a rectangle with rounded corners
printer_draw_text - Draw text
printer_end_doc - Close document
printer_end_page - Close active page
printer_get_option - Retrieve printer configuration data
printer_list - Return an array of printers attached to the server
printer_logical_fontheight - Get logical font height
printer_open - Opens a connection to a printer
printer_select_brush - Select a brush
printer_select_font - Select a font
printer_select_pen - Select a pen
printer_set_option - Configure the printer connection
printer_start_doc - Start a new document
printer_start_page - Start a new page
printer_write - Write data to the printer
PostScript document creation
ps_add_bookmark - Add bookmark to current page
ps_add_launchlink - Adds link which launches file
ps_add_locallink - Adds link to a page in the same document
ps_add_note - Adds note to current page
ps_add_pdflink - Adds link to a page in a second pdf document
ps_add_weblink - Adds link to a web location
ps_arc - Draws an arc counterclockwise
ps_arcn - Draws an arc clockwise
ps_begin_page - Start a new page
ps_begin_pattern - Start a new pattern
ps_begin_template - Start a new template
ps_circle - Draws a circle
ps_clip - Clips drawing to current path
ps_close_image - Closes image and frees memory
ps_close - Closes a PostScript document
ps_closepath_stroke - Closes and strokes path
ps_closepath - Closes path
ps_continue_text - Continue text in next line
ps_curveto - Draws a curve
ps_delete - Deletes all resources of a PostScript document
ps_end_page - End a page
ps_end_pattern - End a pattern
ps_end_template - End a template
ps_fill_stroke - Fills and strokes the current path
ps_fill - Fills the current path
ps_findfont - Loads a font
ps_get_buffer - Fetches the full buffer containig the generated PS data
ps_get_parameter - Gets certain parameters
ps_get_value - Gets certain values
ps_hyphenate - Hyphenates a word
ps_include_file - Reads an external file with raw PostScript code
ps_lineto - Draws a line
ps_makespotcolor - Create spot color
ps_moveto - Sets current point
ps_new - Creates a new PostScript document object
ps_open_file - Opens a file for output
ps_open_image_file - Opens image from file
ps_open_image - Reads an image for later placement
ps_open_memory_image - Takes an GD image and returns an image for placement in a PS document
ps_place_image - Places image on the page
ps_rect - Draws a rectangle
ps_restore - Restore previously save context
ps_rotate - Sets rotation factor
ps_save - Save current context
ps_scale - Sets scaling factor
ps_set_border_color - Sets color of border for annotations
ps_set_border_dash - Sets length of dashes for border of annotations
ps_set_border_style - Sets border style of annotations
ps_set_info - Sets information fields of document
ps_set_parameter - Sets certain parameters
ps_set_text_pos - Sets position for text output
ps_set_value - Sets certain values
ps_setcolor - Sets current color
ps_setdash - Sets appearance of a dashed line
ps_setflat - Sets flatness
ps_setfont - Sets font to use for following output
ps_setgray - Sets gray value
ps_setlinecap - Sets appearance of line ends
ps_setlinejoin - Sets how contected lines are joined
ps_setlinewidth - Sets width of a line
ps_setmiterlimit - Sets the miter limit
ps_setoverprintmode - Sets overprint mode
ps_setpolydash - Sets appearance of a dashed line
ps_shading_pattern - Creates a pattern based on a shading
ps_shading - Creates a shading for later use
ps_shfill - Fills an area with a shading
ps_show_boxed - Output text in a box
ps_show_xy2 - Output text at position
ps_show_xy - Output text at given position
ps_show2 - Output a text at current position
ps_show - Output text
ps_string_geometry - Gets geometry of a string
ps_stringwidth - Gets width of a string
ps_stroke - Draws the current path
ps_symbol_name - Gets name of a glyph
ps_symbol_width - Gets width of a glyph
ps_symbol - Output a glyph
ps_translate - Sets translation
Pspell
pspell_add_to_personal - Add the word to a personal wordlist
pspell_add_to_session - Add the word to the wordlist in the current session
pspell_check - Check a word
pspell_clear_session - Clear the current session
pspell_config_create - Create a config used to open a dictionary
pspell_config_data_dir - location of language data files
pspell_config_dict_dir - Location of the main word list
pspell_config_ignore - Ignore words less than N characters long
pspell_config_mode - Change the mode number of suggestions returned
pspell_config_personal - Set a file that contains personal wordlist
pspell_config_repl - Set a file that contains replacement pairs
pspell_config_runtogether - Consider run-together words as valid compounds
pspell_config_save_repl - Determine whether to save a replacement pairs list
along with the wordlist
pspell_new_config - Load a new dictionary with settings based on a given config
pspell_new_personal - Load a new dictionary with personal wordlist
pspell_new - Load a new dictionary
pspell_save_wordlist - Save the personal wordlist to a file
pspell_store_replacement - Store a replacement pair for a word
pspell_suggest - Suggest spellings of a word
qtdom
qdom_error - Returns the error string from the last QDOM operation or FALSE if no errors occurred
qdom_tree - Creates a tree of an XML string
Radius
radius_acct_open - Creates a Radius handle for accounting
radius_add_server - Adds a server
radius_auth_open - Creates a Radius handle for authentication
radius_close - Frees all ressources
radius_config - Causes the library to read the given configuration file
radius_create_request - Create accounting or authentication request
radius_cvt_addr - Converts raw data to IP-Address
radius_cvt_int - Converts raw data to integer
radius_cvt_string - Converts raw data to string
radius_demangle_mppe_key - Derives mppe-keys from mangled data
radius_demangle - Demangles data
radius_get_attr - Extracts an attribute
radius_get_vendor_attr - Extracts a vendor specific attribute
radius_put_addr - Attaches an IP-Address attribute
radius_put_attr - Attaches a binary attribute
radius_put_int - Attaches an integer attribute
radius_put_string - Attaches a string attribute
radius_put_vendor_addr - Attaches a vendor specific IP-Address attribute
radius_put_vendor_attr - Attaches a vendor specific binary attribute
radius_put_vendor_int - Attaches a vendor specific integer attribute
radius_put_vendor_string - Attaches a vendor specific string attribute
radius_request_authenticator - Returns the request authenticator
radius_send_request - Sends the request and waites for a reply
radius_server_secret - Returns the shared secret
radius_strerror - Returns an error message
Rar Archiving
rar_wrapper_cache_stats - Cache hits and misses for the URL wrapper
RarArchive::close - Close RAR archive and free all resources
RarArchive::getComment - Get comment text from the RAR archive
RarArchive::getEntries - Get full list of entries from the RAR archive
RarArchive::getEntry - Get entry object from the RAR archive
RarArchive::isBroken - Test whether an archive is broken (incomplete)
RarArchive::isSolid - Check whether the RAR archive is solid
RarArchive::open - Open RAR archive
RarArchive::setAllowBroken - Whether opening broken archives is allowed
RarArchive::__toString - Get text representation
RarEntry::extract - Extract entry from the archive
RarEntry::getAttr - Get attributes of the entry
RarEntry::getCrc - Get CRC of the entry
RarEntry::getFileTime - Get entry last modification time
RarEntry::getHostOs - Get entry host OS
RarEntry::getMethod - Get pack method of the entry
RarEntry::getName - Get name of the entry
RarEntry::getPackedSize - Get packed size of the entry
RarEntry::getStream - Get file handler for entry
RarEntry::getUnpackedSize - Get unpacked size of the entry
RarEntry::getVersion - Get minimum version of RAR program required to unpack the entry
RarEntry::isDirectory - Test whether an entry represents a directory
RarEntry::isEncrypted - Test whether an entry is encrypted
RarEntry::__toString - Get text representation of entry
RarException::isUsingExceptions - Check whether error handling with exceptions is in use
RarException::setUsingExceptions - Activate and deactivate error handling with exceptions
GNU Readline
readline_add_history - Adds a line to the history
readline_callback_handler_install - Initializes the readline callback interface and terminal, prints the prompt and returns immediately
readline_callback_handler_remove - Removes a previously installed callback handler and restores terminal settings
readline_callback_read_char - Reads a character and informs the readline callback interface when a line is received
readline_clear_history - Clears the history
readline_completion_function - Registers a completion function
readline_info - Gets/sets various internal readline variables
readline_list_history - Lists the history
readline_on_new_line - Inform readline that the cursor has moved to a new line
readline_read_history - Reads the history
readline_redisplay - Redraws the display
readline_write_history - Writes the history
readline - Reads a line
GNU Recode
recode_file - Recode from file to file according to recode request
recode_string - Recode a string according to a recode request
recode - Alias of recode_string
Reflection
Reflection::export - Exports
Reflection::getModifierNames - Gets modifier names
ReflectionClass::__clone - Clones object
ReflectionClass::__construct - Constructs a ReflectionClass
ReflectionClass::export - Exports a class
ReflectionClass::getConstant - Gets defined constants
ReflectionClass::getConstants - Gets constants
ReflectionClass::getConstructor - Gets constructor
ReflectionClass::getDefaultProperties - Gets default properties
ReflectionClass::getDocComment - Gets doc comments
ReflectionClass::getEndLine - Gets end line
ReflectionClass::getExtension - Gets extension info
ReflectionClass::getExtensionName - Gets an extensions name
ReflectionClass::getFileName - Gets the filename of the file in which the class has been defined
ReflectionClass::getInterfaceNames - Gets the interface names
ReflectionClass::getInterfaces - Gets the interfaces
ReflectionClass::getMethod - Gets a ReflectionMethod
ReflectionClass::getMethods - Gets a list of methods
ReflectionClass::getModifiers - Gets modifiers
ReflectionClass::getName - Gets class name
ReflectionClass::getNamespaceName - Gets namespace name
ReflectionClass::getParentClass - Gets parent class
ReflectionClass::getProperties - Gets properties
ReflectionClass::getProperty - Gets property
ReflectionClass::getShortName - Gets short name
ReflectionClass::getStartLine - Gets starting line number
ReflectionClass::getStaticProperties - Gets static properties
ReflectionClass::getStaticPropertyValue - Gets static property value
ReflectionClass::hasConstant - Checks if constant is defined
ReflectionClass::hasMethod - Checks if method is defined
ReflectionClass::hasProperty - Checks if property is defined
ReflectionClass::implementsInterface - Implements interface
ReflectionClass::inNamespace - Checks if in namespace
ReflectionClass::isAbstract - Checks if class is abstract
ReflectionClass::isFinal - Checks if class is final
ReflectionClass::isInstance - Checks class for instance
ReflectionClass::isInstantiable - Checks if instantiable
ReflectionClass::isInterface - Checks if interface
ReflectionClass::isInternal - Checks if internal
ReflectionClass::isIterateable - Checks if iterateable
ReflectionClass::isSubclassOf - Checks if a subclass
ReflectionClass::isUserDefined - Checks if user defined
ReflectionClass::newInstance - Creates a new cass instance from given arguments.
ReflectionClass::newInstanceArgs - Creates a new cass instance from given arguments.
ReflectionClass::setStaticPropertyValue - Sets static property value
ReflectionClass::__toString - Returns the string representation of the ReflectionClass object.
ReflectionExtension::__clone - Clones
ReflectionExtension::__construct - Constructs a ReflectionExtension
ReflectionExtension::export - Export
ReflectionExtension::getClasses - Gets classes
ReflectionExtension::getClassNames - Gets class names
ReflectionExtension::getConstants - Gets constants
ReflectionExtension::getDependencies - Gets dependencies
ReflectionExtension::getFunctions - Gets extension functions
ReflectionExtension::getINIEntries - Gets extension ini entries
ReflectionExtension::getName - Gets extension name
ReflectionExtension::getVersion - Gets extension version
ReflectionExtension::info - Gets extension info
ReflectionExtension::__toString - To string
ReflectionFunction::__construct - Constructs a ReflectionFunction object
ReflectionFunction::export - Exports function
ReflectionFunction::invoke - Invokes function
ReflectionFunction::invokeArgs - Invokes function args
ReflectionFunction::isDisabled - Checks if function is disabled
ReflectionFunction::__toString - To string
ReflectionFunctionAbstract::__clone - Clones function
ReflectionFunctionAbstract::getDocComment - Gets doc comment
ReflectionFunctionAbstract::getEndLine - Gets end line number
ReflectionFunctionAbstract::getExtension - Gets extension info
ReflectionFunctionAbstract::getExtensionName - Gets extension name
ReflectionFunctionAbstract::getFileName - Gets file name
ReflectionFunctionAbstract::getName - Gets function name
ReflectionFunctionAbstract::getNamespaceName - Gets namespace name
ReflectionFunctionAbstract::getNumberOfParameters - Gets number of parameters
ReflectionFunctionAbstract::getNumberOfRequiredParameters - Gets number of required parameters
ReflectionFunctionAbstract::getParameters - Gets parameters
ReflectionFunctionAbstract::getShortName - Gets function short name
ReflectionFunctionAbstract::getStartLine - Gets starting line number
ReflectionFunctionAbstract::getStaticVariables - Gets static variables
ReflectionFunctionAbstract::inNamespace - Checks if function in namespace
ReflectionFunctionAbstract::isClosure - Checks if closure
ReflectionFunctionAbstract::isDeprecated - Checks if deprecated
ReflectionFunctionAbstract::isInternal - Checks if is internal
ReflectionFunctionAbstract::isUserDefined - Checks if user defined
ReflectionFunctionAbstract::returnsReference - Checks if returns reference
ReflectionFunctionAbstract::__toString - To string
ReflectionMethod::__construct - Constructs a ReflectionMethod
ReflectionMethod::export - Export a reflection method.
ReflectionMethod::getDeclaringClass - Gets declaring class for the reflected method.
ReflectionMethod::getModifiers - Gets the method modifiers
ReflectionMethod::getPrototype - Gets the method prototype (if there is one).
ReflectionMethod::invoke - Invoke
ReflectionMethod::invokeArgs - Invoke args
ReflectionMethod::isAbstract - Checks if method is abstract
ReflectionMethod::isConstructor - Checks if method is a constructor
ReflectionMethod::isDestructor - Checks if method is a destructor
ReflectionMethod::isFinal - Checks if method is final
ReflectionMethod::isPrivate - Checks if method is private
ReflectionMethod::isProtected - Checks if method is protected
ReflectionMethod::isPublic - Checks if method is public
ReflectionMethod::isStatic - Checks if method is static
ReflectionMethod::setAccessible - Set method accessibility
ReflectionMethod::__toString - Returns the string representation of the Reflection method object.
ReflectionObject::__construct - Constructs a ReflectionObject
ReflectionObject::export - Export
ReflectionParameter::allowsNull - Checks if null is allowed
ReflectionParameter::__clone - Clone
ReflectionParameter::__construct - Construct
ReflectionParameter::export - Exports
ReflectionParameter::getClass - Get class
ReflectionParameter::getDeclaringClass - Gets declaring class
ReflectionParameter::getDeclaringFunction - Gets declaring function
ReflectionParameter::getDefaultValue - Gets default parameter value
ReflectionParameter::getName - Gets parameter name
ReflectionParameter::getPosition - Gets parameter position
ReflectionParameter::isArray - Checks if parameter expects an array
ReflectionParameter::isDefaultValueAvailable - Checks if a default value is available
ReflectionParameter::isOptional - Checks if optional
ReflectionParameter::isPassedByReference - Checks if passed by reference
ReflectionParameter::__toString - To string
ReflectionProperty::__clone - Clone
ReflectionProperty::__construct - Construct a ReflectionProperty object
ReflectionProperty::export - Export
ReflectionProperty::getDeclaringClass - Gets declaring class
ReflectionProperty::getDocComment - Gets doc comment
ReflectionProperty::getModifiers - Gets modifiers
ReflectionProperty::getName - Gets property name
ReflectionProperty::getValue - Gets value
ReflectionProperty::isDefault - Checks if default value
ReflectionProperty::isPrivate - Checks if property is private
ReflectionProperty::isProtected - Checks if property is protected
ReflectionProperty::isPublic - Checks if property is public
ReflectionProperty::isStatic - Checks if property is static
ReflectionProperty::setAccessible - Set property accessibility
ReflectionProperty::setValue - Set property value
ReflectionProperty::__toString - To string
Reflector::export - Exports
Reflector::__toString - To string
Regular Expression (POSIX Extended)
ereg_replace - Replace regular expression
ereg - Regular expression match
eregi_replace - Replace regular expression case insensitive
eregi - Case insensitive regular expression match
split - Split string into array by regular expression
spliti - Split string into array by regular expression case insensitive
sql_regcase - Make regular expression for case insensitive match
RPM Header Reading
rpm_close - Closes an RPM file
rpm_get_tag - Retrieves a header tag from an RPM file
rpm_is_valid - Tests a filename for validity as an RPM file
rpm_open - Opens an RPM file
rpm_version - Returns a string representing the current version of the
rpmreader extension
runkit
Runkit_Sandbox - Runkit Sandbox Class -- PHP Virtual Machine
Runkit_Sandbox_Parent - Runkit Anti-Sandbox Class
runkit_class_adopt - Convert a base class to an inherited class, add ancestral methods when appropriate
runkit_class_emancipate - Convert an inherited class to a base class, removes any method whose scope is ancestral
runkit_constant_add - Similar to define(), but allows defining in class definitions as well
runkit_constant_redefine - Redefine an already defined constant
runkit_constant_remove - Remove/Delete an already defined constant
runkit_function_add - Add a new function, similar to create_function
runkit_function_copy - Copy a function to a new function name
runkit_function_redefine - Replace a function definition with a new implementation
runkit_function_remove - Remove a function definition
runkit_function_rename - Change a function's name
runkit_import - Process a PHP file importing function and class definitions, overwriting where appropriate
runkit_lint_file - Check the PHP syntax of the specified file
runkit_lint - Check the PHP syntax of the specified php code
runkit_method_add - Dynamically adds a new method to a given class
runkit_method_copy - Copies a method from class to another
runkit_method_redefine - Dynamically changes the code of the given method
runkit_method_remove - Dynamically removes the given method
runkit_method_rename - Dynamically changes the name of the given method
runkit_return_value_used - Determines if the current functions return value will be used
runkit_sandbox_output_handler - Specify a function to capture and/or process output from a runkit sandbox
runkit_superglobals - Return numerically indexed array of registered superglobals
Simple Asynchronous Messaging
SAMConnection->commit - Commits (completes) the current unit of work.
SAMConnection->connect - Establishes a connection to a Messaging Server
SAMConnection->__construct - Creates a new connection to a Messaging Server
SAMConnection->disconnect - Disconnects from a Messaging Server
SAMConnection->errno - Contains the unique numeric error code of the last executed SAM operation.
SAMConnection->error - Contains the text description of the last failed SAM operation.
SAMConnection->isConnected - Queries whether a connection is established to a Messaging Server
SAMConnection->peek - Read a message from a queue without removing it from the queue.
SAMConnection->peekAll - Read one or more messages from a queue without removing it from the queue.
SAMConnection->receive - Receive a message from a queue or subscription.
SAMConnection->remove - Remove a message from a queue.
SAMConnection->rollback - Cancels (rolls back) an in-flight unit of work.
SAMConnection->send - Send a message to a queue or publish an item to a topic.
SAMConnection::setDebug - Turn on or off additional debugging output.
SAMConnection->subscribe - Create a subscription to a specified topic.
SAMConnection->unsubscribe - Cancel a subscription to a specified topic.
SAMMessage->body - The body of the message.
SAMMessage->__construct - Creates a new Message object
SAMMessage->header - The header properties of the message.
SCA
SCA_LocalProxy::createDataObject - create an SDO
SCA_SoapProxy::createDataObject - create an SDO
SCA::createDataObject - create an SDO
SCA::getService - Obtain a proxy for a service
Break the silence operator
SDO XML Data Access Service
SDO_DAS_XML_Document::getRootDataObject - Returns the root SDO_DataObject
SDO_DAS_XML_Document::getRootElementName - Returns root element's name
SDO_DAS_XML_Document::getRootElementURI - Returns root element's URI string
SDO_DAS_XML_Document::setEncoding - Sets the given string as encoding
SDO_DAS_XML_Document::setXMLDeclaration - Sets the xml declaration
SDO_DAS_XML_Document::setXMLVersion - Sets the given string as xml version
SDO_DAS_XML::addTypes - To load a second or subsequent schema file to a SDO_DAS_XML object
SDO_DAS_XML::create - To create SDO_DAS_XML object for a given schema file
SDO_DAS_XML::createDataObject - Creates SDO_DataObject for a given namespace URI and type name
SDO_DAS_XML::createDocument - Creates an XML Document object from scratch, without the need to load a document from a file or string.
SDO_DAS_XML::loadFile - Returns SDO_DAS_XML_Document object for a given path to xml instance document
SDO_DAS_XML::loadString - Returns SDO_DAS_XML_Document for a given xml instance string
SDO_DAS_XML::saveFile - Saves the SDO_DAS_XML_Document object to a file
SDO_DAS_XML::saveString - Saves the SDO_DAS_XML_Document object to a string
Service Data Objects
SDO_DAS_ChangeSummary::beginLogging - Begin change logging
SDO_DAS_ChangeSummary::endLogging - End change logging
SDO_DAS_ChangeSummary::getChangeType - Get the type of change made to an SDO_DataObject
SDO_DAS_ChangeSummary::getChangedDataObjects - Get the changed data objects from a change summary
SDO_DAS_ChangeSummary::getOldContainer - Get the old container for a deleted SDO_DataObject
SDO_DAS_ChangeSummary::getOldValues - Get the old values for a given changed SDO_DataObject
SDO_DAS_ChangeSummary::isLogging - Test to see whether change logging is switched on
SDO_DAS_DataFactory::addPropertyToType - Adds a property to a type
SDO_DAS_DataFactory::addType - Add a new type to a model
SDO_DAS_DataFactory::getDataFactory - Get a data factory instance
SDO_DAS_DataObject::getChangeSummary - Get a data object's change summary
SDO_DAS_Setting::getListIndex - Get the list index for a changed many-valued property
SDO_DAS_Setting::getPropertyIndex - Get the property index for a changed property
SDO_DAS_Setting::getPropertyName - Get the property name for a changed property
SDO_DAS_Setting::getValue - Get the old value for the changed property
SDO_DAS_Setting::isSet - Test whether a property was set prior to being modified
SDO_DataFactory::create - Create an SDO_DataObject
SDO_DataObject::clear - Clear an SDO_DataObject's properties
SDO_DataObject::createDataObject - Create a child SDO_DataObject
SDO_DataObject::getContainer - Get a data object's container
SDO_DataObject::getSequence - Get the sequence for a data object
SDO_DataObject::getTypeName - Return the name of the type for a data object.
SDO_DataObject::getTypeNamespaceURI - Return the namespace URI of the type for a data object.
SDO_Exception::getCause - Get the cause of the exception.
SDO_List::insert - Insert into a list
SDO_Model_Property::getContainingType - Get the SDO_Model_Type which contains this property
SDO_Model_Property::getDefault - Get the default value for the property
SDO_Model_Property::getName - Get the name of the SDO_Model_Property
SDO_Model_Property::getType - Get the SDO_Model_Type of the property
SDO_Model_Property::isContainment - Test to see if the property defines a containment relationship
SDO_Model_Property::isMany - Test to see if the property is many-valued
SDO_Model_ReflectionDataObject::__construct - Construct an SDO_Model_ReflectionDataObject
SDO_Model_ReflectionDataObject::export - Get a string describing the SDO_DataObject.
SDO_Model_ReflectionDataObject::getContainmentProperty - Get the property which defines the containment relationship to the data object
SDO_Model_ReflectionDataObject::getInstanceProperties - Get the instance properties of the SDO_DataObject
SDO_Model_ReflectionDataObject::getType - Get the SDO_Model_Type for the SDO_DataObject
SDO_Model_Type::getBaseType - Get the base type for this type
SDO_Model_Type::getName - Get the name of the type
SDO_Model_Type::getNamespaceURI - Get the namespace URI of the type
SDO_Model_Type::getProperties - Get the SDO_Model_Property objects defined for the type
SDO_Model_Type::getProperty - Get an SDO_Model_Property of the type
SDO_Model_Type::isAbstractType - Test to see if this SDO_Model_Type is an abstract data type
SDO_Model_Type::isDataType - Test to see if this SDO_Model_Type is a primitive data type
SDO_Model_Type::isInstance - Test for an SDO_DataObject being an instance of this SDO_Model_Type
SDO_Model_Type::isOpenType - Test to see if this type is an open type
SDO_Model_Type::isSequencedType - Test to see if this is a sequenced type
SDO_Sequence::getProperty - Return the property for the specified sequence index.
SDO_Sequence::insert - Insert into a sequence
SDO_Sequence::move - Move an item to another sequence position
SDO Relational Data Access Service
SDO_DAS_Relational::applyChanges - Applies the changes made to a data graph back to the database.
SDO_DAS_Relational::__construct - Creates an instance of a Relational Data Access Service
SDO_DAS_Relational::createRootDataObject - Returns the special root object in an otherwise
empty data graph. Used when creating a data graph from scratch.
SDO_DAS_Relational::executePreparedQuery - Executes an SQL query passed as a prepared statement, with a
list of values to substitute for placeholders, and return the
results as a normalised data graph.
SDO_DAS_Relational::executeQuery - Executes a given SQL query against a relational database
and returns the results as a normalised data graph.
Semaphore, Shared Memory and IPC
ftok - Convert a pathname and a project identifier to a System V IPC key
msg_get_queue - Create or attach to a message queue
msg_queue_exists - Check whether a message queue exists
msg_receive - Receive a message from a message queue
msg_remove_queue - Destroy a message queue
msg_send - Send a message to a message queue
msg_set_queue - Set information in the message queue data structure
msg_stat_queue - Returns information from the message queue data structure
sem_acquire - Acquire a semaphore
sem_get - Get a semaphore id
sem_release - Release a semaphore
sem_remove - Remove a semaphore
shm_attach - Creates or open a shared memory segment
shm_detach - Disconnects from shared memory segment
shm_get_var - Returns a variable from shared memory
shm_has_var - Check whether a specific entry exists
shm_put_var - Inserts or updates a variable in shared memory
shm_remove_var - Removes a variable from shared memory
shm_remove - Removes shared memory from Unix systems
PostgreSQL Session Save Handler
session_pgsql_add_error - Increments error counts and sets last error message
session_pgsql_get_error - Returns number of errors and last error message
session_pgsql_get_field - Get custom field value
session_pgsql_reset - Reset connection to session database servers
session_pgsql_set_field - Set custom field value
session_pgsql_status - Get current save handler status
Session Handling
session_cache_expire - Return current cache expire
session_cache_limiter - Get and/or set the current cache limiter
session_commit - Alias of session_write_close
session_decode - Decodes session data from a string
session_destroy - Destroys all data registered to a session
session_encode - Encodes the current session data as a string
session_get_cookie_params - Get the session cookie parameters
session_id - Get and/or set the current session id
session_is_registered - Find out whether a global variable is registered in a session
session_module_name - Get and/or set the current session module
session_name - Get and/or set the current session name
session_regenerate_id - Update the current session id with a newly generated one
session_register - Register one or more global variables with the current session
session_save_path - Get and/or set the current session save path
session_set_cookie_params - Set the session cookie parameters
session_set_save_handler - Sets user-level session storage functions
session_start - Initialize session data
session_unregister - Unregister a global variable from the current session
session_unset - Free all session variables
session_write_close - Write session data and end session
Shared Memory
shmop_close - Close shared memory block
shmop_delete - Delete shared memory block
shmop_open - Create or open shared memory block
shmop_read - Read data from shared memory block
shmop_size - Get size of shared memory block
shmop_write - Write data into shared memory block
SimpleXML
SimpleXMLElement::addAttribute - Adds an attribute to the SimpleXML element
SimpleXMLElement::addChild - Adds a child element to the XML node
SimpleXMLElement::asXML - Return a well-formed XML string based on SimpleXML element
SimpleXMLElement::attributes - Identifies an element's attributes
SimpleXMLElement::children - Finds children of given node
SimpleXMLElement::__construct - Creates a new SimpleXMLElement object
SimpleXMLElement::count - Counts the children of an element
SimpleXMLElement::getDocNamespaces - Returns namespaces declared in document
SimpleXMLElement::getName - Gets the name of the XML element
SimpleXMLElement::getNamespaces - Returns namespaces used in document
SimpleXMLElement::registerXPathNamespace - Creates a prefix/ns context for the next XPath query
SimpleXMLElement::xpath - Runs XPath query on XML data
simplexml_import_dom - Get a SimpleXMLElement object from a DOM node.
simplexml_load_file - Interprets an XML file into an object
simplexml_load_string - Interprets a string of XML into an object
SNMP
snmp_get_quick_print - Fetches the current value of the UCD library's quick_print setting
snmp_get_valueretrieval - Return the method how the SNMP values will be returned
snmp_read_mib - Reads and parses a MIB file into the active MIB tree
snmp_set_enum_print - Return all values that are enums with their enum value instead of the raw integer
snmp_set_oid_numeric_print - Return all objects including their respective object id within the specified one
snmp_set_oid_output_format - Set the OID output format
snmp_set_quick_print - Set the value of quick_print within the UCD SNMP library
snmp_set_valueretrieval - Specify the method how the SNMP values will be returned
snmpget - Fetch an SNMP object
snmpgetnext - Fetch a SNMP object
snmprealwalk - Return all objects including their respective object ID within the specified one
snmpset - Set an SNMP object
snmpwalk - Fetch all the SNMP objects from an agent
snmpwalkoid - Query for a tree of information about a network entity
SOAP
is_soap_fault - Checks if a SOAP call has failed
use_soap_error_handler - Set whether to use the SOAP error handler
SoapClient::__call - Calls a SOAP function (deprecated)
SoapClient::__construct - SoapClient constructor
SoapClient::__doRequest - Performs a SOAP request
SoapClient::__getFunctions - Returns list of available SOAP functions
SoapClient::__getLastRequest - Returns last SOAP request
SoapClient::__getLastRequestHeaders - Returns the SOAP headers from the last request
SoapClient::__getLastResponse - Returns last SOAP response
SoapClient::__getLastResponseHeaders - Returns the SOAP headers from the last response
SoapClient::__getTypes - Returns a list of SOAP types
SoapClient::__setCookie - The __setCookie purpose
SoapClient::__setLocation - Sets the location of the Web service to use
SoapClient::__setSoapHeaders - Sets SOAP headers for subsequent calls
SoapClient::__soapCall - Calls a SOAP function
SoapClient::SoapClient - SoapClient constructor
SoapServer::addFunction - Adds one or more functions to handle SOAP requests
SoapServer::addSoapHeader - Add a SOAP header to the response
SoapServer::__construct - SoapServer constructor
SoapServer::fault - Issue SoapServer fault indicating an error
SoapServer::getFunctions - Returns list of defined functions
SoapServer::handle - Handles a SOAP request
SoapServer::setClass - Sets the class which handles SOAP requests
SoapServer::setObject - Sets the object which will be used to handle SOAP requests
SoapServer::setPersistence - Sets SoapServer persistence mode
SoapServer::SoapServer - SoapServer constructor
SoapFault::__construct - SoapFault constructor
SoapFault::SoapFault - SoapFault constructor
SoapFault::__toString - Obtain a string representation of a SoapFault
SoapHeader::__construct - SoapHeader constructor
SoapHeader::SoapHeader - SoapHeader constructor
SoapParam::__construct - SoapParam constructor
SoapParam::SoapParam - SoapParam constructor
SoapVar::__construct - SoapVar constructor
SoapVar::SoapVar - SoapVar constructor
Sockets
socket_accept - Accepts a connection on a socket
socket_bind - Binds a name to a socket
socket_clear_error - Clears the error on the socket or the last error code
socket_close - Closes a socket resource
socket_connect - Initiates a connection on a socket
socket_create_listen - Opens a socket on port to accept connections
socket_create_pair - Creates a pair of indistinguishable sockets and stores them in an array
socket_create - Create a socket (endpoint for communication)
socket_get_option - Gets socket options for the socket
socket_getpeername - Queries the remote side of the given socket which may either result in host/port or in a Unix filesystem path, dependent on its type
socket_getsockname - Queries the local side of the given socket which may either result in host/port or in a Unix filesystem path, dependent on its type
socket_last_error - Returns the last error on the socket
socket_listen - Listens for a connection on a socket
socket_read - Reads a maximum of length bytes from a socket
socket_recv - Receives data from a connected socket
socket_recvfrom - Receives data from a socket whether or not it is connection-oriented
socket_select - Runs the select() system call on the given arrays of sockets with a specified timeout
socket_send - Sends data to a connected socket
socket_sendto - Sends a message to a socket, whether it is connected or not
socket_set_block - Sets blocking mode on a socket resource
socket_set_nonblock - Sets nonblocking mode for file descriptor fd
socket_set_option - Sets socket options for the socket
socket_shutdown - Shuts down a socket for receiving, sending, or both
socket_strerror - Return a string describing a socket error
socket_write - Write to a socket
Apache Solr
solr_get_version - Returns the current version of the Apache Solr extension
SolrUtils::digestXmlResponse - Parses an response XML string into a SolrObject
SolrUtils::escapeQueryChars - Escapes a lucene query string
SolrUtils::getSolrVersion - Returns the current version of the Solr extension
SolrUtils::queryPhrase - Prepares a phrase from an unescaped lucene string
SolrInputDocument::addField - Adds a field to the document
SolrInputDocument::clear - Resets the input document
SolrInputDocument::__clone - Creates a copy of a SolrDocument
SolrInputDocument::__construct - Constructor
SolrInputDocument::deleteField - Removes a field from the document
SolrInputDocument::__destruct - Destructor
SolrInputDocument::fieldExists - Checks if a field exists
SolrInputDocument::getBoost - Retrieves the current boost value for the document
SolrInputDocument::getField - Retrieves a field by name
SolrInputDocument::getFieldBoost - Retrieves the boost value for a particular field
SolrInputDocument::getFieldCount - Returns the number of fields in the document
SolrInputDocument::getFieldNames - Returns an array containing all the fields in the document
SolrInputDocument::merge - Merges one input document into another
SolrInputDocument::reset - This is an alias of SolrInputDocument::clear
SolrInputDocument::setBoost - Sets the boost value for this document
SolrInputDocument::setFieldBoost - Sets the index-time boost value for a field
SolrInputDocument::sort - Sorts the fields within the document
SolrInputDocument::toArray - Returns an array representation of the input document
SolrDocument::addField - Adds a field to the document
SolrDocument::clear - Drops all the fields in the document
SolrDocument::__clone - Creates a copy of a SolrDocument object
SolrDocument::__construct - Constructor
SolrDocument::current - Retrieves the current field
SolrDocument::deleteField - Removes a field from the document
SolrDocument::__destruct - Destructor
SolrDocument::fieldExists - Checks if a field exists in the document
SolrDocument::__get - Acess the field as a property
SolrDocument::getField - Retrieves a field by name
SolrDocument::getFieldCount - Returns the number of fields in this document
SolrDocument::getFieldNames - Returns an array of fields names in the document
SolrDocument::getInputDocument - Returns a SolrInputDocument equivalent of the object
SolrDocument::__isset - Checks if a field exists
SolrDocument::key - Retrieves the current key
SolrDocument::merge - Merges source to the current SolrDocument
SolrDocument::next - Moves the internal pointer to the next field
SolrDocument::offsetExists - Checks if a particular field exists
SolrDocument::offsetGet - Retrieves a field
SolrDocument::offsetSet - Adds a field to the document
SolrDocument::offsetUnset - Removes a field
SolrDocument::reset - This is an alias to SolrDocument::clear()
SolrDocument::rewind - Resets the internal pointer to the beginning
SolrDocument::serialize - Used for custom serialization
SolrDocument::__set - Adds another field to the document
SolrDocument::sort - Sorts the fields in the document
SolrDocument::toArray - Returns an array representation of the document
SolrDocument::unserialize - Custom serialization of SolrDocument objects
SolrDocument::__unset - Removes a field from the document
SolrDocument::valid - Checks if the current position internally is still valid
SolrDocumentField::__construct - Constructor
SolrDocumentField::__destruct - Destructor
SolrObject::__construct - Creates Solr object
SolrObject::__destruct - Destructor
SolrObject::getPropertyNames - Returns an array of all the names of the properties
SolrObject::offsetExists - Checks if the property exists
SolrObject::offsetGet - Used to retrieve a property
SolrObject::offsetSet - Sets the value for a property
SolrObject::offsetUnset - Sets the value for the property
SolrClient::addDocument - Adds a document to the index
SolrClient::addDocuments - Adds a collection of SolrInputDocument instances to the index
SolrClient::commit - Finalizes all add/deletes made to the index
SolrClient::__construct - Constructor for the SolrClient object
SolrClient::deleteById - Delete by Id
SolrClient::deleteByIds - Deletes by Ids
SolrClient::deleteByQueries - Removes all documents matching any of the queries
SolrClient::deleteByQuery - Deletes all documents matching the given query
SolrClient::__destruct - Destructor for SolrClient
SolrClient::getDebug - Returns the debug data for the last connection attempt
SolrClient::getOptions - Returns the client options set internally
SolrClient::optimize - Defragments the index
SolrClient::ping - Checks if Solr server is still up
SolrClient::query - Sends a query to the server
SolrClient::request - Sends a raw update request
SolrClient::rollback - Rollbacks all add/deletes made to the index since the last commit
SolrClient::setResponseWriter - Sets the response writer used to prepare the response from Solr
SolrClient::setServlet - Changes the specified servlet type to a new value
SolrClient::threads - Checks the threads status
SolrResponse::getDigestedResponse - Returns the XML response as serialized PHP data
SolrResponse::getHttpStatus - Returns the HTTP status of the response
SolrResponse::getHttpStatusMessage - Returns more details on the HTTP status
SolrResponse::getRawRequest - Returns the raw request sent to the Solr server
SolrResponse::getRawRequestHeaders - Returns the raw request headers sent to the Solr server
SolrResponse::getRawResponse - Returns the raw response from the server
SolrResponse::getRawResponseHeaders - Returns the raw response headers from the server
SolrResponse::getRequestUrl - Returns the full URL the request was sent to
SolrResponse::getResponse - Returns a SolrObject representing the XML response from the server
SolrResponse::setParseMode - Sets the parse mode
SolrResponse::success - Was the request a success
SolrQueryResponse::__construct - Constructor
SolrQueryResponse::__destruct - Destructor
SolrUpdateResponse::__construct - Constructor
SolrUpdateResponse::__destruct - Destructor
SolrPingResponse::__construct - Constructor
SolrPingResponse::__destruct - Destructor
SolrPingResponse::getResponse - Returns the response from the server
SolrGenericResponse::__construct - Constructor
SolrGenericResponse::__destruct - Destructor
SolrParams::add - This is an alias for SolrParams::addParam
SolrParams::addParam - Adds a parameter to the object
SolrParams::get - This is an alias for SolrParams::getParam
SolrParams::getParam - Returns a parameter value
SolrParams::getParams - Returns an array of non URL-encoded parameters
SolrParams::getPreparedParams - Returns an array of URL-encoded parameters
SolrParams::serialize - Used for custom serialization
SolrParams::set - An alias of SolrParams::setParam
SolrParams::setParam - Sets the parameter to the specified value
SolrParams::toString - Returns all the name-value pair parameters in the object
SolrParams::unserialize - Used for custom serialization
SolrModifiableParams::__construct - Constructor
SolrModifiableParams::__destruct - Destructor
SolrQuery::addFacetDateField - Maps to facet.date
SolrQuery::addFacetDateOther - Adds another facet.date.other parameter
SolrQuery::addFacetField - Adds another field to the facet
SolrQuery::addFacetQuery - Adds a facet query
SolrQuery::addField - Specifies which fields to return in the result
SolrQuery::addFilterQuery - Specifies a filter query
SolrQuery::addHighlightField - Maps to hl.fl
SolrQuery::addMltField - Sets a field to use for similarity
SolrQuery::addMltQueryField - Maps to mlt.qf
SolrQuery::addSortField - Used to control how the results should be sorted
SolrQuery::addStatsFacet - Requests a return of sub results for values within the given facet
SolrQuery::addStatsField - Maps to stats.field parameter
SolrQuery::__construct - Constructor
SolrQuery::__destruct - Destructor
SolrQuery::getFacet - Returns the value of the facet parameter
SolrQuery::getFacetDateEnd - Returns the value for the facet.date.end parameter
SolrQuery::getFacetDateFields - Returns all the facet.date fields
SolrQuery::getFacetDateGap - Returns the value of the facet.date.gap parameter
SolrQuery::getFacetDateHardEnd - Returns the value of the facet.date.hardend parameter
SolrQuery::getFacetDateOther - Returns the value for the facet.date.other parameter
SolrQuery::getFacetDateStart - Returns the lower bound for the first date range for all date faceting on this field
SolrQuery::getFacetFields - Returns all the facet fields
SolrQuery::getFacetLimit - Returns the maximum number of constraint counts that should be returned for the facet fields
SolrQuery::getFacetMethod - Returns the value of the facet.method parameter
SolrQuery::getFacetMinCount - Returns the minimum counts for facet fields should be included in the response
SolrQuery::getFacetMissing - Returns the current state of the facet.missing parameter
SolrQuery::getFacetOffset - Returns an offset into the list of constraints to be used for pagination
SolrQuery::getFacetPrefix - Returns the facet prefix
SolrQuery::getFacetQueries - Returns all the facet queries
SolrQuery::getFacetSort - Returns the facet sort type
SolrQuery::getFields - Returns the list of fields that will be returned in the response
SolrQuery::getFilterQueries - Returns an array of filter queries
SolrQuery::getHighlight - Returns the state of the hl parameter
SolrQuery::getHighlightAlternateField - Returns the highlight field to use as backup or default
SolrQuery::getHighlightFields - Returns all the fields that Solr should generate highlighted snippets for
SolrQuery::getHighlightFormatter - Returns the formatter for the highlighted output
SolrQuery::getHighlightFragmenter - Returns the text snippet generator for highlighted text
SolrQuery::getHighlightFragsize - Returns the number of characters of fragments to consider for highlighting
SolrQuery::getHighlightHighlightMultiTerm - Returns whether or not to enable highlighting for range/wildcard/fuzzy/prefix queries
SolrQuery::getHighlightMaxAlternateFieldLength - Returns the maximum number of characters of the field to return
SolrQuery::getHighlightMaxAnalyzedChars - Returns the maximum number of characters into a document to look for suitable snippets
SolrQuery::getHighlightMergeContiguous - Returns whether or not the collapse contiguous fragments into a single fragment
SolrQuery::getHighlightRegexMaxAnalyzedChars - Returns the maximum number of characters from a field when using the regex fragmenter
SolrQuery::getHighlightRegexPattern - Returns the regular expression for fragmenting
SolrQuery::getHighlightRegexSlop - Returns the deviation factor from the ideal fragment size
SolrQuery::getHighlightRequireFieldMatch - Returns if a field will only be highlighted if the query matched in this particular field
SolrQuery::getHighlightSimplePost - Returns the text which appears after a highlighted term
SolrQuery::getHighlightSimplePre - Returns the text which appears before a highlighted term
SolrQuery::getHighlightSnippets - Returns the maximum number of highlighted snippets to generate per field
SolrQuery::getHighlightUsePhraseHighlighter - Returns the state of the hl.usePhraseHighlighter parameter
SolrQuery::getMlt - Returns whether or not MoreLikeThis results should be enabled
SolrQuery::getMltBoost - Returns whether or not the query will be boosted by the interesting term relevance
SolrQuery::getMltCount - Returns the number of similar documents to return for each result
SolrQuery::getMltFields - Returns all the fields to use for similarity
SolrQuery::getMltMaxNumQueryTerms - Returns the maximum number of query terms that will be included in any generated query
SolrQuery::getMltMaxNumTokens - Returns the maximum number of tokens to parse in each document field that is not stored with TermVector support
SolrQuery::getMltMaxWordLength - Returns the maximum word length above which words will be ignored
SolrQuery::getMltMinDocFrequency - Returns the treshold frequency at which words will be ignored which do not occur in at least this many docs
SolrQuery::getMltMinTermFrequency - Returns the frequency below which terms will be ignored in the source document
SolrQuery::getMltMinWordLength - Returns the minimum word length below which words will be ignored
SolrQuery::getMltQueryFields - Returns the query fields and their boosts
SolrQuery::getQuery - Returns the main query
SolrQuery::getRows - Returns the maximum number of documents
SolrQuery::getSortFields - Returns all the sort fields
SolrQuery::getStart - Returns the offset in the complete result set
SolrQuery::getStats - Returns whether or not stats is enabled
SolrQuery::getStatsFacets - Returns all the stats facets that were set
SolrQuery::getStatsFields - Returns all the statistics fields
SolrQuery::getTerms - Returns whether or not the TermsComponent is enabled
SolrQuery::getTermsField - Returns the field from which the terms are retrieved
SolrQuery::getTermsIncludeLowerBound - Returns whether or not to include the lower bound in the result set
SolrQuery::getTermsIncludeUpperBound - Returns whether or not to include the upper bound term in the result set
SolrQuery::getTermsLimit - Returns the maximum number of terms Solr should return
SolrQuery::getTermsLowerBound - Returns the term to start at
SolrQuery::getTermsMaxCount - Returns the maximum document frequency
SolrQuery::getTermsMinCount - Returns the minimum document frequency to return in order to be included
SolrQuery::getTermsPrefix - Returns the term prefix
SolrQuery::getTermsReturnRaw - Whether or not to return raw characters
SolrQuery::getTermsSort - Returns an integer indicating how terms are sorted
SolrQuery::getTermsUpperBound - Returns the term to stop at
SolrQuery::getTimeAllowed - Returns the time in milliseconds allowed for the query to finish
SolrQuery::removeFacetDateField - Removes one of the facet date fields
SolrQuery::removeFacetDateOther - Removes one of the facet.date.other parameters
SolrQuery::removeFacetField - Removes one of the facet.date parameters
SolrQuery::removeFacetQuery - Removes one of the facet.query parameters
SolrQuery::removeField - Removes a field from the list of fields
SolrQuery::removeFilterQuery - Removes a filter query
SolrQuery::removeHighlightField - Removes one of the fields used for highlighting
SolrQuery::removeMltField - Removes one of the moreLikeThis fields
SolrQuery::removeMltQueryField - Removes one of the moreLikeThis query fields
SolrQuery::removeSortField - Removes one of the sort fields
SolrQuery::removeStatsFacet - Removes one of the stats.facet parameters
SolrQuery::removeStatsField - Removes one of the stats.field parameters
SolrQuery::setEchoHandler - Toggles the echoHandler parameter
SolrQuery::setEchoParams - Determines what kind of parameters to include in the response
SolrQuery::setExplainOther - Sets the explainOther common query parameter
SolrQuery::setFacet - Maps to the facet parameter. Enables or disables facetting
SolrQuery::setFacetDateEnd - Maps to facet.date.end
SolrQuery::setFacetDateGap - Maps to facet.date.gap
SolrQuery::setFacetDateHardEnd - Maps to facet.date.hardend
SolrQuery::setFacetDateStart - Maps to facet.date.start
SolrQuery::setFacetEnumCacheMinDefaultFrequency - Sets the minimum document frequency used for determining term count
SolrQuery::setFacetLimit - Maps to facet.limit
SolrQuery::setFacetMethod - Specifies the type of algorithm to use when faceting a field
SolrQuery::setFacetMinCount - Maps to facet.mincount
SolrQuery::setFacetMissing - Maps to facet.missing
SolrQuery::setFacetOffset - Sets the offset into the list of constraints to allow for pagination
SolrQuery::setFacetPrefix - Specifies a string prefix with which to limits the terms on which to facet
SolrQuery::setFacetSort - Determines the ordering of the facet field constraints
SolrQuery::setHighlight - Enables or disables highlighting
SolrQuery::setHighlightAlternateField - Specifies the backup field to use
SolrQuery::setHighlightFormatter - Specify a formatter for the highlight output
SolrQuery::setHighlightFragmenter - Sets a text snippet generator for highlighted text
SolrQuery::setHighlightFragsize - The size of fragments to consider for highlighting
SolrQuery::setHighlightHighlightMultiTerm - Use SpanScorer to highlight phrase terms
SolrQuery::setHighlightMaxAlternateFieldLength - Sets the maximum number of characters of the field to return
SolrQuery::setHighlightMaxAnalyzedChars - Specifies the number of characters into a document to look for suitable snippets
SolrQuery::setHighlightMergeContiguous - Whether or not to collapse contiguous fragments into a single fragment
SolrQuery::setHighlightRegexMaxAnalyzedChars - Specify the maximum number of characters to analyze
SolrQuery::setHighlightRegexPattern - Specify the regular expression for fragmenting
SolrQuery::setHighlightRegexSlop - Sets the factor by which the regex fragmenter can stray from the ideal fragment size
SolrQuery::setHighlightRequireFieldMatch - Require field matching during highlighting
SolrQuery::setHighlightSimplePost - Sets the text which appears after a highlighted term
SolrQuery::setHighlightSimplePre - Sets the text which appears before a highlighted term
SolrQuery::setHighlightSnippets - Sets the maximum number of highlighted snippets to generate per field
SolrQuery::setHighlightUsePhraseHighlighter - Whether to highlight phrase terms only when they appear within the query phrase
SolrQuery::setMlt - Enables or disables moreLikeThis
SolrQuery::setMltBoost - Set if the query will be boosted by the interesting term relevance
SolrQuery::setMltCount - Set the number of similar documents to return for each result
SolrQuery::setMltMaxNumQueryTerms - Sets the maximum number of query terms included
SolrQuery::setMltMaxNumTokens - Specifies the maximum number of tokens to parse
SolrQuery::setMltMaxWordLength - Sets the maximum word length
SolrQuery::setMltMinDocFrequency - Sets the mltMinDoc frequency
SolrQuery::setMltMinTermFrequency - Sets the frequency below which terms will be ignored in the source docs
SolrQuery::setMltMinWordLength - Sets the minimum word length
SolrQuery::setOmitHeader - Exclude the header from the returned results
SolrQuery::setQuery - Sets the search query
SolrQuery::setRows - Specifies the maximum number of rows to return in the result
SolrQuery::setShowDebugInfo - Flag to show debug information
SolrQuery::setStart - Specifies the number of rows to skip
SolrQuery::setStats - Enables or disables the Stats component
SolrQuery::setTerms - Enables or disables the TermsComponent
SolrQuery::setTermsField - Sets the name of the field to get the Terms from
SolrQuery::setTermsIncludeLowerBound - Include the lower bound term in the result set
SolrQuery::setTermsIncludeUpperBound - Include the upper bound term in the result set
SolrQuery::setTermsLimit - Sets the maximum number of terms to return
SolrQuery::setTermsLowerBound - Specifies the Term to start from
SolrQuery::setTermsMaxCount - Sets the maximum document frequency
SolrQuery::setTermsMinCount - Sets the minimum document frequency
SolrQuery::setTermsPrefix - Restrict matches to terms that start with the prefix
SolrQuery::setTermsReturnRaw - Return the raw characters of the indexed term
SolrQuery::setTermsSort - Specifies how to sort the returned terms
SolrQuery::setTermsUpperBound - Sets the term to stop at
SolrQuery::setTimeAllowed - The time allowed for search to finish
SolrException::getInternalInfo - Returns internal information where the Exception was thrown
SolrClientException::getInternalInfo - Returns internal information where the Exception was thrown
SolrIllegalArgumentException::getInternalInfo - Returns internal information where the Exception was thrown
SolrIllegalOperationException::getInternalInfo - Returns internal information where the Exception was thrown
Sphinx Client
SphinxClient::addQuery - Add query to multi-query batch
SphinxClient::buildExcerpts - Build text snippets
SphinxClient::buildKeywords - Extract keywords from query
SphinxClient::close - Closes previously opened persistent connection
SphinxClient::__construct - Create a new SphinxClient object
SphinxClient::escapeString - Escape special characters
SphinxClient::getLastError - Get the last error message
SphinxClient::getLastWarning - Get the last warning
SphinxClient::open - Opens persistent connection to the server
SphinxClient::query - Execute search query
SphinxClient::resetFilters - Clear all filters
SphinxClient::resetGroupBy - Clear all group-by settings
SphinxClient::runQueries - Run a batch of search queries
SphinxClient::setArrayResult - Change the format of result set array
SphinxClient::setConnectTimeout - Set connection timeout
SphinxClient::setFieldWeights - Set field weights
SphinxClient::setFilter - Add new integer values set filter
SphinxClient::setFilterFloatRange - Add new float range filter
SphinxClient::setFilterRange - Add new integer range filter
SphinxClient::setGeoAnchor - Set anchor point for a geosphere distance calculations
SphinxClient::setGroupBy - Set grouping attribute
SphinxClient::setGroupDistinct - Set attribute name for per-group distinct values count calculations
SphinxClient::setIDRange - Set a range of accepted document IDs
SphinxClient::setIndexWeights - Set per-index weights
SphinxClient::setLimits - Set offset and limit of the result set
SphinxClient::setMatchMode - Set full-text query matching mode
SphinxClient::setMaxQueryTime - Set maximum query time
SphinxClient::setOverride - Sets temporary per-document attribute value
overrides
SphinxClient::setRankingMode - Set ranking mode
SphinxClient::setRetries - Set retry count and delay
SphinxClient::setSelect - Set select clause
SphinxClient::setServer - Set searchd host and port
SphinxClient::setSortMode - Set matches sorting mode
SphinxClient::status - Queries searchd status
SphinxClient::updateAttributes - Update document attributes
SPL Type Handling
SplInt::__construct - Constructs an integer object type
SplFloat::__construct - Constructs a float object type
SplEnum::__construct - Constructs an enumeger object type
SplBool::__construct - Constructs a bool object type
SplString::__construct - Constructs a string object type
Standard PHP Library (SPL)
SplDoublyLinkedList - The SplDoublyLinkedList class
SplStack - The SplStack class
SplQueue - The SplQueue class
SplHeap - The SplHeap class
SplMaxHeap - The SplMaxHeap class
SplMinHeap - The SplMinHeap class
SplPriorityQueue - The SplPriorityQueue class
SplFixedArray - The SplFixedArray class
SplObjectStorage - The SplObjectStorage class
AppendIterator - The AppendIterator class
ArrayIterator - The ArrayIterator class
CachingIterator - The CachingIterator class
DirectoryIterator - The DirectoryIterator class
EmptyIterator - The EmptyIterator class
FilesystemIterator - The FilesystemIterator class
FilterIterator - The FilterIterator class
GlobIterator - The GlobIterator class
InfiniteIterator - The InfiniteIterator class
IteratorIterator - The IteratorIterator class
LimitIterator - The LimitIterator class
MultipleIterator - The MultipleIterator class
NoRewindIterator - The NoRewindIterator class
ParentIterator - The ParentIterator class
RecursiveArrayIterator - The RecursiveArrayIterator class
RecursiveCachingIterator - The RecursiveCachingIterator class
RecursiveDirectoryIterator - The RecursiveDirectoryIterator class
RecursiveFilterIterator - The RecursiveFilterIterator class
RecursiveIteratorIterator - The RecursiveIteratorIterator class
RecursiveRegexIterator - The RecursiveRegexIterator class
RecursiveTreeIterator - The RecursiveTreeIterator class
RegexIterator - The RegexIterator class
SimpleXMLIterator - The SimpleXMLIterator class
Countable - The Countable interface
OuterIterator - The OuterIterator interface
RecursiveIterator - The RecursiveIterator interface
SeekableIterator - The SeekableIterator interface
BadFunctionCallException - The BadFunctionCallException class
BadMethodCallException - The BadMethodCallException class
DomainException - The DomainException class
InvalidArgumentException - The InvalidArgumentException class
LengthException - The LengthException class
LogicException - The LogicException class
OutOfBoundsException - The OutOfBoundsException class
OutOfRangeException - The OutOfRangeException class
OverflowException - The OverflowException class
RangeException - The RangeException class
RuntimeException - The RuntimeException class
UnderflowException - The UnderflowException class
UnexpectedValueException - The UnexpectedValueException class
class_implements - Return the interfaces which are implemented by the given class
class_parents - Return the parent classes of the given class
iterator_apply - Call a function for every element in an iterator
iterator_count - Count the elements in an iterator
iterator_to_array - Copy the iterator into an array
spl_autoload_call - Try all registered __autoload() function to load the requested class
spl_autoload_extensions - Register and return default file extensions for spl_autoload
spl_autoload_functions - Return all registered __autoload() functions
spl_autoload_register - Register given function as __autoload() implementation
spl_autoload_unregister - Unregister given function as __autoload() implementation
spl_autoload - Default implementation for __autoload()
spl_classes - Return available SPL classes
spl_object_hash - Return hash id for given object
SplFileInfo - The SplFileInfo class
SplFileObject - The SplFileObject class
SplTempFileObject - The SplTempFileObject class
ArrayObject - The ArrayObject class
SplObserver - The SplObserver interface
SplSubject - The SplSubject interface
SPPLUS Payment System
calcul_hmac - Obtain a hmac key (needs 8 arguments)
calculhmac - Obtain a hmac key (needs 2 arguments)
nthmac - Obtain a nthmac key (needs 2 arguments)
signeurlpaiement - Obtain the payment url (needs 2 arguments)
SQLite
sqlite_array_query - Execute a query against a given database and returns an array
sqlite_busy_timeout - Set busy timeout duration, or disable busy handlers
sqlite_changes - Returns the number of rows that were changed by the most
recent SQL statement
sqlite_close - Closes an open SQLite database
sqlite_column - Fetches a column from the current row of a result set
sqlite_create_aggregate - Register an aggregating UDF for use in SQL statements
sqlite_create_function - Registers a "regular" User Defined Function for use in SQL statements
sqlite_current - Fetches the current row from a result set as an array
sqlite_error_string - Returns the textual description of an error code
sqlite_escape_string - Escapes a string for use as a query parameter
sqlite_exec - Executes a result-less query against a given database
sqlite_factory - Opens an SQLite database and returns an SQLiteDatabase object
sqlite_fetch_all - Fetches all rows from a result set as an array of arrays
sqlite_fetch_array - Fetches the next row from a result set as an array
sqlite_fetch_column_types - Return an array of column types from a particular table
sqlite_fetch_object - Fetches the next row from a result set as an object
sqlite_fetch_single - Fetches the first column of a result set as a string
sqlite_fetch_string - Alias of sqlite_fetch_single
sqlite_field_name - Returns the name of a particular field
sqlite_has_more - Finds whether or not more rows are available
sqlite_has_prev - Returns whether or not a previous row is available
sqlite_key - Returns the current row index
sqlite_last_error - Returns the error code of the last error for a database
sqlite_last_insert_rowid - Returns the rowid of the most recently inserted row
sqlite_libencoding - Returns the encoding of the linked SQLite library
sqlite_libversion - Returns the version of the linked SQLite library
sqlite_next - Seek to the next row number
sqlite_num_fields - Returns the number of fields in a result set
sqlite_num_rows - Returns the number of rows in a buffered result set
sqlite_open - Opens an SQLite database and create the database if it does not exist
sqlite_popen - Opens a persistent handle to an SQLite database and create the database if it does not exist
sqlite_prev - Seek to the previous row number of a result set
sqlite_query - Executes a query against a given database and returns a result handle
sqlite_rewind - Seek to the first row number
sqlite_seek - Seek to a particular row number of a buffered result set
sqlite_single_query - Executes a query and returns either an array for one single column or the value of the first row
sqlite_udf_decode_binary - Decode binary data passed as parameters to an UDF
sqlite_udf_encode_binary - Encode binary data before returning it from an UDF
sqlite_unbuffered_query - Execute a query that does not prefetch and buffer all data
sqlite_valid - Returns whether more rows are available
SQLite3
SQLite3::changes - Returns the number of database rows that were changed (or inserted or
deleted) by the most recent SQL statement
SQLite3::close - Closes the database connection
SQLite3::__construct - Instantiates an SQLite3 object and opens an SQLite 3 database
SQLite3::createAggregate - Registers a PHP function for use as an SQL aggregate function
SQLite3::createFunction - Registers a PHP function for use as an SQL scalar function
SQLite3::escapeString - Returns a string that has been properly escaped
SQLite3::exec - Executes a result-less query against a given database
SQLite3::lastErrorCode - Returns the numeric result code of the most recent failed SQLite request
SQLite3::lastErrorMsg - Returns English text describing the most recent failed SQLite request
SQLite3::lastInsertRowID - Returns the row ID of the most recent INSERT into the database
SQLite3::loadExtension - Attempts to load an SQLite extension library
SQLite3::open - Opens an SQLite database
SQLite3::prepare - Prepares an SQL statement for execution
SQLite3::query - Executes an SQL query
SQLite3::querySingle - Executes a query and returns a single result
SQLite3::version - Returns the SQLite3 library version as a string constant and as a number
SQLite3Stmt::bindParam - Binds a parameter to a statement variable
SQLite3Stmt::bindValue - Binds the value of a parameter to a statement variable
SQLite3Stmt::clear - Clears all current bound parameters
SQLite3Stmt::close - Closes the prepared statement
SQLite3Stmt::execute - Executes a prepared statement and returns a result set object
SQLite3Stmt::paramCount - Returns the number of parameters within the prepared statement
SQLite3Stmt::reset - Resets the prepared statement
SQLite3Result::columnName - Returns the name of the nth column
SQLite3Result::columnType - Returns the type of the nth column
SQLite3Result::fetchArray - Fetches a result row as an associative or numerically indexed array or both
SQLite3Result::finalize - Closes the result set
SQLite3Result::numColumns - Returns the number of columns in the result set
SQLite3Result::reset - Resets the result set back to the first row
Secure Shell2
ssh2_auth_hostbased_file - Authenticate using a public hostkey
ssh2_auth_none - Authenticate as "none"
ssh2_auth_password - Authenticate over SSH using a plain password
ssh2_auth_pubkey_file - Authenticate using a public key
ssh2_connect - Connect to an SSH server
ssh2_exec - Execute a command on a remote server
ssh2_fetch_stream - Fetch an extended data stream
ssh2_fingerprint - Retrieve fingerprint of remote server
ssh2_methods_negotiated - Return list of negotiated methods
ssh2_publickey_add - Add an authorized publickey
ssh2_publickey_init - Initialize Publickey subsystem
ssh2_publickey_list - List currently authorized publickeys
ssh2_publickey_remove - Remove an authorized publickey
ssh2_scp_recv - Request a file via SCP
ssh2_scp_send - Send a file via SCP
ssh2_sftp_lstat - Stat a symbolic link
ssh2_sftp_mkdir - Create a directory
ssh2_sftp_readlink - Return the target of a symbolic link
ssh2_sftp_realpath - Resolve the realpath of a provided path string
ssh2_sftp_rename - Rename a remote file
ssh2_sftp_rmdir - Remove a directory
ssh2_sftp_stat - Stat a file on a remote filesystem
ssh2_sftp_symlink - Create a symlink
ssh2_sftp_unlink - Delete a file
ssh2_sftp - Initialize SFTP subsystem
ssh2_shell - Request an interactive shell
ssh2_tunnel - Open a tunnel through a remote server
Statistics
stats_absolute_deviation - Returns the absolute deviation of an array of values
stats_cdf_beta - CDF function for BETA Distribution. Calculates any one parameter of the beta distribution given values for the others.
stats_cdf_binomial - Calculates any one parameter of the binomial distribution given values for the others.
stats_cdf_cauchy - Not documented
stats_cdf_chisquare - Calculates any one parameter of the chi-square distribution given values for the others.
stats_cdf_exponential - Not documented
stats_cdf_f - Calculates any one parameter of the F distribution given values for the others.
stats_cdf_gamma - Calculates any one parameter of the gamma distribution given values for the others.
stats_cdf_laplace - Not documented
stats_cdf_logistic - Not documented
stats_cdf_negative_binomial - Calculates any one parameter of the negative binomial distribution given values for the others.
stats_cdf_noncentral_chisquare - Calculates any one parameter of the non-central chi-square distribution given values for the others.
stats_cdf_noncentral_f - Calculates any one parameter of the Non-central F distribution given values for the others.
stats_cdf_poisson - Calculates any one parameter of the Poisson distribution given values for the others.
stats_cdf_t - Calculates any one parameter of the T distribution given values for the others.
stats_cdf_uniform - Not documented
stats_cdf_weibull - Not documented
stats_covariance - Computes the covariance of two data sets
stats_den_uniform - Not documented
stats_dens_beta - Not documented
stats_dens_cauchy - Not documented
stats_dens_chisquare - Not documented
stats_dens_exponential - Not documented
stats_dens_f - Description
stats_dens_gamma - Not documented
stats_dens_laplace - Not documented
stats_dens_logistic - Not documented
stats_dens_negative_binomial - Not documented
stats_dens_normal - Not documented
stats_dens_pmf_binomial - Not documented
stats_dens_pmf_hypergeometric - Description
stats_dens_pmf_poisson - Not documented
stats_dens_t - Not documented
stats_dens_weibull - Not documented
stats_harmonic_mean - Returns the harmonic mean of an array of values
stats_kurtosis - Computes the kurtosis of the data in the array
stats_rand_gen_beta - Generates beta random deviate
stats_rand_gen_chisquare - Generates random deviate from the distribution of a chisquare with "df" degrees of freedom random variable.
stats_rand_gen_exponential - Generates a single random deviate from an exponential distribution with mean "av"
stats_rand_gen_f - Generates a random deviate
stats_rand_gen_funiform - Generates uniform float between low (exclusive) and high (exclusive)
stats_rand_gen_gamma - Generates random deviates from a gamma distribution
stats_rand_gen_ibinomial_negative - Generates a single random deviate from a negative binomial distribution. Arguments : n - the number of trials in the negative binomial distribution from which a random deviate is to be generated (n > 0), p - the probability of an event (0 < p < 1)).
stats_rand_gen_ibinomial - Generates a single random deviate from a binomial distribution whose number of trials is "n" (n >= 0) and whose probability of an event in each trial is "pp" ([0;1]). Method : algorithm BTPE
stats_rand_gen_int - Generates random integer between 1 and 2147483562
stats_rand_gen_ipoisson - Generates a single random deviate from a Poisson distribution with mean "mu" (mu >= 0.0).
stats_rand_gen_iuniform - Generates integer uniformly distributed between LOW (inclusive) and HIGH (inclusive)
stats_rand_gen_noncenral_chisquare - Generates random deviate from the distribution of a noncentral chisquare with "df" degrees of freedom and noncentrality parameter "xnonc". d must be >= 1.0, xnonc must >= 0.0
stats_rand_gen_noncentral_f - Generates a random deviate from the noncentral F (variance ratio) distribution with "dfn" degrees of freedom in the numerator, and "dfd" degrees of freedom in the denominator, and noncentrality parameter "xnonc". Method : directly generates ratio of noncentral numerator chisquare variate to central denominator chisquare variate.
stats_rand_gen_noncentral_t - Generates a single random deviate from a noncentral T distribution
stats_rand_gen_normal - Generates a single random deviate from a normal distribution with mean, av, and standard deviation, sd (sd >= 0). Method : Renames SNORM from TOMS as slightly modified by BWB to use RANF instead of SUNIF.
stats_rand_gen_t - Generates a single random deviate from a T distribution
stats_rand_get_seeds - Not documented
stats_rand_phrase_to_seeds - generate two seeds for the RGN random number generator
stats_rand_ranf - Returns a random floating point number from a uniform distribution over 0 - 1 (endpoints of this interval are not returned) using the current generator
stats_rand_setall - Not documented
stats_skew - Computes the skewness of the data in the array
stats_standard_deviation - Returns the standard deviation
stats_stat_binomial_coef - Not documented
stats_stat_correlation - Not documented
stats_stat_gennch - Not documented
stats_stat_independent_t - Not documented
stats_stat_innerproduct - Description
stats_stat_noncentral_t - Calculates any one parameter of the noncentral t distribution give values for the others.
stats_stat_paired_t - Not documented
stats_stat_percentile - Not documented
stats_stat_powersum - Not documented
stats_variance - Returns the population variance
Stomp Client
stomp_connect_error - Returns a string description of the last connect error
stomp_version - Gets the current stomp extension version
Stomp::abort - Rolls back a transaction in progress
Stomp::ack - Acknowledges consumption of a message
Stomp::begin - Starts a transaction
Stomp::commit - Commits a transaction in progress
Stomp::__construct - Opens a connection
Stomp::__destruct - Closes stomp connection
Stomp::error - Gets the last stomp error
Stomp::getReadTimeout - Gets read timeout
Stomp::getSessionId - Gets the current stomp session ID
Stomp::hasFrame - Indicates whether or not there is a frame ready to read
Stomp::readFrame - Reads the next frame
Stomp::send - Sends a message
Stomp::setReadTimeout - Sets read timeout
Stomp::subscribe - Registers to listen to a given destination
Stomp::unsubscribe - Removes an existing subscription
StompFrame::__construct - Constructor
Streams
streamWrapper::__construct - Constructs a new stream wrapper
streamWrapper::dir_closedir - Close directory handle
streamWrapper::dir_opendir - Open directory handle
streamWrapper::dir_readdir - Read entry from directory handle
streamWrapper::dir_rewinddir - Rewind directory handle
streamWrapper::mkdir - Create a directory
streamWrapper::rename - Renames a file or directory
streamWrapper::rmdir - Removes a directory
streamWrapper::stream_cast - Retrieve the underlaying resource
streamWrapper::stream_close - Close an resource
streamWrapper::stream_eof - Tests for end-of-file on a file pointer
streamWrapper::stream_flush - Flushes the output
streamWrapper::stream_lock - Advisory file locking
streamWrapper::stream_open - Opens file or URL
streamWrapper::stream_read - Read from stream
streamWrapper::stream_seek - Seeks to specific location in a stream
streamWrapper::stream_set_option - Change stream options
streamWrapper::stream_stat - Retrieve information about a file resource
streamWrapper::stream_tell - Retrieve the current position of a stream
streamWrapper::stream_write - Write to stream
streamWrapper::unlink - Delete a file
streamWrapper::url_stat - Retrieve information about a file
set_socket_blocking - Alias of stream_set_blocking
stream_bucket_append - Append bucket to brigade
stream_bucket_make_writeable - Return a bucket object from the brigade for operating on
stream_bucket_new - Create a new bucket for use on the current stream
stream_bucket_prepend - Prepend bucket to brigade
stream_context_create - Create a streams context
stream_context_get_default - Retreive the default streams context
stream_context_get_options - Retrieve options for a stream/wrapper/context
stream_context_get_params - Retrieves parameters from a context
stream_context_set_default - Set the default streams context
stream_context_set_option - Sets an option for a stream/wrapper/context
stream_context_set_params - Set parameters for a stream/wrapper/context
stream_copy_to_stream - Copies data from one stream to another
stream_encoding - Set character set for stream encoding
stream_filter_append - Attach a filter to a stream
stream_filter_prepend - Attach a filter to a stream
stream_filter_register - Register a user defined stream filter
stream_filter_remove - Remove a filter from a stream
stream_get_contents - Reads remainder of a stream into a string
stream_get_filters - Retrieve list of registered filters
stream_get_line - Gets line from stream resource up to a given delimiter
stream_get_meta_data - Retrieves header/meta data from streams/file pointers
stream_get_transports - Retrieve list of registered socket transports
stream_get_wrappers - Retrieve list of registered streams
stream_is_local - Checks if a stream is a local stream
stream_notification_callback - A callback function for the notification context paramater
stream_register_wrapper - Alias of stream_wrapper_register
stream_resolve_include_path - Resolve filename against the include path
stream_select - Runs the equivalent of the select() system call on the given
arrays of streams with a timeout specified by tv_sec and tv_usec
stream_set_blocking - Set blocking/non-blocking mode on a stream
stream_set_read_buffer - Set read file buffering on the given stream
stream_set_timeout - Set timeout period on a stream
stream_set_write_buffer - Sets write file buffering on the given stream
stream_socket_accept - Accept a connection on a socket created by stream_socket_server
stream_socket_client - Open Internet or Unix domain socket connection
stream_socket_enable_crypto - Turns encryption on/off on an already connected socket
stream_socket_get_name - Retrieve the name of the local or remote sockets
stream_socket_pair - Creates a pair of connected, indistinguishable socket streams
stream_socket_recvfrom - Receives data from a socket, connected or not
stream_socket_sendto - Sends a message to a socket, whether it is connected or not
stream_socket_server - Create an Internet or Unix domain server socket
stream_socket_shutdown - Shutdown a full-duplex connection
stream_supports_lock - Tells whether the stream supports locking.
stream_wrapper_register - Register a URL wrapper implemented as a PHP class
stream_wrapper_restore - Restores a previously unregistered built-in wrapper
stream_wrapper_unregister - Unregister a URL wrapper
Strings
addcslashes - Quote string with slashes in a C style
addslashes - Quote string with slashes
bin2hex - Convert binary data into hexadecimal representation
chop - Alias of rtrim
chr - Return a specific character
chunk_split - Split a string into smaller chunks
convert_cyr_string - Convert from one Cyrillic character set to another
convert_uudecode - Decode a uuencoded string
convert_uuencode - Uuencode a string
count_chars - Return information about characters used in a string
crc32 - Calculates the crc32 polynomial of a string
crypt - One-way string hashing
echo - Output one or more strings
explode - Split a string by string
fprintf - Write a formatted string to a stream
get_html_translation_table - Returns the translation table used by htmlspecialchars and htmlentities
hebrev - Convert logical Hebrew text to visual text
hebrevc - Convert logical Hebrew text to visual text with newline conversion
html_entity_decode - Convert all HTML entities to their applicable characters
htmlentities - Convert all applicable characters to HTML entities
htmlspecialchars_decode - Convert special HTML entities back to characters
htmlspecialchars - Convert special characters to HTML entities
implode - Join array elements with a string
join - Alias of implode
lcfirst - Make a string's first character lowercase
levenshtein - Calculate Levenshtein distance between two strings
localeconv - Get numeric formatting information
ltrim - Strip whitespace (or other characters) from the beginning of a string
md5_file - Calculates the md5 hash of a given file
md5 - Calculate the md5 hash of a string
metaphone - Calculate the metaphone key of a string
money_format - Formats a number as a currency string
nl_langinfo - Query language and locale information
nl2br - Inserts HTML line breaks before all newlines in a string
number_format - Format a number with grouped thousands
ord - Return ASCII value of character
parse_str - Parses the string into variables
print - Output a string
printf - Output a formatted string
quoted_printable_decode - Convert a quoted-printable string to an 8 bit string
quoted_printable_encode - Convert a 8 bit string to a quoted-printable string
quotemeta - Quote meta characters
rtrim - Strip whitespace (or other characters) from the end of a string
setlocale - Set locale information
sha1_file - Calculate the sha1 hash of a file
sha1 - Calculate the sha1 hash of a string
similar_text - Calculate the similarity between two strings
soundex - Calculate the soundex key of a string
sprintf - Return a formatted string
sscanf - Parses input from a string according to a format
str_getcsv - Parse a CSV string into an array
str_ireplace - Case-insensitive version of str_replace.
str_pad - Pad a string to a certain length with another string
str_repeat - Repeat a string
str_replace - Replace all occurrences of the search string with the replacement string
str_rot13 - Perform the rot13 transform on a string
str_shuffle - Randomly shuffles a string
str_split - Convert a string to an array
str_word_count - Return information about words used in a string
strcasecmp - Binary safe case-insensitive string comparison
strchr - Alias of strstr
strcmp - Binary safe string comparison
strcoll - Locale based string comparison
strcspn - Find length of initial segment not matching mask
strip_tags - Strip HTML and PHP tags from a string
stripcslashes - Un-quote string quoted with addcslashes
stripos - Find position of first occurrence of a case-insensitive string
stripslashes - Un-quotes a quoted string
stristr - Case-insensitive strstr
strlen - Get string length
strnatcasecmp - Case insensitive string comparisons using a "natural order" algorithm
strnatcmp - String comparisons using a "natural order" algorithm
strncasecmp - Binary safe case-insensitive string comparison of the first n characters
strncmp - Binary safe string comparison of the first n characters
strpbrk - Search a string for any of a set of characters
strpos - Find position of first occurrence of a string
strrchr - Find the last occurrence of a character in a string
strrev - Reverse a string
strripos - Find position of last occurrence of a case-insensitive string in a string
strrpos - Find position of last occurrence of a char in a string
strspn - Finds the length of the first segment of a string consisting
entirely of characters contained within a given mask.
strstr - Find first occurrence of a string
strtok - Tokenize string
strtolower - Make a string lowercase
strtoupper - Make a string uppercase
strtr - Translate certain characters
substr_compare - Binary safe comparison of two strings from an offset, up to length characters
substr_count - Count the number of substring occurrences
substr_replace - Replace text within a portion of a string
substr - Return part of a string
trim - Strip whitespace (or other characters) from the beginning and end of a string
ucfirst - Make a string's first character uppercase
ucwords - Uppercase the first character of each word in a string
vfprintf - Write a formatted string to a stream
vprintf - Output a formatted string
vsprintf - Return a formatted string
wordwrap - Wraps a string to a given number of characters
Subversion
svn_add - Schedules the addition of an item in a working directory
svn_auth_get_parameter - Retrieves authentication parameter
svn_auth_set_parameter - Sets an authentication parameter
svn_blame - Get the SVN blame for a file
svn_cat - Returns the contents of a file in a repository
svn_checkout - Checks out a working copy from the repository
svn_cleanup - Recursively cleanup a working copy directory, finishing incomplete operations and removing locks
svn_client_version - Returns the version of the SVN client libraries
svn_commit - Sends changes from the local working copy to the repository
svn_delete - Delete items from a working copy or repository.
svn_diff - Recursively diffs two paths
svn_export - Export the contents of a SVN directory
svn_fs_abort_txn - Abort a transaction, returns true if everything is okay, false otherwise
svn_fs_apply_text - Creates and returns a stream that will be used to replace
svn_fs_begin_txn2 - Create a new transaction
svn_fs_change_node_prop - Return true if everything is ok, false otherwise
svn_fs_check_path - Determines what kind of item lives at path in a given repository fsroot
svn_fs_contents_changed - Return true if content is different, false otherwise
svn_fs_copy - Copies a file or a directory, returns true if all is ok, false otherwise
svn_fs_delete - Deletes a file or a directory, return true if all is ok, false otherwise
svn_fs_dir_entries - Enumerates the directory entries under path; returns a hash of dir names to file type
svn_fs_file_contents - Returns a stream to access the contents of a file from a given version of the fs
svn_fs_file_length - Returns the length of a file from a given version of the fs
svn_fs_is_dir - Return true if the path points to a directory, false otherwise
svn_fs_is_file - Return true if the path points to a file, false otherwise
svn_fs_make_dir - Creates a new empty directory, returns true if all is ok, false otherwise
svn_fs_make_file - Creates a new empty file, returns true if all is ok, false otherwise
svn_fs_node_created_rev - Returns the revision in which path under fsroot was created
svn_fs_node_prop - Returns the value of a property for a node
svn_fs_props_changed - Return true if props are different, false otherwise
svn_fs_revision_prop - Fetches the value of a named property
svn_fs_revision_root - Get a handle on a specific version of the repository root
svn_fs_txn_root - Creates and returns a transaction root
svn_fs_youngest_rev - Returns the number of the youngest revision in the filesystem
svn_import - Imports an unversioned path into a repository
svn_log - Returns the commit log messages of a repository URL
svn_ls - Returns list of directory contents in repository URL, optionally at revision number
svn_mkdir - Creates a directory in a working copy or repository
svn_repos_create - Create a new subversion repository at path
svn_repos_fs_begin_txn_for_commit - Create a new transaction
svn_repos_fs_commit_txn - Commits a transaction and returns the new revision
svn_repos_fs - Gets a handle on the filesystem for a repository
svn_repos_hotcopy - Make a hot-copy of the repos at repospath; copy it to destpath
svn_repos_open - Open a shared lock on a repository.
svn_repos_recover - Run recovery procedures on the repository located at path.
svn_revert - Revert changes to the working copy
svn_status - Returns the status of working copy files and directories
svn_update - Update working copy
Shockwave Flash
swf_actiongeturl - Get a URL from a Shockwave Flash movie
swf_actiongotoframe - Play a frame and then stop
swf_actiongotolabel - Display a frame with the specified label
swf_actionnextframe - Go forward one frame
swf_actionplay - Start playing the flash movie from the current frame
swf_actionprevframe - Go backwards one frame
swf_actionsettarget - Set the context for actions
swf_actionstop - Stop playing the flash movie at the current frame
swf_actiontogglequality - Toggle between low and high quality
swf_actionwaitforframe - Skip actions if a frame has not been loaded
swf_addbuttonrecord - Controls location, appearance and active area of the current button
swf_addcolor - Set the global add color to the rgba value specified
swf_closefile - Close the current Shockwave Flash file
swf_definebitmap - Define a bitmap
swf_definefont - Defines a font
swf_defineline - Define a line
swf_definepoly - Define a polygon
swf_definerect - Define a rectangle
swf_definetext - Define a text string
swf_endbutton - End the definition of the current button
swf_enddoaction - End the current action
swf_endshape - Completes the definition of the current shape
swf_endsymbol - End the definition of a symbol
swf_fontsize - Change the font size
swf_fontslant - Set the font slant
swf_fonttracking - Set the current font tracking
swf_getbitmapinfo - Get information about a bitmap
swf_getfontinfo - Gets font information
swf_getframe - Get the frame number of the current frame
swf_labelframe - Label the current frame
swf_lookat - Define a viewing transformation
swf_modifyobject - Modify an object
swf_mulcolor - Sets the global multiply color to the rgba value specified
swf_nextid - Returns the next free object id
swf_oncondition - Describe a transition used to trigger an action list
swf_openfile - Open a new Shockwave Flash file
swf_ortho2 - Defines 2D orthographic mapping of user coordinates onto the current viewport
swf_ortho - Defines an orthographic mapping of user coordinates onto the current viewport
swf_perspective - Define a perspective projection transformation
swf_placeobject - Place an object onto the screen
swf_polarview - Define the viewer's position with polar coordinates
swf_popmatrix - Restore a previous transformation matrix
swf_posround - Enables or Disables the rounding of the translation when objects are placed or moved
swf_pushmatrix - Push the current transformation matrix back unto the stack
swf_removeobject - Remove an object
swf_rotate - Rotate the current transformation
swf_scale - Scale the current transformation
swf_setfont - Change the current font
swf_setframe - Switch to a specified frame
swf_shapearc - Draw a circular arc
swf_shapecurveto3 - Draw a cubic bezier curve
swf_shapecurveto - Draw a quadratic bezier curve between two points
swf_shapefillbitmapclip - Set current fill mode to clipped bitmap
swf_shapefillbitmaptile - Set current fill mode to tiled bitmap
swf_shapefilloff - Turns off filling
swf_shapefillsolid - Set the current fill style to the specified color
swf_shapelinesolid - Set the current line style
swf_shapelineto - Draw a line
swf_shapemoveto - Move the current position
swf_showframe - Display the current frame
swf_startbutton - Start the definition of a button
swf_startdoaction - Start a description of an action list for the current frame
swf_startshape - Start a complex shape
swf_startsymbol - Define a symbol
swf_textwidth - Get the width of a string
swf_translate - Translate the current transformations
swf_viewport - Select an area for future drawing
Swish Indexing
Swish::__construct - Construct a Swish object
Swish->getMetaList - Get the list of meta entries for the index
Swish->getPropertyList - Get the list of properties for the index
Swish->prepare - Prepare a search query
Swish->query - Execute a query and return results object
SwishResult->getMetaList - Get a list of meta entries
SwishResult->stem - Stems the given word
SwishResults->getParsedWords - Get an array of parsed words
SwishResults->getRemovedStopwords - Get an array of stopwords removed from the query
SwishResults->nextResult - Get the next search result
SwishResults->seekResult - Set current seek pointer to the given position
SwishSearch->execute - Execute the search and get the results
SwishSearch->resetLimit - Reset the search limits
SwishSearch->setLimit - Set the search limits
SwishSearch->setPhraseDelimiter - Set the phrase delimiter
SwishSearch->setSort - Set the sort order
SwishSearch->setStructure - Set the structure flag in the search object
Sybase
sybase_affected_rows - Gets number of affected rows in last query
sybase_close - Closes a Sybase connection
sybase_connect - Opens a Sybase server connection
sybase_data_seek - Moves internal row pointer
sybase_deadlock_retry_count - Sets the deadlock retry count
sybase_fetch_array - Fetch row as array
sybase_fetch_assoc - Fetch a result row as an associative array
sybase_fetch_field - Get field information from a result
sybase_fetch_object - Fetch a row as an object
sybase_fetch_row - Get a result row as an enumerated array
sybase_field_seek - Sets field offset
sybase_free_result - Frees result memory
sybase_get_last_message - Returns the last message from the server
sybase_min_client_severity - Sets minimum client severity
sybase_min_error_severity - Sets minimum error severity
sybase_min_message_severity - Sets minimum message severity
sybase_min_server_severity - Sets minimum server severity
sybase_num_fields - Gets the number of fields in a result set
sybase_num_rows - Get number of rows in a result set
sybase_pconnect - Open persistent Sybase connection
sybase_query - Sends a Sybase query
sybase_result - Get result data
sybase_select_db - Selects a Sybase database
sybase_set_message_handler - Sets the handler called when a server message is raised
sybase_unbuffered_query - Send a Sybase query and do not block
TCP Wrappers
tcpwrap_check - Performs a tcpwrap check
Tidy
tidy::body - Returns a tidyNode object starting from the <body> tag of the tidy parse tree
tidy::cleanRepair - Execute configured cleanup and repair operations on parsed markup
tidy::__construct - Constructs a new tidy object
tidy::diagnose - Run configured diagnostics on parsed and repaired markup
tidy::getConfig - Get current Tidy configuration
tidy::htmlver - Get the Detected HTML version for the specified document
tidy::getOpt - Returns the value of the specified configuration option for the tidy document
tidy::getoptdoc - Returns the documentation for the given option name
tidy::getRelease - Get release date (version) for Tidy library
tidy::getStatus - Get status of specified document
tidy::head - Returns a tidyNode object starting from the <head> tag of the tidy parse tree
tidy::html - Returns a tidyNode object starting from the <html> tag of the tidy parse tree
tidy::isXhtml - Indicates if the document is a XHTML document
tidy::isXml - Indicates if the document is a generic (non HTML/XHTML) XML document
tidy::parseFile - Parse markup in file or URI
tidy::parseString - Parse a document stored in a string
tidy::repairFile - Repair a file and return it as a string
tidy::repairString - Repair a string using an optionally provided configuration file
tidy::root - Returns a tidyNode object representing the root of the tidy parse tree
tidyNode::getParent - Returns the parent node of the current node
tidyNode::hasChildren - Checks if a node has children
tidyNode::hasSiblings - Checks if a node has siblings
tidyNode::isAsp - Checks if this node is ASP
tidyNode::isComment - Checks if a node represents a comment
tidyNode::isHtml - Checks if a node is part of a HTML document
tidyNode::isJste - Checks if this node is JSTE
tidyNode::isPhp - Checks if a node is PHP
tidyNode::isText - Checks if a node represents text (no markup)
ob_tidyhandler - ob_start callback function to repair the buffer
tidy_access_count - Returns the Number of Tidy accessibility warnings encountered for specified document
tidy_config_count - Returns the Number of Tidy configuration errors encountered for specified document
tidy_error_count - Returns the Number of Tidy errors encountered for specified document
tidy_get_error_buffer - Return warnings and errors which occurred parsing the specified document
tidy_get_output - Return a string representing the parsed tidy markup
tidy_load_config - Load an ASCII Tidy configuration file with the specified encoding
tidy_reset_config - Restore Tidy configuration to default values
tidy_save_config - Save current settings to named file
tidy_set_encoding - Set the input/output character encoding for parsing markup
tidy_setopt - Updates the configuration settings for the specified tidy document
tidy_warning_count - Returns the Number of Tidy warnings encountered for specified document
Tokenizer
token_get_all - Split given source into PHP tokens
token_name - Get the symbolic name of a given PHP token
tokyo_tyrant
TokyoTyrant::add - Adds to a numeric key
TokyoTyrant::connect - Connect to a database
TokyoTyrant::connectUri - Connects to a database
TokyoTyrant::__construct - Construct a new TokyoTyrant object
TokyoTyrant::copy - Copies the database
TokyoTyrant::ext - Execute a remote script
TokyoTyrant::fwmKeys - Returns the forward matching keys
TokyoTyrant::get - The get purpose
TokyoTyrant::getIterator - Get an iterator
TokyoTyrant::num - Number of records in the database
TokyoTyrant::out - Removes records
TokyoTyrant::put - Puts values
TokyoTyrant::putCat - Concatenates to a record
TokyoTyrant::putKeep - Puts a record
TokyoTyrant::putNr - Puts value
TokyoTyrant::putShl - Concatenates to a record
TokyoTyrant::restore - Restore the database
TokyoTyrant::setMaster - Set the replication master
TokyoTyrant::size - Returns the size of the value
TokyoTyrant::stat - Get statistics
TokyoTyrant::sync - Synchronize the database
TokyoTyrant::tune - Tunes connection values
TokyoTyrant::vanish - Empties the database
TokyoTyrantTable::add - Adds a record
TokyoTyrantTable::genUid - Generate unique id
TokyoTyrantTable::get - Get a row
TokyoTyrantTable::getIterator - Get an iterator
TokyoTyrantTable::getQuery - Get a query object
TokyoTyrantTable::out - Remove records
TokyoTyrantTable::put - Store a row
TokyoTyrantTable::putCat - Concatenates to a row
TokyoTyrantTable::putKeep - Put a new record
TokyoTyrantTable::putNr - Puts value
TokyoTyrantTable::putShl - Concatenates to a record
TokyoTyrantTable::setIndex - Sets index
TokyoTyrantQuery::addCond - Adds a condition to the query
TokyoTyrantQuery::__construct - Construct a new query
TokyoTyrantQuery::count - Counts records
TokyoTyrantQuery::current - Returns the current element
TokyoTyrantQuery::hint - Get the hint string of the query
TokyoTyrantQuery::key - Returns the current key
TokyoTyrantQuery::metaSearch - Retrieve records with multiple queries
TokyoTyrantQuery::next - Moves the iterator to next entry
TokyoTyrantQuery::out - Removes records based on query
TokyoTyrantQuery::rewind - Rewinds the iterator
TokyoTyrantQuery::search - Searches records
TokyoTyrantQuery::setLimit - Limit results
TokyoTyrantQuery::valid - Checks the validity of current item
ODBC (Unified)
odbc_autocommit - Toggle autocommit behaviour
odbc_binmode - Handling of binary column data
odbc_close_all - Close all ODBC connections
odbc_close - Close an ODBC connection
odbc_columnprivileges - Lists columns and associated privileges for the given table
odbc_columns - Lists the column names in specified tables
odbc_commit - Commit an ODBC transaction
odbc_connect - Connect to a datasource
odbc_cursor - Get cursorname
odbc_data_source - Returns information about a current connection
odbc_do - Alias of odbc_exec
odbc_error - Get the last error code
odbc_errormsg - Get the last error message
odbc_exec - Prepare and execute an SQL statement
odbc_execute - Execute a prepared statement
odbc_fetch_array - Fetch a result row as an associative array
odbc_fetch_into - Fetch one result row into array
odbc_fetch_object - Fetch a result row as an object
odbc_fetch_row - Fetch a row
odbc_field_len - Get the length (precision) of a field
odbc_field_name - Get the columnname
odbc_field_num - Return column number
odbc_field_precision - Alias of odbc_field_len
odbc_field_scale - Get the scale of a field
odbc_field_type - Datatype of a field
odbc_foreignkeys - Retrieves a list of foreign keys
odbc_free_result - Free resources associated with a result
odbc_gettypeinfo - Retrieves information about data types supported by the data source
odbc_longreadlen - Handling of LONG columns
odbc_next_result - Checks if multiple results are available
odbc_num_fields - Number of columns in a result
odbc_num_rows - Number of rows in a result
odbc_pconnect - Open a persistent database connection
odbc_prepare - Prepares a statement for execution
odbc_primarykeys - Gets the primary keys for a table
odbc_procedurecolumns - Retrieve information about parameters to procedures
odbc_procedures - Get the list of procedures stored in a specific data source
odbc_result_all - Print result as HTML table
odbc_result - Get result data
odbc_rollback - Rollback a transaction
odbc_setoption - Adjust ODBC settings
odbc_specialcolumns - Retrieves special columns
odbc_statistics - Retrieve statistics about a table
odbc_tableprivileges - Lists tables and the privileges associated with each table
odbc_tables - Get the list of table names stored in a specific data source
URLs
base64_decode - Decodes data encoded with MIME base64
base64_encode - Encodes data with MIME base64
get_headers - Fetches all the headers sent by the server in response to a HTTP request
get_meta_tags - Extracts all meta tag content attributes from a file and returns an array
http_build_query - Generate URL-encoded query string
parse_url - Parse a URL and return its components
rawurldecode - Decode URL-encoded strings
rawurlencode - URL-encode according to RFC 1738
urldecode - Decodes URL-encoded string
urlencode - URL-encodes string
Variable handling
debug_zval_dump - Dumps a string representation of an internal zend value to output
doubleval - Alias of floatval
empty - Determine whether a variable is empty
floatval - Get float value of a variable
get_defined_vars - Returns an array of all defined variables
get_resource_type - Returns the resource type
gettype - Get the type of a variable
import_request_variables - Import GET/POST/Cookie variables into the global scope
intval - Get the integer value of a variable
is_array - Finds whether a variable is an array
is_bool - Finds out whether a variable is a boolean
is_callable - Verify that the contents of a variable can be called as a function
is_double - Alias of is_float
is_float - Finds whether the type of a variable is float
is_int - Find whether the type of a variable is integer
is_integer - Alias of is_int
is_long - Alias of is_int
is_null - Finds whether a variable is NULL
is_numeric - Finds whether a variable is a number or a numeric string
is_object - Finds whether a variable is an object
is_real - Alias of is_float
is_resource - Finds whether a variable is a resource
is_scalar - Finds whether a variable is a scalar
is_string - Find whether the type of a variable is string
isset - Determine if a variable is set and is not NULL
print_r - Prints human-readable information about a variable
serialize - Generates a storable representation of a value
settype - Set the type of a variable
strval - Get string value of a variable
unserialize - Creates a PHP value from a stored representation
unset - Unset a given variable
var_dump - Dumps information about a variable
var_export - Outputs or returns a parsable string representation of a variable
vpopmail
vpopmail_add_alias_domain_ex - Add alias to an existing virtual domain
vpopmail_add_alias_domain - Add an alias for a virtual domain
vpopmail_add_domain_ex - Add a new virtual domain
vpopmail_add_domain - Add a new virtual domain
vpopmail_add_user - Add a new user to the specified virtual domain
vpopmail_alias_add - Insert a virtual alias
vpopmail_alias_del_domain - Deletes all virtual aliases of a domain
vpopmail_alias_del - Deletes all virtual aliases of a user
vpopmail_alias_get_all - Get all lines of an alias for a domain
vpopmail_alias_get - Get all lines of an alias for a domain
vpopmail_auth_user - Attempt to validate a username/domain/password
vpopmail_del_domain_ex - Delete a virtual domain
vpopmail_del_domain - Delete a virtual domain
vpopmail_del_user - Delete a user from a virtual domain
vpopmail_error - Get text message for last vpopmail error
vpopmail_passwd - Change a virtual user's password
vpopmail_set_user_quota - Sets a virtual user's quota
W32api
w32api_deftype - Defines a type for use with other w32api_functions
w32api_init_dtype - Creates an instance of the data type typename and fills it with the values passed
w32api_invoke_function - Invokes function funcname with the arguments passed after the function name
w32api_register_function - Registers function function_name from library with PHP
w32api_set_call_method - Sets the calling method used
WDDX
wddx_add_vars - Add variables to a WDDX packet with the specified ID
wddx_deserialize - Alias of wddx_unserialize
wddx_packet_end - Ends a WDDX packet with the specified ID
wddx_packet_start - Starts a new WDDX packet with structure inside it
wddx_serialize_value - Serialize a single value into a WDDX packet
wddx_serialize_vars - Serialize variables into a WDDX packet
wddx_unserialize - Unserializes a WDDX packet
win32ps
win32_ps_list_procs - List running processes
win32_ps_stat_mem - Stat memory utilization
win32_ps_stat_proc - Stat process
win32service
win32_create_service - Creates a new service entry in the SCM database
win32_delete_service - Deletes a service entry from the SCM database
win32_get_last_control_message - Returns the last control message that was sent to this service
win32_query_service_status - Queries the status of a service
win32_set_service_status - Update the service status
win32_start_service_ctrl_dispatcher - Registers the script with the SCM, so that it can act as the service with the given name
win32_start_service - Starts a service
win32_stop_service - Stops a service
Windows Cache for PHP
wincache_fcache_fileinfo - Retrieves information about files cached in the file cache
wincache_fcache_meminfo - Retrieves information about file cache memory usage
wincache_lock - Acquires an exclusive lock on a given key
wincache_ocache_fileinfo - Retrieves information about files cached in the opcode cache
wincache_ocache_meminfo - Retrieves information about opcode cache memory usage
wincache_refresh_if_changed - Refreshes the cache entries for the cached files
wincache_rplist_fileinfo - Retrieves information about resolve file path cache
wincache_rplist_meminfo - Retrieves information about memory usage by the resolve file path cache
wincache_scache_info - Retrieves information about files cached in the session cache
wincache_scache_meminfo - Retrieves information about session cache memory usage
wincache_ucache_add - Adds a variable in user cache only if variable does not already exist in the cache
wincache_ucache_cas - Compares the variable with old value and assigns new value to it
wincache_ucache_clear - Deletes entire content of the user cache
wincache_ucache_dec - Decrements the value associated with the key
wincache_ucache_delete - Deletes variables from the user cache
wincache_ucache_exists - Checks if a variable exists in the user cache
wincache_ucache_get - Gets a variable stored in the user cache
wincache_ucache_inc - Increments the value associated with the key
wincache_ucache_info - Retrieves information about data stored in the user cache
wincache_ucache_meminfo - Retrieves information about user cache memory usage
wincache_ucache_set - Adds a variable in user cache and overwrites a variable if it already exists in the cache
wincache_unlock - Releases an exclusive lock on a given key
xattr
xattr_get - Get an extended attribute
xattr_list - Get a list of extended attributes
xattr_remove - Remove an extended attribute
xattr_set - Set an extended attribute
xattr_supported - Check if filesystem supports extended attributes
xdiff
xdiff_file_bdiff_size - Read a size of file created by applying a binary diff
xdiff_file_bdiff - Make binary diff of two files
xdiff_file_bpatch - Patch a file with a binary diff
xdiff_file_diff_binary - Alias of xdiff_file_bdiff
xdiff_file_diff - Make unified diff of two files
xdiff_file_merge3 - Merge 3 files into one
xdiff_file_patch_binary - Alias of xdiff_file_bpatch
xdiff_file_patch - Patch a file with an unified diff
xdiff_file_rabdiff - Make binary diff of two files using the Rabin's polynomial fingerprinting algorithm
xdiff_string_bdiff_size - Read a size of file created by applying a binary diff
xdiff_string_bdiff - Make binary diff of two strings
xdiff_string_bpatch - Patch a string with a binary diff
xdiff_string_diff_binary - Alias of xdiff_string_bdiff
xdiff_string_diff - Make unified diff of two strings
xdiff_string_merge3 - Merge 3 strings into one
xdiff_string_patch_binary - Alias of xdiff_string_bpatch
xdiff_string_patch - Patch a string with an unified diff
xdiff_string_rabdiff - Make binary diff of two strings using the Rabin's polynomial fingerprinting algorithm
XML Parser
utf8_decode - Converts a string with ISO-8859-1 characters encoded with UTF-8
to single-byte ISO-8859-1
utf8_encode - Encodes an ISO-8859-1 string to UTF-8
xml_error_string - Get XML parser error string
xml_get_current_byte_index - Get current byte index for an XML parser
xml_get_current_column_number - Get current column number for an XML parser
xml_get_current_line_number - Get current line number for an XML parser
xml_get_error_code - Get XML parser error code
xml_parse_into_struct - Parse XML data into an array structure
xml_parse - Start parsing an XML document
xml_parser_create_ns - Create an XML parser with namespace support
xml_parser_create - Create an XML parser
xml_parser_free - Free an XML parser
xml_parser_get_option - Get options from an XML parser
xml_parser_set_option - Set options in an XML parser
xml_set_character_data_handler - Set up character data handler
xml_set_default_handler - Set up default handler
xml_set_element_handler - Set up start and end element handlers
xml_set_end_namespace_decl_handler - Set up end namespace declaration handler
xml_set_external_entity_ref_handler - Set up external entity reference handler
xml_set_notation_decl_handler - Set up notation declaration handler
xml_set_object - Use XML Parser within an object
xml_set_processing_instruction_handler - Set up processing instruction (PI) handler
xml_set_start_namespace_decl_handler - Set up start namespace declaration handler
xml_set_unparsed_entity_decl_handler - Set up unparsed entity declaration handler
XMLReader
XMLReader::close - Close the XMLReader input
XMLReader::expand - Returns a copy of the current node as a DOM object
XMLReader::getAttribute - Get the value of a named attribute
XMLReader::getAttributeNo - Get the value of an attribute by index
XMLReader::getAttributeNs - Get the value of an attribute by localname and URI
XMLReader::getParserProperty - Indicates if specified property has been set
XMLReader::isValid - Indicates if the parsed document is valid
XMLReader::lookupNamespace - Lookup namespace for a prefix
XMLReader::moveToAttribute - Move cursor to a named attribute
XMLReader::moveToAttributeNo - Move cursor to an attribute by index
XMLReader::moveToAttributeNs - Move cursor to a named attribute
XMLReader::moveToElement - Position cursor on the parent Element of current Attribute
XMLReader::moveToFirstAttribute - Position cursor on the first Attribute
XMLReader::moveToNextAttribute - Position cursor on the next Attribute
XMLReader::next - Move cursor to next node skipping all subtrees
XMLReader::open - Set the URI containing the XML to parse
XMLReader::read - Move to next node in document
XMLReader::readInnerXML - Retrieve XML from current node
XMLReader::readOuterXML - Retrieve XML from current node, including it self
XMLReader::readString - Reads the contents of the current node as an string
XMLReader::setParserProperty - Set or Unset parser options
XMLReader::setRelaxNGSchema - Set the filename or URI for a RelaxNG Schema
XMLReader::setRelaxNGSchemaSource - Set the data containing a RelaxNG Schema
XMLReader::setSchema - Validate document against XSD
XMLReader::XML - Set the data containing the XML to parse
XML-RPC
xmlrpc_decode_request - Decodes XML into native PHP types
xmlrpc_decode - Decodes XML into native PHP types
xmlrpc_encode_request - Generates XML for a method request
xmlrpc_encode - Generates XML for a PHP value
xmlrpc_get_type - Gets xmlrpc type for a PHP value
xmlrpc_is_fault - Determines if an array value represents an XMLRPC fault
xmlrpc_parse_method_descriptions - Decodes XML into a list of method descriptions
xmlrpc_server_add_introspection_data - Adds introspection documentation
xmlrpc_server_call_method - Parses XML requests and call methods
xmlrpc_server_create - Creates an xmlrpc server
xmlrpc_server_destroy - Destroys server resources
xmlrpc_server_register_introspection_callback - Register a PHP function to generate documentation
xmlrpc_server_register_method - Register a PHP function to resource method matching method_name
xmlrpc_set_type - Sets xmlrpc type, base64 or datetime, for a PHP string value
XMLWriter
XMLWriter::endAttribute - End attribute
XMLWriter::endCData - End current CDATA
XMLWriter::endComment - Create end comment
XMLWriter::endDocument - End current document
XMLWriter::endDTDAttlist - End current DTD AttList
XMLWriter::endDTDElement - End current DTD element
XMLWriter::endDTDEntity - End current DTD Entity
XMLWriter::endDTD - End current DTD
XMLWriter::endElement - End current element
XMLWriter::endPI - End current PI
XMLWriter::flush - Flush current buffer
XMLWriter::fullEndElement - End current element
XMLWriter::openMemory - Create new xmlwriter using memory for string output
XMLWriter::openURI - Create new xmlwriter using source uri for output
XMLWriter::outputMemory - Returns current buffer
XMLWriter::setIndentString - Set string used for indenting
XMLWriter::setIndent - Toggle indentation on/off
XMLWriter::startAttributeNS - Create start namespaced attribute
XMLWriter::startAttribute - Create start attribute
XMLWriter::startCData - Create start CDATA tag
XMLWriter::startComment - Create start comment
XMLWriter::startDocument - Create document tag
XMLWriter::startDTDAttlist - Create start DTD AttList
XMLWriter::startDTDElement - Create start DTD element
XMLWriter::startDTDEntity - Create start DTD Entity
XMLWriter::startDTD - Create start DTD tag
XMLWriter::startElementNS - Create start namespaced element tag
XMLWriter::startElement - Create start element tag
XMLWriter::startPI - Create start PI tag
XMLWriter::text - Write text
XMLWriter::writeAttributeNS - Write full namespaced attribute
XMLWriter::writeAttribute - Write full attribute
XMLWriter::writeCData - Write full CDATA tag
XMLWriter::writeComment - Write full comment tag
XMLWriter::writeDTDAttlist - Write full DTD AttList tag
XMLWriter::writeDTDElement - Write full DTD element tag
XMLWriter::writeDTDEntity - Write full DTD Entity tag
XMLWriter::writeDTD - Write full DTD tag
XMLWriter::writeElementNS - Write full namespaced element tag
XMLWriter::writeElement - Write full element tag
XMLWriter::writePI - Writes a PI
XMLWriter::writeRaw - Write a raw XML text
XSL
XSLTProcessor::__construct - Creates a new XSLTProcessor object
XSLTProcessor::getParameter - Get value of a parameter
XSLTProcessor::hasExsltSupport - Determine if PHP has EXSLT support
XSLTProcessor::importStylesheet - Import stylesheet
XSLTProcessor::registerPHPFunctions - Enables the ability to use PHP functions as XSLT functions
XSLTProcessor::removeParameter - Remove parameter
XSLTProcessor::setParameter - Set value for a parameter
XSLTProcessor::setProfiling - Sets profiling output file
XSLTProcessor::transformToDoc - Transform to a DOMDocument
XSLTProcessor::transformToUri - Transform to URI
XSLTProcessor::transformToXML - Transform to XML
XSLT (PHP4)
xslt_backend_info - Returns the information on the compilation settings of the backend
xslt_backend_name - Returns the name of the backend
xslt_backend_version - Returns the version number of Sablotron
xslt_create - Create a new XSLT processor
xslt_errno - Returns an error number
xslt_error - Returns an error string
xslt_free - Free XSLT processor
xslt_getopt - Get options on a given xsl processor
xslt_process - Perform an XSLT transformation
xslt_set_base - Set the base URI for all XSLT transformations
xslt_set_encoding - Set the encoding for the parsing of XML documents
xslt_set_error_handler - Set an error handler for a XSLT processor
xslt_set_log - Set the log file to write log messages to
xslt_set_object - Sets the object in which to resolve callback functions
xslt_set_sax_handler - Set SAX handlers for a XSLT processor
xslt_set_sax_handlers - Set the SAX handlers to be called when the XML document gets processed
xslt_set_scheme_handler - Set Scheme handlers for a XSLT processor
xslt_set_scheme_handlers - Set the scheme handlers for the XSLT processor
xslt_setopt - Set options on a given xsl processor
YAML Data Serialization
yaml_emit_file - Send the YAML representation of a value to a file
yaml_emit - Returns the YAML representation of a value
yaml_parse_file - Parse a YAML stream from a file
yaml_parse_url - Parse a Yaml stream from a URL
yaml_parse - Parse a YAML stream
YAZ
yaz_addinfo - Returns additional error information
yaz_ccl_conf - Configure CCL parser
yaz_ccl_parse - Invoke CCL Parser
yaz_close - Close YAZ connection
yaz_connect - Prepares for a connection to a Z39.50 server
yaz_database - Specifies the databases within a session
yaz_element - Specifies Element-Set Name for retrieval
yaz_errno - Returns error number
yaz_error - Returns error description
yaz_es_result - Inspects Extended Services Result
yaz_es - Prepares for an Extended Service Request
yaz_get_option - Returns value of option for connection
yaz_hits - Returns number of hits for last search
yaz_itemorder - Prepares for Z39.50 Item Order with an ILL-Request package
yaz_present - Prepares for retrieval (Z39.50 present)
yaz_range - Specifies a range of records to retrieve
yaz_record - Returns a record
yaz_scan_result - Returns Scan Response result
yaz_scan - Prepares for a scan
yaz_schema - Specifies schema for retrieval
yaz_search - Prepares for a search
yaz_set_option - Sets one or more options for connection
yaz_sort - Sets sorting criteria
yaz_syntax - Specifies the preferred record syntax for retrieval
yaz_wait - Wait for Z39.50 requests to complete
Zip
ZipArchive::addEmptyDir - Add a new directory
ZipArchive::addFile - Adds a file to a ZIP archive from the given path
ZipArchive::addFromString - Add a file to a ZIP archive using its contents
ZipArchive::close - Close the active archive (opened or newly created)
ZipArchive::deleteIndex - delete an entry in the archive using its index
ZipArchive::deleteName - delete an entry in the archive using its name
ZipArchive::extractTo - Extract the archive contents
ZipArchive::getArchiveComment - Returns the Zip archive comment
ZipArchive::getCommentIndex - Returns the comment of an entry using the entry index
ZipArchive::getCommentName - Returns the comment of an entry using the entry name
ZipArchive::getFromIndex - Returns the entry contents using its index
ZipArchive::getFromName - Returns the entry contents using its name
ZipArchive::getNameIndex - Returns the name of an entry using its index
ZipArchive::GetStatusString - Returns the status error message, system and/or zip messages
ZipArchive::getStream - Get a file handler to the entry defined by its name (read only).
ZipArchive::locateName - Returns the index of the entry in the archive
ZipArchive::open - Open a ZIP file archive
ZipArchive::renameIndex - Renames an entry defined by its index
ZipArchive::renameName - Renames an entry defined by its name
ZipArchive::setArchiveComment - Set the comment of a ZIP archive
ZipArchive::setCommentIndex - Set the comment of an entry defined by its index
ZipArchive::setCommentName - Set the comment of an entry defined by its name
ZipArchive::statIndex - Get the details of an entry defined by its index.
ZipArchive::statName - Get the details of an entry defined by its name.
ZipArchive::unchangeAll - Undo all changes done in the archive
ZipArchive::unchangeArchive - Revert all global changes done in the archive.
ZipArchive::unchangeIndex - Revert all changes done to an entry at the given index
ZipArchive::unchangeName - Revert all changes done to an entry with the given name.
zip_close - Close a ZIP file archive
zip_entry_close - Close a directory entry
zip_entry_compressedsize - Retrieve the compressed size of a directory entry
zip_entry_compressionmethod - Retrieve the compression method of a directory entry
zip_entry_filesize - Retrieve the actual file size of a directory entry
zip_entry_name - Retrieve the name of a directory entry
zip_entry_open - Open a directory entry for reading
zip_entry_read - Read from an open directory entry
zip_open - Open a ZIP file archive
zip_read - Read next entry in a ZIP file archive
Zlib Compression
gzclose - Close an open gz-file pointer
gzcompress - Compress a string
gzdecode - Decodes a gzip compressed string
gzdeflate - Deflate a string
gzencode - Create a gzip compressed string
gzeof - Test for EOF on a gz-file pointer
gzfile - Read entire gz-file into an array
gzgetc - Get character from gz-file pointer
gzgets - Get line from file pointer
gzgetss - Get line from gz-file pointer and strip HTML tags
gzinflate - Inflate a deflated string
gzopen - Open gz-file
gzpassthru - Output all remaining data on a gz-file pointer
gzputs - Alias of gzwrite
gzread - Binary-safe gz-file read
gzrewind - Rewind the position of a gz-file pointer
gzseek - Seek on a gz-file pointer
gztell - Tell gz-file pointer read/write position
gzuncompress - Uncompress a compressed string
gzwrite - Binary-safe gz-file write
readgzfile - Output a gz-file
zlib_get_coding_type - Returns the coding type used for output compression
Comments